The Microsoft Visual C++ Runtime is essential for running applications developed with Visual C++ and facilitates the execution of C++ programs without requiring full installation of the Visual Studio environment.
Here’s a simple code snippet demonstrating the use of a basic C++ command to display "Hello, Palworld!" on the console:
#include <iostream>
int main() {
std::cout << "Hello, Palworld!" << std::endl;
return 0;
}
Understanding Microsoft Visual C++ Runtime
What is Microsoft Visual C++ Runtime?
Microsoft Visual C++ Runtime is a set of dynamic link libraries (DLL) that C++ applications use to execute various functions. These libraries provide crucial elements like standard input/output processing, string manipulation, and memory management, which are essential for running applications smoothly.
The runtime environment allows developers to write code once and have it run across various systems, enhancing the portability of applications. In the context of gaming, the runtime helps maintain consistent performance and efficiency, which is vital for user experience.
Why is It Important for Game Development?
In game development, particularly with microsoft visual c++ runtime palworld, the importance of the runtime cannot be overstated. It serves as the backbone that supports game execution, allowing programmers to implement complex algorithms and processes needed for gameplay.
Common use cases of the runtime in gaming include handling graphics rendering, physics calculations, and player interactions. Each of these components is integral to creating a seamless gaming experience, devoid of lags or crashes, which is especially significant in a dynamic game like Palworld.
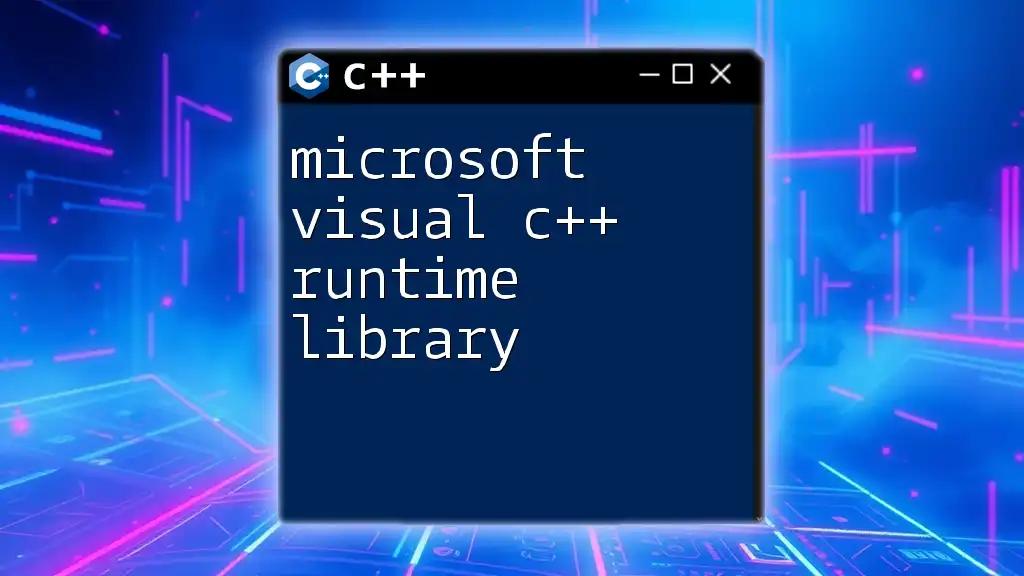
Palworld: A Brief Overview
What is Palworld?
Palworld is an innovative game that merges life simulation with open-world exploration and adventure. Players can capture and train creatures called "Pals," engage in farming, crafting, and combat. It's available on multiple platforms, including consoles and PC, drawing a wide audience with its unique blend of genres.
The Role of C++ in Palworld
C++ is the primary programming language in Palworld due to its high performance, which is crucial in resource-intensive environments. The language's benefits include fine-grained control over system resources, which is essential for rendering detailed graphics and ensuring smooth gameplay.
C++ allows developers to optimize their code for speed — critical when handling multiple in-game actions and interactions simultaneously.
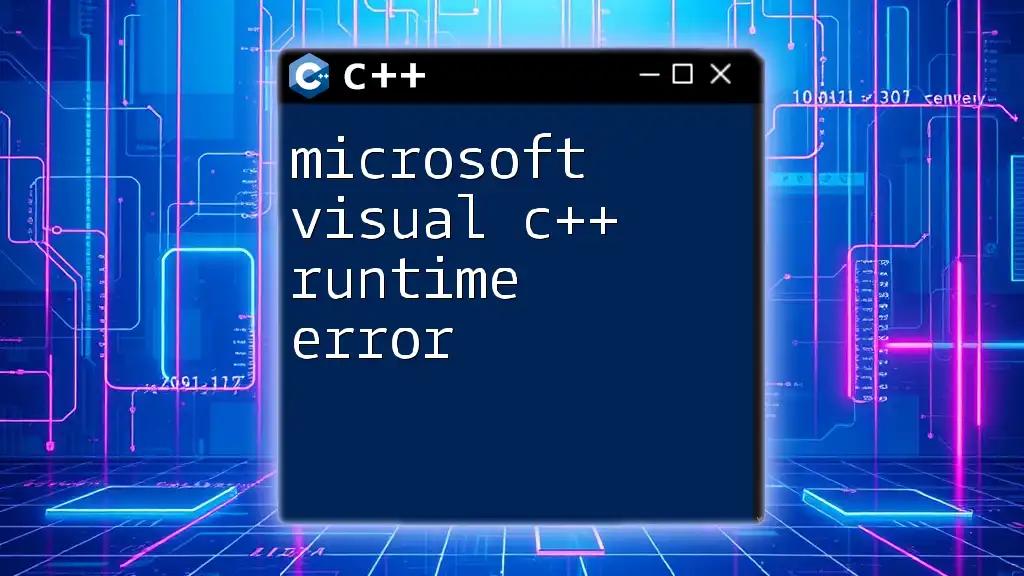
Setting Up Microsoft Visual C++ Runtime for Palworld
Minimum System Requirements
Before diving into microsoft visual c++ runtime palworld, it’s vital to ensure that your system meets the minimum requirements. These typically include:
- Operating System: Windows 10 or later
- Processor: Intel Core i5 or equivalent
- RAM: At least 8 GB
- Graphics: Dedicated GPU with DirectX 11 support
Installation Steps
To enjoy Palworld without issues related to runtime, follow these installation steps for Microsoft Visual C++ Redistributable:
- Go to the official Microsoft website.
- Search for the Visual C++ Redistributable package.
- Select the version compatible with your system (x86 for 32-bit or x64 for 64-bit).
- Download and run the installer, following the on-screen instructions.
Once installed, you can verify the installation by checking the app list in the Control Panel or using the Visual Studio installer options.

Common Issues and Troubleshooting
Runtime Errors in Palworld
Even with the proper setup, users may face various runtime errors while playing Palworld. Common errors include missing DLL files and compatibility issues, which can disrupt the gaming experience.
Solutions to Common Problems
Missing DLL Files
This issue frequently arises when essential runtime files are not available. The typical solution is to reinstall the Visual C++ Redistributable package.
If you encounter a specific DLL error, it might be helpful to either:
- Manually search for that DLL file online and place it in the game directory, or
- Use the Windows System File Checker (SFC) tool to restore missing files. You can run this tool by entering the following command into the Command Prompt:
sfc /scannow
Incompatibility Issues
If an older version of Visual C++ is causing issues, it’s advisable to uninstall it and install the latest version. It's crucial to maintain compatibility with the specific games and applications you plan to run. Additionally, check for game updates that might address such issues.
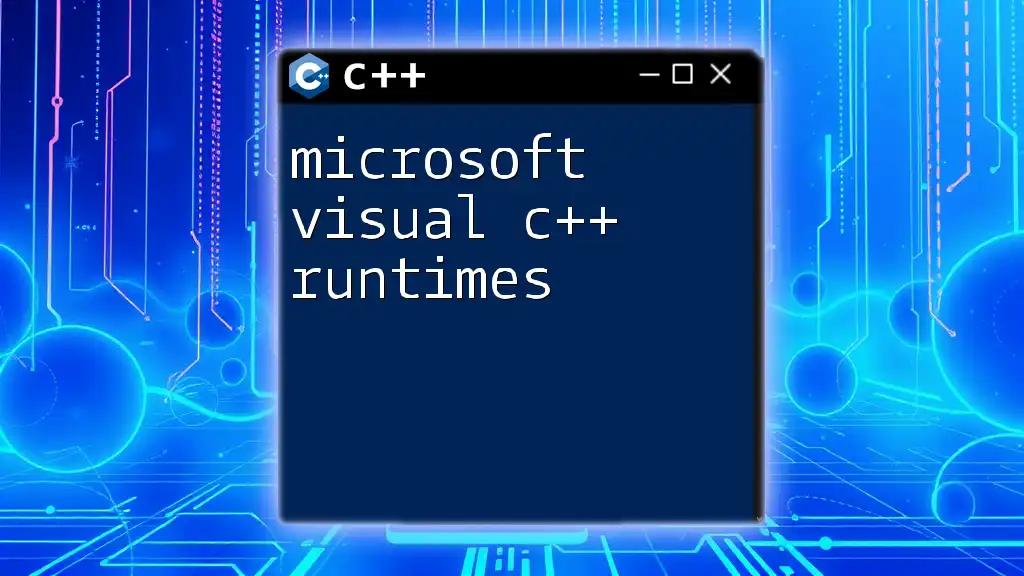
Best Practices for Using Microsoft Visual C++ in Palworld
Optimizing C++ Code for Performance
To ensure that your game runs seamlessly, optimizing C++ code is vital. Strategies include minimizing resource-heavy operations such as:
- Reducing unnecessary calculations in loops
- Using smart pointers for efficient memory management
Consider the following example demonstrating a basic loop optimization:
for (size_t i = 0; i < entityCount; ++i) {
// Perform action on entities.
entities[i]->update();
}
By minimizing computational overhead in performance-critical paths, you improve the overall game experience.
Debugging and Testing
Debugging is a critical part of the game development process. Tools such as the Visual Studio Debugger allow developers to set breakpoints, inspect variables, and track down logical errors.
For a simple debug environment setup, you can add a debugging statement:
std::cout << "Current Player Health: " << player.getHealth() << std::endl;
This helps track values during the game's execution, making identifying issues much easier.
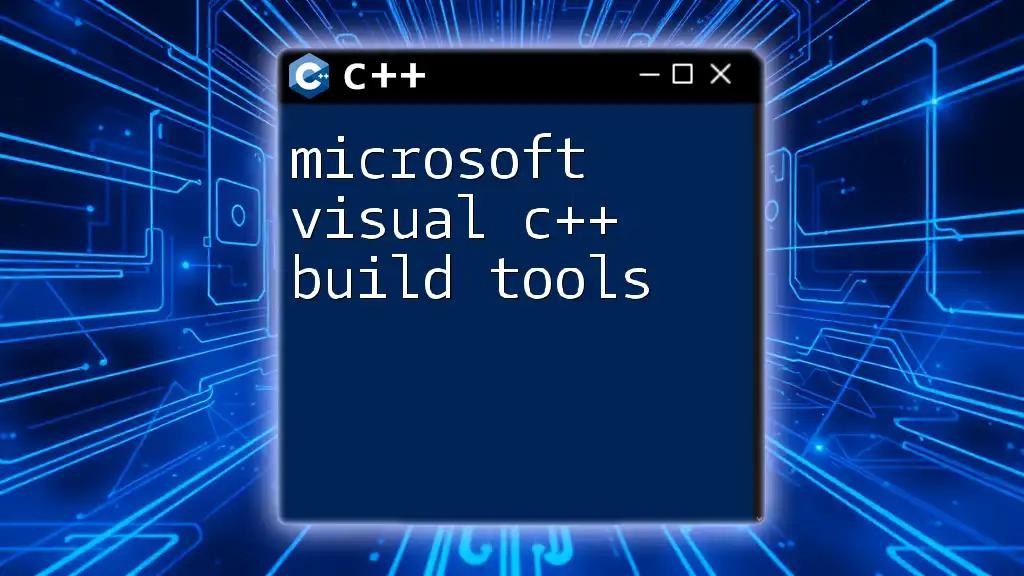
Advanced C++ Features Used in Palworld
Object-Oriented Programming Concepts
Object-Oriented Programming (OOP) is a cornerstone of C++ and plays a pivotal role in Palworld’s development. Key concepts of OOP include:
- Encapsulation: Keeps the internal state of objects hidden and exposes only necessary parts.
- Inheritance: Enables new classes to derive properties and behaviors from existing ones. This is useful for creating specialized creatures in Palworld.
For example:
class Pal {
public:
virtual void battle() = 0; // Pure virtual function
};
class FirePal : public Pal {
public:
void battle() override {
std::cout << "FirePal unleashes fire attack!" << std::endl;
}
};
Template Programming
Templates provide a way to write generic and reusable code, which is of great benefit in games needing to manage various types of objects dynamically. Here’s a simple example of using a template function:
template <typename T>
void addEntity(std::vector<T>& entities, T entity) {
entities.push_back(entity);
}
This allows the game to handle different entity types without rewriting function logic for each class.
Memory Management
Efficient memory management is crucial in game development to prevent leaks and ensure optimal performance. C++ provides direct control over memory allocation, giving developers the ability to create objects dynamically. Here’s an example illustrating the use of `new` and `delete`:
Pal* myPal = new FirePal(); // Memory allocated
// Use myPal...
delete myPal; // Memory released
By properly managing memory usage, developers can prevent issues like fragmentation and leaks, which can degrade gameplay performance over time.
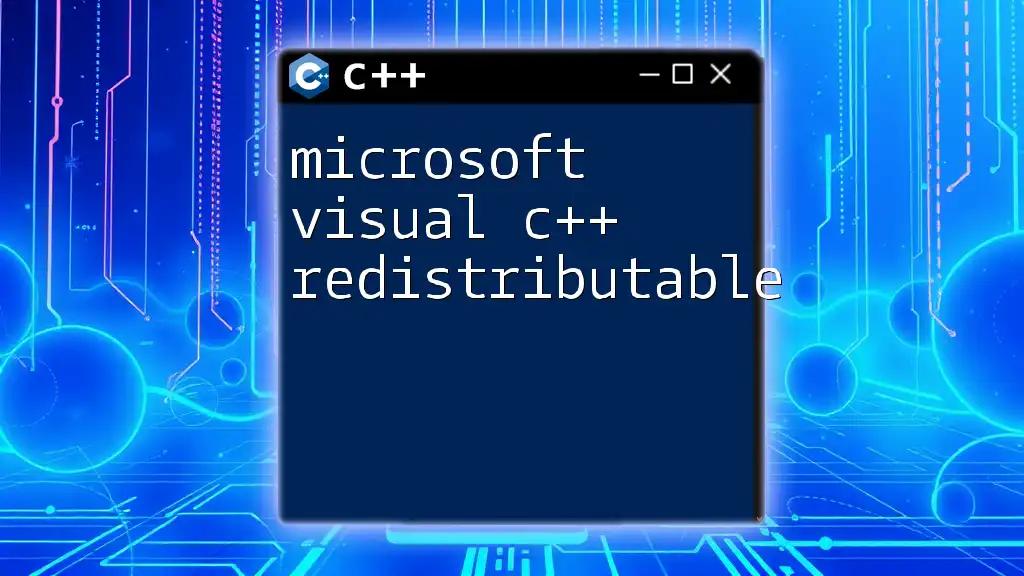
Conclusion
Understanding and utilizing the microsoft visual c++ runtime palworld is integral to navigating the complexities of game development. By optimizing C++ code, troubleshooting runtime issues, and employing advanced programming concepts, developers can enhance the gaming experience significantly. Embrace the challenges and explore the rich potential of C++ programming in shaping immersive and engaging gameplay in Palworld.

Additional Resources
For those looking to dive deeper into Microsoft Visual C++ and its applications in gaming, consider visiting the official Microsoft documentation, enrolling in recommended C++ courses, or engaging with online communities dedicated to C++ development. These resources offer valuable insights and guidance as you venture into the world of game programming.