Microsoft Visual C++ (MSVC) is an integrated development environment (IDE) from Microsoft that provides tools for developing C++ applications on Windows platforms.
Here’s a simple code snippet demonstrating how to print "Hello, World!" using MSVC:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is Microsoft Visual C++?
Microsoft Visual C++ is an integrated development environment (IDE) from Microsoft that allows developers to create applications in C and C++. As a part of the Visual Studio suite, it offers a user-friendly interface and a robust set of tools aimed at enhancing the coding experience. Visual C++ is essential for developers because it combines powerful language features with advanced tooling for building high-performance applications.
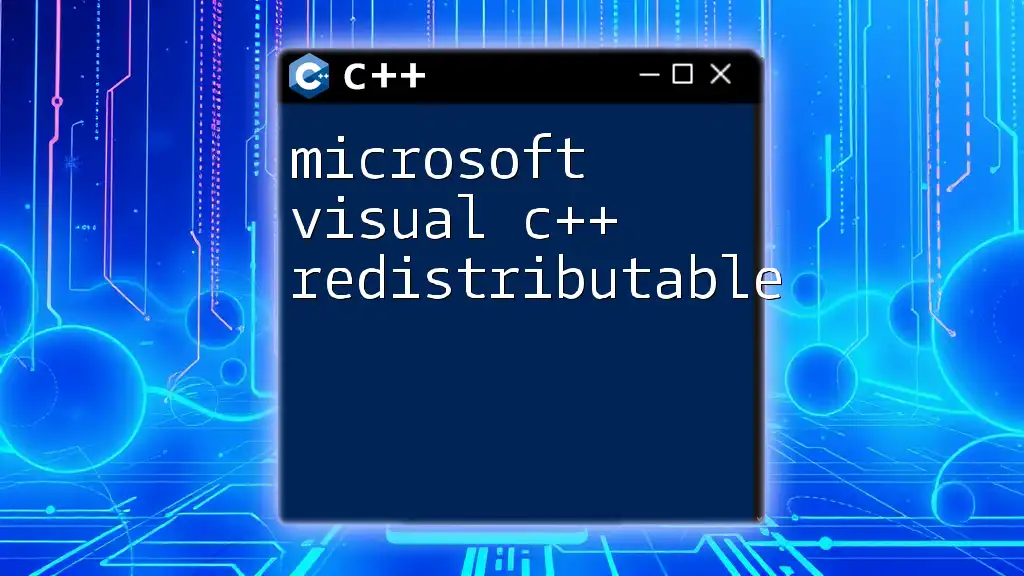
History and Evolution
The origins of Microsoft Visual C++ date back to 1993, with the release of Visual C++ 1.0. Over the years, Visual C++ has undergone significant transformations, introducing numerous features that have kept it relevant. Key releases include:
- Visual C++ 6.0 (1998) introduced MFC enhancements and a more intuitive IDE.
- Visual Studio .NET (2002) marked a transition to .NET architecture and support for managed code.
- Recent versions have incorporated support for modern C++ standards, such as C++11, C++14, and C++17.
These advancements not only improved the development process but also reinforced Visual C++ as a pillar in software development.
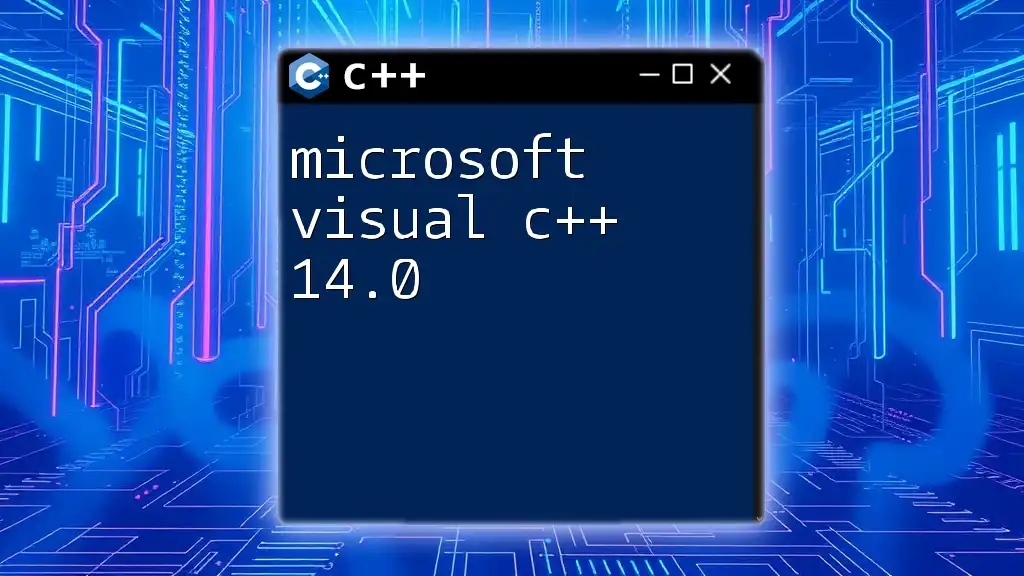
Getting Started with Microsoft Visual C++
System Requirements
To successfully run Microsoft Visual C++, you'll need to ensure your system meets the following minimum specifications:
- Operating System: Windows 10 or later.
- Processor: 1.8 GHz or faster.
- RAM: 2 GB minimum (8 GB recommended).
- Disk Space: Up to 35 GB for a full installation.
Installation Process
Installing Microsoft Visual C++ is a straightforward process:
- Download the installer for Visual Studio from the official Microsoft website.
- Launch the installer and select the "Desktop development with C++" workload.
- Follow the on-screen prompts to customize your installation (if desired) and complete the installation.
Common installation issues can include missing dependencies or insufficient disk space. Ensure you meet all requirements and, if necessary, clear up some storage before attempting installation.
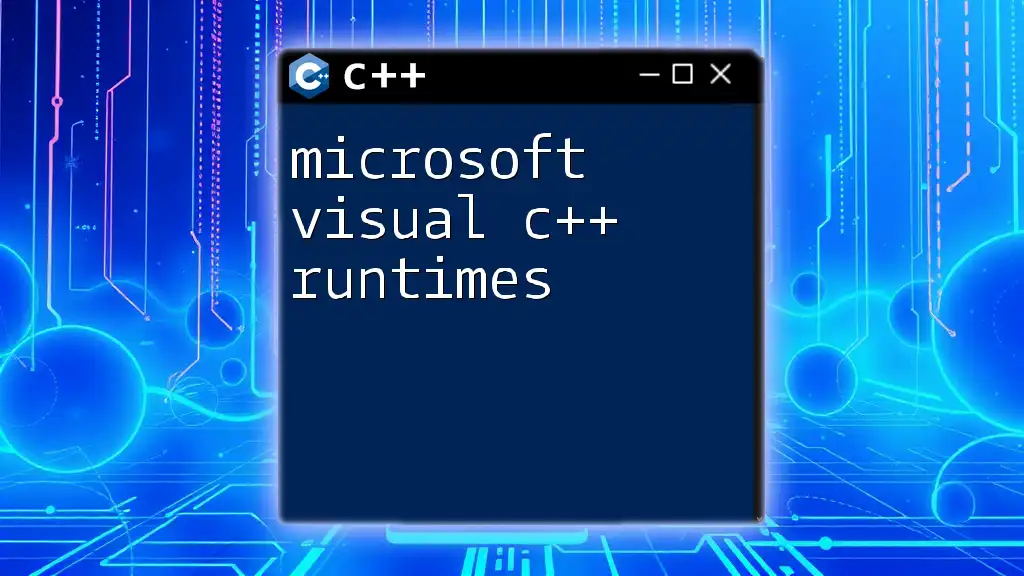
The Visual C++ IDE
Overview of the User Interface
The Visual C++ IDE presents a rich environment with several components designed to facilitate programming:
- Solution Explorer: Manages your projects and files.
- Editor Window: Where you'll write and edit your code.
- Toolbox: Provides various controls and components for your applications.
Each of these components is crafted to enhance your productivity, allowing seamless navigation and file management.
Customizing the IDE
Personalizing your development environment can boost productivity:
- Changing Themes: Access the options menu to switch between light and dark themes.
- Managing Projects: Organize files into solutions, making it easier to navigate larger codebases through custom project structures.
Customization options allow you to tailor the IDE to fit your workflow preferences.
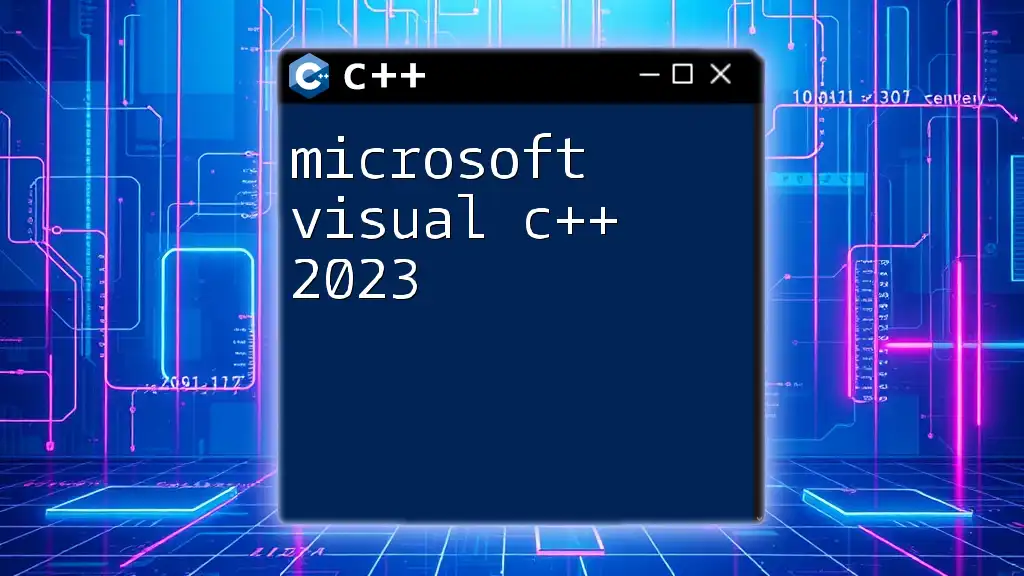
Creating Your First Project
Setting Up a New Project
Starting a new project is both exciting and essential for learning Visual C++. To create a console application:
- Open Visual Studio and select “Create a new project.”
- Choose the “Console App” template.
- Name your project and set its location.
- Click “Create” to finalize the setup.
Understanding Project Structure
Understanding the project structure is crucial for efficient navigation. Typically, you'll find:
- Source Files (.cpp): Hold your main application code.
- Header Files (.h): Contain declarations and function prototypes.
- Solution File (.sln): Represents the entire project collection.
Running Your First Program
Now, it’s time to write your first program. A classic starting point is to create a “Hello, World!” application:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
To run this code, hit the ‘Run’ button in the IDE or press F5. If all goes well, you'll see "Hello, World!" appear in your console window. This simple exercise helps you become familiar with the basic functionalities of Microsoft Visual C++.
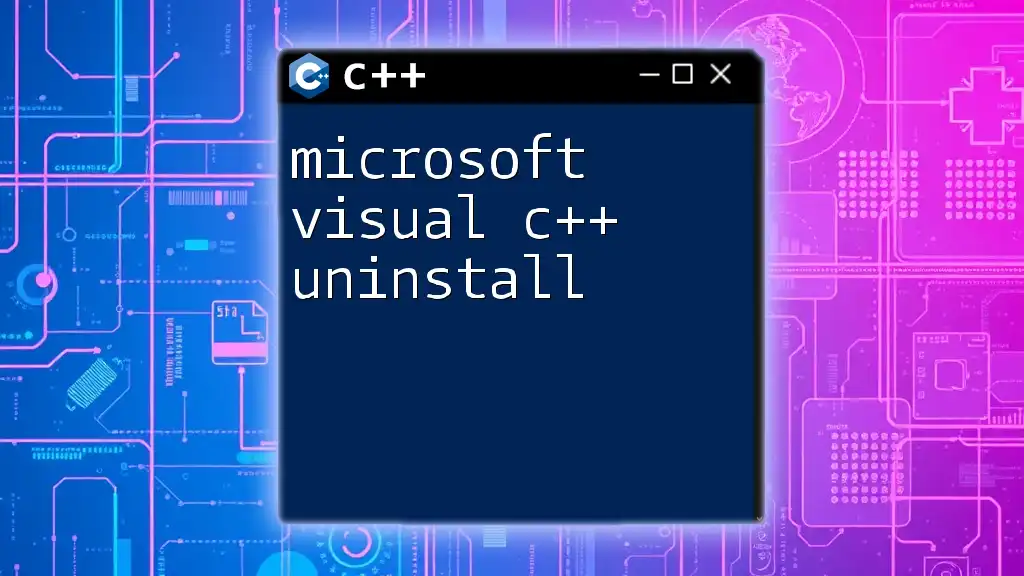
Key Features of Microsoft Visual C++
Built-in Debugger
Visual C++ comes equipped with a sophisticated debugging tool. You can:
- Set Breakpoints: Click on the margin next to the line number to pause execution.
- Step Through Code: Use F10/F11 to step over or into functions, making it easier to trace and diagnose issues in your applications.
Code Refactoring Tools
Visual C++ features tools that assist in improving and maintaining your codebase:
- Use the "Rename" feature to change variable names throughout your project seamlessly.
- The "Extract Method" feature simplifies code readability by breaking complex functions into smaller, named ones.
Smart Code Assistance
IntelliSense is a powerful tool built into Visual C++ that offers:
- Autocompletion: Automatically suggests possible completions based on context.
- Tooltips: Provides context-sensitive information about functions and variables as you type.
These features significantly enhance productivity by reducing boilerplate coding and preventing errors.
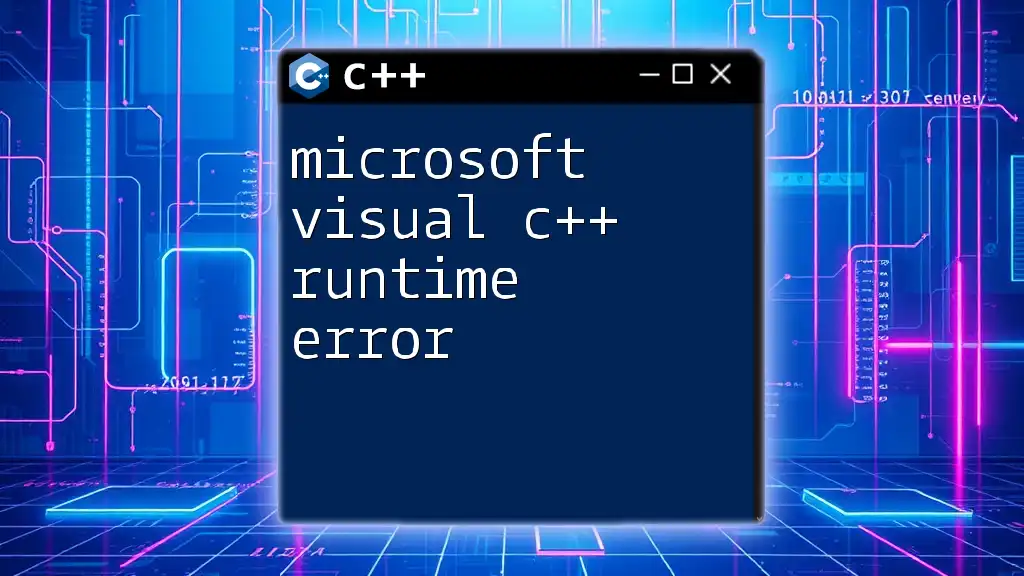
Working with Libraries and Dependencies
Understanding Libraries
Libraries are a fundamental aspect of C++ programming. They allow you to utilize pre-existing code for common tasks. The two main types of libraries are:
- Static Libraries: Linked at compile-time, resulting in a larger executable.
- Dynamic Libraries: Loaded at runtime, providing memory efficiency but requiring the library to be present.
Adding External Libraries
To add external libraries in Visual C++:
- Right-click on your project in Solution Explorer and select “Properties.”
- Navigate to “Linker” and then “Input.”
- Add the path to your library files.
Using the Standard Template Library (STL)
The STL is a powerful library in C++ that provides ready-to-use classes and functions. For example, let’s utilize a vector:
#include <vector>
#include <iostream>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
This simple program demonstrates how to store and manipulate dynamic arrays efficiently. Mastering the STL can greatly simplify coding routines and enhance performance in your applications.
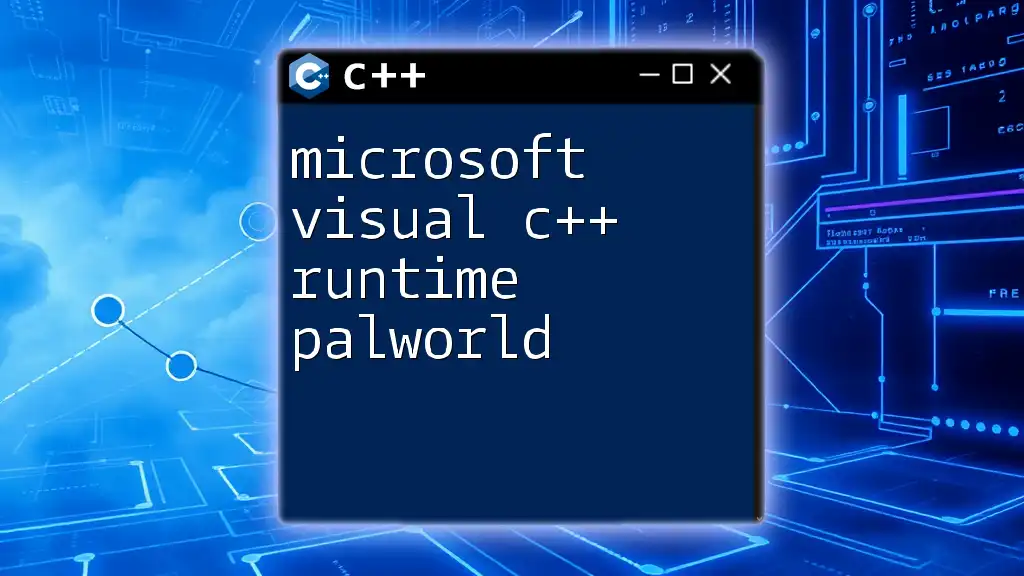
Advanced Features of Microsoft Visual C++
Profiling Tools
Performance profiling is essential for identifying bottlenecks in your application. Visual C++ provides:
- Built-in Profiling Tools: Monitor runtime behavior and memory usage.
- Diagnostic Tools: Analyze CPU and memory consumption to optimize your code.
Learning to use these tools effectively can significantly enhance the performance of your applications.
Unit Testing Support
Visual C++ offers integration with various unit testing frameworks, such as Google Test. To set up unit testing:
- Right-click on your solution and select “Add > New Project.”
- Choose the test project template and follow prompts to include it in your solution.
Unit testing helps ensure that your code behaves as expected, thus enhancing overall code quality.
Support for C++11/14/17
Microsoft Visual C++ keeps up to date with the latest C++ standards, including features like:
- Lambda Expressions: Allowing for inline function definitions.
- Smart Pointers: Helping with memory management.
- Auto Keyword: Enabling automatic type deduction.
This support is crucial for writing modern, efficient, and maintainable C++ code.
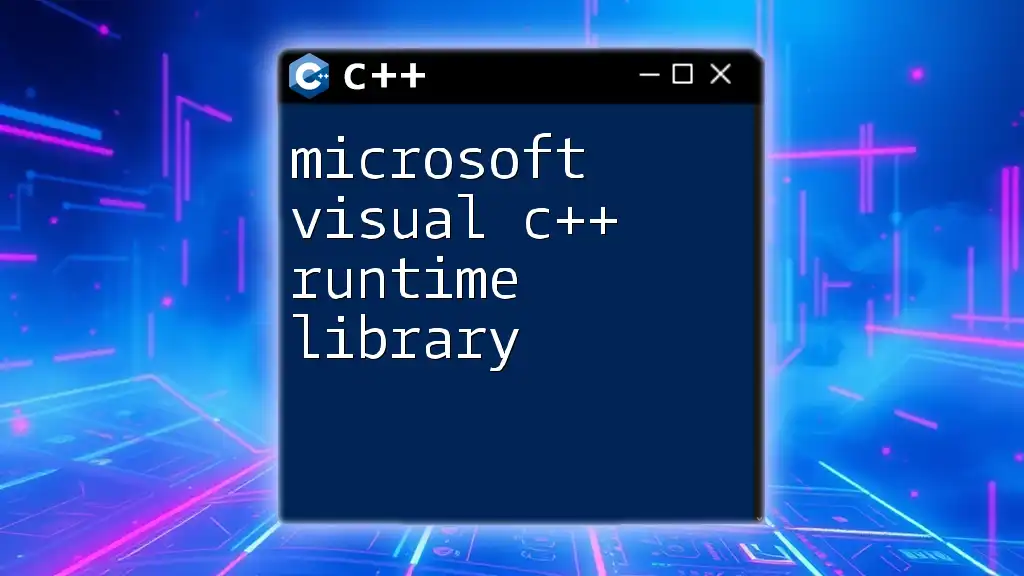
Common Pitfalls and Troubleshooting
Common Errors and Fixes
When working with Microsoft Visual C++, you might encounter various compilation errors.
- Missing Semicolons: This is one of the most common issues.
- Mismatched Parentheses or Braces: Always check your syntax.
Debugging is crucial; use the built-in tools to identify and resolve these issues.
Best Practices for Writing Clean Code
Maintaining clean and organized code is paramount. Follow general best practices:
- Use Descriptive Naming Conventions: Clearly name variables, functions, and classes to convey their purpose.
- Comment Your Code: Provide context for complex logic to make it easier for yourself and others to understand later.
Resources for Further Help
If you find yourself needing additional support, there are numerous resources available:
- Microsoft Documentation: Official guides and tutorials.
- Community Forums: Engage with communities such as Stack Overflow for questions and troubleshooting.
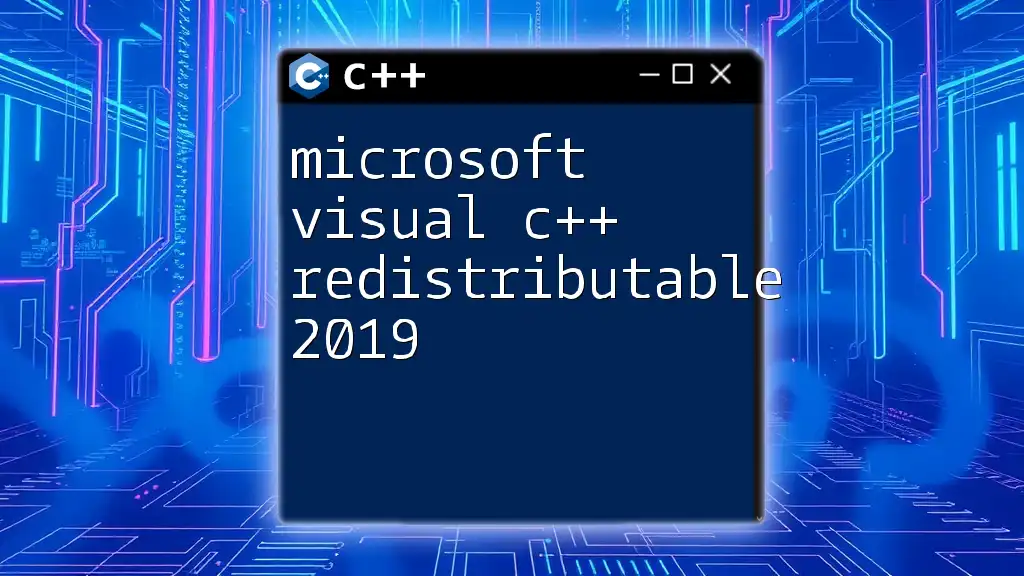
Conclusion
Mastering Microsoft Visual C++ opens doors to advanced programming techniques and high-performance application development. By leveraging its tools, IDE features, and libraries, you can enhance both your coding skills and productivity.
Next Steps
Embarking on your journey with Microsoft Visual C++ will lead you to deeper explorations of C++ programming. As you progress, consider joining classes or online communities to further cultivate your abilities in this powerful language.
Call to Action
Stay tuned for our upcoming courses where we break down Microsoft Visual C++ features! You can also sign up for our newsletter to receive valuable tips, tutorials, and resources straight to your inbox.
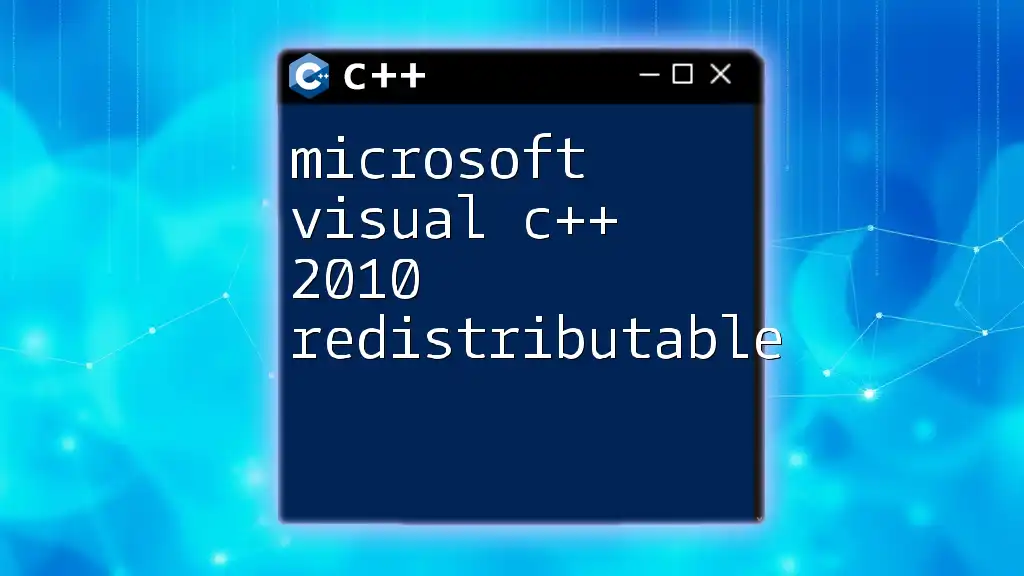
Additional Resources
To enhance your learning journey, consider these recommended resources:
- Books: Look for titles on C++ and Microsoft Visual C++ for a deeper understanding.
- Online Communities: Engage in forums such as Reddit’s r/cpp or other programming groups for support and discussion.