The "Microsoft Visual C++ 2022 x86 Minimum Runtime" refers to the essential runtime libraries required to run applications developed with Visual C++ 2022 targeting the x86 architecture, ensuring that the necessary components are available on the system for proper execution.
Here’s a simple code snippet demonstrating the use of a basic C++ program with a simple output:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is Minimum Runtime?
Definition of Minimum Runtime
In the context of Microsoft Visual C++, minimum runtime refers to a streamlined set of libraries and components that are essential for executing C++ applications. It reduces the amount of code and dependencies required, thus allowing developers to create lightweight applications that can run with minimal system overhead.
Importance of Minimum Runtime
The significance of minimum runtime revolves around several key points:
-
Dependency Management: By focusing on a minimal set of libraries, developers can simplify dependency management. This ensures that applications are less prone to runtime errors caused by missing or incompatible libraries.
-
Performance Optimization: Applications built with minimum runtime tend to perform better. This is because they utilize fewer resources, making them quicker to load and execute, which is particularly beneficial for applications running on limited hardware.
-
Compatibility: Minimum runtime libraries are designed to provide essential support across a wide range of systems. This improves compatibility, allowing applications to run smoothly across different environments without requiring extensive additional installations.
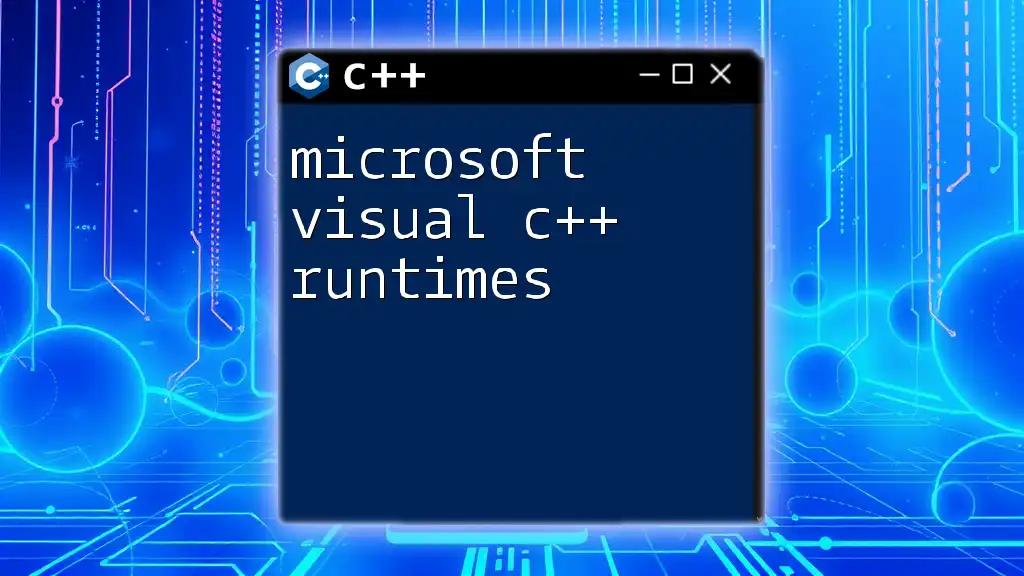
Installing Microsoft Visual C++ 2022
System Requirements
Before installing Microsoft Visual C++ 2022, ensure that your system meets the following requirements:
-
Hardware Requirements: A computer with a compatible processor and at least 4 GB of RAM is recommended for optimal performance.
-
Software Prerequisites: Make sure your operating system is up to date—Windows 10 or later is ideal for using Visual C++ 2022 effectively.
Installation Steps
To install Microsoft Visual C++ 2022, follow these straightforward steps:
-
Download the Installer: Visit the official Microsoft website and download the Visual Studio Installer. Choosing the Community version is a good starting point, as it's free for individual developers.
-
Launch the Installer: Run the downloaded installer and select the components you wish to install.
-
Choosing Minimum Runtime Options: During the installation process, look for options pertaining to minimal runtime. This ensures that only the necessary runtime libraries are included.
Here's a simplified view of what to look for in the installation screen:
- C++ Desktop Development: Check this option to ensure you have the necessary tools.
- Minimum Runtime Libraries: Opt for this to limit the installation to only essential components.

Configuring Minimum Runtime in Visual C++
Setting Up Your Project
Once you have Visual C++ installed, it’s time to set up your project. Follow these steps to create a new C++ project:
- Launch Visual Studio and click on Create a new project.
- Select C++ from the available languages and choose Console Application to start with a basic structure.
You might see a boilerplate code similar to the following:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Configuring Project Properties
Next, you need to adjust the project properties to use minimal runtime:
-
Accessing Project Properties: Right-click on your project name in the Solution Explorer and choose Properties.
-
Setting the Runtime Library: Under C/C++ > Code Generation, you can specify the runtime library your application will use. Opt for the `/MT` option to utilize the Multi-threaded runtime, which is optimized for performance.
Here’s how you can do this in code:
#pragma comment(linker, "/MT") // Using Multi-threaded runtime
By setting this configuration, you ensure that the project takes advantage of the reduced overhead associated with the minimum runtime.
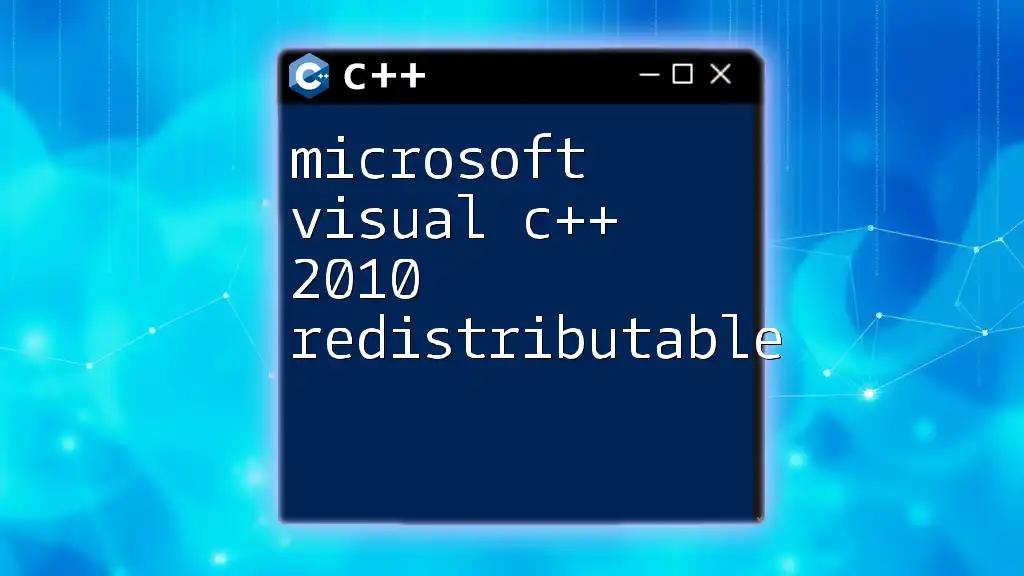
Understanding Minimum Runtime Libraries
Overview of Runtime Libraries
Runtime libraries are essential components that provide the necessary support for executing C++ programs. They offer basic functionalities like input/output operations, memory management, and error handling.
Key Libraries and Their Functions
In Microsoft Visual C++ 2022, several core libraries come into play with the x86 minimum runtime:
-
C Runtime (CRT): This forms the backbone of C++ applications, handling input/output operations and various runtime functions. It provides a standard way to perform tasks common across different codebases (e.g., string manipulation, file operations).
-
Standard Template Library (STL): The STL includes a powerful set of C++ libraries that allow developers to utilize data structures (like vectors and lists) and algorithms (such as sorting). It enhances code efficiency and readability.
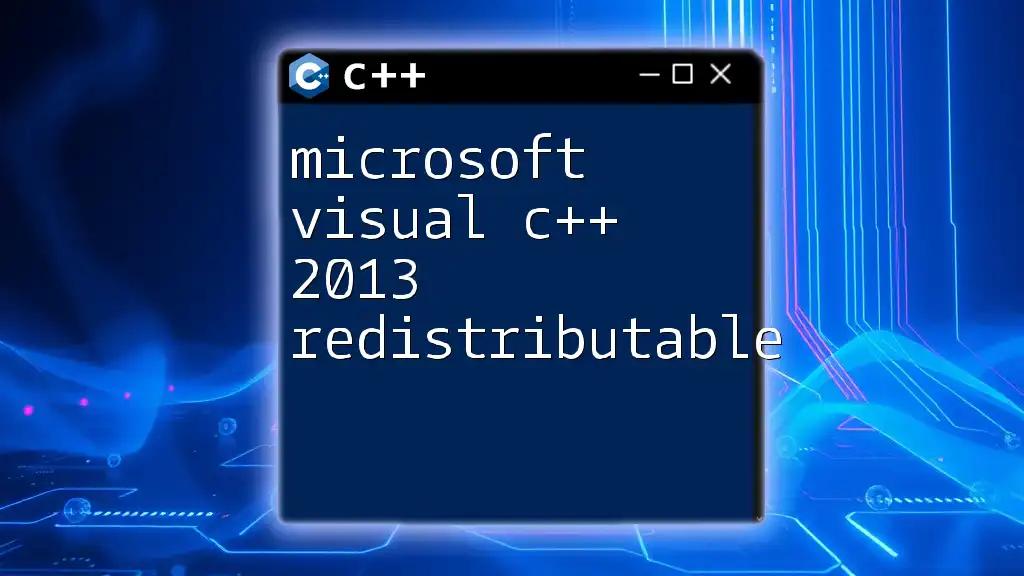
Developing with Minimum Runtime: Best Practices
Writing Efficient Code
When developing applications using the minimum runtime, it’s crucial to adhere to best practices to enhance efficiency and performance:
-
Avoiding Memory Leaks: One of the common pitfalls in C++ programming is memory management. Use smart pointers or ensure proper deletion of allocated memory to prevent leaks.
-
Optimizing Performance: Strive for optimal code logic. Avoid redundancy in your algorithms and utilize STL when appropriate to keep your codebase clean and efficient.
Testing Your Application
Effective testing is essential to ensure your application runs smoothly when utilizing minimum runtime:
- Debugging Techniques: Incorporate logging or assert statements to help detect and analyze runtime issues promptly. For example:
#include <cassert>
int main() {
int a = 5;
assert(a > 0); // This will raise an error if 'a' is not greater than 0.
return 0;
}
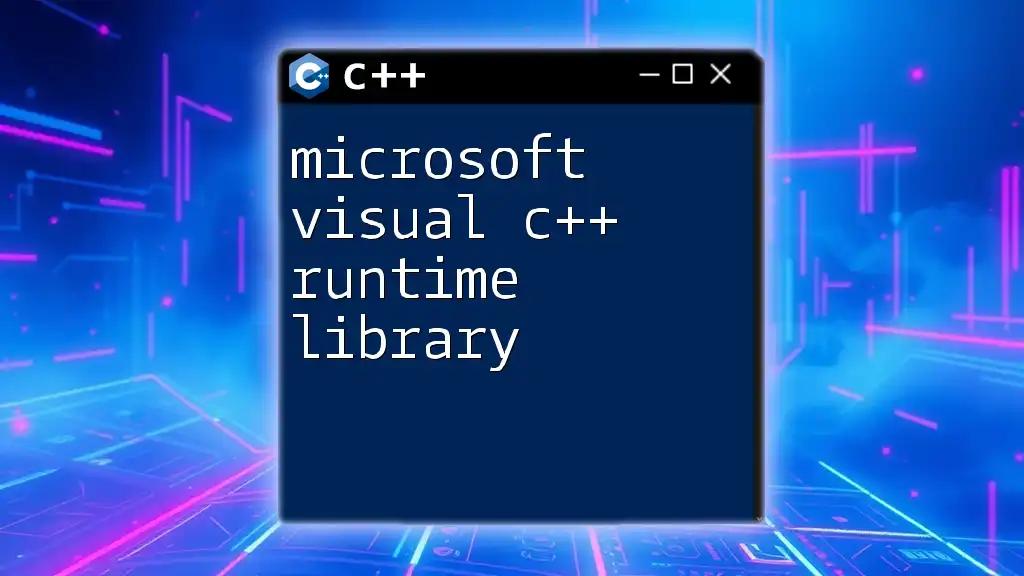
Distributing Applications with Minimum Runtime
Packaging Your Application
When it's time to distribute your application, packaging it correctly is vital:
- Including Runtime Libraries: If your application requires specific runtime libraries to function, ensure you include them in your installer package. This avoids the "missing DLL" issues that many users face.
Deployment Considerations
As part of deployment, several factors should be considered:
-
Target Systems: Ensure that you test your application on various hardware configurations and operating systems. It will help identify any compatibility issues beforehand.
-
Version Control: Consistency between development environments and production servers is crucial. Utilize version control systems like Git to manage changes effectively.

Common Issues and Troubleshooting
Common Errors
While working with the minimum runtime in Microsoft Visual C++, you may encounter various errors:
-
Runtime Errors: These may arise from misconfigured runtime environments. Ensure all necessary components are present at both development and deployment stages.
-
Dependency Issues: If an application crashes due to a missing dependency, double-check that all required libraries are packaged correctly. Utilizing tools like Dependency Walker can assist in identifying missing DLLs.
Resources for Further Help
For continued learning and troubleshooting, consider these resources:
-
Official Microsoft Documentation: The Microsoft Docs site offers a wealth of information on Visual C++ and its runtime libraries.
-
Community Forums: Engaging with platforms like Stack Overflow or the Microsoft Developer Community can provide additional insights and solutions from fellow C++ developers.
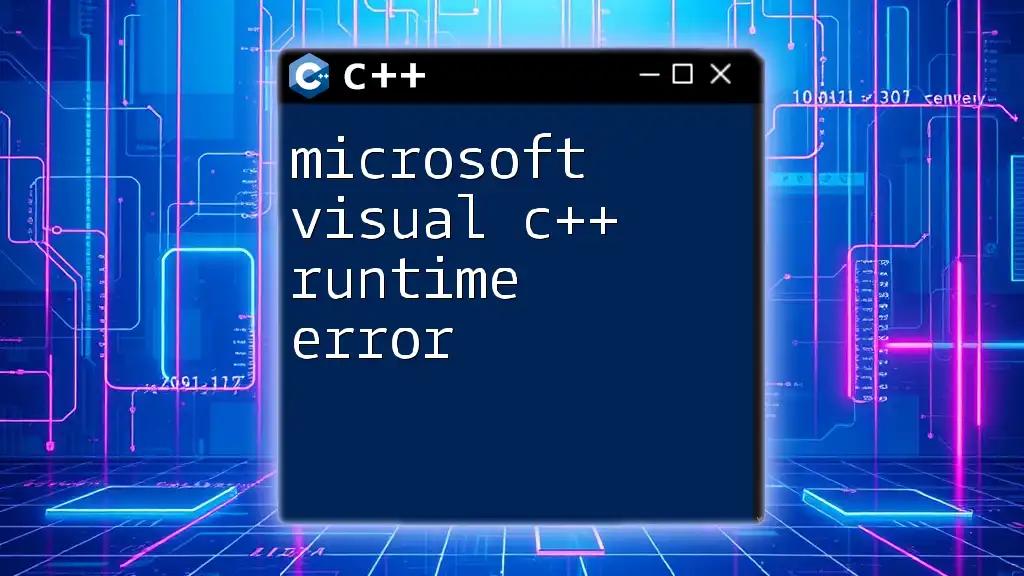
Conclusion
Understanding the Microsoft Visual C++ 2022 x86 minimum runtime is crucial for developing efficient and reliable applications. By embracing minimum runtime principles, you can significantly enhance your development process, streamline your applications, and ensure broader compatibility across different systems.
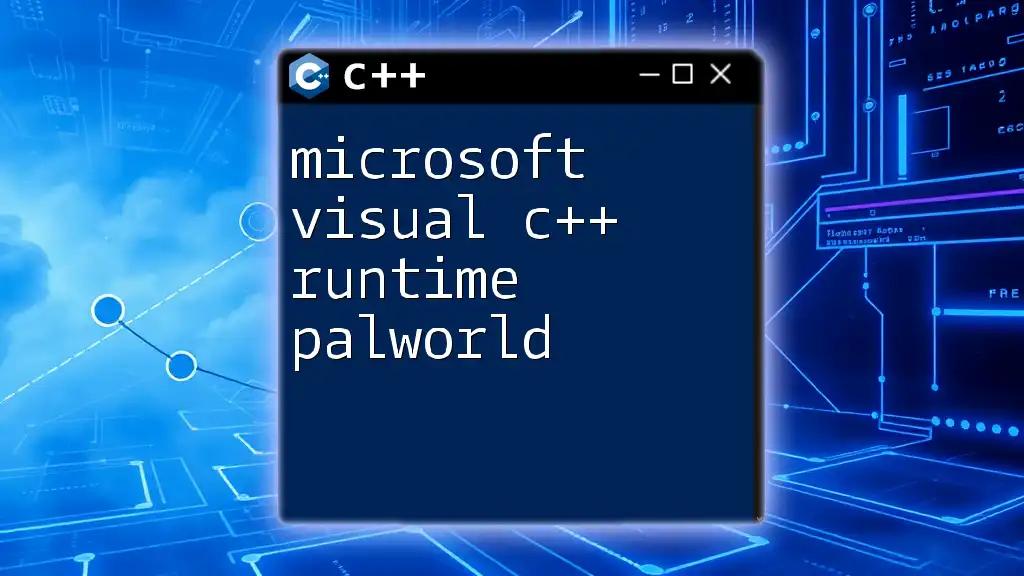
Call to Action
Ready to deepen your C++ skills? Join our community to access exclusive resources and courses designed for aspiring developers. Start learning today, and transform your approach to C++ programming!