Quick sort is an efficient, divide-and-conquer sorting algorithm that partitions an array into subarrays based on a pivot element, recursively sorting the subarrays.
Here's a simple implementation in C++:
#include <iostream>
using namespace std;
void quickSort(int arr[], int low, int high) {
if (low < high) {
int pivot = arr[high];
int i = (low - 1);
for (int j = low; j < high; j++) {
if (arr[j] < pivot) {
i++;
swap(arr[i], arr[j]);
}
}
swap(arr[i + 1], arr[high]);
int pi = i + 1;
quickSort(arr, low, pi - 1);
quickSort(arr, pi + 1, high);
}
}
int main() {
int arr[] = {10, 7, 8, 9, 1, 5};
int n = sizeof(arr)/sizeof(arr[0]);
quickSort(arr, 0, n-1);
cout << "Sorted array: ";
for (int i = 0; i < n; i++)
cout << arr[i] << " ";
return 0;
}
Understanding Quick Sort Algorithm in C++
Quick sort is a highly efficient sorting algorithm and is based on the divide-and-conquer principle. It operates by dividing a large array into smaller sub-arrays, then recursively sorting those sub-arrays. This recursive approach helps achieve a time complexity that, in the best and average cases, is O(n log n), while the worst-case scenario is O(n²). However, with good pivot selection, the worst-case scenario can often be avoided.
The quick sort algorithm functions as follows:
- Choose a pivot element from the array.
- Partition the array into two halves based on the pivot.
- Recursively apply the quick sort to the sub-arrays formed by the partitioning step.
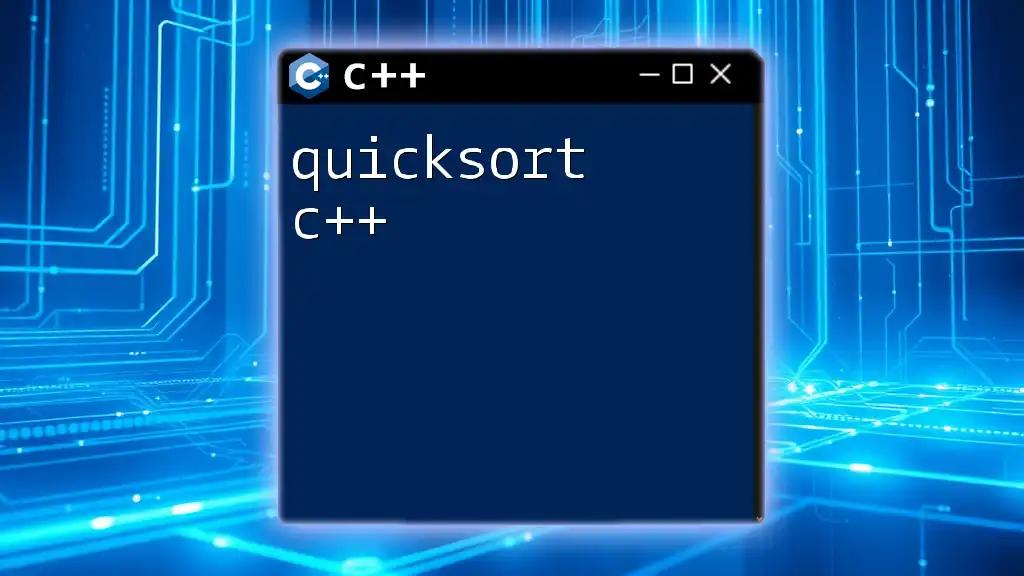
Implementing the C++ Quicksort Algorithm
To implement the quick sort algorithm efficiently in C++, we need to ensure our development environment is set up for C++ coding. The basic structure of our algorithm will involve a function named `quickSort`, which calls another function for partitioning the array.
Here’s a basic outline of the structure:
void quickSort(int arr[], int low, int high) {
// Implementation details here
}
Partition Function Explained
The partition function plays a vital role in the quick sort algorithm. It rearranges the elements in the array so that elements less than the pivot are on its left, and those greater are on its right. The pivot itself is now in its correct sorted position.
Here’s a sample implementation of the `partition` function:
int partition(int arr[], int low, int high) {
int pivot = arr[high]; // Choose the last element as pivot
int i = (low - 1); // Index of smaller element
for (int j = low; j < high; j++) {
if (arr[j] < pivot) {
i++;
swap(arr[i], arr[j]); // Swap if current element is smaller than pivot
}
}
swap(arr[i + 1], arr[high]); // Place pivot in the correct position
return (i + 1); // Return index of the pivot
}

Step-by-Step Breakdown of Quick Sort in C++
Choosing a Pivot
Choosing an appropriate pivot is critical in achieving optimal performance. The way we select the pivot can drastically affect the efficiency of quick sort. Common strategies include choosing:
- The first element.
- The last element.
- A random element.
- The median of three (first, middle, last elements).
Finding a balance in pivot selection can help to maintain efficiency, particularly in cases where values are already sorted or contain many duplicates.
Recursive Nature of Quick Sort
The recursive aspect of quick sort allows it to sort the array in smaller chunks. In the quick sort function, it checks if the current sub-array has more than one element to sort. If so, it splits the array and recursively sorts the left and right sides:
if (low < high) {
int pi = partition(arr, low, high); // Partitioning the array
quickSort(arr, low, pi - 1); // Recursively sort left side
quickSort(arr, pi + 1, high); // Recursively sort right side
}
This recursive approach is what makes quick sort robust and versatile.

Advantages and Disadvantages of Quick Sorting in C++
Benefits of Quick Sort
- Average-case performance: Quick sort is often faster than other sorting algorithms, such as merge sort and heap sort because it has good locality of reference and less overhead for small datasets.
- In-place sorting: The quick sort algorithm does not require additional space for sorting, having low memory overhead.
- Cache efficiency: Because of how it works, quick sort makes better use of the memory cache which boosts the performance significantly.
Drawbacks of Quick Sort
- Worst-case performance: In cases of already sorted data or when all elements are identical, the performance can degrade to O(n²).
- Not stable: Quick sort is not a stable sorting algorithm, which means that equal elements may not retain their relative positions after sorting.
- Inefficient for small datasets: For small arrays, other algorithms, like insertion sort, can outperform quick sort due to lower overhead.

Optimizing the Quick Sort C++ Algorithm
To enhance the efficiency of the quick sort implementation, various optimizations can be applied:
- Hybrid Approach: You might switch to a different sorting algorithm for small sub-arrays (e.g., insertion sort) to speed things up.
- Tail Recursion Optimization: Use iteration for one side of the recursion — this can help reduce the call stack and improve performance.
- Randomized Pivot: Randomly selecting a pivot can help mitigate the risk of hitting the worst-case performance in sorted or nearly sorted arrays.

Example Use Case: Sorting an Array using C++ Quick Sort
Here’s a complete example demonstrating the quick sort algorithm in C++:
#include <iostream>
using namespace std;
void quickSort(int arr[], int low, int high);
int partition(int arr[], int low, int high);
int main() {
int arr[] = {10, 7, 8, 9, 1, 5};
int n = sizeof(arr) / sizeof(arr[0]);
quickSort(arr, 0, n - 1);
cout << "Sorted array: \n";
for (int i = 0; i < n; i++)
cout << arr[i] << " ";
return 0;
}
void quickSort(int arr[], int low, int high) {
if (low < high) {
int pi = partition(arr, low, high);
quickSort(arr, low, pi - 1);
quickSort(arr, pi + 1, high);
}
}
int partition(int arr[], int low, int high) {
int pivot = arr[high];
int i = (low - 1);
for (int j = low; j < high; j++) {
if (arr[j] < pivot) {
i++;
swap(arr[i], arr[j]);
}
}
swap(arr[i + 1], arr[high]);
return (i + 1);
}
In this example, an array of integers is sorted using the quick sort algorithm, demonstrating how straightforward it can be to implement this powerful sorting technique in C++.
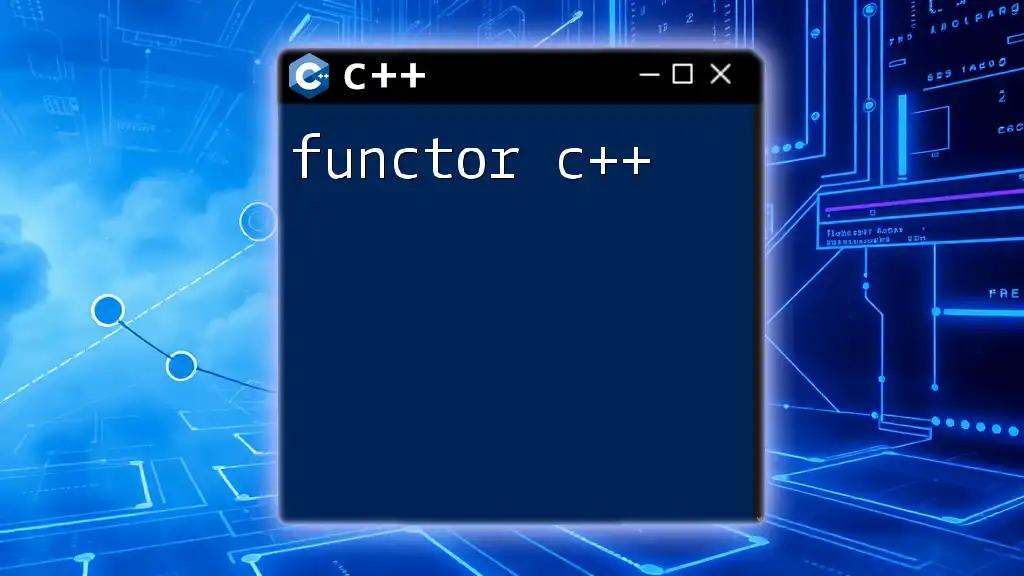
Real-World Applications of Quick Sort C++
The quick sort C++ algorithm is commonly used in various applications, particularly in situations where:
- Large datasets require quick access and sorting capabilities, such as databases or file systems.
- Implementing sorting functions in libraries where performance is critical.
- Developing algorithms for search engines, where data needs to be indexed efficiently.
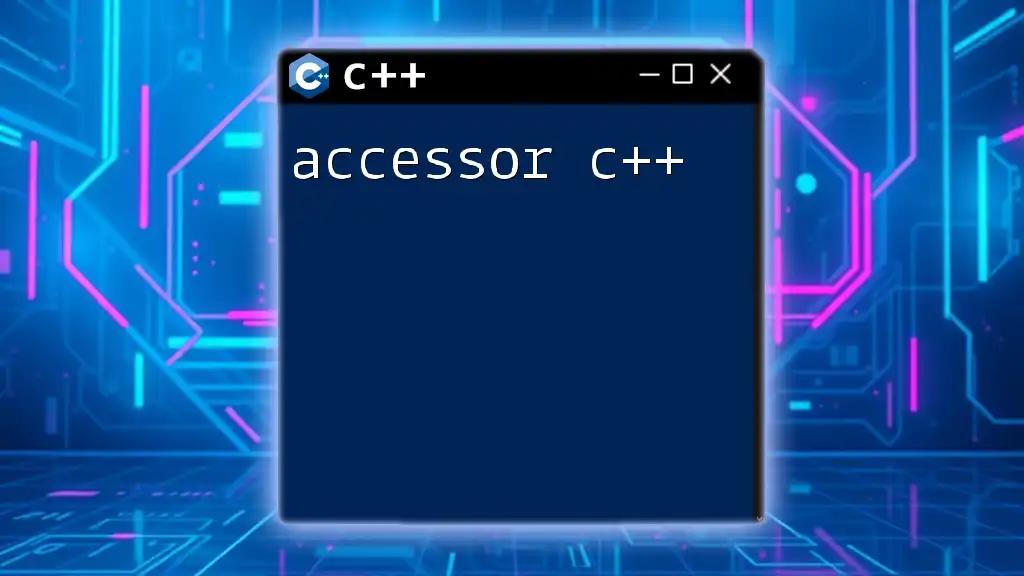
Conclusion
Quick sort is a robust and efficient sorting algorithm with significant advantages if implemented properly. By understanding its mechanics and continuously experimenting with pivot selection and optimization techniques, programmers can leverage quick sort to efficiently handle various data sorting tasks effectively.
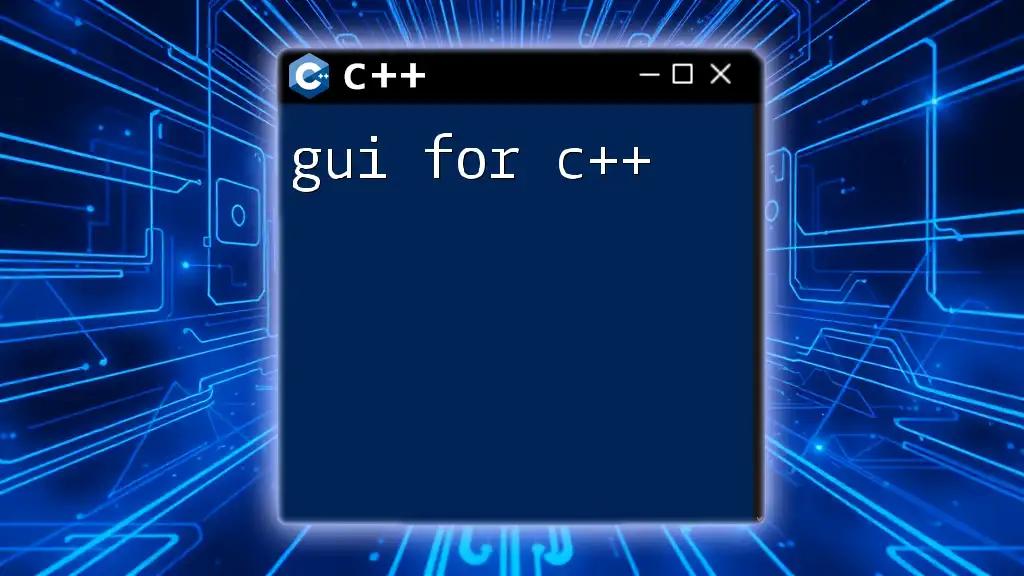
Additional Resources
To deepen your understanding of the quick sort algorithm and C++ programming in general, consider pursuing books focused on algorithms, online courses dedicated to C++ development, and tutorials that provide further insights into advanced sorting techniques.