Quicksort is an efficient sorting algorithm that uses a divide-and-conquer approach to sort an array by selecting a 'pivot' element and partitioning the other elements into those less than or greater than the pivot.
#include <iostream>
#include <vector>
void quicksort(std::vector<int>& arr, int low, int high) {
if (low < high) {
int pivot = arr[high];
int i = low - 1;
for (int j = low; j < high; j++) {
if (arr[j] < pivot) {
i++;
std::swap(arr[i], arr[j]);
}
}
std::swap(arr[i + 1], arr[high]);
int partitionIndex = i + 1;
quicksort(arr, low, partitionIndex - 1);
quicksort(arr, partitionIndex + 1, high);
}
}
int main() {
std::vector<int> data = {10, 7, 8, 9, 1, 5};
quicksort(data, 0, data.size() - 1);
for (int num : data) std::cout << num << " ";
return 0;
}
What is Quicksort?
Quicksort is a highly efficient sorting algorithm that employs a divide-and-conquer strategy to sort elements in an array or list. First developed by British computer scientist Tony Hoare in 1960, Quicksort is celebrated for its efficiency and ability to handle large datasets.
When compared to other sorting algorithms like Bubble Sort or Merge Sort, Quicksort often outperforms them, particularly for medium to large-sized datasets. Its average-time complexity is O(n log n), making it a go-to choice for many applications.
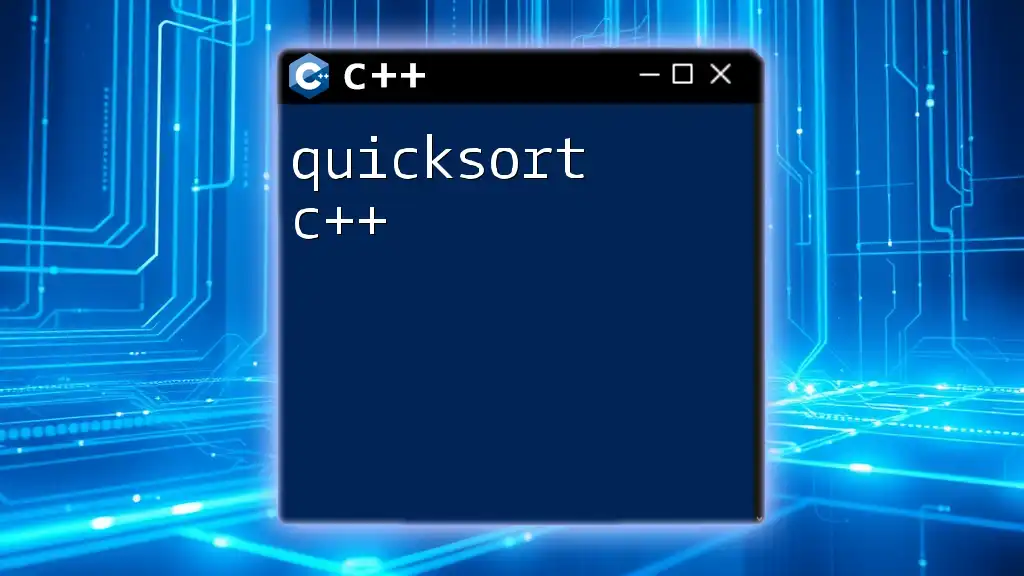
How Quicksort Works
The Basic Idea
At the heart of Quicksort lies the concept of the pivot. The algorithm selects a pivot element from the array; this element determines how the array will be partitioned. The key is that all elements less than the pivot are moved to its left, and all elements greater than the pivot are moved to its right, effectively dividing the data into two sub-arrays.
Steps Involved in Quicksort
The steps involved in executing Quicksort are as follows:
-
Selecting the Pivot: A pivot element is chosen from the array. Different strategies exist for selecting the pivot, such as choosing the first element, last element, or even the median.
-
Partitioning the Array: This process rearranges the elements around the pivot. After partitioning, the pivot is in its final position.
-
Recursively Sorting the Partitions: With the pivot in place, the two sub-arrays (elements left of the pivot and elements right of the pivot) are then sorted independently through the same Quicksort process.
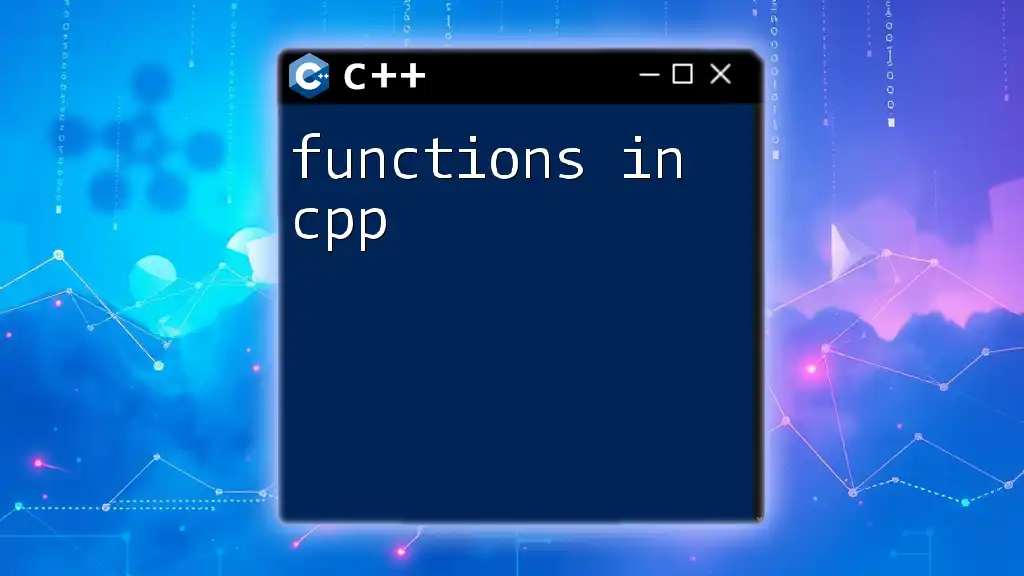
Visualizing Quicksort
Step-by-Step Visualization
Understanding Quicksort can be simplified through visualization. Imagine you have the unsorted array:
[10, 7, 8, 9, 1, 5]
- Choose a pivot (say, the last element, 5).
- Partition the array so elements less than 5 move to the left and those greater move to the right, resulting in:
[1, 5, 8, 9, 10, 7] (5 is now in its correct position)
- The process continues, applying Quicksort first to the left sub-array `[1]` (which is already sorted) and then to the right sub-array `[8, 9, 10, 7]` until all sub-arrays are processed.
Example of Quicksort in Action
Taking the previous array example, the complete process would look like this:
- Initial array: `[10, 7, 8, 9, 1, 5]`
- Choose pivot (5), partition: `[1, 5, 8, 9, 10, 7]`
- Left sub-array `[1]`: already sorted
- Process right sub-array `[8, 9, 10, 7]` with pivot (7):
[5] + [7, 8, 9, 10]
=> Pivot (7), partition: [5, 7, 8, 9, 10]
Finally, you end up with a sorted array:
[1, 5, 7, 8, 9, 10]
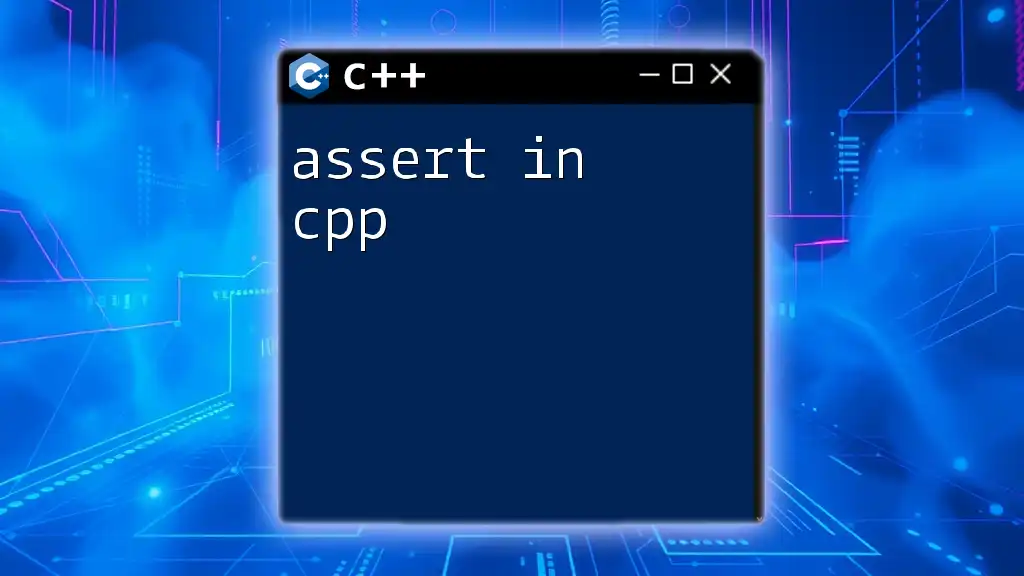
Implementation of Quicksort in C++
Basic Implementation
Here’s a straightforward implementation of Quicksort in C++:
#include <iostream>
void swap(int &a, int &b) {
int temp = a;
a = b;
b = temp;
}
int partition(int arr[], int low, int high) {
int pivot = arr[high]; // pivot
int i = (low - 1); // Index of smaller element
for (int j = low; j < high; j++) {
if (arr[j] < pivot) {
i++; // increment index of smaller element
swap(arr[i], arr[j]);
}
}
swap(arr[i + 1], arr[high]);
return (i + 1);
}
void quickSort(int arr[], int low, int high) {
if (low < high) {
int pi = partition(arr, low, high);
// Recursively sort elements before partition and after partition
quickSort(arr, low, pi - 1);
quickSort(arr, pi + 1, high);
}
}
Explanation of Each Function
- swap: A utility function that swaps two integers, which aids in rearranging elements.
- partition: This function organizes the elements around the pivot and returns the pivot's final position.
- quickSort: The main function that implements the recursive sorting mechanism of Quicksort.
Enhancements and Variants
To optimize performance, you can consider the following variants and enhancements:
-
Median-of-Three Pivot Selection: Instead of simply using the last element as the pivot, you can choose the median of the first, middle, and last elements to reduce the chance of poor performance on sorted or nearly-sorted data.
-
Tail Call Optimization: This is a technique to reduce the stack space in recursion. The idea is to call `quickSort` for the smaller partition first, and then use a loop for the larger partition.
-
Iterative Approach: For further performance gains, especially to avoid hitting stack limits, an iterative approach can be implemented using manual stack management.
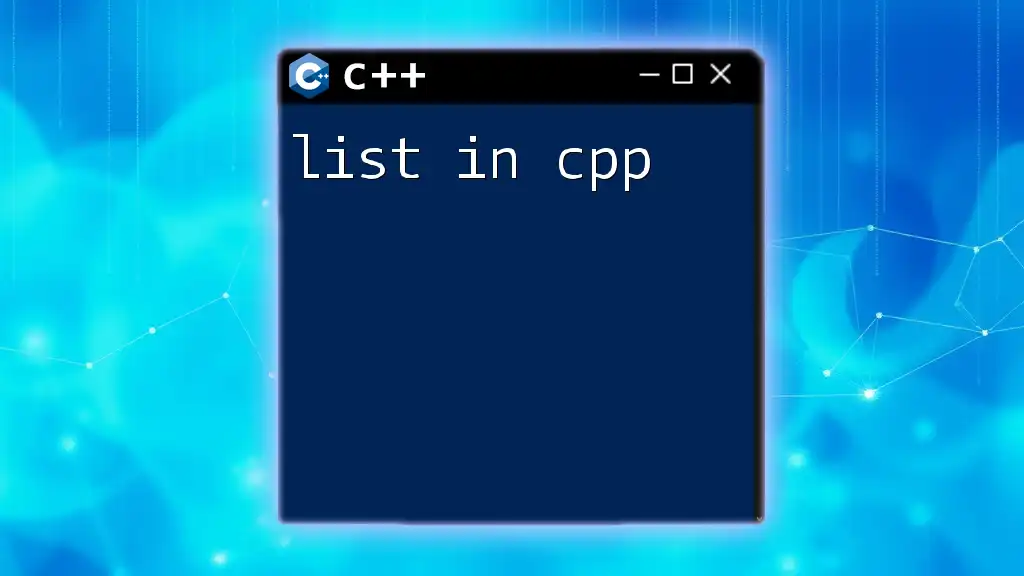
Analyzing Quicksort
Time Complexity
Quicksort operates with varying efficiency depending on the pivot selection and data arrangement:
- Best Case: O(n log n) occurs when the pivot divides the array into two equal halves.
- Average Case: O(n log n) is observed on average, as most random inputs will tend to balance out.
- Worst Case: O(n^2) arises when the pivot is the smallest or largest element repeatedly, which can occur in already sorted arrays (if not properly handled).
Space Complexity
The space complexity of Quicksort is primarily related to the recursion stack. In the average and best cases, it requires O(log n) space due to the depths of the recursive calls. However, if the worst-case scenario unfolds, it can reach O(n) as each recursive call may take an entire partition.
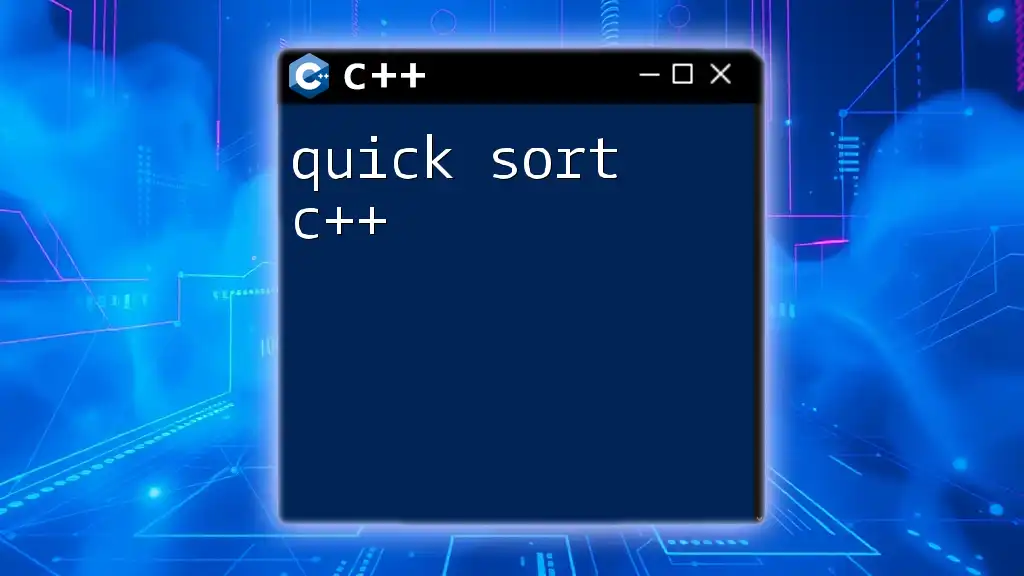
Pros and Cons of Quicksort
Advantages
- Efficiency: Quicksort is often faster than other O(n log n) sorting algorithms, especially on average.
- In-Place Sorting: It uses a small amount of additional memory, making it efficient with memory resources.
- Real-world Performance: It's exceptionally efficient for large datasets.
Disadvantages
- Worst-case performance: The algorithm can degenerate to O(n^2) if not implemented carefully (i.e., using a poor pivot).
- Complexity: Compared to simpler algorithms like Bubble Sort, understanding and implementing Quicksort can be more challenging.
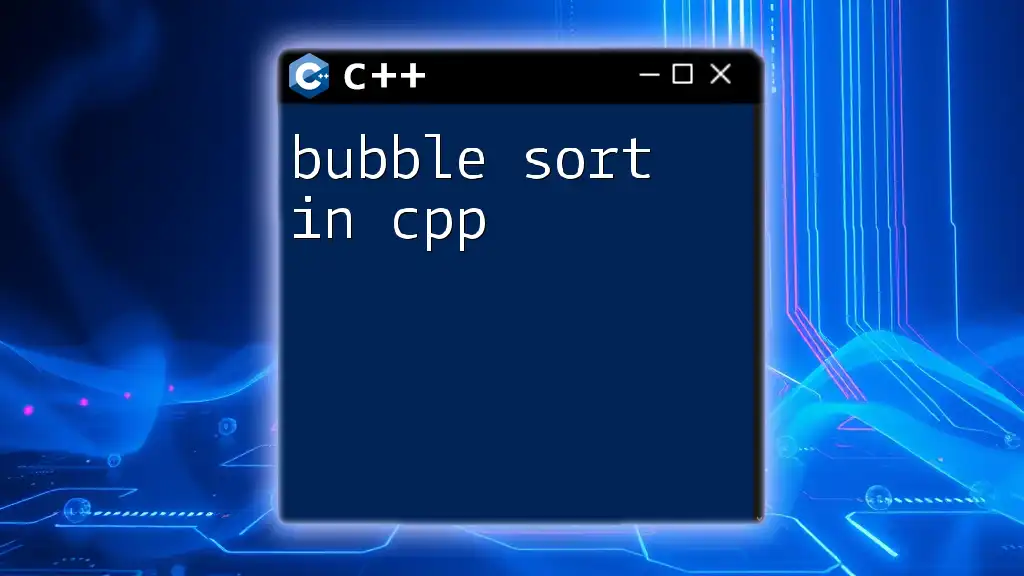
Practical Applications of Quicksort
Quicksort is widely used in various programming scenarios, including:
- Used in popular programming libraries and frameworks, for instance, the C++ Standard Library's `std::sort` function may implement Quicksort or a hybrid sorting method.
- Useful in applications requiring frequent sorting operations, such as database management systems and large-scale data processing.
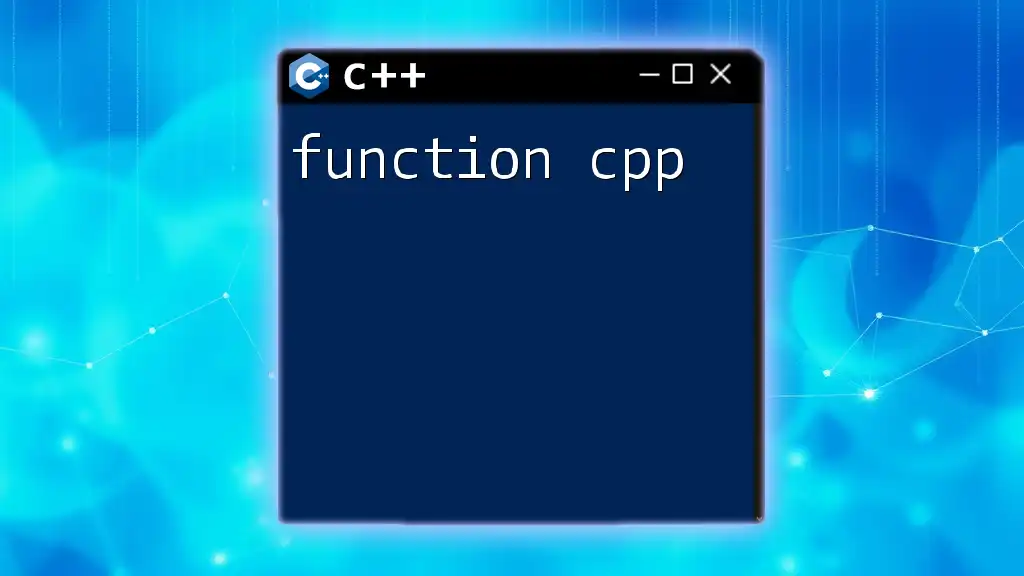
Conclusion
In essence, Quicksort is an indispensable tool in the realm of C++ programming. Mastering this algorithm opens up numerous possibilities in efficient data manipulation and sorting tasks. Practicing its implementation and understanding its mechanisms can significantly enhance your programming skill set.
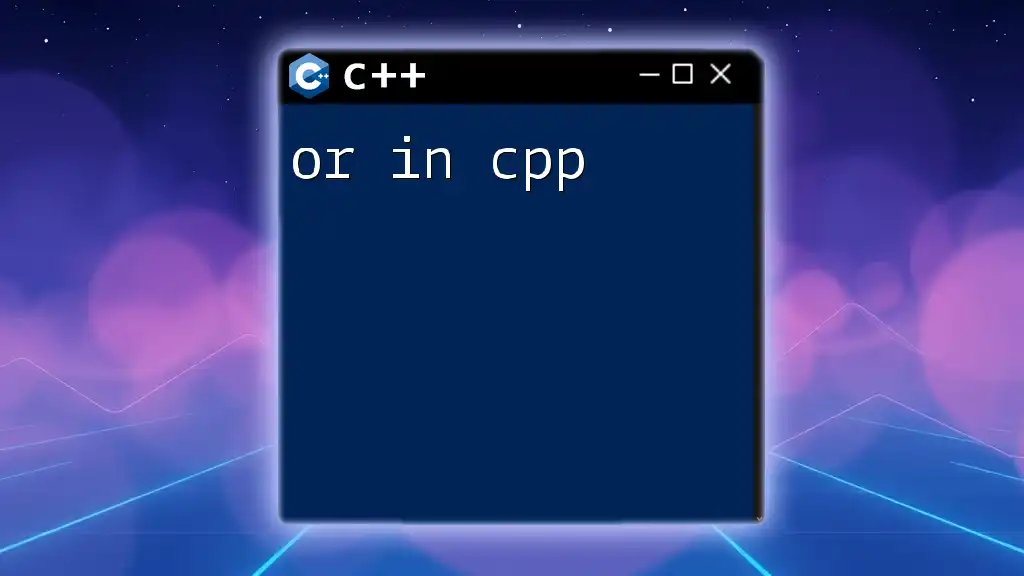
Additional Resources
For further reading and mastery of sorting algorithms, consider exploring advanced textbooks and online tutorials that delve deeper into the intricacies of sorting methods, their applications, and optimizations.
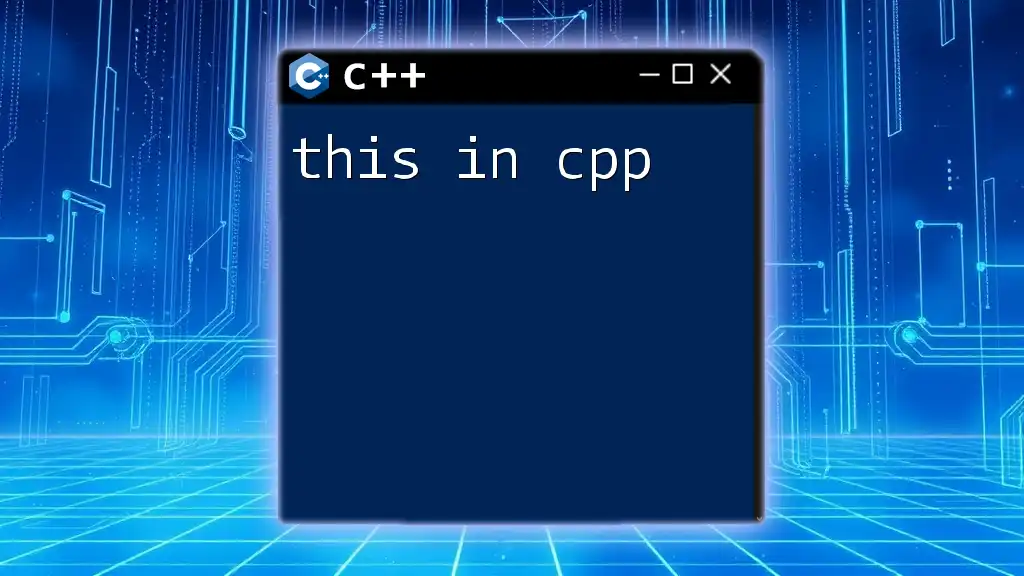
Call to Action
We invite you to share your experiences with implementing Quicksort in C++. Do you have any enhancements or insights to offer? Join the discussion and subscribe for more enriching programming tutorials!