In C++, a `list` is a sequence container that allows non-contiguous memory allocation, providing efficient insertions and deletions from any position in the list.
Here’s a simple code snippet demonstrating how to use a `list` in C++:
#include <iostream>
#include <list>
int main() {
std::list<int> myList = {1, 2, 3, 4, 5};
myList.push_back(6); // Add element at the end
for (int n : myList) {
std::cout << n << " "; // Output: 1 2 3 4 5 6
}
return 0;
}
Understanding Lists in C++
What is a List?
A list in C++ refers to a sequence container that implements a doubly linked list. This means that each element (or node) in the list contains a pointer to both the next and the previous node. Consequently, it allows for efficient insertions and deletions at any position since it doesn't require shifting elements as in other containers like `vector`.
Lists can be particularly useful when the primary operations performed on a collection of data involve frequent insertions or deletions.
Types of Lists
When discussing lists, there are two primary types:
-
Single Linked List: In this structure, each node points only to the next node. While this type of list uses less memory, it does not allow for backward traversal.
-
Doubly Linked List: This is the standard implementation used by the C++ Standard Library's `list`. Each node maintains pointers to both the next and previous nodes, allowing for bi-directional traversal.
Understanding when to use one over the other typically depends on your application's requirements for traversal and performance efficiency.
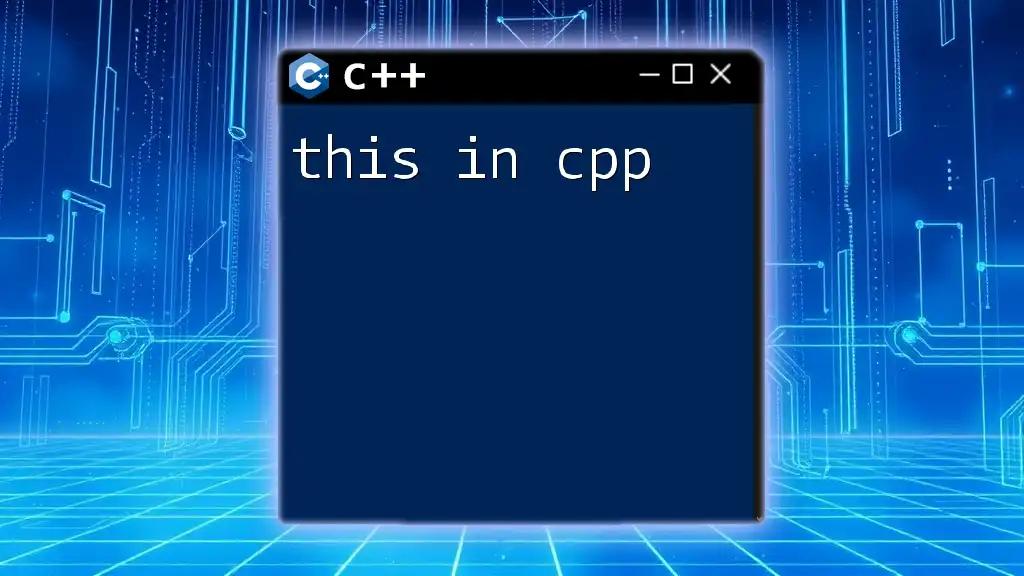
The C++ List Container
Including the List Header
To work with lists in C++, you need to include the necessary header file. You can do this with the following line of code:
#include <list>
Declaration and Initialization
You can create lists by declaring them and optionally initializing them with values. Here are a couple of examples:
std::list<int> myList; // Creates an empty list
std::list<int> myListWithValues = {1, 2, 3, 4, 5}; // Initializes a list with specified values
In these examples, `myList` is an empty list, while `myListWithValues` is initialized with five integers. This demonstrates the flexibility available when managing lists in C++.

Common Operations on Lists
Adding Elements
To add elements to a list, C++ provides several methods:
- Push Operations: The `push_back()` function appends an element to the end of the list, while `push_front()` adds an element to the beginning.
myList.push_back(6); // Adds 6 at the end of the list
myList.push_front(0); // Adds 0 at the beginning of the list
- Insert Operation: If you need to insert an element at a specific position, you can use the `insert()` method. This method takes an iterator pointing to the position where you want to insert the new element.
auto it = myList.begin();
myList.insert(it, -1); // Inserts -1 at the beginning
Removing Elements
Removing elements in a list can also be accomplished through various methods:
- Pop Operations: The `pop_back()` method removes the last element, while `pop_front()` removes the first element from the list.
myList.pop_back(); // Removes the last element
myList.pop_front(); // Removes the first element
- Remove by Value and Position: For instance, you can use `remove()` to delete all occurrences of a specific value or `erase()` to remove elements by iterator.
myList.remove(3); // Removes all occurrences of the number 3
Accessing Elements
When it comes to accessing elements in a list, it is essential to understand iterators. Using iterators allows you to traverse the list:
for(auto it = myList.begin(); it != myList.end(); ++it) {
std::cout << *it << " ";
}
Additionally, you can use the `front()` and `back()` methods to quickly retrieve the first and last elements of the list, respectively.

Advanced Features of Lists
Sorting and Reversing
C++ lists come equipped with built-in functionalities for sorting and reversing:
- Sorting a List: You can sort a list in ascending order using the `sort()` method.
myList.sort(); // Sorts the list in ascending order
- Reversing a List: Similarly, you can use `reverse()` to change the order of elements from last to first.
myList.reverse(); // Reverses the order of elements in the list
Merging and Splicing
The list container also supports merging and splicing:
- Merging Two Lists: To merge two sorted lists into one, you can use the `merge()` function, which combines elements from two lists into a single sorted list.
std::list<int> anotherList = {10, 20, 30};
myList.merge(anotherList); // Merges anotherList into myList
- Splicing Elements: The `splice()` function allows you to insert elements from one list to another at any position.
std::list<int> sourceList = {100, 200};
myList.splice(myList.begin(), sourceList); // Moves elements from sourceList to the front of myList
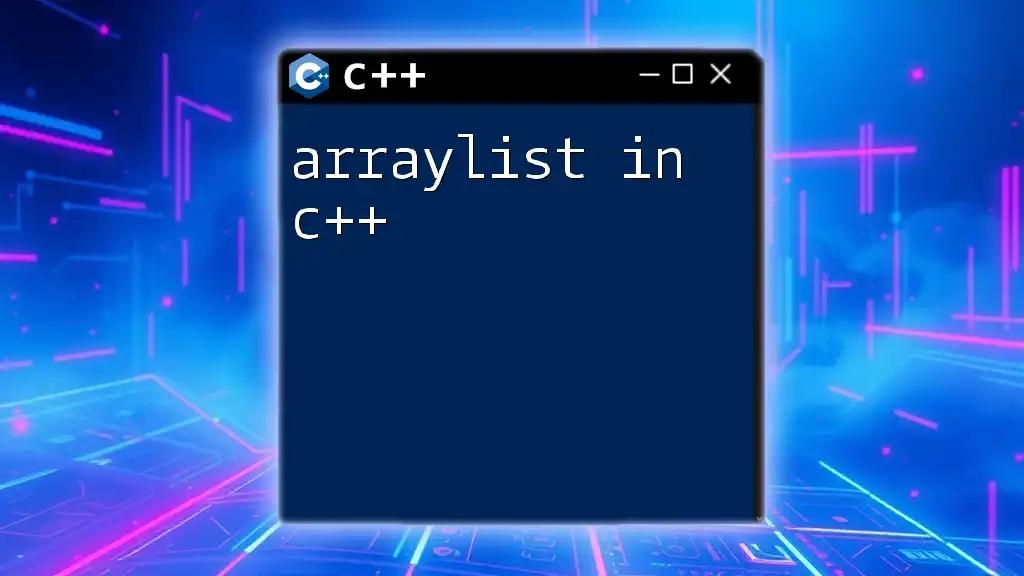
Pros and Cons of Using Lists
Advantages of Lists
Lists offer several benefits:
-
Dynamic Sizing: Unlike arrays, lists can grow and shrink in size easily without sacrificing speed.
-
Efficient Insertions and Deletions: The linked list structure allows for constant time insertions and deletions when suitably positioned.
Disadvantages of Lists
However, lists do come with some downsides:
-
Higher Memory Overhead: Each element requires additional memory for the pointers to adjacent nodes, which can lead to inefficient space utilization compared to tighter structures.
-
Slower Access Times: Accessing elements by index can be significantly slower because it requires traversal from the beginning of the list.

Common Use Cases for Lists
When should a programmer choose to use a list? Situations that benefit from a list's properties include:
-
Real-time applications where data involves frequent insert operations or removals, such as job scheduling systems.
-
Programs that require applying sorting or merging of collections of data, benefiting from built-in operations.
Understanding the strengths and weaknesses of the `list` in C++ can help you make better decisions about when to employ it versus other data structures.
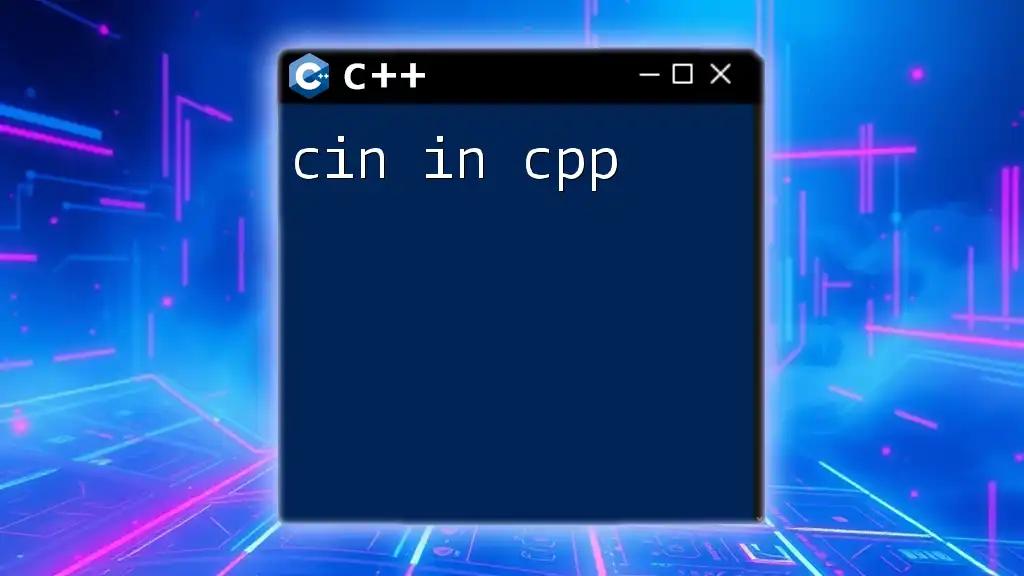
Conclusion
Lists in C++ provide a powerful, flexible way to manipulate collections of data, especially in scenarios that require frequent insertions and deletions. With their rich set of functionalities, lists prove to be an essential tool in a C++ programmer's toolkit.
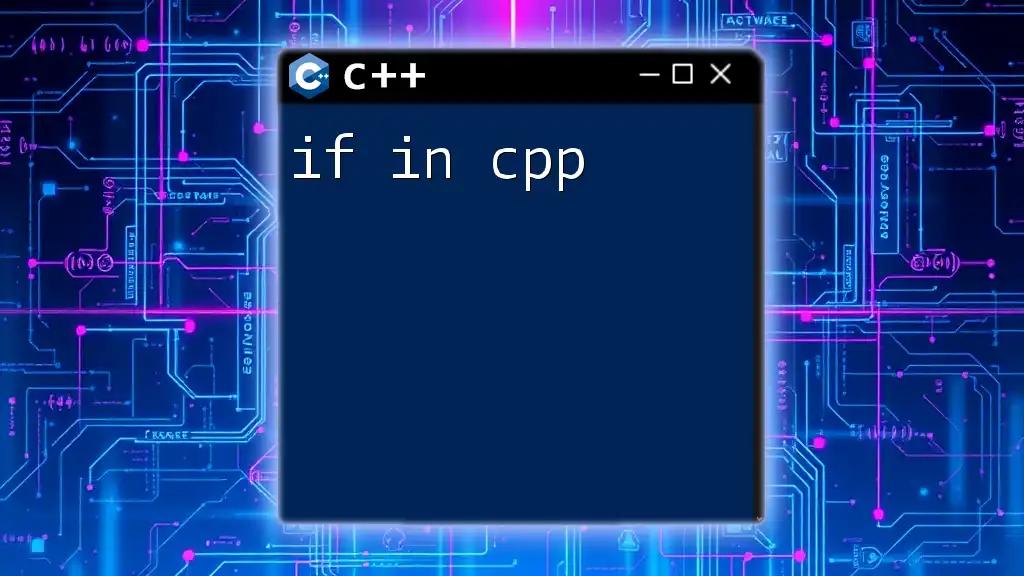
Further Reading
For those looking to deepen their understanding of lists and C++ container classes, numerous resources are available, including textbooks focused on the C++ Standard Library, online programming courses, and official documentation. Exploring these can offer new perspectives and advanced techniques to maximize the utility of lists in C++ applications.

Code Files & Examples
To enhance your learning experience, consider checking out code samples or repositories that showcase practical implementations of lists in C++. Experimenting with different operations reinforces comprehension and builds confidence in utilizing lists.