The C++ string class provides a flexible way to manipulate sequences of characters, allowing operations like concatenation, substring extraction, and more, as shown in the following example:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello";
str += " World!"; // Concatenate strings
std::cout << str << std::endl; // Output: Hello World!
return 0;
}
What is the C++ String Class?
The C++ String Class is a powerful feature of the C++ Standard Library that provides an abstraction for handling strings, or sequences of characters. Unlike C-style strings, which are essentially arrays of characters terminated by a null character, the string class in cpp provides a flexible, dynamic, and safer way to manipulate text data.
One of the key advantages of using the `std::string` class over C-style strings is that it automatically handles memory management. You do not have to worry about buffer overflows, as the string class can dynamically resize itself as needed. This brings a significant improvement in both safety and ease of use for your applications.
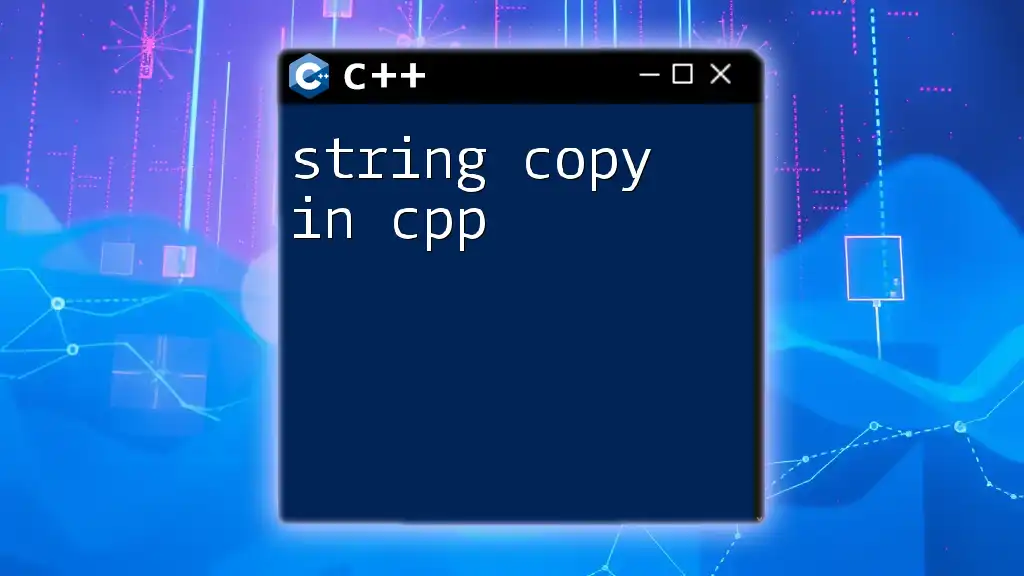
Basic Usage of the C++ String Class
Creating Strings
The string class in cpp allows you to create string objects in a straightforward manner. You can declare and initialize a string using either of the following syntaxes:
#include <iostream>
#include <string>
int main() {
std::string str1 = "Hello, World!";
std::string str2; // Default initialization
return 0;
}
In this snippet, `str1` is initialized with the value `"Hello, World!"`, while `str2` remains an empty string.
String Characteristics
One of the most compelling features of the string class in cpp is its dynamic nature. When you store text within a string object, the class automatically manages the memory. You don't need to specify a size beforehand; the class knows how to resize itself when you add or remove characters.
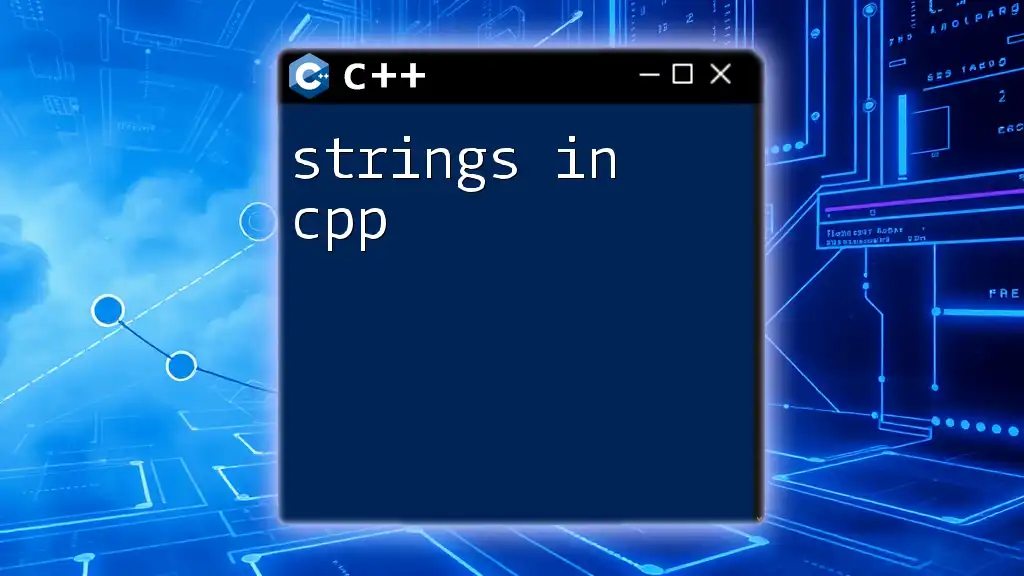
Common C++ String Class Methods
Length and Size
Two essential methods of the `std::string` class are `.length()` and `.size()`, which are used to return the number of characters in the string:
std::string str = "Hello";
std::cout << str.length(); // Outputs: 5
std::cout << str.size(); // Outputs: 5
Both methods provide the same result, although `.size()` is more commonly used in contexts where capacity, rather than the number of elements, is emphasized.
Accessing Characters
Access to characters in a string can be achieved in two primary ways: using the `operator[]` or the `.at()` method.
char firstChar = str[0]; // 'H'
char secondChar = str.at(1); // 'e'
While both methods provide access to individual characters, `.at()` performs bounds checking, which can help prevent runtime errors.
Concatenation
Joining strings is a common operation, easily achieved with either the `+` operator or the `.append()` method:
std::string str3 = str + " World!";
str.append(" Concatenation");
In this example, `str3` becomes `"Hello World!"`, and the original `str` ends with `" Concatenation"`.
Substring Operations
Extracting a portion of a string is straightforward using the `.substr(start, length)` method:
std::string substring = str.substr(0, 5); // "Hello"
This extracts five characters, starting from index 0.
Find and Replace
The string class in cpp provides convenient methods to search for and replace substrings. You can use the `.find()` method to locate a substring and the `.replace()` method to replace it.
size_t pos = str.find("World");
if (pos != std::string::npos) { // Check if the substring exists
str.replace(pos, 5, "C++"); // Now str is "Hello C++!"
}
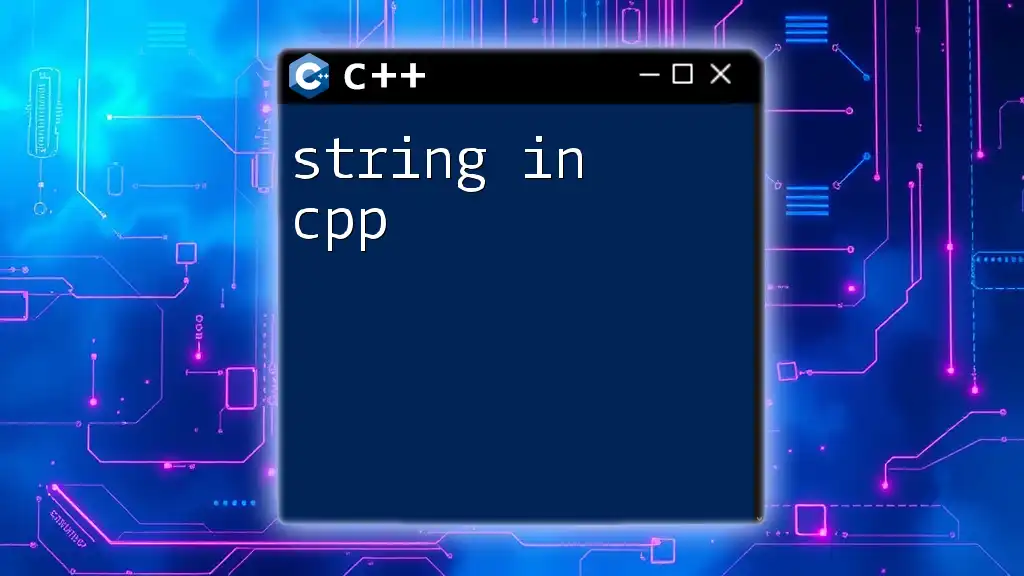
Advanced String Class Methods
Inserting and Erasing
Strings can be manipulated further by using the `.insert()` and `.erase()` methods. For example, you can insert text at a specific position or erase a portion of a string:
str.insert(5, " Beautiful"); // Results in "Hello Beautiful C++!"
str.erase(0, 6); // Removes "Hello"
Comparing Strings
To compare strings, you can use standard comparison operators (`==`, `!=`, `<`, `>`) or the `.compare()` method:
if (str1 == str2) {
std::cout << "Strings are equal";
}
This is useful for determining the relationship between two string values.
String Conversion
Converting between strings and numbers is often essential. The C++ Standard Library offers facilities such as `.to_string()` for numbers and `std::stoi()` for converting strings to integers:
int num = 54321;
std::string numStr = std::to_string(num); // "54321"
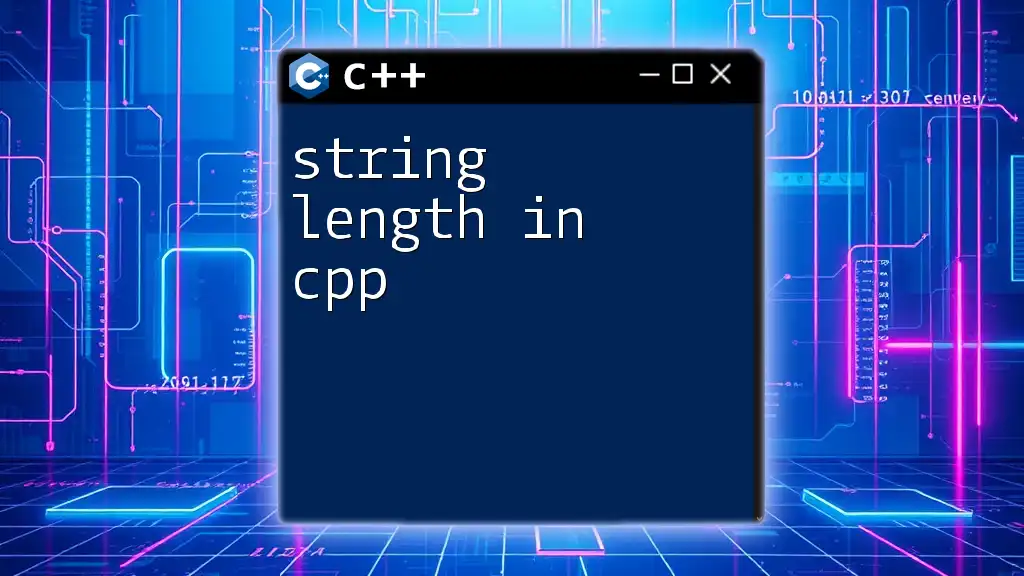
String Iterators
Using Iterators with String
The string class in cpp also supports iterators, which allow you to traverse through a string’s characters easily:
for (auto it = str.begin(); it != str.end(); ++it) {
std::cout << *it << " ";
}
This loop prints each character in the string individually.
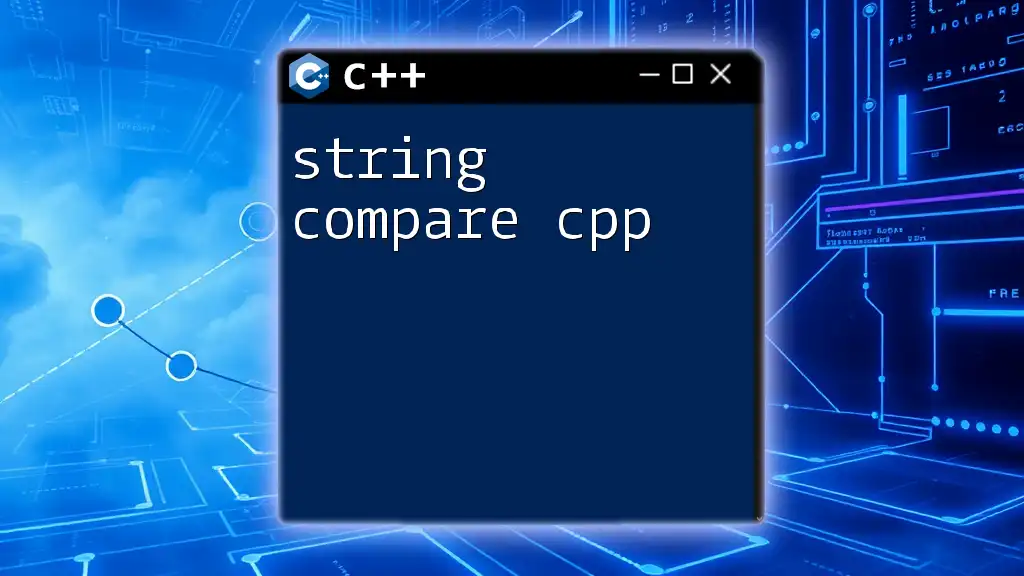
String Stream Classes
Brief Overview of `std::stringstream`
The `std::stringstream` is another powerful tool that allows input and output operations on strings. It can be used to build and format strings dynamically. For example:
std::stringstream ss;
ss << "Hello " << "World!";
std::string output = ss.str(); // "Hello World!"
This approach is particularly useful when dealing with multiple data types in string formatting.
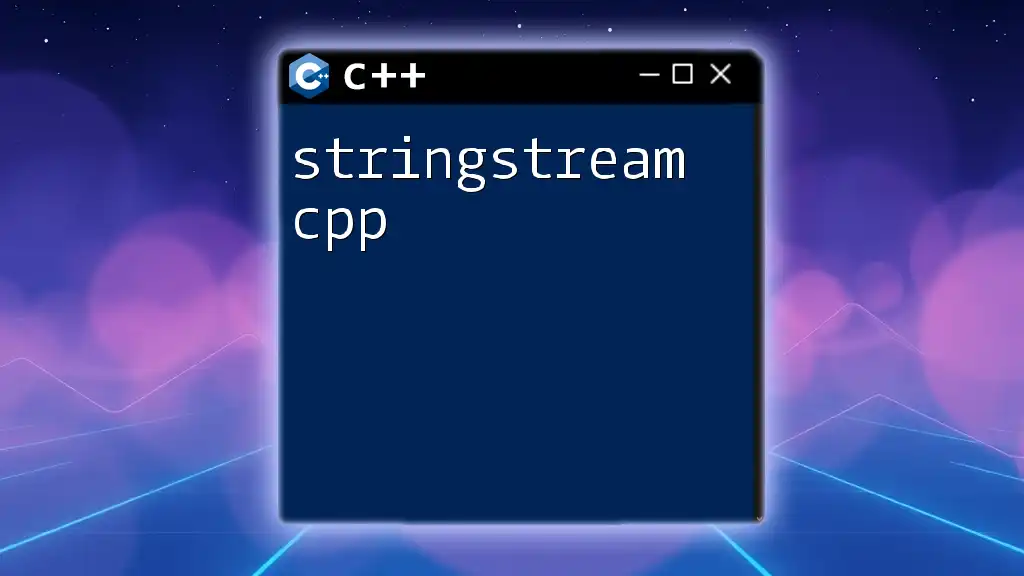
Common Use Cases
Understanding the functionality of the string class in cpp leads to various real-world applications. Common scenarios include:
- User Input Handling: Capturing and processing textual input from end-users.
- File I/O Operations: Reading and writing strings to files for data storage.
- Text Manipulation: Performing search, edit, and formatting tasks in applications.
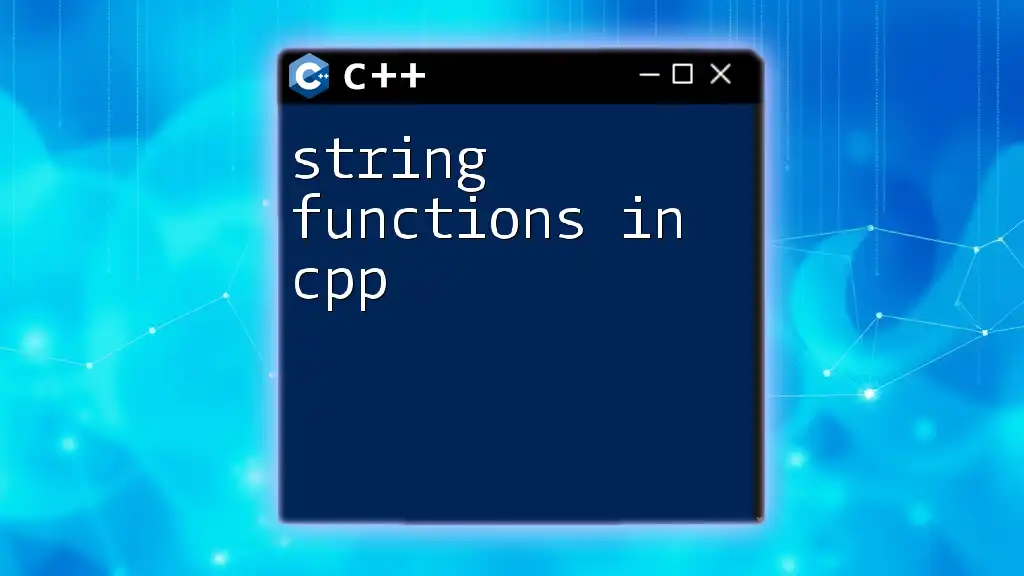
Tips and Best Practices
To make the most of the C++ String Class, consider the following best practices:
- Avoid Unnecessary Copies: Use `const` references where applicable to avoid copying large strings unnecessarily.
- Utilize Standard Library Functions: Using these functions promotes clarity and safety in your code.
- Know Your Small String Optimization: C++ implements an optimization technique for short strings to minimize dynamic allocations, which enhances performance.
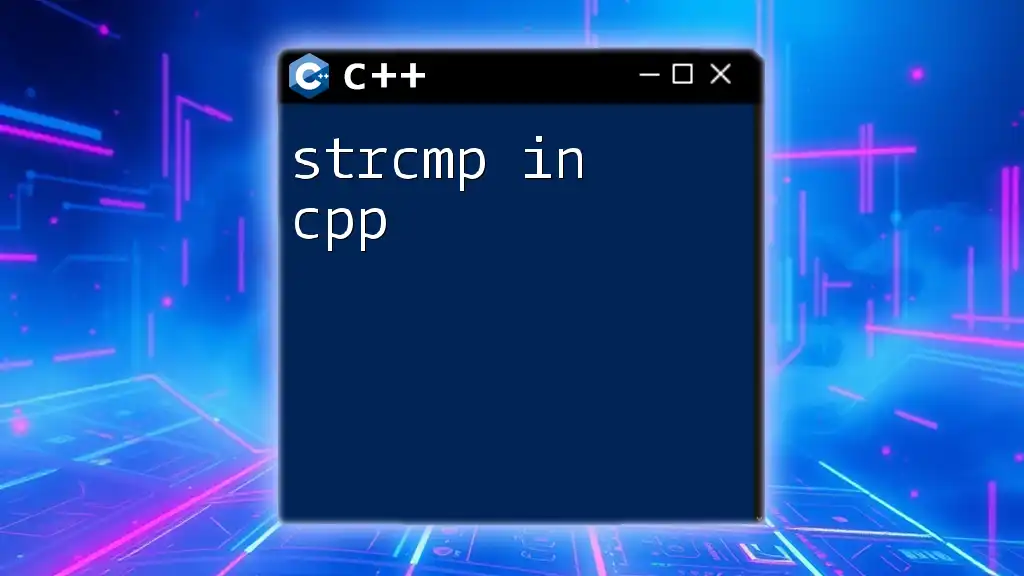
Conclusion
Mastering the string class in cpp is crucial for effective C++ programming. With its vast array of methods and capabilities, you can handle text data efficiently and securely. As with any programming skill, continued practice and learning will enhance your string manipulation abilities, making you a more proficient coder in C++.
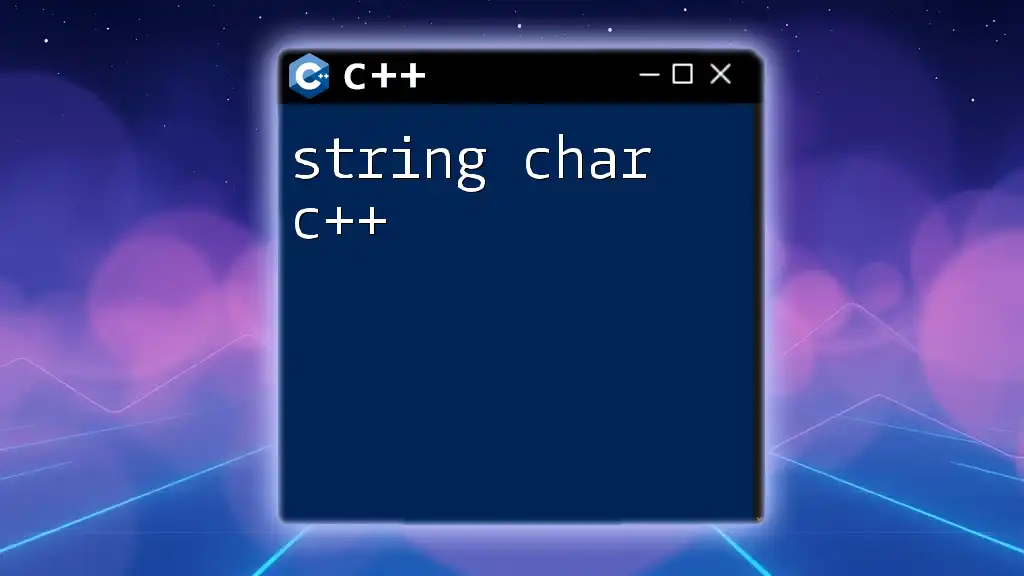
Further Learning Resources
For those interested in diving deeper, numerous resources are available, including reputable books, online courses, and official documentation. Engaging with these materials will not only improve your understanding of the string class in cpp but also other vital aspects of C++ programming.