In C++, you can determine the length of a string using the `length()` method or the `size()` method from the `std::string` class.
Here's a code snippet demonstrating both methods:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World!";
std::cout << "Length of the string: " << str.length() << std::endl; // Using length()
std::cout << "Size of the string: " << str.size() << std::endl; // Using size()
return 0;
}
Understanding Strings in C++
What is a String?
In C++, a string is a sequence of characters used to store textual data. Strings are essential to programming as they allow you to work with text-based input and output. In C++, there are two primary forms of strings: C-style strings and C++ strings (std::string).
C-style Strings vs C++ Strings
- C-style strings are arrays of characters terminated by a null character (`'\0'`). They do not provide built-in methods to manipulate the string easily, leading to complex memory management.
- C++ strings utilize the `std::string` class from the C++ Standard Library. They offer powerful features such as dynamic resizing, built-in methods for string manipulation, and automatic memory management.
Using `std::string` is generally recommended due to its ease of use and reduced potential for errors.
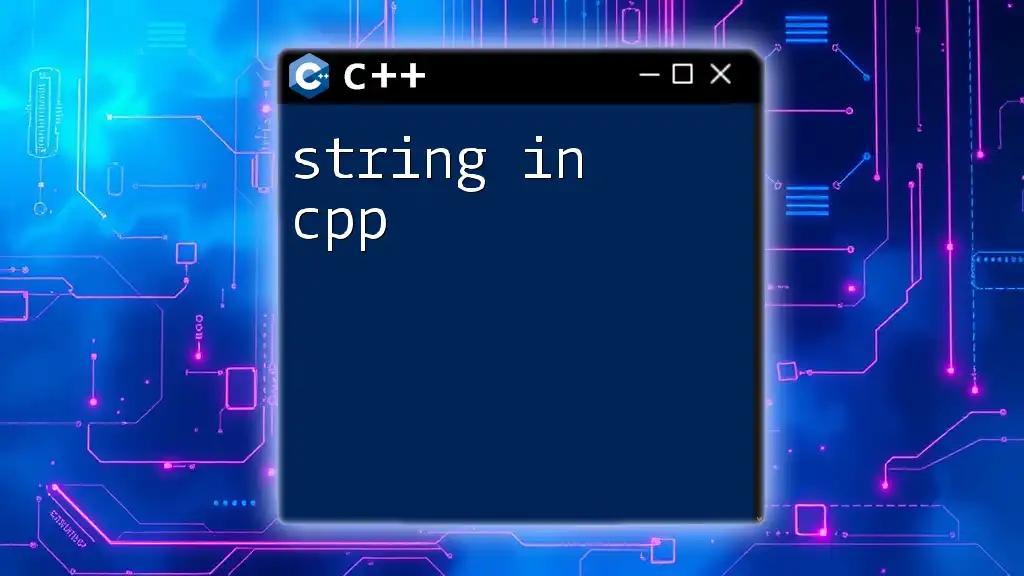
Measuring String Length in C++
Getting Started with String Length
Understanding string length is crucial in many programming scenarios. String length refers to the number of characters in a string, not counting the null terminator in C-style strings.
Using `std::string::length()` Method
The `length()` method provides a straightforward way to find the length of a `std::string`. Below is an example that demonstrates its usage:
#include <iostream>
#include <string>
int main() {
std::string myString = "Hello, World!";
std::cout << "The length of the string is: " << myString.length() << std::endl;
return 0;
}
In this example, the output will be `The length of the string is: 13`, as there are 13 characters in "Hello, World!".
Using `std::string::size()` Method
Interestingly, the `size()` method serves the same purpose as `length()`. It counts the number of characters in the string, offering flexibility depending on your coding style. Here’s a code example:
#include <iostream>
#include <string>
int main() {
std::string myString = "OpenAI";
std::cout << "The size of the string is: " << myString.size() << std::endl;
return 0;
}
Here, the output will be `The size of the string is: 6`. Many programmers prefer `size()` in performance-critical contexts, but both methods are efficient.
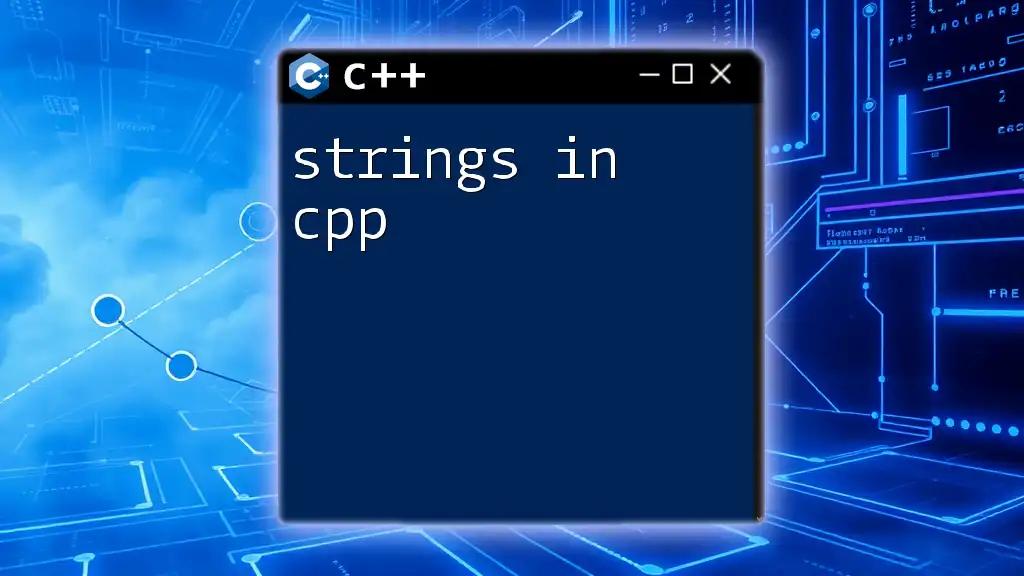
Working With C-Style Strings
Calculating Length with `strlen()`
For C-style strings, you can use the `strlen()` function. This function computes the length of a null-terminated string. Here is how you can use `strlen()`:
#include <iostream>
#include <cstring>
int main() {
const char* myCString = "Learning C++!";
std::cout << "The length of the C-style string is: " << strlen(myCString) << std::endl;
return 0;
}
The output will be `The length of the C-style string is: 15`. Note that `strlen()` effectively counts all characters until it reaches the null character.
Converting Between C++ and C-style Strings
Sometimes you may need to convert between `std::string` and C-style strings. This is essential for interfacing with legacy C APIs. You can use the `c_str()` method to get a C-style string from a `std::string`:
#include <iostream>
#include <string>
#include <cstring>
int main() {
std::string cppString = "C++ Strings";
const char* cString = cppString.c_str();
std::cout << "The length of the C-style string after conversion is: " << strlen(cString) << std::endl;
return 0;
}
This example will produce `The length of the C-style string after conversion is: 12`, demonstrating how easily you can switch between the two forms.

Common Mistakes When Measuring String Length
Off-by-One Errors
A common mistake programmers make when measuring string length is off-by-one errors. This occurs when the programmer forgets that the null character is not counted in the string length. Always remember that C-style strings utilize null-termination.
Misunderstanding String Terminology
Another point of confusion is the terminology surrounding string length. Length refers specifically to the number of characters—not including the null terminator in the case of C-style strings. Ensuring clarity in these terms will help avoid potential bugs in your programs.

Performance Considerations
Optimal Use of String Length Functions
While both `length()` and `size()` are efficient and largely interchangeable for typical use, performance can matter in critical sections of your code. If you're frequently checking the length of a string—especially in loops or complex algorithms—consider caching the length if it will not change during execution.

Summary
Understanding string length in C++ is critical for effective string manipulation and management. Whether utilizing the convenient `std::string` class with its dynamic sizing or employing legacy C-style strings, knowledge of measuring string length will enhance your programming skills.

Additional Resources
For those looking to dive deeper into the world of C++, consider exploring advanced texts on C++ programming or accessing online courses that focus specifically on strings and data types in C++. Engage with online forums, communities, and coding groups to network with other C++ enthusiasts and sharpen your skills.

Call to Action
Feel free to share your experiences or questions regarding string length in C++. Your insights and queries enrich the conversation and help foster a community of learning. Don’t forget to subscribe to our updates for more tips, tricks, and tutorials on mastering C++!