`ostringstream` in C++ is a stream class used for performing output operations on strings, allowing you to concatenate and format text using stream operators.
Here is a code snippet demonstrating its usage:
#include <iostream>
#include <sstream>
int main() {
std::ostringstream oss;
oss << "The answer is: " << 42;
std::string result = oss.str();
std::cout << result << std::endl; // Output: The answer is: 42
return 0;
}
Understanding `ostringstream c++`
What is `ostringstream`?
`ostringstream` is a class in C++ that belongs to the `<sstream>` header. It is designed specifically for outputting formatted strings.
Purpose: The primary role of `ostringstream` is to provide a flexible and powerful mechanism for building strings. Instead of manipulating strings directly, you can use `ostringstream` as a stream where you can insert various types of data, which the class will handle for you and convert into a string format.
Benefits of Using `ostringstream`
Using `ostringstream` offers several advantages:
- Convenience: You can build complex string outputs seamlessly using the `<<` operator. This makes the code cleaner and easier to read.
- Type Safety: `ostringstream` automatically handles conversion from different data types to strings.
- Performance: When constructing strings, especially in loops or large applications, `ostringstream` can be more efficient than constant string concatenation.
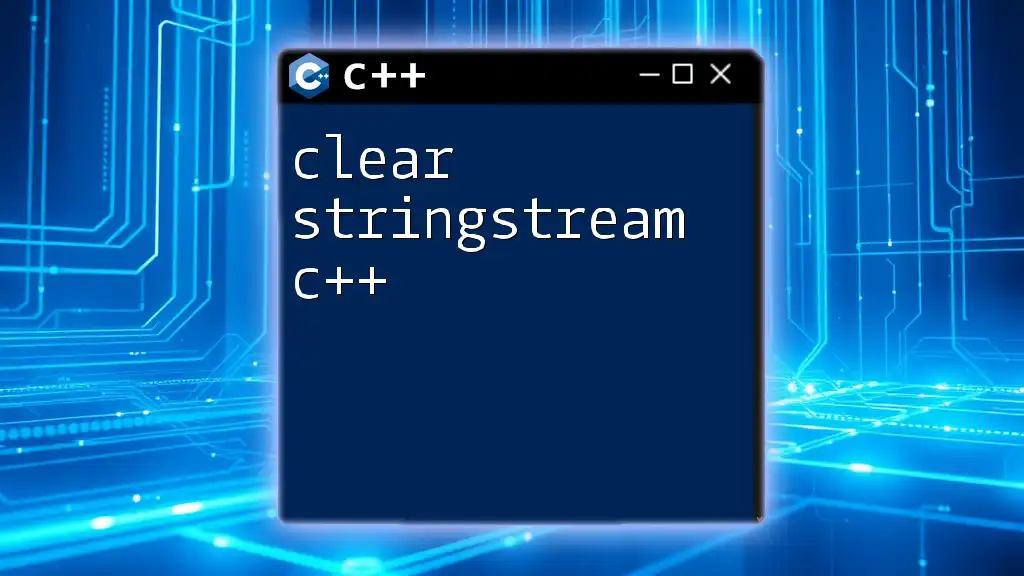
The Basics of `ostringstream`
Creating an `ostringstream` Object
To utilize `ostringstream`, you first need to create an object of this class:
#include <sstream>
std::ostringstream oss;
This simple code snippet creates an instance of `ostringstream` that you'll use to build your string.
Including the Necessary Headers
Before you can use `ostringstream`, make sure you include the correct header:
#include <sstream>
This inclusion is necessary as it defines the `ostringstream` class.
Understanding the Namespace
Remember to always use the `std::` namespace when working with `ostringstream`. It’s crucial for avoiding name clashes and ensuring that your code adheres to C++ standards:
std::ostringstream oss;
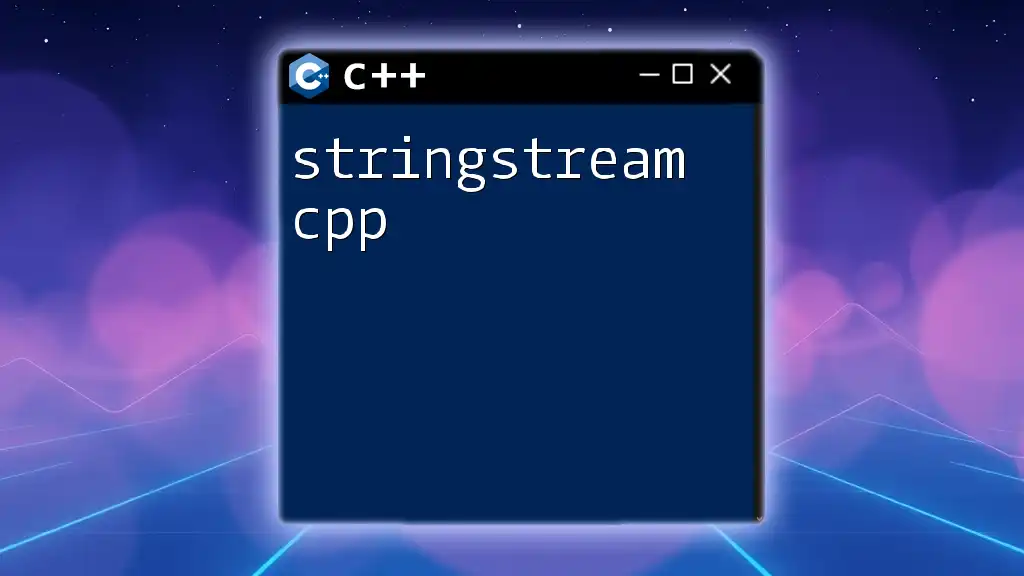
Core Functions of `ostringstream`
Inserting Data into an `ostringstream`
You can insert data into your `ostringstream` object using the `<<` operator. This operator can handle various types of data, including integers, characters, and strings:
oss << "The answer is: " << 42;
This line of code concatenates the string "The answer is: " with the integer 42, creating a cohesive output.
Retrieving the String Value
To obtain the constructed string from your `ostringstream`, you use the `str()` method:
std::string result = oss.str();
This method extracts the contents of the stream into a regular `std::string` that you can use or manipulate further.
Clearing and Resetting the Stream
If you need to reset the contents of your `ostringstream`, you can do it by calling `str()` with an empty string:
oss.str("");
This clears the current stream's contents, allowing you to reuse the `ostringstream` without creating a new object.
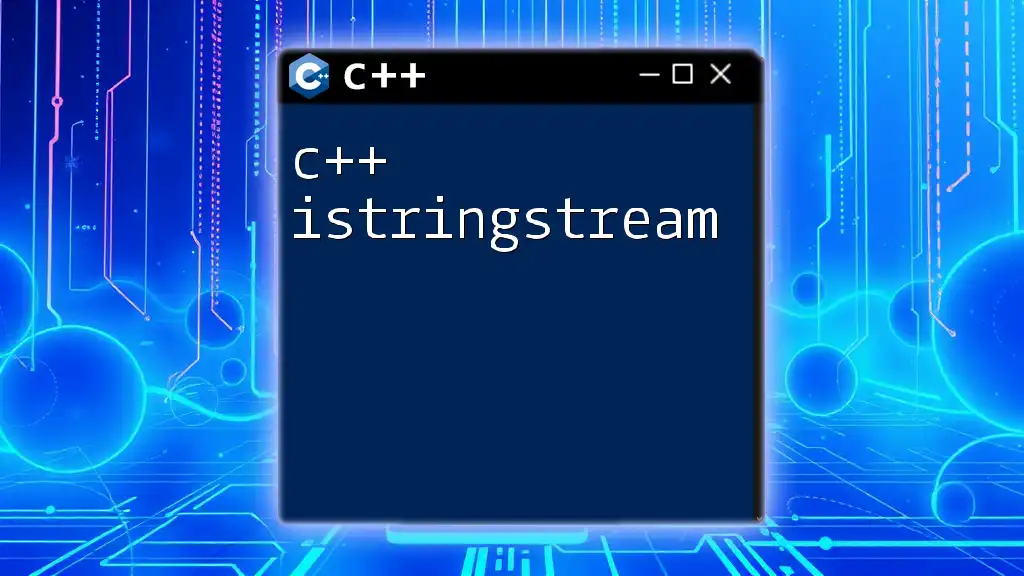
Advanced Usage of `ostringstream`
Formatting Output
`ostringstream` allows for easy formatting of your output, including controlling precision for floating-point numbers and setting fixed-point notation:
#include <iomanip>
oss << std::fixed << std::setprecision(2) << 3.14159;
Using `std::fixed` and `std::setprecision`, you can define how many decimal places to show, making `ostringstream` powerful for formatted output.
Error Handling and State Management
Stream objects in C++ can enter an error state, and it’s important to know how to handle this. For example, you can check if your stream is in a failed state using:
if (oss.fail()) {
// Handle errors
}
This check allows for robust error management, ensuring that any formatting or insertion issues can be addressed immediately.
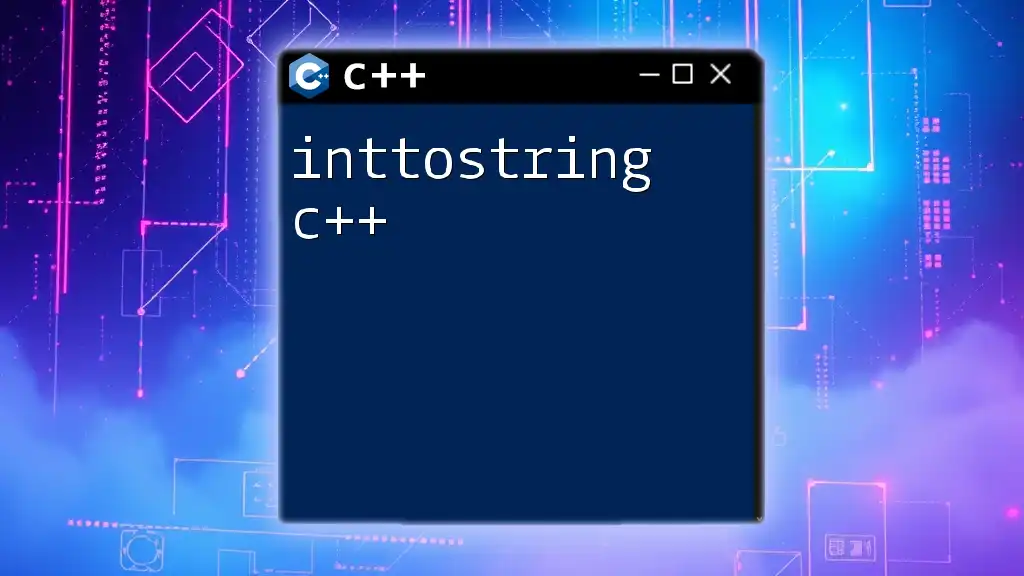
Practical Applications of `ostringstream`
Building Complex Strings
Suppose you want to construct a sentence dynamically. With `ostringstream`, this process becomes straightforward:
std::string name = "Alice";
int age = 30;
oss << name << " is " << age << " years old.";
This example illustrates how smoothly multiple data types can be incorporated into a single cohesive string.
Logging Information
Another practical application of `ostringstream` is in logging systems:
oss << "Error: " << someErrorCode << " occurred at " << timestamp;
By using `ostringstream`, you can dynamically generate logs that can include error codes, timestamps, and any additional context—essential for debugging or monitoring applications.
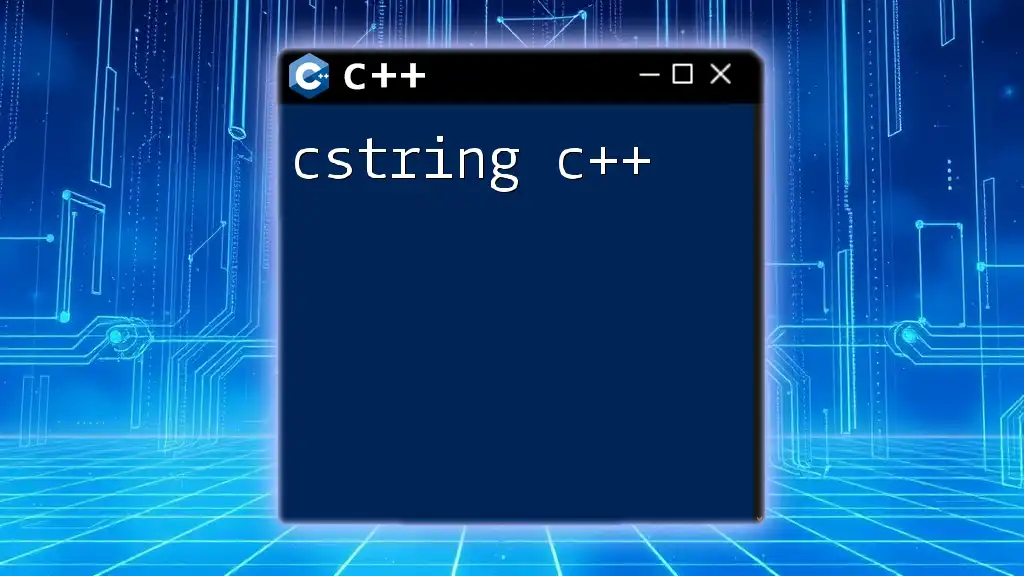
Performance Considerations
Memory Management
While `ostringstream` provides convenience, it's important to remember how it allocates memory. Each time you modify the stream, it may need to reallocate if the buffer size is exceeded. Consider performance carefully when building strings in large loops.
Comparing with Other String Handling Methods
When comparing `ostringstream` with traditional string concatenation (using `+`), you'll find that `ostringstream` can offer better performance, especially when constructing large strings or performing many insertions.
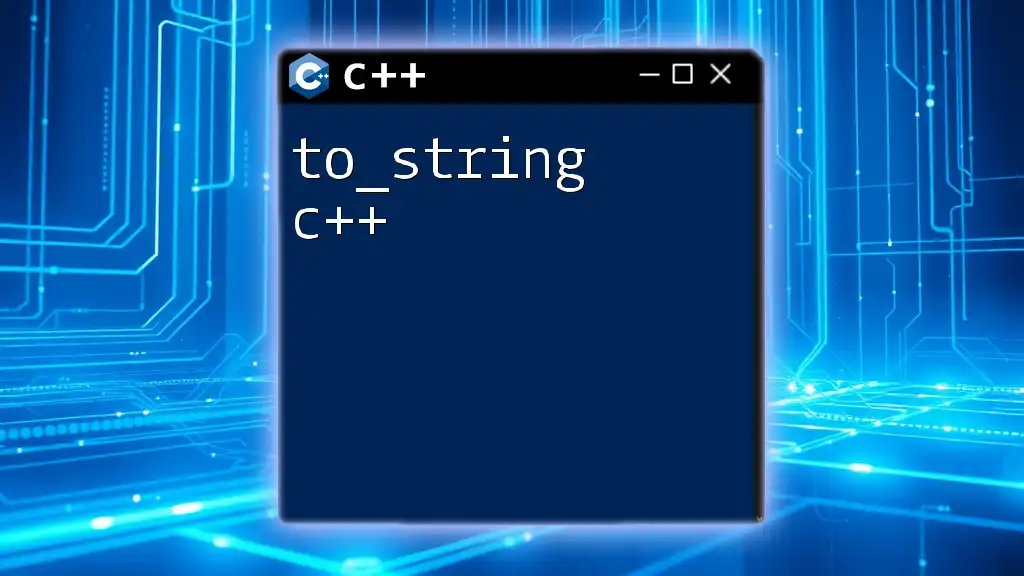
Conclusion
In summary, `ostringstream` is a practical and efficient way to handle string manipulation in C++. It simplifies the process of formatting output and makes the code significantly easier to write and read.
Don’t hesitate to experiment with `ostringstream` in your own C++ projects today! You'll find that the power and flexibility this tool offers will enhance your string handling capabilities and improve your coding experience.
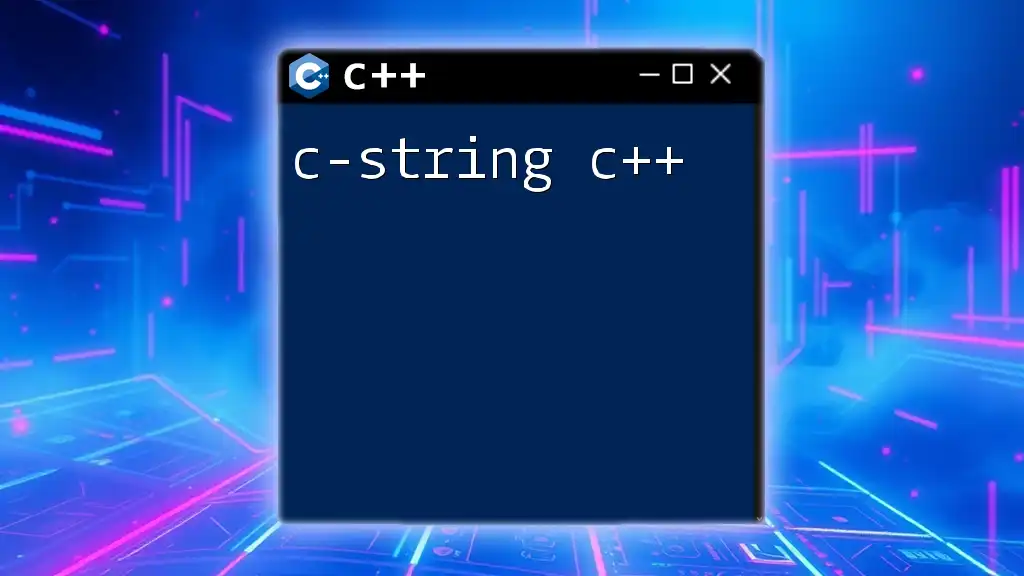
Additional Resources
For further exploration of `ostringstream c++`, consider checking out official C++ documentation and noteworthy online courses that can deepen your understanding. Participate in community forums to ask questions or share your experiences with other developers, fostering a collaborative learning environment.
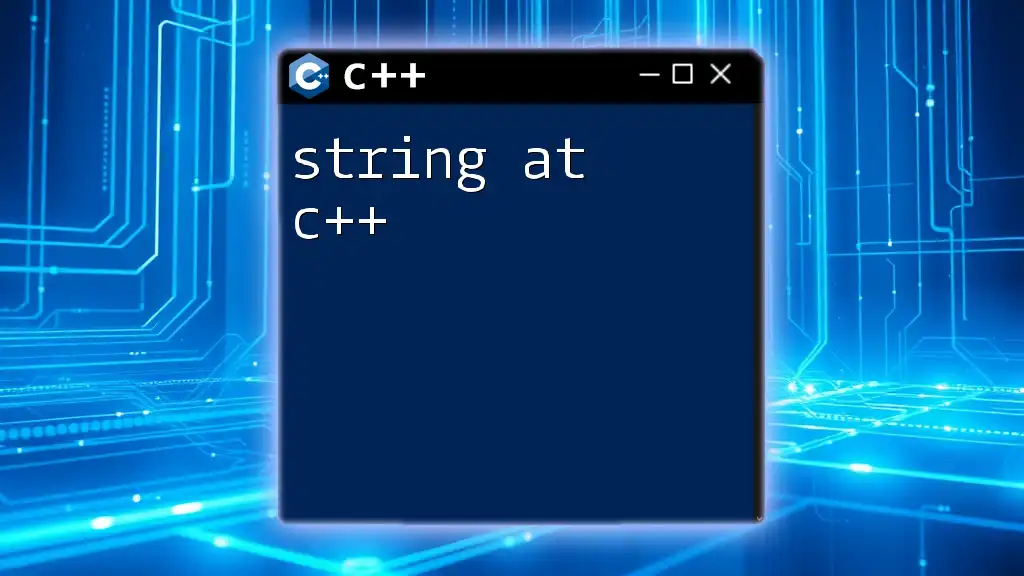
FAQs
What is the difference between `ostringstream` and `istringstream`?
`ostringstream` is used for outputting data into strings, while `istringstream` is used for reading data from strings.
When is it better to use `ostringstream` over other string manipulation methods?
Use `ostringstream` when formatting strings with multiple data types or when performance is essential for constructing complex strings.
Can I use `ostringstream` for internationalization and localization?
Yes, `ostringstream` can work well in different locales, but be mindful of how data formats such as currency, dates, and numbers vary across different cultures.