The C++ `<filesystem>` library provides tools for performing operations on file systems, allowing users to manipulate files and directories efficiently.
Here's a simple example demonstrating how to list all files in a directory using the C++ `<filesystem>` library:
#include <iostream>
#include <filesystem>
namespace fs = std::filesystem;
int main() {
const std::string path = "your_directory_path"; // Replace with your directory
for (const auto& entry : fs::directory_iterator(path)) {
std::cout << entry.path() << std::endl;
}
return 0;
}
What is the C++ Filesystem Library?
The C++ Filesystem Library is a part of the C++ Standard Library introduced in C++17. It provides a set of utilities to work with the filesystem, allowing developers to perform operations such as file and directory management in a straightforward and efficient manner. This includes tasks like navigating the filesystem, querying file properties, and handling file paths.
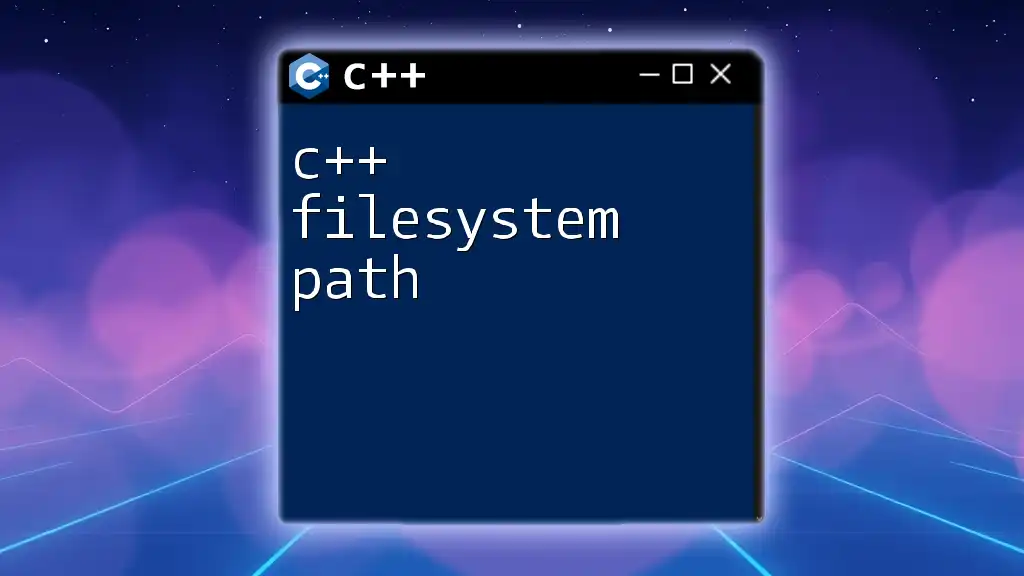
Why Use the Filesystem Library?
Using the filesystem library is advantageous over traditional methods due to its modern approach and ease of use. It abstracts many low-level details, allowing developers to work with files and directories without having to manage raw OS calls directly. It offers a rich set of functionalities while leading to cleaner, more maintainable code.
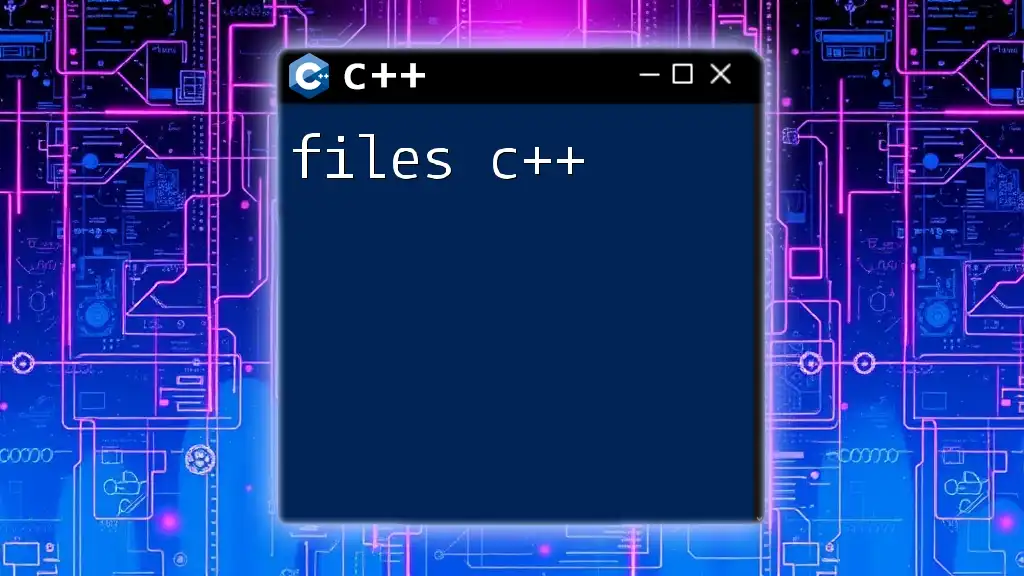
Setting Up Your Environment
Requirements
To use the filesystem library, ensure you have a C++ compiler that supports C++17 or higher. Popular IDEs like Visual Studio, CLion, and Code::Blocks generally support this version. Also, make sure your project is configured to use C++17.
Including the Filesystem Header
To get started with the filesystem library, you’ll need to include its header file in your program:
#include <filesystem>
namespace fs = std::filesystem;
This header provides all the necessary classes and functions for filesystem operations, and creating an alias (`fs`) simplifies your code, making it easier to read and write.
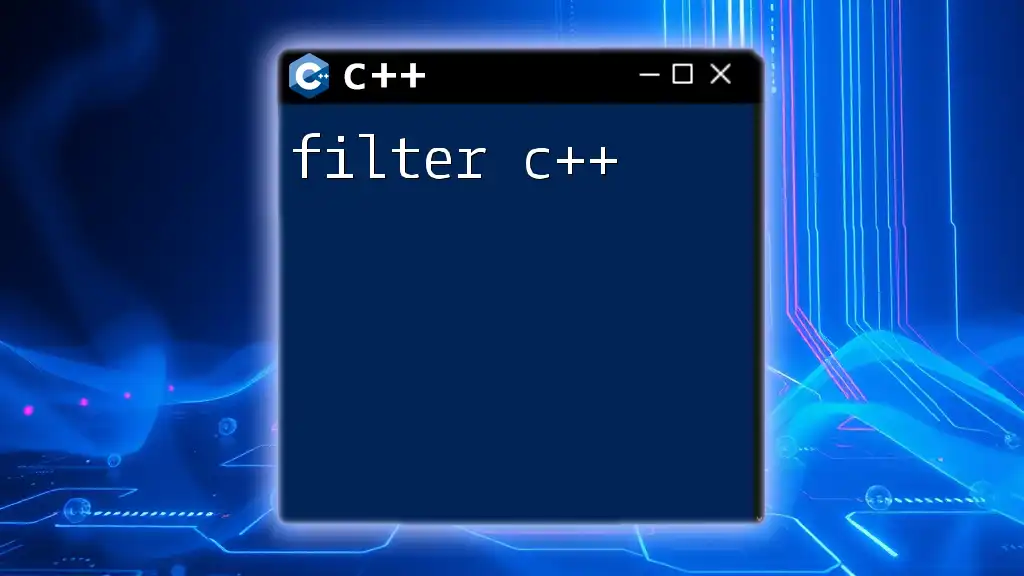
Understanding Basic Concepts
Paths and Directories
A path refers to the location of a file or directory in the filesystem. Paths can be either relative (starting from the current working directory) or absolute (providing a complete address from the root of the filesystem).
You can create a path in C++ using:
fs::path myPath("my_folder/my_file.txt");
File Types
The filesystem library works with various file types, including:
- Regular files: Standard files.
- Directories: Folders containing files or other directories.
- Symbolic links: References to other files or directories.
Understanding these concepts is crucial for successful navigation and manipulation of the filesystem.
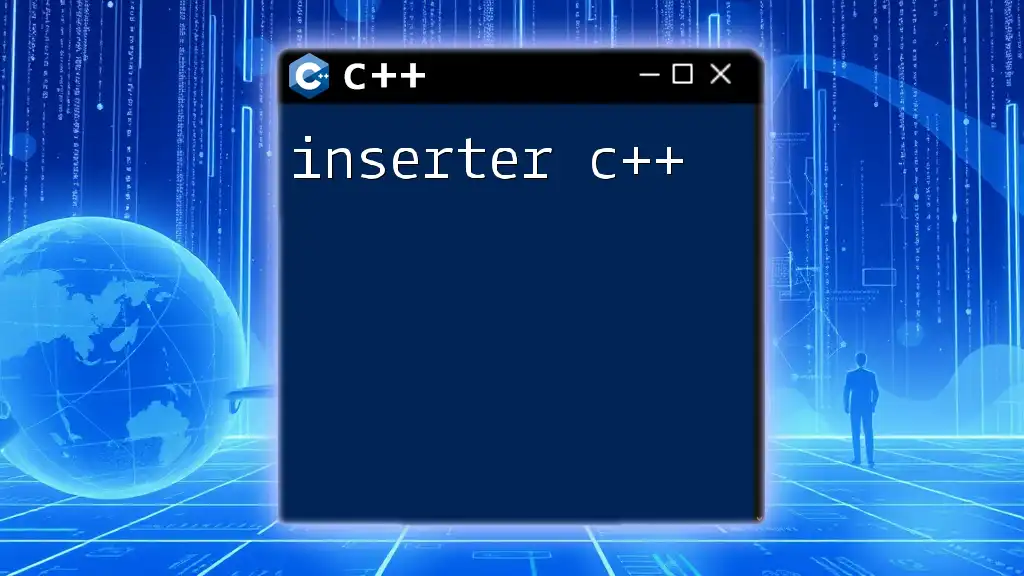
Core Functionality of C++ Filesystem
Navigating the Filesystem
Current Path
You can get or set the current working directory using the `current_path` function. For example, to set the current working directory:
fs::current_path("/new/directory/path");
To retrieve the current path, simply call:
std::cout << fs::current_path() << std::endl;
File and Directory Operations
Creating Directories
Creating directories is straightforward using the `create_directory` function:
if (fs::create_directory("new_directory")) {
std::cout << "Directory created successfully!" << std::endl;
} else {
std::cout << "Directory already exists or couldn't be created." << std::endl;
}
Removing Files and Directories
To remove a file or an empty directory, use the `remove` function. This is how you can safely delete a file:
if (fs::remove("file_to_delete.txt")) {
std::cout << "File deleted successfully!" << std::endl;
}
For directories, use `remove` on empty ones or `remove_all` to delete a directory and all its contents.
Copying and Moving Files
Copying files can be performed with:
fs::copy("source.txt", "destination.txt");
You can move files similarly using:
fs::rename("old_file_name.txt", "new_file_name.txt");
Renaming Files
Renaming a file is as simple as using the `rename` function, which is the previously mentioned `fs::rename`.
Querying Filesystem State
Checking if a Path Exists
You can check if a file or directory exists using the `exists` function:
if (fs::exists("example.txt")) {
std::cout << "The file exists." << std::endl;
} else {
std::cout << "The file does not exist." << std::endl;
}
File Properties
The filesystem library allows you to retrieve file properties, such as its size and last modification time. Here’s how you can get the file size:
std::cout << "File size: " << fs::file_size("example.txt") << " bytes." << std::endl;
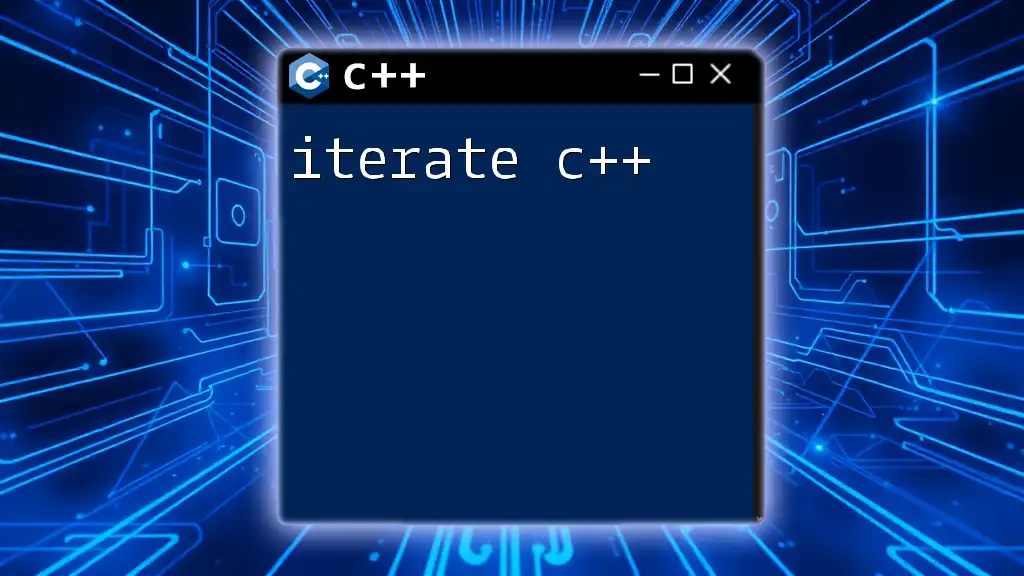
Advanced Features
Iterating Through Directories
Using directory iterators, you can easily navigate through the contents of a directory:
for (const auto& entry : fs::directory_iterator("some_directory")) {
std::cout << entry.path() << std::endl;
}
Handling Exceptions
Exceptions are an integral part of robust file handling. Common exceptions in filesystem operations may include `filesystem_error`. You can catch these exceptions to handle errors gracefully:
try {
fs::remove("non_existent_file.txt");
} catch (const fs::filesystem_error& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
Best Practices for Error Handling
Always validate paths and catch exceptions to ensure your application can handle unexpected scenarios without crashing. Using try-catch blocks is a standard practice to manage filesystem operations effectively.
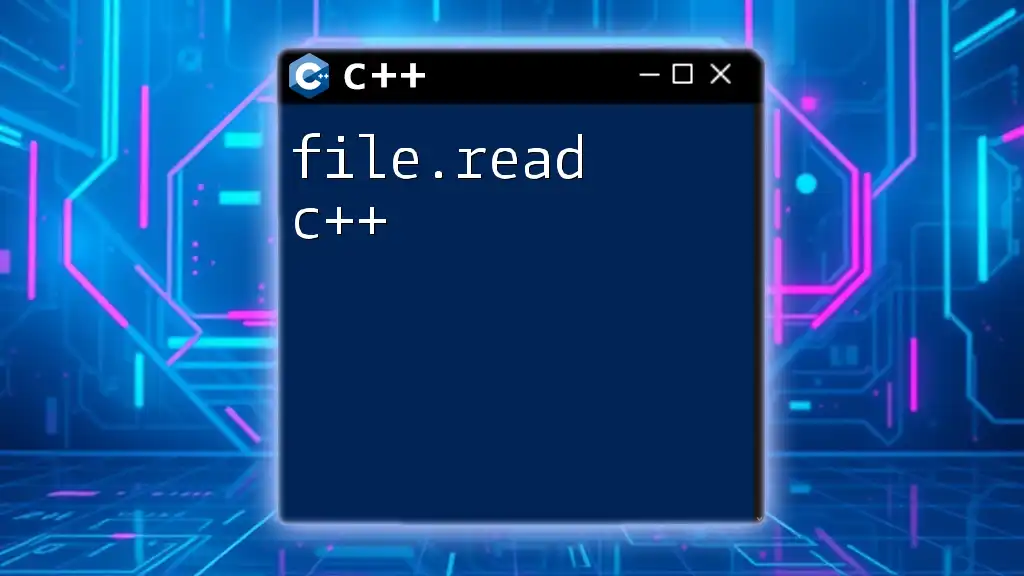
Practical Examples
Example 1: File Backup Utility
This utility copies all files from one directory to another, effectively creating a backup. Here's a simple implementation:
void backupFiles(const fs::path& source, const fs::path& destination) {
fs::create_directory(destination);
for (const auto& entry : fs::directory_iterator(source)) {
if (fs::is_regular_file(entry)) {
fs::copy(entry.path(), destination / entry.path().filename());
std::cout << "Copied: " << entry.path() << std::endl;
}
}
}
This function iterates through each file in the source directory, checking if it’s a regular file, and then copies it to the destination.
Example 2: Simple File Manager
A basic file manager can allow users to list files, copy, and delete them. Here’s a prototype structure:
void listFiles(const fs::path& directory) {
for (const auto& entry : fs::directory_iterator(directory)) {
std::cout << entry.path() << std::endl;
}
}
// Usage
listFiles("my_documents");
This function lists all items in the specified directory, allowing users to see what files and folders are present.
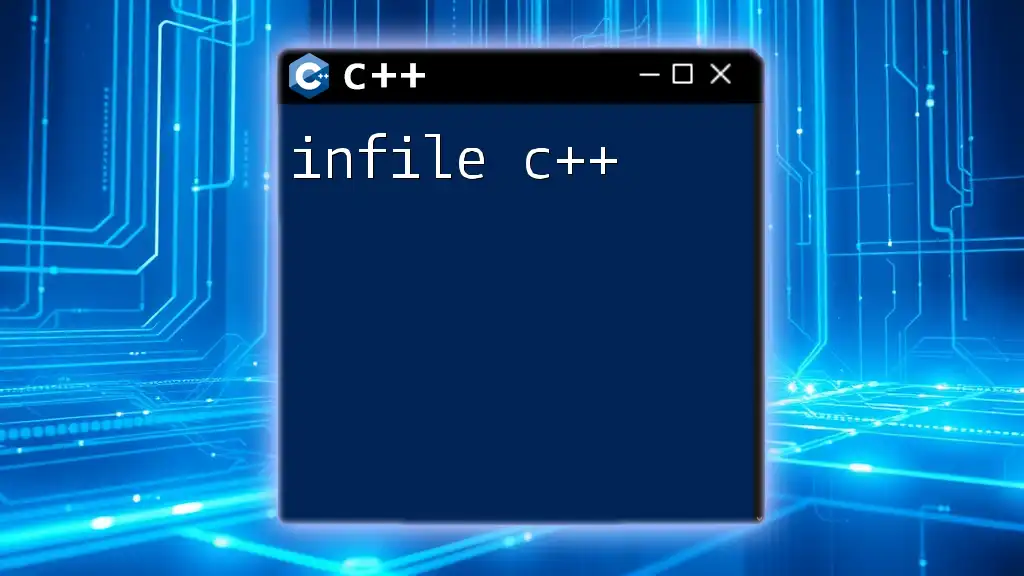
Conclusion
The C++ Filesystem Library greatly simplifies interacting with the filesystem. Through clear syntax and robust functionalities, developers can create applications that effectively manage files and directories. Whether you're backing up files or developing advanced file management systems, the filesystem library provides the tools needed to streamline your operations. Experiment with these examples and take advantage of the rich features offered to enhance your C++ applications.
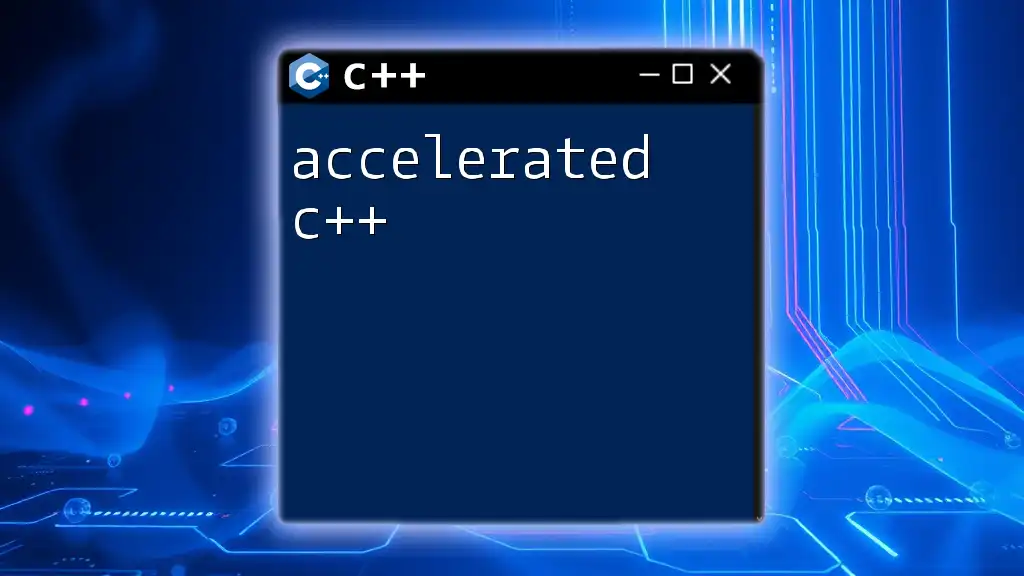
Additional Resources
For further reading and exploration, consider looking into:
- Books and Tutorials: Many online resources provide in-depth tutorials and examples.
- Official C++ Documentation: The official documentation is invaluable for digging deeper into library functionalities and features.