The `sleep` function in C++ is used to pause the execution of a program for a specified number of seconds, helping to manage timing in your applications.
Here's an example code snippet using `std::this_thread::sleep_for` to pause for 2 seconds:
#include <iostream>
#include <thread>
#include <chrono>
int main() {
std::cout << "Sleeping for 2 seconds..." << std::endl;
std::this_thread::sleep_for(std::chrono::seconds(2));
std::cout << "Awake!" << std::endl;
return 0;
}
Understanding the C++ Sleep Function
What is the C++ Sleep Function?
The sleep function in C++ is a critical feature that allows developers to pause the execution of a program for a specified amount of time. This is particularly useful in scenarios such as creating pauses in animations, controlling the execution flow in games, or managing tasks in multi-threaded applications.
One common use case is when building a simple loop that requires intervals between executions to simulate actions or processes. Below is a basic example demonstrating how the sleep function can be used to create a delay:
#include <iostream>
#include <thread>
#include <chrono>
int main() {
std::cout << "Start" << std::endl;
std::this_thread::sleep_for(std::chrono::seconds(2));
std::cout << "End after 2 seconds" << std::endl;
return 0;
}
In this code, the program will pause for 2 seconds between printing "Start" and "End after 2 seconds".
How Sleep Works in C++
The concept of sleeping in C++ is primarily associated with blocking and non-blocking operations. When a thread sleeps, it is blocked from executing until the specified time has elapsed, which allows other threads to execute or conserve system resources.
C++ provides two critical functions for sleeping: `sleep_for` and `sleep_until`. The `sleep_for` function puts the thread to sleep for a specific duration, whereas `sleep_until` suspends the execution until a specific time point.
Here's a brief illustration using both techniques:
#include <iostream>
#include <thread>
#include <chrono>
int main() {
std::cout << "Sleeping for 3 seconds..." << std::endl;
std::this_thread::sleep_for(std::chrono::seconds(3)); // pauses for 3 seconds
std::cout << "Woke up!" << std::endl;
auto wake_time = std::chrono::steady_clock::now() + std::chrono::seconds(2);
std::cout << "Sleeping until a specific time..." << std::endl;
std::this_thread::sleep_until(wake_time); // sleeps until the specific time
std::cout << "Awake after sleep_until!" << std::endl;
return 0;
}

Using Sleep in C++ with Threads
The Role of Threads in C++
Threads enable concurrent execution of tasks within a program, making them essential for achieving optimal performance and responsiveness. When multiple threads are utilized, ensuring they manage shared resources effectively is crucial, and sleep functions often play a significant role in this management.
Implementing Thread Sleep in C++
Making a thread sleep is straightforward in C++. The `std::this_thread::sleep_for` function can be utilized just as easily within a thread as it can in the main execution thread. Here’s an example of how to create a simple thread that sleeps during execution:
#include <iostream>
#include <thread>
void threadFunction() {
std::cout << "Thread sleeping..." << std::endl;
std::this_thread::sleep_for(std::chrono::milliseconds(1000)); // sleeps for 1 second
std::cout << "Thread awake!" << std::endl;
}
int main() {
std::thread t(threadFunction);
t.join(); // Wait for the thread to finish
return 0;
}
In this snippet, a separate thread is created that executes the `threadFunction`, which includes a sleep of 1 second. The `join()` function ensures that the main thread waits for this new thread to complete before proceeding.
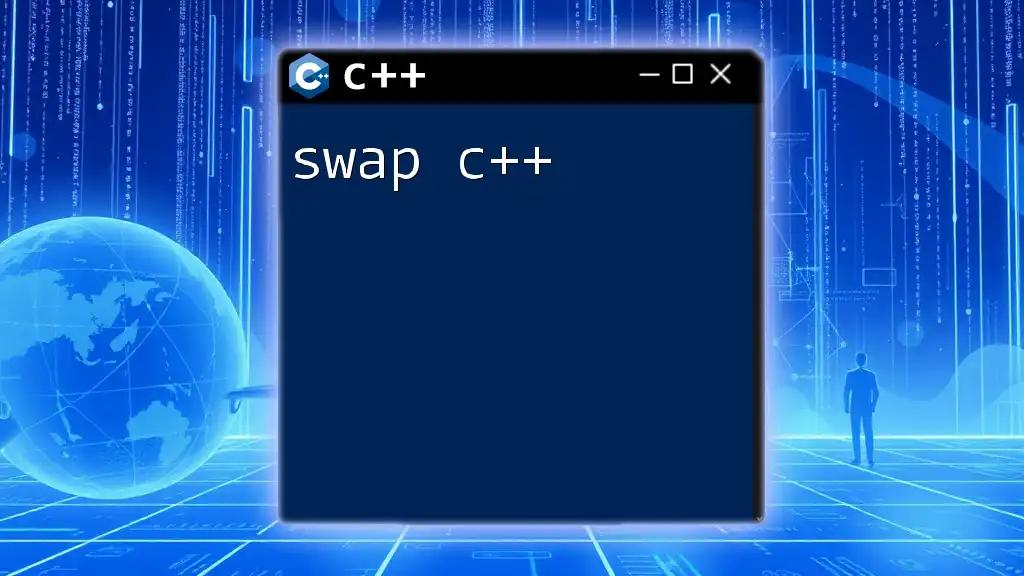
Using Sleep in Linux C++
Linux C++ Sleep Functions
In a Linux environment, C++ programmers have additional options for implementing sleep functionality. Common alternatives include the `usleep` and `nanosleep` functions, which allow for precise control over sleep durations down to microseconds and nanoseconds, respectively.
Example: Sleep in C++ with Linux-specific APIs
Below is an example of how to utilize `usleep` in a Linux environment. Keep in mind that `<unistd.h>` must be included for `usleep` to work:
#include <iostream>
#include <unistd.h> // for usleep
int main() {
std::cout << "Sleeping for 1 second..." << std::endl;
usleep(1000000); // Sleep for 1 million microseconds (1 second)
std::cout << "Awake!" << std::endl;
return 0;
}
In this case, the program pauses for exactly 1 second using `usleep`, demonstrating that various sleep functionalities can exist within different programming contexts.

Practical Applications of Sleep in C++
Real-life Scenarios for Sleep in C++
The sleep c++ function is used across various real-life applications, especially in game development, simulation programs, and even testing frameworks. In game development, a common scenario involves creating a delay for frame updates, ensuring smooth transitions between animations and interactions.
Creating a Simple Game Loop with Sleep
A frequently encountered task in games is maintaining a loop that controls the game state flow. Here’s an example demonstrating how to implement a simple game loop with delays for frame control:
#include <iostream>
#include <thread>
#include <chrono>
int main() {
while (true) {
std::cout << "Game logic executed!" << std::endl;
std::this_thread::sleep_for(std::chrono::milliseconds(33)); // ~30 FPS
}
return 0;
}
In this loop, the program simulates game logic by executing a statement repeatedly, granting approximately 30 frames per second by sleeping for 33 milliseconds each iteration.

Best Practices for Using Sleep in C++
Managing Sleep Duration and Impact
While utilizing sleep functions can be effective, it's essential to understand the impact of the sleep duration on your application. Choosing too long a sleep period can lead to a sluggish user experience, while extremely short durations may lead to high CPU usage or race conditions in multi-threaded applications.
Alternatives to Sleep in C++
In some cases, sleep may not be the best solution, and event-driven programming can be a more suitable alternative. Using tools like condition variables or timer events can help avoid blocking operations altogether, allowing the program to handle other tasks while waiting for specific conditions to be met, leading to a more responsive system.
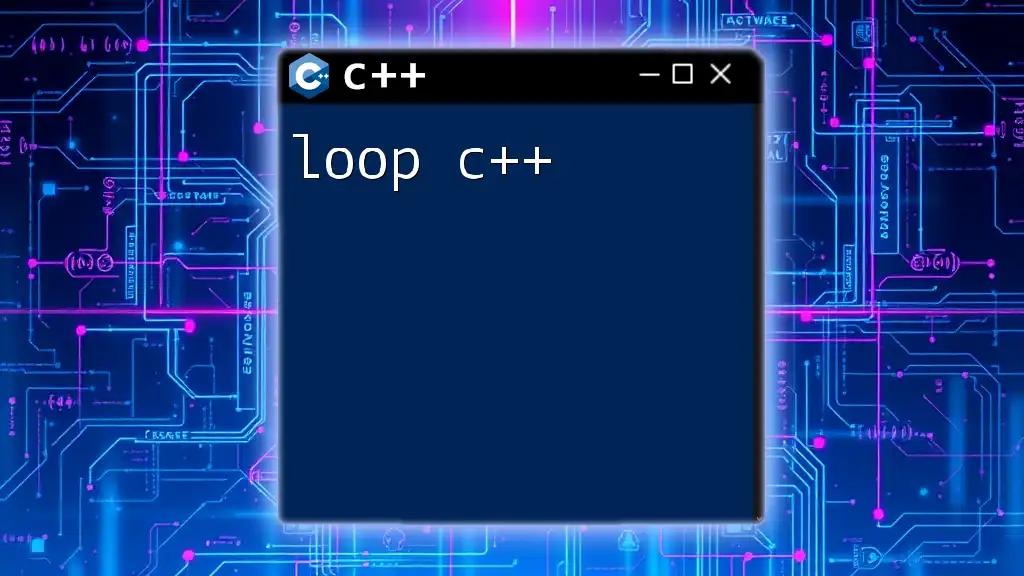
Conclusion
In summary, the sleep c++ function plays an essential role in both single-threaded and multi-threaded applications, facilitating pauses in execution that can help regulate workflow, enhance user experience, and optimize performance. As software becomes increasingly complex, understanding and correctly implementing sleep functionalities will remain a valuable skill for developers. Through various examples, it has become evident how accessible yet powerful sleep functions can be in the C++ programming landscape.
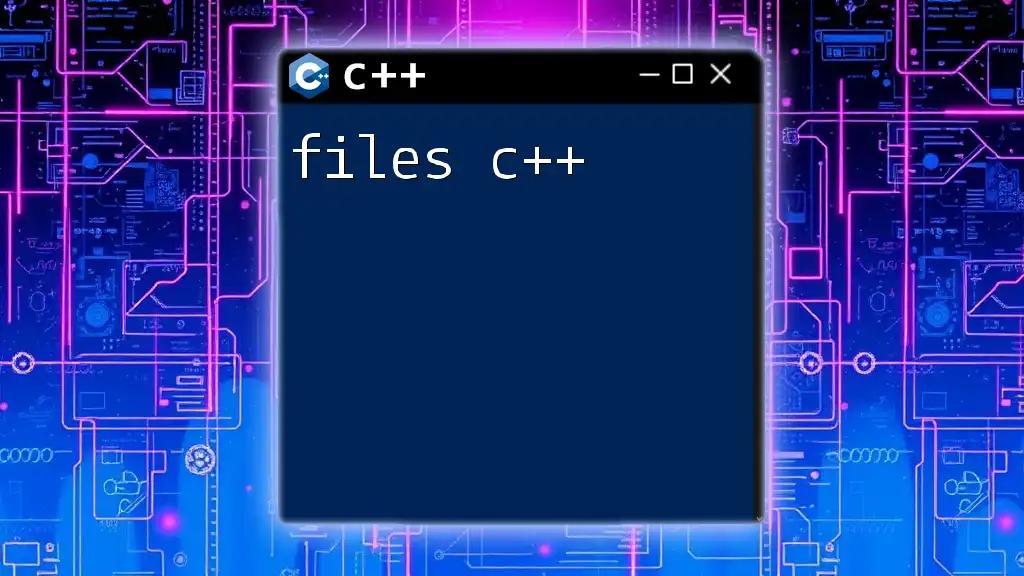
Additional Resources
For further exploration of C++ threading and sleep functions, consider reviewing the official C++ documentation on the `<thread>` library, online courses exploring advanced threading techniques, or tutorials dedicated to sleep in C++. These resources will provide you with a deeper understanding and additional practical applications of the sleep capabilities within C++.