In C++, a table can be represented using a two-dimensional array, allowing you to store and manipulate data in a grid-like structure. Here's a code snippet demonstrating the initialization and access of a 2D array:
#include <iostream>
int main() {
int table[3][3] = { {1, 2, 3}, {4, 5, 6}, {7, 8, 9} };
// Accessing elements of the table
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
std::cout << table[i][j] << " ";
}
std::cout << std::endl;
}
return 0;
}
What is a Table in C++?
A table in C++ commonly refers to an array or a data structure that allows you to store multiple values under a single variable name, organized in a systematic manner. This organization is crucial for efficient data manipulation and retrieval. Tables are used extensively in programming, serving as foundational structures for a variety of applications.

Why Use Tables in C++?
Tables offer several advantages in data organization and storage:
- Efficiency: They allow quick access to data elements via indexing.
- Structured Data: Tables enable the arrangement of data in a structured way, making it more manageable.
- Easy Iteration: With loops, navigating through table elements becomes straightforward.

Types of Tables in C++
One-Dimensional Tables
One-dimensional tables, or arrays, are simple lists of elements that can be accessed by their index. They are foundational to data handling in C++.
Example Code Snippet
#include <iostream>
using namespace std;
int main() {
int numbers[5] = {1, 2, 3, 4, 5};
// Iterating over a one-dimensional table
for (int i = 0; i < 5; i++) {
cout << numbers[i] << " ";
}
return 0;
}
In this code, we declare an array named `numbers` with five integer elements and use a loop to print each element.
Two-Dimensional Tables
Two-dimensional tables, or 2D arrays, represent data in a grid format consisting of rows and columns. They are ideal for representing matrices, tables, and other relationships involving pairs of values.
Example Code Snippet
#include <iostream>
using namespace std;
int main() {
int matrix[3][3] = { {1, 2, 3}, {4, 5, 6}, {7, 8, 9} };
// Accessing and printing elements of a two-dimensional table
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
cout << matrix[i][j] << " ";
}
cout << endl;
}
return 0;
}
In this example, we create a 3x3 matrix and iterate through each element, showcasing how to access and print the values.
Dynamic Tables
When the size of a table cannot be predetermined at compile time, dynamic tables (using dynamic memory allocation) are essential for flexibility.
Example Code Snippet
#include <iostream>
using namespace std;
int main() {
int size;
cout << "Enter the number of elements: ";
cin >> size;
// Creating a dynamic table
int* dynamicArray = new int[size];
// Initializing and accessing the dynamic array
for (int i = 0; i < size; i++) {
dynamicArray[i] = i + 1; // Storing values
}
for (int i = 0; i < size; i++) {
cout << dynamicArray[i] << " ";
}
// Remember to free the allocated memory
delete[] dynamicArray;
return 0;
}
In this code, we ask the user for the size of the table and use `new` to allocate memory dynamically. After usage, it's crucial to release the memory with `delete[]`.
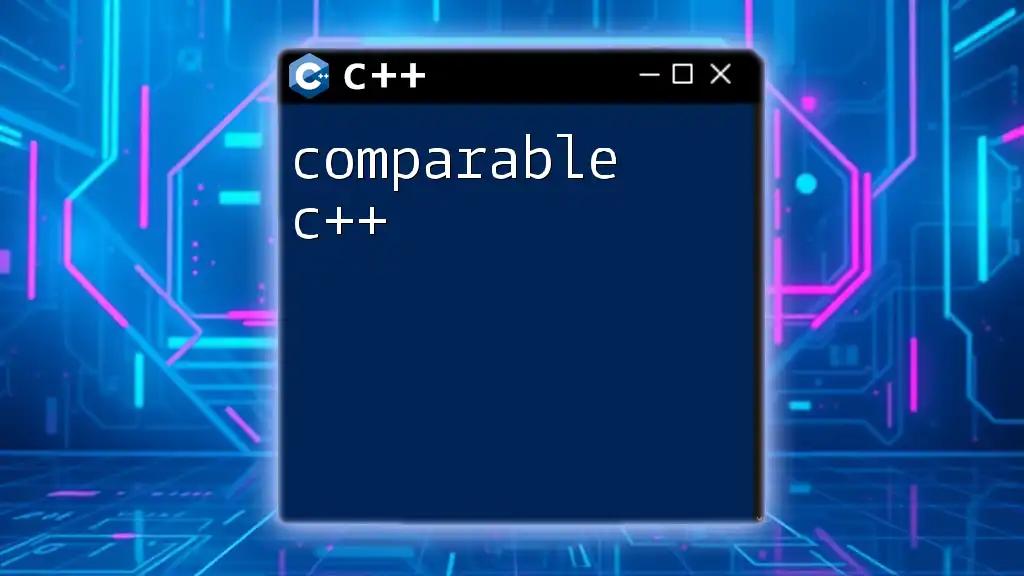
Implementing Tables in C++
Declaring and Initializing Tables
Declaring a table in C++ involves specifying its type and size. There are various methods to initialize tables, such as:
- Static Initialization: Directly during declaration.
- Dynamic Initialization: Assigned during runtime.
Example Code Snippet
int arr[5] = {10, 20, 30, 40, 50}; // Static initialization
int* pArr = new int[5]; // Dynamic initialization
Accessing Table Elements
Accessing elements in a table is done using the index of the element you want to retrieve. In C++, indexing starts at zero, meaning the first element is at index 0.
Example Code Snippet
#include <iostream>
using namespace std;
int main() {
int values[5] = {5, 10, 15, 20, 25};
// Accessing and modifying elements
values[0] = 100; // Modifying the first element
cout << "First Element: " << values[0] << endl; // Accessing the first element
return 0;
}
This snippet demonstrates how to change the first element of the array and print it out.

Advanced Table Operations
Sorting Tables
Sorting is a critical operation, allowing data to be arranged in a particular order. The Bubble Sort algorithm is a simple yet effective way to sort tables.
Example Code Snippet
#include <iostream>
using namespace std;
void bubbleSort(int arr[], int n) {
for(int i = 0; i < n - 1; i++)
for(int j = 0; j < n - i - 1; j++)
if(arr[j] > arr[j + 1]) {
// Swap
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
int main() {
int array[5] = {64, 34, 25, 12, 22};
bubbleSort(array, 5);
cout << "Sorted array: ";
for (int i = 0; i < 5; i++)
cout << array[i] << " ";
return 0;
}
In this example, we implemented a basic Bubble Sort for a one-dimensional array and printed the sorted results.
Searching Tables
Searching for elements efficiently is important. Techniques such as Linear Search and Binary Search have distinct use cases.
Example Code Snippet for Binary Search
#include <iostream>
using namespace std;
int binarySearch(int arr[], int size, int target) {
int left = 0, right = size - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid] == target) return mid;
if (arr[mid] < target) left = mid + 1;
else right = mid - 1;
}
return -1; // Target not found
}
int main() {
int arr[5] = {10, 20, 30, 40, 50};
int index = binarySearch(arr, 5, 30);
if (index != -1)
cout << "Element found at index: " << index << endl;
else
cout << "Element not found!" << endl;
return 0;
}
This code demonstrates an efficient Binary Search on a pre-sorted table, returning the index of the target element.
Multi-Dimensional Table Operations
Dealing with multi-dimensional tables, you can apply the same principles but with more complexity. Operations such as addition of two matrices require systematic iteration through rows and columns.
Example Code Snippet
#include <iostream>
using namespace std;
void addMatrices(int first[3][3], int second[3][3]) {
int result[3][3];
for (int i = 0; i < 3; i++)
for (int j = 0; j < 3; j++)
result[i][j] = first[i][j] + second[i][j];
// Printing result
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++)
cout << result[i][j] << " ";
cout << endl;
}
}
int main() {
int matrix1[3][3] = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
int matrix2[3][3] = {{9, 8, 7}, {6, 5, 4}, {3, 2, 1}};
addMatrices(matrix1, matrix2);
return 0;
}
This sample function, `addMatrices`, performs element-wise addition of two 3x3 matrices, highlighting multi-dimensional operations effectively.

Best Practices for Using Tables in C++
Memory Management
When you allocate memory dynamically for tables, it's vital to manage it correctly to prevent memory leaks. Always use `delete` or `delete[]` for deallocation.
Example Code Snippet
int* myArray = new int[size];
// ... use the array ...
delete[] myArray; // Freeing allocated memory
Performance Considerations
When using tables, consider their size and dimension carefully. Large tables can lead to performance issues if not managed properly. Strive for optimal sizing and dimensioning to ensure your programs remain efficient.
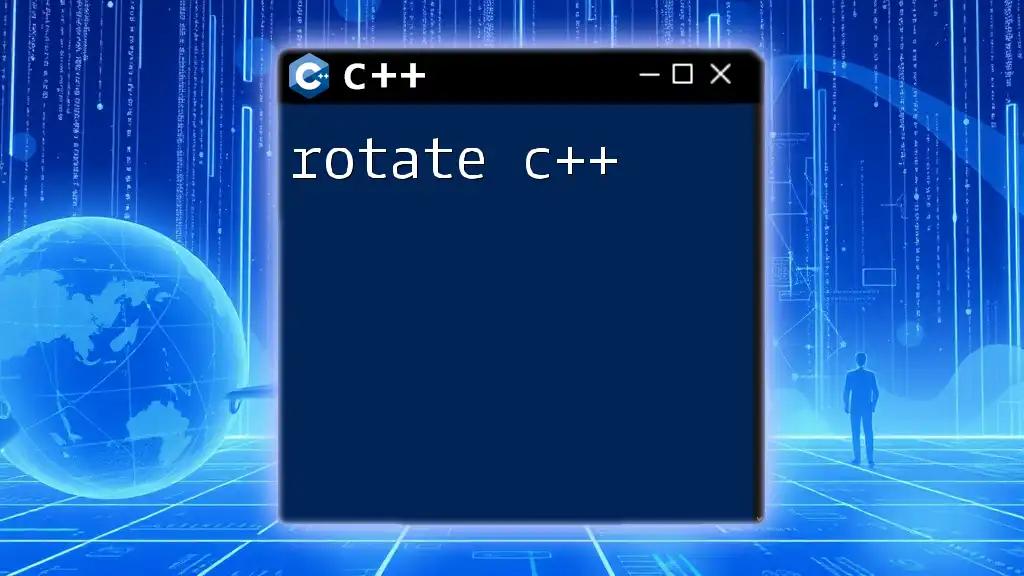
Common Mistakes to Avoid
Index Out-of-Bounds Errors
Accessing elements outside the predefined range of a table can lead to runtime errors. Always ensure your indices are within valid limits.
Improper Memory Management
Errors in memory management can lead to leaks or crashes. Always ensure every `new` has a corresponding `delete` and check for null pointers before dereferencing.

Conclusion
In summary, using tables in C++ can streamline data management and provide robust solutions for various programming challenges. Understanding the implementation, operations, and pitfalls of tables will enhance your competency in C++.

Next Steps
As you delve deeper into C++, explore other data structures such as vectors, lists, and maps for a more comprehensive understanding of handling data efficiently in diverse scenarios.