A hash table in C++ is a data structure that implements an associative array, allowing for efficient data retrieval using a key-value pair method.
Here’s a simple example demonstrating how to use a hash table with `std::unordered_map`:
#include <iostream>
#include <unordered_map>
int main() {
std::unordered_map<std::string, int> hashTable;
hashTable["apple"] = 1;
hashTable["banana"] = 2;
std::cout << "The value associated with 'apple' is: " << hashTable["apple"] << std::endl;
return 0;
}
What is a Hash Table?
A hash table is a data structure that implements an associative array abstract data type, a structure that can map keys to values. Hash tables are designed to provide fast data retrieval, making them an excellent choice when quick lookups are necessary. The underlying mechanism uses a hash function to compute an index into an array of buckets or slots, from which the desired value can be found.
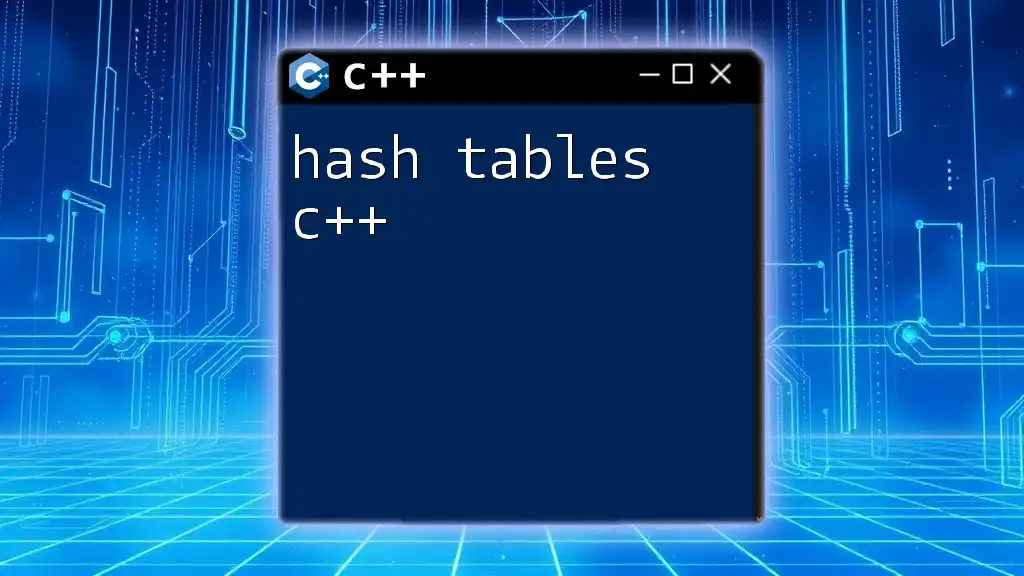
Why Use Hash Tables?
Hash tables offer several compelling benefits:
- Constant Time Complexity: The average time complexity for lookup, insertion, and deletion operations is O(1), making hash tables exceptionally efficient.
- Flexible Data Handling: They can efficiently manage large datasets with dynamic insertions and deletions.
- Use Cases: They are commonly used in databases, caches, and sets where quick access to elements is crucial.
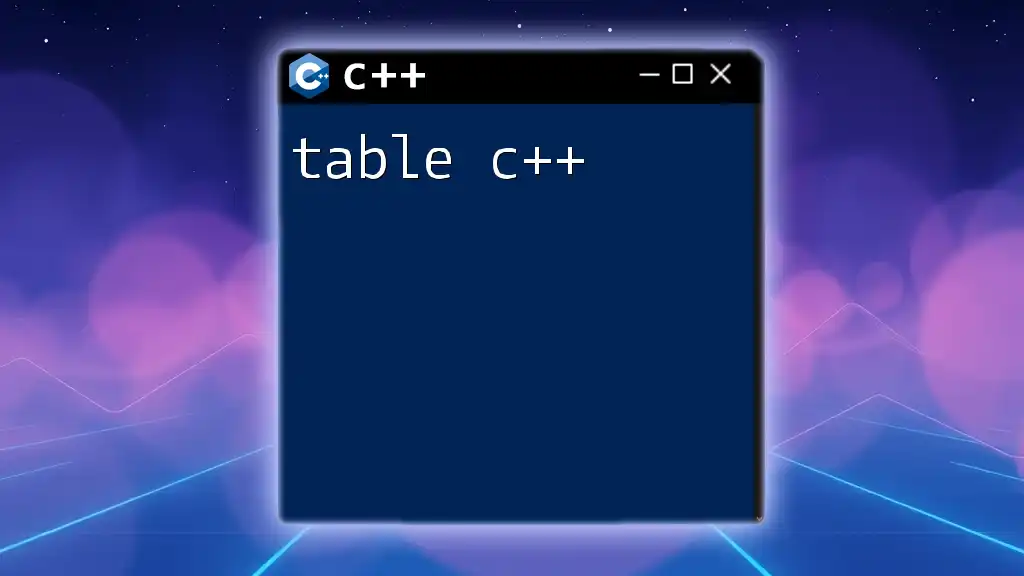
Understanding C++ Hash Tables
Key Components of a Hash Table
A hash table consists of keys and values. Each key is unique, and the hash table stores a mapping between each key and its corresponding value.
Buckets serve as storage units, and the number of buckets usually determines the capacity of the hash table. Each bucket can hold multiple key-value pairs, particularly in cases of collision.
Hash Functions
A hash function is a critical component of a hash table. It transforms the key into an index in the array of buckets. The key characteristics of a good hash function include:
- Deterministic: The same input must always produce the same output.
- Uniform Distribution: It should evenly distribute keys across buckets to minimize collisions.
- Efficient Computation: The function should be computationally inexpensive.
Common hash functions in C++ include modulus operations, polynomial accumulation, and multiplicative hashing methods.
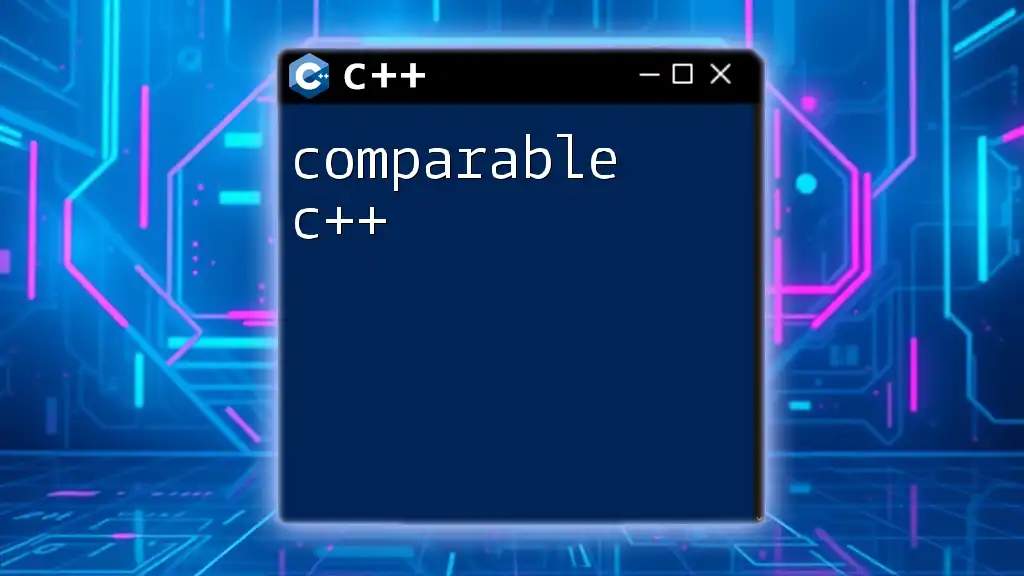
Implementing a Hash Table in C++
Basic Structure of a Hash Table Class
Below is a basic structure for a hash table class in C++:
template <typename K, typename V>
class HashTable {
private:
int capacity;
std::vector<std::list<std::pair<K, V>>> table;
public:
HashTable(int size) : capacity(size) {
table.resize(capacity);
}
~HashTable() {
// Optional cleanup if necessary
}
The `HashTable` class uses a vector of lists to handle collisions via chaining.
Member Functions Overview
Insert Function
The insert function adds a key-value pair to the hash table. If the key already exists, it updates the value.
void insert(K key, V value) {
int index = hashFunction(key) % capacity;
auto& cell = table[index];
auto it = std::find_if(cell.begin(), cell.end(), [&key](const std::pair<K, V>& pair) {
return pair.first == key;
});
if (it != cell.end()) {
it->second = value; // Update existing value
} else {
cell.emplace_back(key, value); // Insert new key-value pair
}
}
Find Function
The find function retrieves the value associated with a given key:
V find(K key) {
int index = hashFunction(key) % capacity;
auto& cell = table[index];
auto it = std::find_if(cell.begin(), cell.end(), [&key](const std::pair<K, V>& pair) {
return pair.first == key;
});
if (it != cell.end()) {
return it->second;
} else {
throw std::runtime_error("Key not found");
}
}
Delete Function
The delete function removes a key-value pair from the hash table:
void remove(K key) {
int index = hashFunction(key) % capacity;
auto& cell = table[index];
auto it = std::find_if(cell.begin(), cell.end(), [&key](const std::pair<K, V>& pair) {
return pair.first == key;
});
if (it != cell.end()) {
cell.erase(it); // Remove key-value pair
}
}
Example Implementation of a Hash Table in C++
Combining the methods we’ve discussed, here’s a complete example of a hash table implementation:
#include <iostream>
#include <vector>
#include <list>
#include <utility>
#include <stdexcept>
#include <algorithm>
template <typename K, typename V>
class HashTable {
private:
int capacity;
std::vector<std::list<std::pair<K, V>>> table;
int hashFunction(K key) {
return std::hash<K>()(key);
}
public:
HashTable(int size) : capacity(size) {
table.resize(capacity);
}
void insert(K key, V value) {
int index = hashFunction(key) % capacity;
auto& cell = table[index];
auto it = std::find_if(cell.begin(), cell.end(), [&key](const std::pair<K, V>& pair) {
return pair.first == key;
});
if (it != cell.end()) {
it->second = value; // Update existing value
} else {
cell.emplace_back(key, value); // Insert new key-value pair
}
}
V find(K key) {
int index = hashFunction(key) % capacity;
auto& cell = table[index];
auto it = std::find_if(cell.begin(), cell.end(), [&key](const std::pair<K, V>& pair) {
return pair.first == key;
});
if (it != cell.end()) {
return it->second;
} else {
throw std::runtime_error("Key not found");
}
}
void remove(K key) {
int index = hashFunction(key) % capacity;
auto& cell = table[index];
auto it = std::find_if(cell.begin(), cell.end(), [&key](const std::pair<K, V>& pair) {
return pair.first == key;
});
if (it != cell.end()) {
cell.erase(it); // Remove key-value pair
}
}
};
int main() {
HashTable<std::string, int> ht(10);
ht.insert("apple", 50);
std::cout << "Value for 'apple': " << ht.find("apple") << std::endl;
ht.remove("apple");
try {
ht.find("apple");
} catch (const std::runtime_error& e) {
std::cout << e.what() << std::endl; // Outputs "Key not found"
}
return 0;
}
In this example, we define a `HashTable` template class that supports basic operations: insertion, deletion, and searching. The hash function utilizes the standard library's hash function for efficient key indexing.
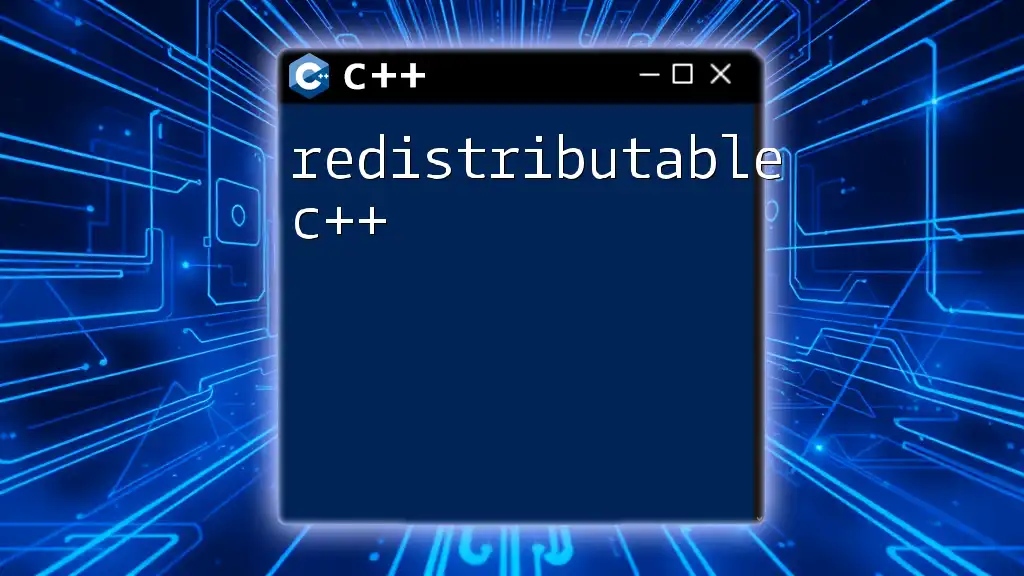
Performance Analysis of Hash Tables
Time Complexity
The performance of hash tables can vary based on several factors, including the quality of the hash function and the load factor (number of elements/buckets).
- Average Case: O(1) for insertion, deletion, and search.
- Worst Case: O(n) when all keys collide (all in one bucket), although this is rare with a good hash function.
Space Complexity
The space complexity of a hash table largely depends on its capacity and the number of entries. In general, it is O(n) where n is the number of key-value pairs, plus the overhead for buckets.
Trade-offs often exist between space and performance; allocating more memory can lead to fewer collisions and faster access times.
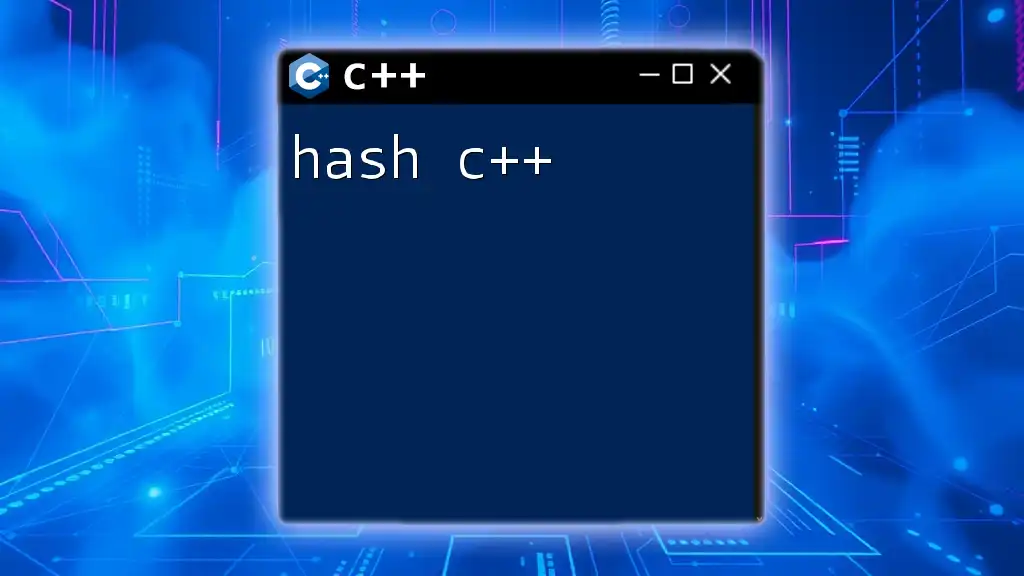
Common Pitfalls in Hash Table Implementation
Collision Handling Issues
Collisions occur when two keys hash to the same index. Chaining is one common strategy for collision resolution where multiple entries can exist in the same bucket. If mishandled, collisions can significantly deteriorate the table's efficiency.
It is crucial to implement a robust collision resolution strategy, such as chaining or open addressing, to ensure consistent performance.
Inefficient Hash Functions
A poor hash function can lead to clustering of keys, causing performance degradation. Avoid using overly simplistic functions which result in non-uniform distributions.
Tips for designing effective hash functions include:
- Combine multiple sources of information from the key.
- Employ multiplicative hashing or other proven methods.
- Test your function with various key distributions.
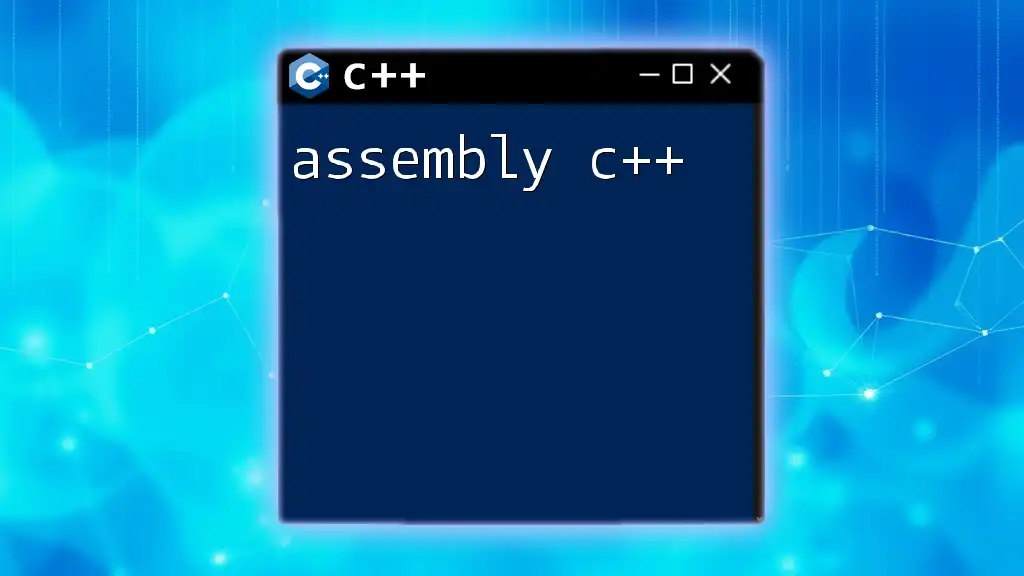
Advanced Topics in C++ Hash Tables
Dynamic Resizing
As a hash table gets filled, performance may suffer due to increased collisions. Dynamic resizing involves creating a larger array and rehashing existing elements when a certain load factor threshold is reached. Implementing this can ensure that the hash table maintains efficiency as it grows.
Using STL's Unordered Map
C++ provides a built-in solution for hash tables in the form of `std::unordered_map`. This offers benefits such as:
- Reduced implementation time.
- Optimized performance, as it is part of the standard library.
- Exception handling and other features out-of-the-box.
However, when considering `std::unordered_map`, be aware of its abstraction which may not allow for custom data entry optimizations or specific memory usage strategies.
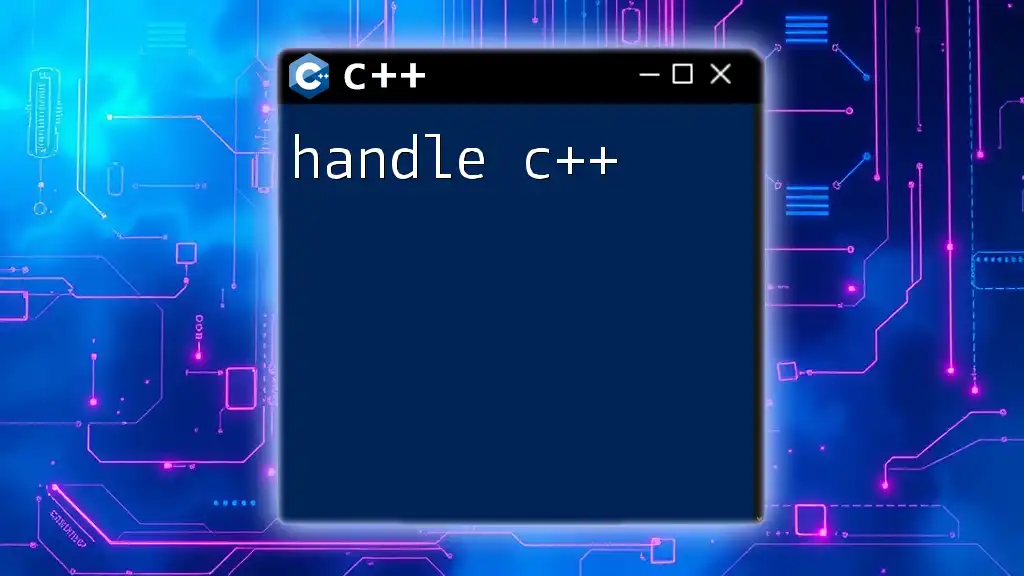
Conclusion
In summary, hash tables are a powerful and efficient means of storing and retrieving data in C++. Their support for quick lookup and dynamic data handling makes them a staple in computer science. Understanding their implementation, performance characteristics, and potential pitfalls is essential for leveraging their capabilities effectively.
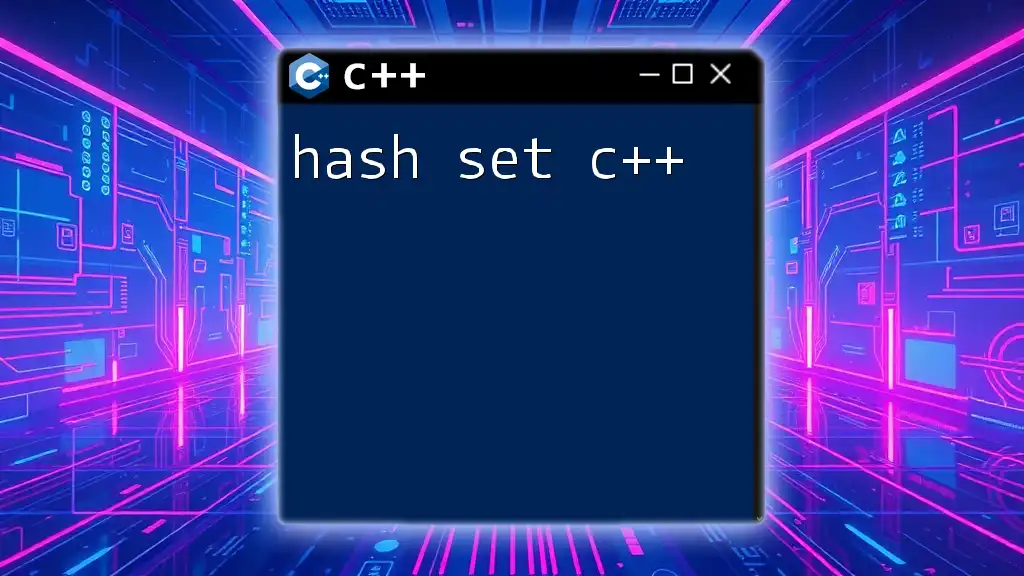
Additional Resources
To further enhance your understanding of hash tables, consider exploring the following resources:
- Books: Look for titles on data structures and algorithms that delve into hash tables.
- Websites: Utilize platforms like GeeksforGeeks, tutorialspoint, and Cplusplus.com.
- Courses: Online courses on C++ programming often cover hash table implementations.
By practicing the implementation of hash tables in various applications, you will solidify your understanding and become proficient in using these vital data structures.