A hash set in C++ is an unordered collection of unique elements that provides average constant-time complexity for insertions, deletions, and lookups, leveraging hash functions for efficient storage.
Here’s a simple example using `std::unordered_set`:
#include <iostream>
#include <unordered_set>
int main() {
std::unordered_set<int> mySet = {1, 2, 3, 4, 5};
mySet.insert(6); // Adding an element
mySet.erase(3); // Removing an element
for (int num : mySet) {
std::cout << num << " "; // Output will be unordered
}
return 0;
}
What is a Hash Set?
A hash set is a collection of unique elements that allows for efficient data retrieval. In programming, this data structure is invaluable for scenarios requiring unique item storage and quick lookups. Hash sets leverage the hashing technique to achieve constant average time complexity for operations like insertion, deletion, and searching.
Why Use Hash Sets in C++?
When it comes to choosing a data structure, the importance of performance and memory efficiency cannot be understated. Hash sets in C++ provide numerous advantages, such as:
- Performance: With average case time complexities of O(1) for insertions and lookups, hash sets excel in situations that require frequent access to elements.
- Uniqueness: Automatic management of unique entries means that duplicates are inherently avoided.
- Flexibility: Hash sets allow storage of various data types, accommodating a wide range of applications.
Real-world applications of hash sets include caching results, maintaining unique collections of items, and implementing algorithms where quick membership checks are essential.
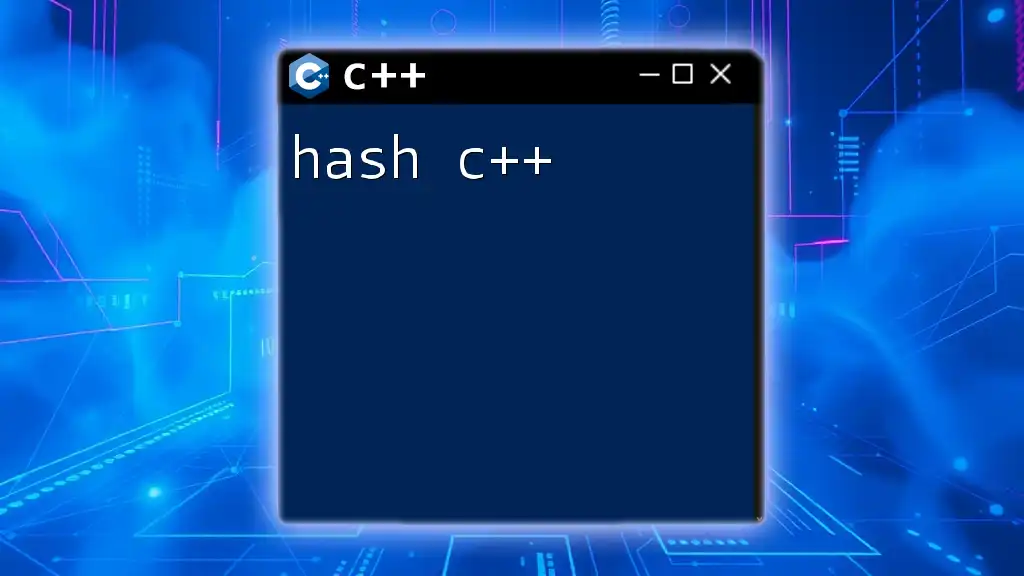
Overview of hashset C++
What is std::unordered_set?
In C++, the std::unordered_set is a part of the Standard Template Library (STL) that implements a hash set. Its primary features include:
- No order: Unlike `std::set`, which maintains a sorted order, `std::unordered_set` does not guarantee any particular order of elements.
- Using hashes: Behind the scenes, it uses hash functions to manage elements, providing better average performance for insertion and lookup operations.
Understanding the properties of `std::unordered_set` allows developers to effectively use this data structure in applications where order does not matter but performance does.
When to Use std::unordered_set
There are specific scenarios where `std::unordered_set` shines:
- Frequent Insertions / Deletions: If your application requires handling a lot of additions or removals, this structure is ideal.
- Checking Membership: When you need to check if an item exists in a collection rapidly, hash sets are the go-to.
- Unique Collection Management: Managing a collection of items where duplicates are not allowed is seamless with hash sets.
In terms of time complexity, the operations in `std::unordered_set` are remarkably efficient:
- Insert: O(1) on average
- Find: O(1) on average
- Erase: O(1) on average
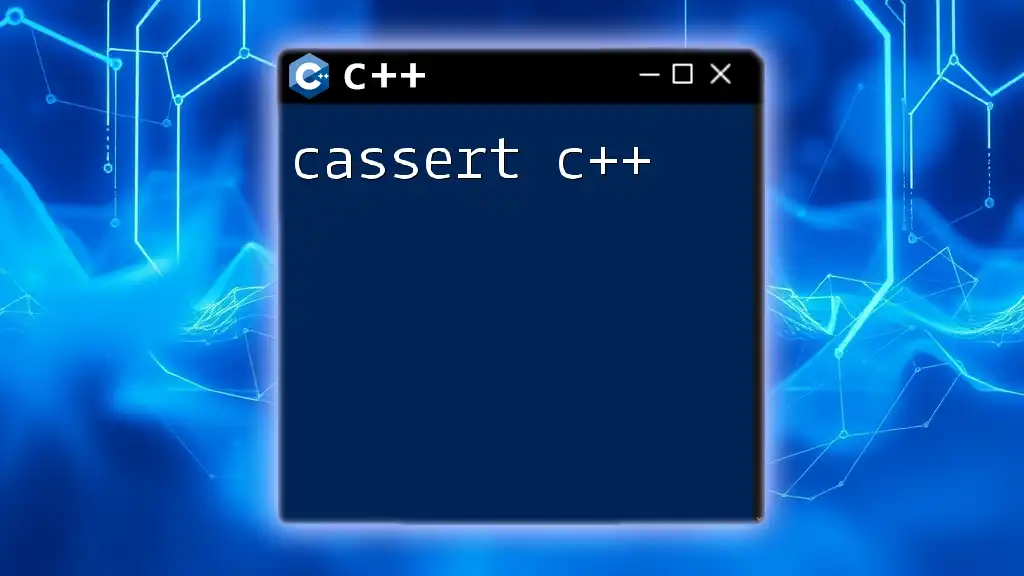
Basic Operations in Hash Sets
Inserting Elements
Adding elements to a hash set is straightforward. The `insert` function can be used like so:
#include <iostream>
#include <unordered_set>
int main() {
std::unordered_set<int> mySet;
mySet.insert(10);
mySet.insert(20); // 20 is added to the set
return 0;
}
Searching for Elements
To determine if an element exists in a hash set, the `find` method is utilized. If the element is found, an iterator to that element is returned; otherwise, it returns `end()`.
if (mySet.find(10) != mySet.end()) {
std::cout << "Found!" << std::endl;
} else {
std::cout << "Not found!" << std::endl;
}
Deleting Elements
Removing elements is done using the `erase` method. This allows you to specify which item you want to remove from the collection.
mySet.erase(10); // Removes the element with value 10
Checking Size
To check how many elements are stored in the hash set, use the `size()` method. It returns the count of unique items.
std::cout << "Size of mySet: " << mySet.size() << std::endl;
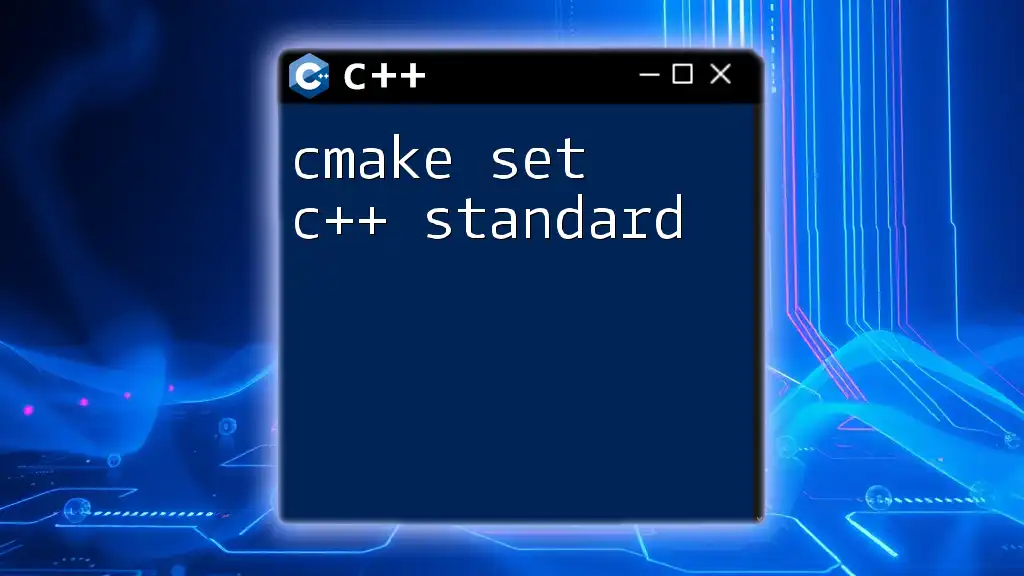
Advanced Features of hashset C++
Iterating Over a Hash Set
Iterating through a hash set is easy and can be done using a range-based for loop. Here's an example:
for (const auto& elem : mySet) {
std::cout << elem << " ";
}
This iterates over each element in `mySet` and prints it out.
Custom Hash Functions
In some cases, you may need to store custom data types in your hash set. By defining your own hash function, you can customize how elements are hashed in your collection:
struct CustomHash {
std::size_t operator()(const std::string& key) const {
return std::hash<std::string>()(key);
}
};
std::unordered_set<std::string, CustomHash> myCustomSet;
Handling Collisions
Collisions occur when two different elements hash to the same index. Hash sets manage these through techniques like chaining or open addressing. Understanding how your hash set resolves these issues can influence your design decisions.
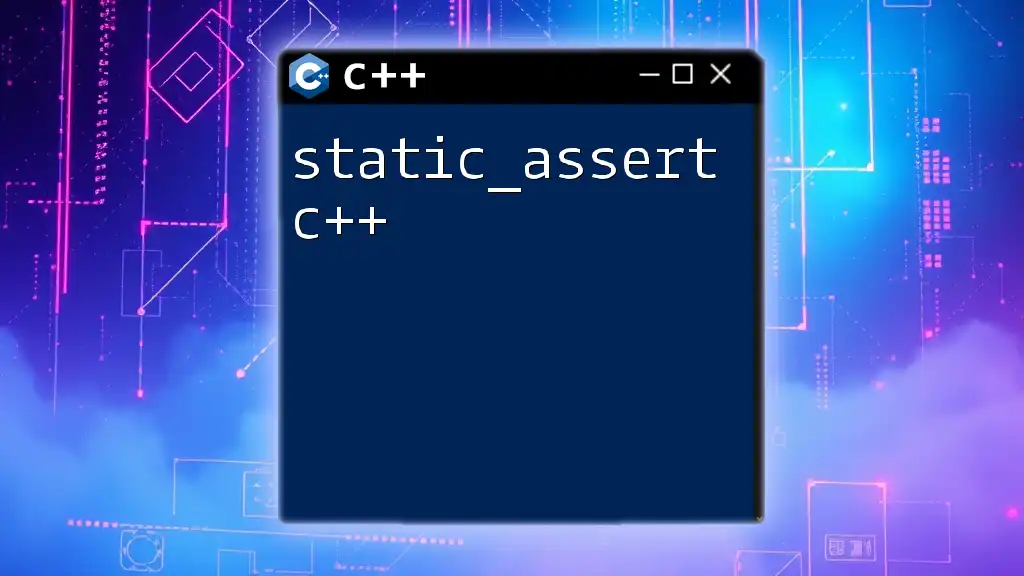
Common Pitfalls and Best Practices
Avoiding Duplicates
A significant benefit of hash sets is that they inherently prevent duplicate entries. This is particularly useful when trying to maintain a unique collection of elements.
Performance Considerations
While hash sets offer excellent average performance, they also have specific memory costs associated with them. When the number of unique elements grows significantly, consider the trade-off between memory usage and performance. Always assess whether a hash set is the best choice for your particular dataset size and application requirements.
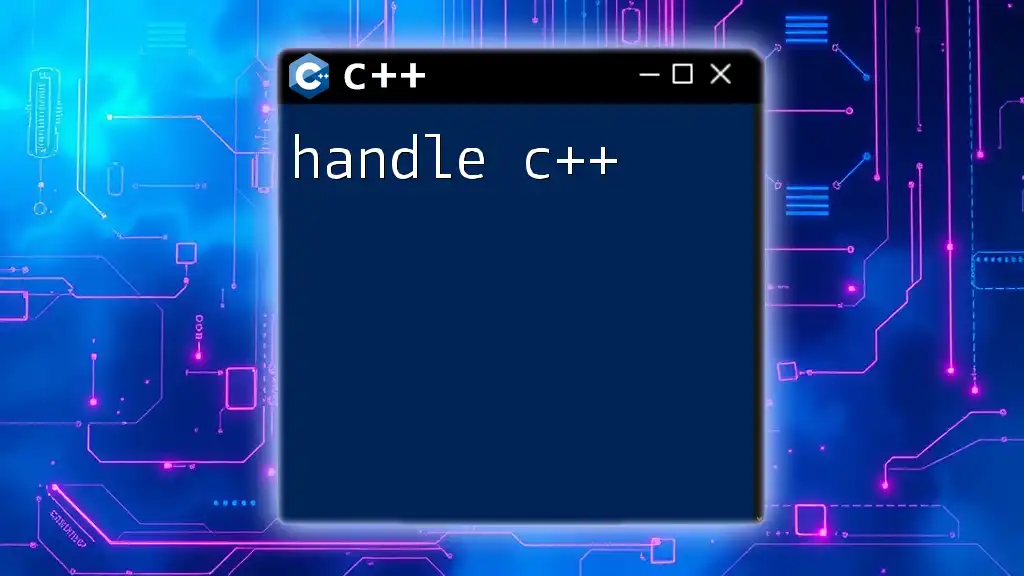
Conclusion
When to Choose Hash Sets for Your C++ Projects
In summary, hash sets (or `std::unordered_set` in C++) should be your go-to data structure when you require rapid access, insertion of unique elements, and performance efficiency in your C++ applications. With a firm grasp of basic operations and advanced features, you are now ready to implement hash sets effectively.
Additional Resources
For further exploration of hash sets, refer to the official [C++ Documentation](https://en.cppreference.com/w/cpp/container/unordered_set) and consider using tools like Visual Studio Code or CLion to experiment with examples and exercises. Happy coding!