To set the C++ standard in a CMake project, use the `set()` command along with `CMAKE_CXX_STANDARD` to specify the desired version.
set(CMAKE_CXX_STANDARD 11) # Sets the C++ standard to C++11
What is CMake?
CMake is a widely-used open-source tool designed to manage the build process of software written in C and C++. It acts as a build system generator, facilitating the configuration of build parameters, environment settings, and compiler specifics.
By defining a `CMakeLists.txt` file, developers can describe their project structure, dependencies, and target settings, allowing CMake to generate native build files for various compilers and platforms. This versatility makes it essential for C++ projects aiming for cross-platform compatibility.
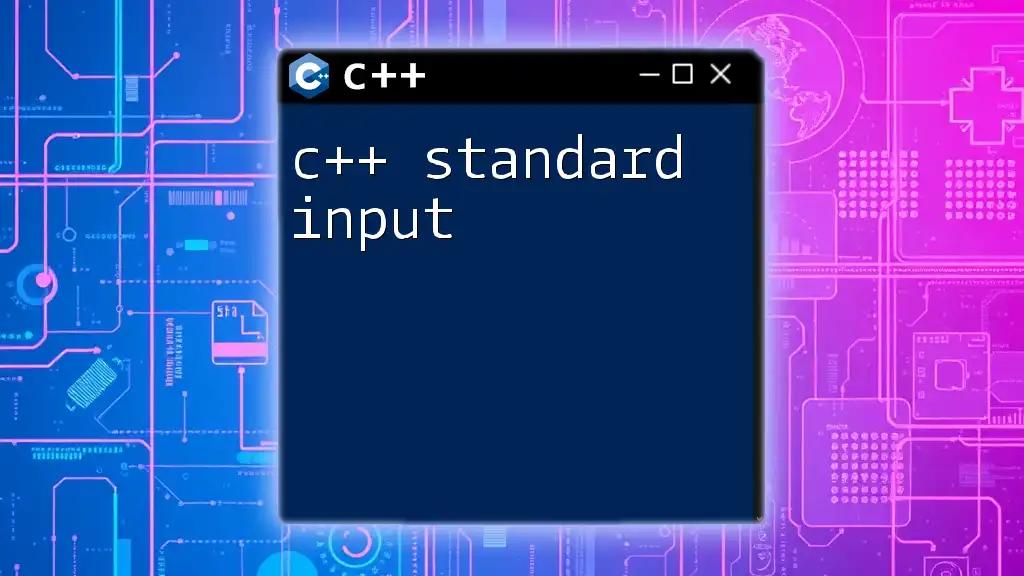
Why Set the C++ Standard?
The C++ standard specifies the syntax and semantics of the C++ programming language, encompassing various features introduced in different versions, such as C++11, C++14, C++17, and C++20. Each version brings enhancements and optimizations that can significantly improve code quality, performance, and efficiency.
Setting the C++ standard in your project is crucial for several reasons:
- Compatibility: Specifying a C++ standard ensures that your code will compile correctly across different compilers. If a newer language feature is available, CMake will raise an error for compilers that do not support that feature, allowing for seamless integration.
- Feature Access: Each version of the C++ standard introduces new capabilities. If your project relies on a specific feature, you must set the appropriate standard to access those enhancements.

How to Set the C++ Standard in CMake
Basic Configuration
To set the C++ standard in your `CMakeLists.txt` file, you can use the `CMAKE_CXX_STANDARD` variable, which allows you to specify the desired version of C++ for your entire project.
Here’s a simple setup:
cmake_minimum_required(VERSION 3.1)
project(MyProject)
set(CMAKE_CXX_STANDARD 14)
In this example:
- `cmake_minimum_required` specifies the minimum version of CMake required to build your project.
- `project` defines the project name.
- `set(CMAKE_CXX_STANDARD 14)` instructs CMake to use the C++14 standard.
Specifying C++ Standard Version
In addition to specifying the standard, it’s essential to manage how strictly CMake adheres to that standard. This is where `CMAKE_CXX_STANDARD_REQUIRED` and `CMAKE_CXX_EXTENSIONS` come into play.
You can enforce the standard as follows:
set(CMAKE_CXX_STANDARD 17)
set(CMAKE_CXX_STANDARD_REQUIRED ON) # Make the standard mandatory
set(CMAKE_CXX_EXTENSIONS OFF) # Use standard C++ without compiler-specific extensions
Explanation:
- `CMAKE_CXX_STANDARD_REQUIRED ON` ensures that if a compiler does not support the specified standard, an error will be produced rather than falling back to an earlier standard.
- `CMAKE_CXX_EXTENSIONS OFF` directs CMake to utilize only the standard C++ syntax, instead of any compiler-specific extensions (such as GNU extensions). This promotes portability and compatibility.
Using Target Properties
CMake also provides the ability to specify the C++ standard on a per-target basis, which is useful when you have multiple executables or libraries within one project that may require different standards.
You can achieve this using the `target_compile_features` command as shown below:
add_executable(MyExecutable main.cpp)
target_compile_features(MyExecutable PRIVATE cxx_std_17) // Specify features per target
By using `PRIVATE`, this requirement applies only to `MyExecutable`, allowing other targets to use a different standard if needed. This granularity also helps in managing dependencies more effectively.

Common Issues and Solutions
Compiler Compatibility
While setting the C++ standard is beneficial, it may lead to compatibility issues with older compilers. Some compilers might not support newer versions of C++, which can hinder your project from compiling accurately.
To address this, it’s crucial to check the documentation for your compiler’s supported C++ standards. This allows you to decide whether to preemptively set the standard to an earlier version or implement conditional settings based on the compiler being used.
CMake Error Messages
When misconfiguring the C++ standard, you might encounter error messages during the build process. These could indicate an unsupported C++ feature based on the specified standard.
For example, if you mistakenly attempt to use a C++20 feature while targeting a compiler that only supports C++14, CMake will issue an error. To resolve this:
- Double-check the C++ standard version you're specifying in your `CMakeLists.txt`.
- Consult the compiler documentation for supported standards.

Best Practices for Setting the C++ Standard
Standardizing Across Projects
To ensure consistency, standardize the C++ version across all modules and components of your project. This not only enhances collaboration between team members but simplifies maintenance and debugging efforts.
Documentation and Comments
Thoroughly document the `CMakeLists.txt` file by adding comments to clarify the rationale behind setting a specific C++ standard or feature. This practice aids future developers (including yourself) in understanding decisions made during the configuration phase.
Testing Across Compilers
Given the varying levels of C++ standard support among different compilers, it’s best practice to test your project with multiple compilers. This helps identify compatibility issues early in the development process, ensuring your application runs smoothly on all intended platforms.

Conclusion
Setting the C++ standard using CMake is a straightforward yet critical component of managing C++ projects effectively. By understanding CMake’s configuration options and best practices, you can leverage the full power of modern C++ and ensure compatibility across different compilers.
Encourage experimentation with various C++ standards and stay updated with the features they offer. By doing so, you enhance your coding experience and make the most of the modern C++ programming landscape.

Additional Resources
For those looking to dive deeper into CMake and its configuration capabilities, consider exploring the following resources:
- The [official CMake documentation](https://cmake.org/documentation/) for detailed guidance on CMake commands and configuration.
- References to the [ISO C++ standards](https://isocpp.org/) for comprehensive insights into each version of the C++ language.
- Additional articles and tutorials focused on CMake’s best practices and modern C++ programming techniques.