The C++ Standard Library is a collection of pre-written classes and functions that provide essential utilities and data structures, allowing developers to efficiently manipulate data and perform various operations in their programs.
Here’s a simple example demonstrating the use of the vector class from the C++ Standard Library:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
Understanding the C++ Standard Library
The C++ Standard Library provides a set of commonly used classes and functions, which can save developers a significant amount of time when writing code. By understanding the key components, you can leverage the power of the `cpp standard library` to enhance your programming efficiency.
Main Components of the C++ Standard Library
Containers are objects that manage collections of other objects. This can include dynamic arrays, lists, trees, and more. The C++ Standard Library defines several key container types:
-
`std::vector`: A dynamic array that allows fast random access and can resize itself automatically. It's an excellent choice for most general purposes.
Example usage:
#include <vector> #include <iostream> int main() { std::vector<int> numbers = {1, 2, 3, 4, 5}; numbers.push_back(6); // Adding an element for (const auto &num : numbers) { std::cout << num << " "; } return 0; }
-
`std::list`: A doubly linked list that allows for fast insertions and deletions from anywhere in the list.
-
`std::map` and `std::set`: Associative containers that store unique keys in a sorted order. Maps associate keys with values, while sets store only keys.
Algorithms in the `cpp standard library` are implemented as template functions, which means they can work with any container type. For example, sorting functions allow you to sort containers easily.
Here’s a quick example of sorting a `std::vector`:
#include <vector>
#include <algorithm>
#include <iostream>
int main() {
std::vector<int> nums = {4, 2, 5, 1, 3};
std::sort(nums.begin(), nums.end()); // Sort the vector
for (const auto &num : nums) {
std::cout << num << " ";
}
return 0;
}
Iterators are used to access the elements of a container. They provide a way to iterate through elements without exposing the underlying structure of the container. The `cpp standard library` defines different types of iterators, including:
- Input Iterators: Allows reading of data.
- Output Iterators: Allows writing of data.
- Bidirectional Iterators: Allows traversal in both directions (e.g., `std::list`).
Here's a basic example of using an iterator:
#include <vector>
#include <iostream>
int main() {
std::vector<int> nums = {1, 2, 3, 4, 5};
for (std::vector<int>::iterator it = nums.begin(); it != nums.end(); ++it) {
std::cout << *it << " ";
}
return 0;
}
Detailed Exploration of Key Components
Containers in Depth
Dynamic Array - `std::vector`
The `std::vector` is one of the most versatile containers available. It can resize automatically, making it suitable for situations where the number of elements is not known in advance. The overhead comes from its potential need to reallocate memory as elements are added.
Associative Containers - `std::map` and `std::set`
Both `std::map` and `std::set` utilize tree structures (commonly red-black trees) to maintain order.
For instance, when you use a map to associate a name with a score:
#include <map>
#include <iostream>
int main() {
std::map<std::string, int> scores;
scores["Alice"] = 90;
scores["Bob"] = 85;
for (const auto& pair : scores) {
std::cout << pair.first << ": " << pair.second << "\n";
}
return 0;
}
In this code, the names are stored as unique keys, paired with integer scores.
Specialized Containers - `std::array` and `std::deque`
`std::array` is a fixed-size array that provides the benefits of a standard array with added functionality. On the other hand, `std::deque` allows fast insertions and deletions from both ends.
Algorithms and Their Importance
The importance of using algorithms cannot be overstated, as they can greatly improve the performance and readability of your code. The C++ Standard Library provides a wide range of algorithms for various tasks, such as searching, sorting, and modifying collections.
A commonly used search algorithm is `std::find`, which searches for an element within a container:
#include <vector>
#include <algorithm>
#include <iostream>
int main() {
std::vector<int> nums = {1, 2, 3, 4, 5};
auto it = std::find(nums.begin(), nums.end(), 3);
if (it != nums.end()) {
std::cout << "Found: " << *it << "\n";
}
return 0;
}
Iterators Explained
Iterators simplify the process of iterating through a container. They act as a bridge between containers and algorithms. By using iterators, you can write generic code that works with any container type in C++. Different types of iterators serve different purposes:
- Forward Iterators: Can move only in one direction.
- Bidirectional Iterators: Can move in both directions.
- Random Access Iterators: Can jump to any position directly (like iterators for `std::vector`).
Advanced Usage of the C++ Standard Library
Smart Pointers and Memory Management
One of the more advanced components of the `cpp standard library` is its memory management features, particularly smart pointers. Smart pointers, like `std::unique_ptr`, `std::shared_ptr`, and `std::weak_ptr`, help manage the lifetime of dynamically allocated objects and prevent memory leaks.
Example:
#include <memory>
#include <iostream>
int main() {
auto ptr = std::make_unique<int>(42); // Creates a unique pointer
std::cout << *ptr << "\n";
return 0;
}
Exception Handling in Standard Library
Proper error handling is critical in software development. The C++ Standard Library provides robust support for exceptions, allowing you to catch errors and handle them gracefully.
An example:
#include <iostream>
#include <stdexcept>
int main() {
try {
throw std::runtime_error("An error occurred!");
} catch (const std::runtime_error &e) {
std::cout << "Caught: " << e.what() << "\n";
}
return 0;
}
Performance Considerations when Using C++ Standard Library
While the `cpp standard library` offers an extensive range of tools and functionalities, its performance can vary significantly based on your choices. It is essential to understand the complexity of different algorithms and the performance characteristics of various containers. For instance, while `std::vector` offers fast random access, its insertion and deletion can be costly if they occur in the middle of the vector. On the other hand, `std::list` can perform those operations more efficiently.
Best Practices for Using the C++ Standard Library
To make the most out of the `cpp standard library`, consider the following best practices:
- Consistent Naming and Style Guidelines: Adopt a consistent naming and style convention to improve readability.
- Memory Management Tips: Utilize smart pointers to mitigate memory management issues.
- Utilizing Header Files Properly: Always include the necessary headers such as `#include <vector>` or `#include <algorithm>` to ensure correct usage.
- Version Control and Compatibility: Be mindful of newer C++ standards (like C++11, C++14). Stay updated on changes to ensure code compatibility and leverage new features.
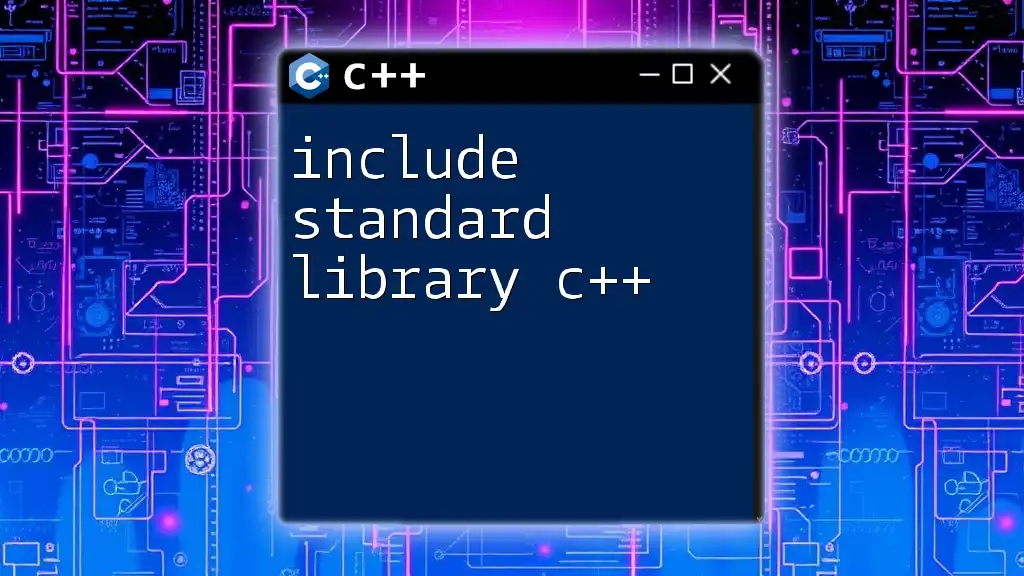
Conclusion
By understanding the C++ Standard Library, you empower yourself with the ability to write efficient, maintainable, and powerful C++ code. Explore all its components and take advantage of this invaluable resource in your programming journey.
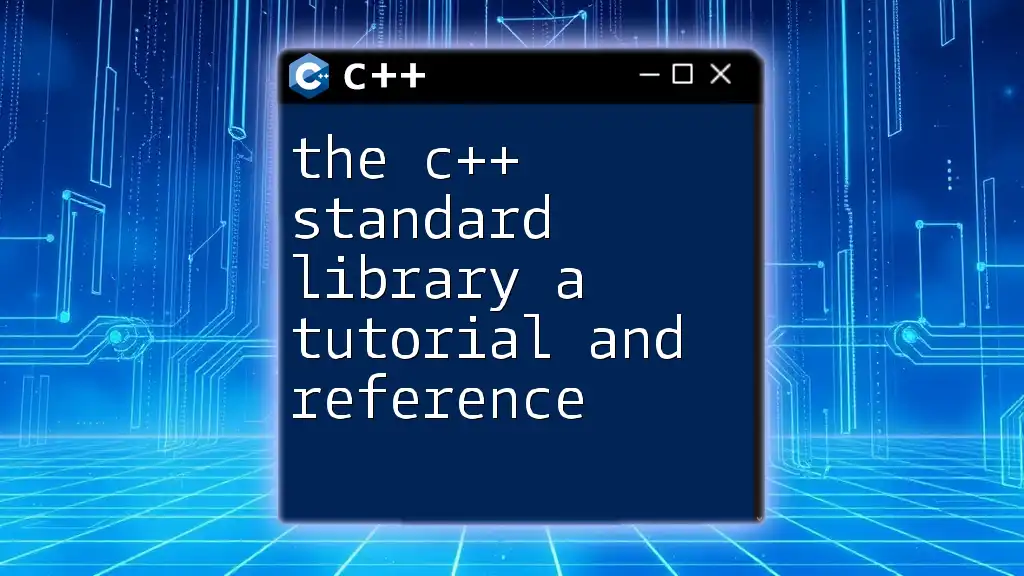
Additional Resources
For further learning, consider checking out books and online platforms that delve into more advanced topics and real-world applications of the `cpp standard library`. Practice consistently, and soon you'll find yourself leveraging the full potential of these powerful tools in your projects!
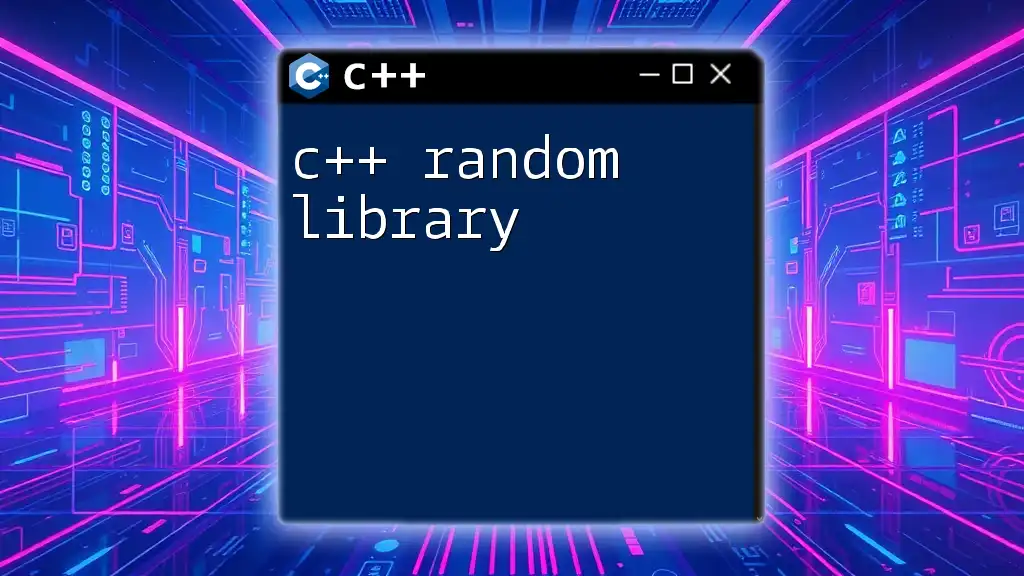
FAQs About the C++ Standard Library
- What is the difference between C++ Standard Library and the C++ Standard Template Library? The Standard Library encompasses all standard components, including basic input/output, whereas the STL primarily refers to the algorithms and containers.
- How to get started with the C++ Standard Library? Begin with basic tutorials, practice using containers and algorithms, and progressively tackle more complex projects.
- What are common problems encountered when learning the C++ Standard Library, and how are they resolved? Beginners often struggle with understanding iterators and memory management; resolving these issues typically involves targeted practice and studying examples.