In C++, including the standard library allows you to access its rich set of built-in functions and classes essential for program development.
#include <iostream>
Understanding the Standard Library in C++
What is the Standard Library?
The C++ Standard Library is a comprehensive set of classes and functions that allows programmers to perform common tasks efficiently and effectively. It includes a wide array of components, such as input/output facilities, data structures (like vectors and lists), algorithms, and more. Leveraging the standard library can drastically improve the quality of your code, enhance productivity, and ensure compatibility across different platforms.
History and Evolution
The C++ Standard Library has evolved significantly since its inception:
- C++98: The first standardized version established the foundation, including containers and algorithms.
- C++11: Introduced many new features, such as smart pointers, lambda expressions, and new library components like `std::thread`, which revamped concurrency.
- C++14: Added improvements and bug fixes to the existing features.
- C++17: Brought further enhancements, including filesystem support and optional types.
- C++20: Marked a milestone with concepts, ranges, and coroutines, making the standard library even more robust.
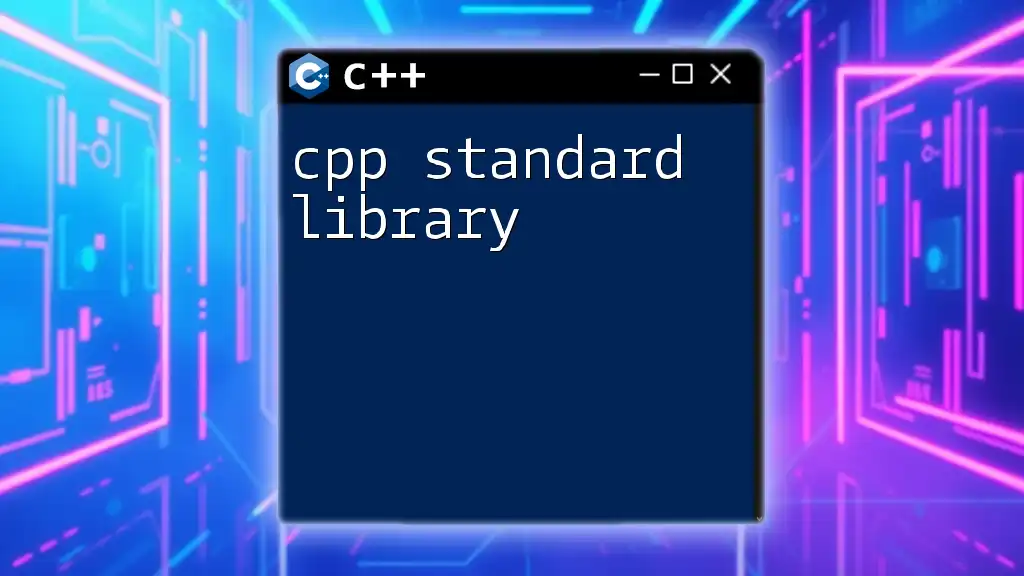
Getting Started with `#include`
What is the `#include` Directive?
The `#include` directive directs the preprocessor to include the contents of a specified file, typically a header file. This enables the use of predefined functions and classes in your program, which drastically reduces the amount of code you have to write from scratch.
Syntax of the `#include` Directive
There are two common ways to include headers in C++:
-
Using angle brackets (`< >`): This tells the compiler to look for the header file in the system directories. For instance:
#include <iostream>
-
Using double quotes (`" "`): This directs the compiler to first look in the local directory where your source file resides. If it doesn’t find the file there, it checks the system directories:
#include "myheader.h"
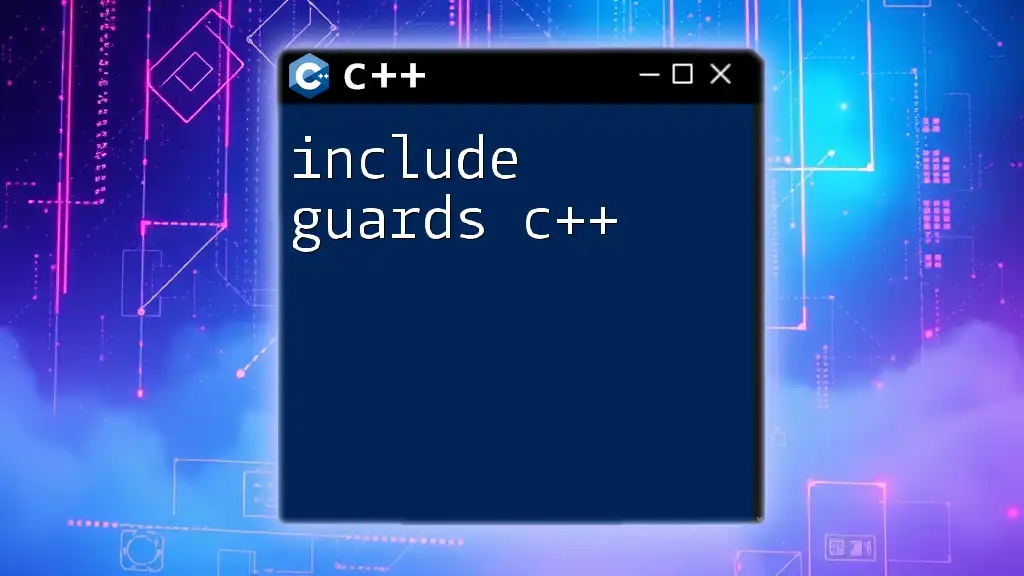
Commonly Used Standard Library Headers
Basic Input/Output
`#include <iostream>`
The `<iostream>` header facilitates input and output operations in C++. It contains the definitions for objects like `std::cin`, `std::cout`, and `std::endl`.
Example: Simple input/output using `cin` and `cout`
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this example, `std::cout` outputs the string "Hello, World!" to the console.
String Manipulation
`#include <string>`
The `<string>` header allows you to work with string data and provides a full-featured string class.
Example: Basic string operations
#include <iostream>
#include <string>
int main() {
std::string name = "Alice";
std::cout << "Name: " << name << std::endl;
return 0;
}
Here, the program initializes a string variable `name` and outputs its value.
Data Structures
`#include <vector>`
Vectors are part of the standard library and provide dynamic arrays that can change size. They are highly useful for managing collections of related data.
Example: Creating and manipulating a vector
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for(int num : numbers) {
std::cout << num << " ";
}
return 0;
}
In this snippet, a vector named `numbers` is created and then iterated over to print each element.
Algorithms
`#include <algorithm>`
The algorithms header provides a collection of functions to perform various operations on containers, such as sorting, searching, and manipulation.
Example: Using `std::sort` to sort a vector
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {5, 3, 4, 1, 2};
std::sort(numbers.begin(), numbers.end());
for(int num : numbers) {
std::cout << num << " ";
}
return 0;
}
This code sorts the vector `numbers` in ascending order and prints the sorted values.
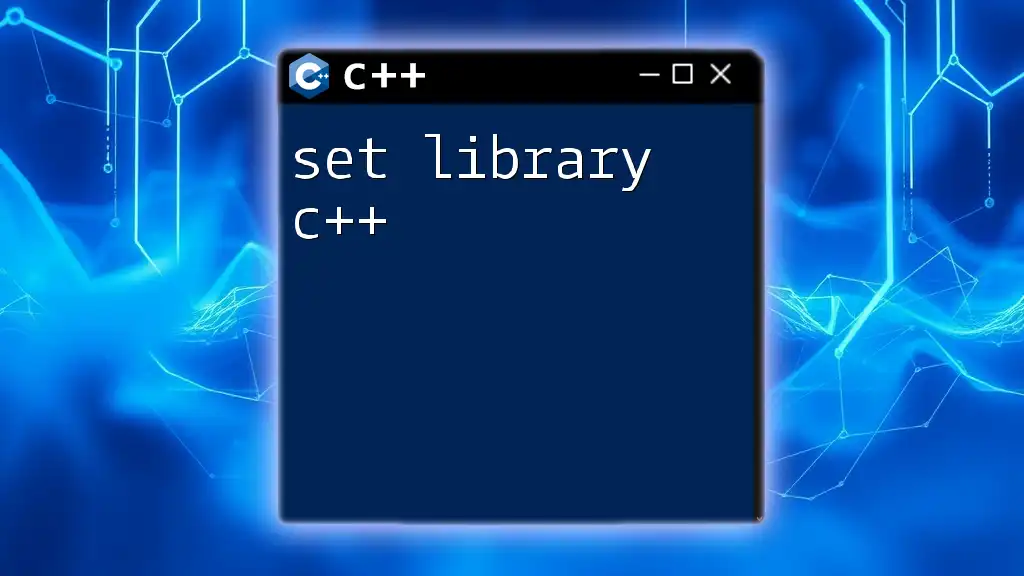
Advanced Library Features
Utilizing Multiple Headers
In C++, you can include multiple headers to combine various functionalities seamlessly.
Example: Combining functionalities
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {5, 3, 4, 1, 2};
std::sort(numbers.begin(), numbers.end());
for(auto num : numbers) {
std::cout << num << " ";
}
return 0;
}
This example covers the usage of input/output, a vector, and an algorithm for sorting.
Understanding Namespace
Namespaces help to organize code and prevent naming conflicts. The std namespace includes all the functionalities provided by the standard library.
Examples of using `namespace std`:
#include <iostream>
using namespace std;
int main() {
cout << "This is easier!" << endl;
return 0;
}
In this example, `using namespace std;` simplifies the code by allowing you to use standard library components without prefixing them with `std::`.
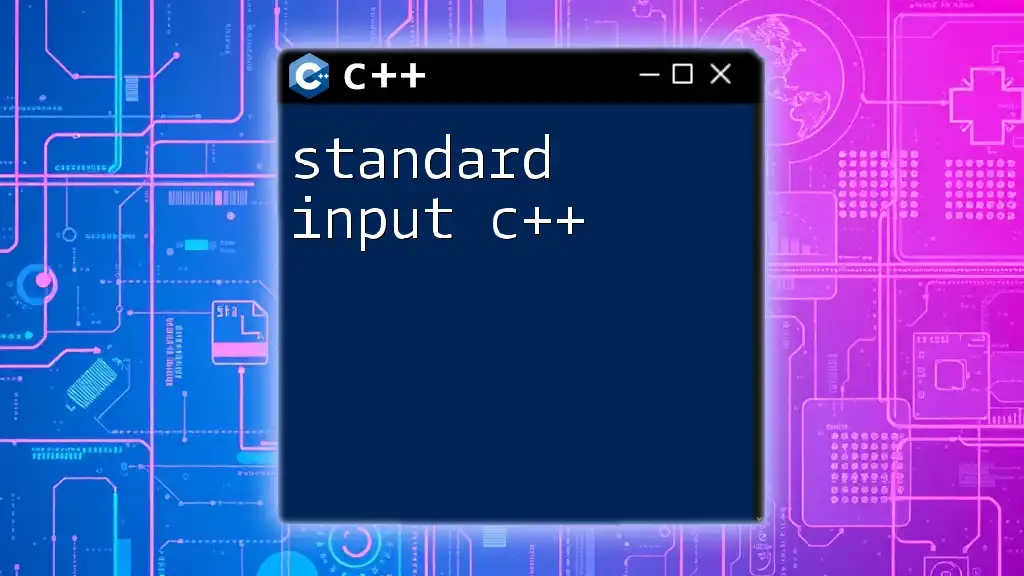
Best Practices for Using Standard Library
Efficient Header Inclusion
Including only the headers you need can improve compilation time and reduce dependencies.
- Always include headers that provide the necessary functionalities.
- Use forward declarations where possible to avoid unnecessary inclusions and minimize dependencies.
Understanding Compilation Units
Headers affect compile-time by defining how many files your compiler needs to process. Organizing your project structure and reducing cyclic dependencies can significantly improve your build times.
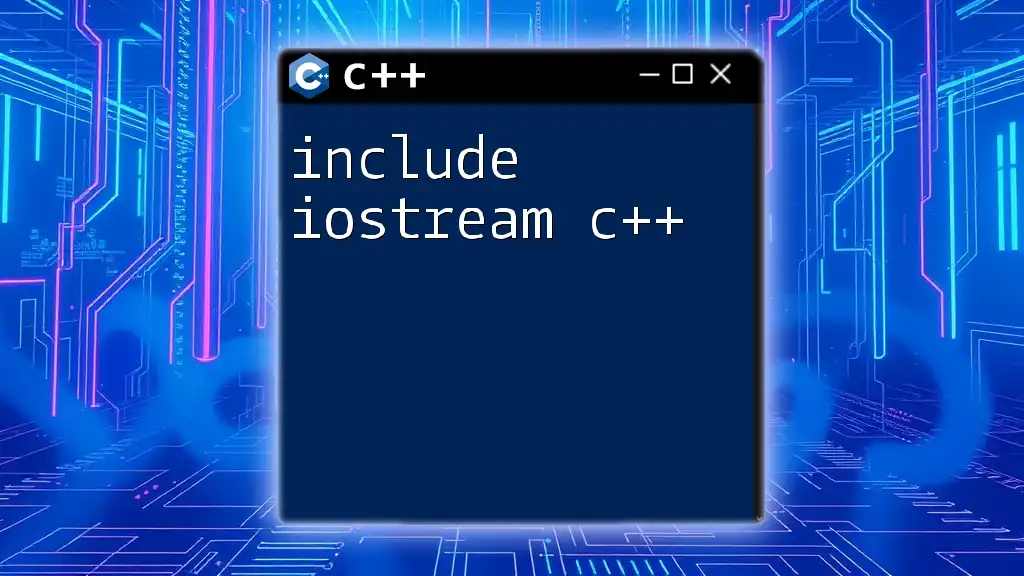
Conclusion
Incorporating the standard library into your C++ projects is not only a matter of convenience but also a means to enhance your program's efficiency and maintainability. By familiarizing yourself with various headers and functionalities, you can leverage pre-built components to accelerate your development process. Don’t hesitate to experiment with the different aspects of the standard library, and consider pursuing further resources to deepen your understanding.