The C++ Standard Library's `set` is a type of associative container that stores unique elements in a specific order, providing efficient insertion, deletion, and lookup operations.
Here's a simple code snippet demonstrating the use of a `set` in C++:
#include <iostream>
#include <set>
int main() {
std::set<int> mySet = {5, 2, 8, 1, 3};
for (int num : mySet) {
std::cout << num << " ";
}
return 0;
}
What is a Set?
In programming, a set is a collection of unique elements that inherently prohibits duplicates. This makes sets particularly useful in scenarios where the uniqueness of items is crucial. Sets form an unordered collection, meaning they do not maintain the order of elements as they are added.
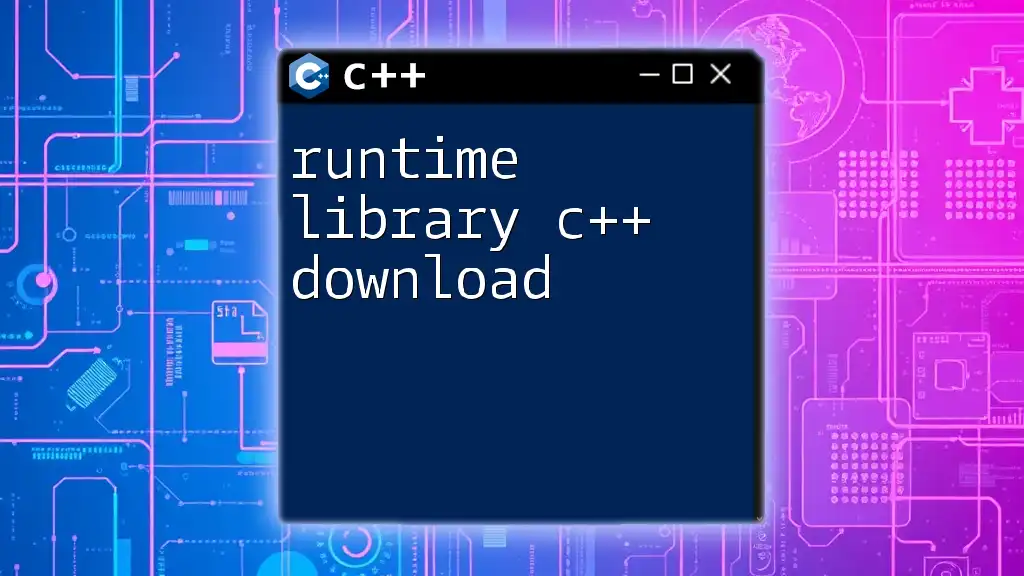
Why Use Sets in C++?
Using the set library in C++ offers significant advantages over other data structures. The most notable benefits include:
- Automatic handling of unique values: When you add an element to a set, if that element already exists, it will not be added again, thus preventing duplicates.
- Fast access and modification: The underlying structure of a set allows for average-time complexity of O(log n) for both search and insertion operations. This efficiency is critical when dealing with large datasets.
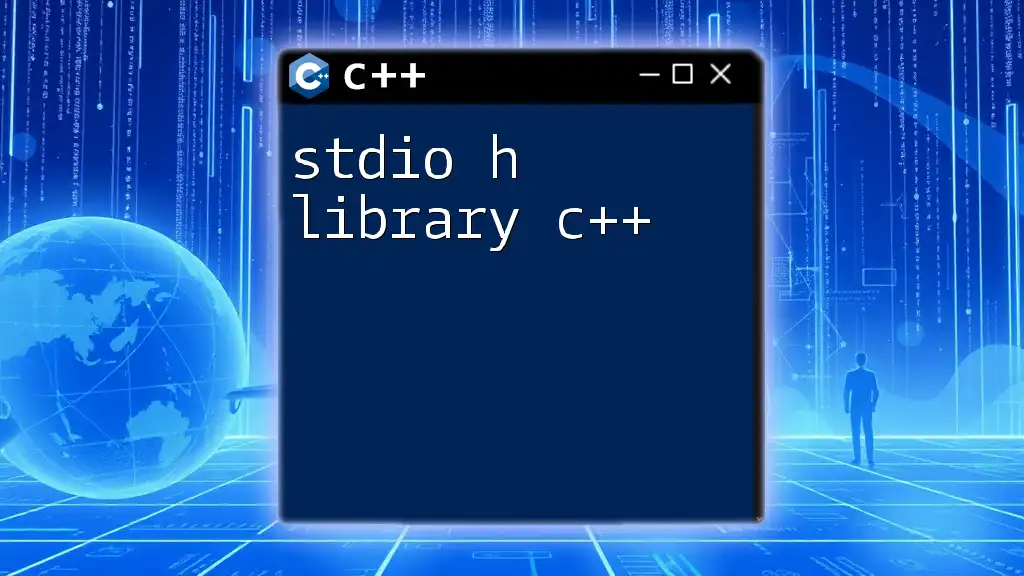
Overview of the C++ Set Library
Header File and Namespace
To utilize the set library in C++, you need to include the appropriate header:
#include <set>
Additionally, since the set functionalities are part of the `std` namespace, you either need to prefix your set objects with `std::` or use the directive `using namespace std;`.
Types of Sets in C++
std::set
`std::set` is the typical implementation of a set in C++. It maintains its elements in a sorted order and guarantees that all elements are unique. The properties of `std::set` make it the go-to choice when you need to enforce uniqueness but still require sorting.
std::multiset
On the other hand, `std::multiset` allows for duplicate elements while also maintaining a sorted order. If your application needs to count occurrences of items or manage duplicate values, `std::multiset` is the appropriate choice.
Comparing Sets with Other Containers
It’s essential to understand how sets compare with other containers in C++. Vectors and lists are ordered, meaning they respect the order in which elements are inserted, and they can contain duplicates. Maps, which work with key-value pairs, have different performance characteristics and are not suitable for situations requiring only unique elements. Using sets is beneficial when your primary concern is uniqueness and efficient membership testing.
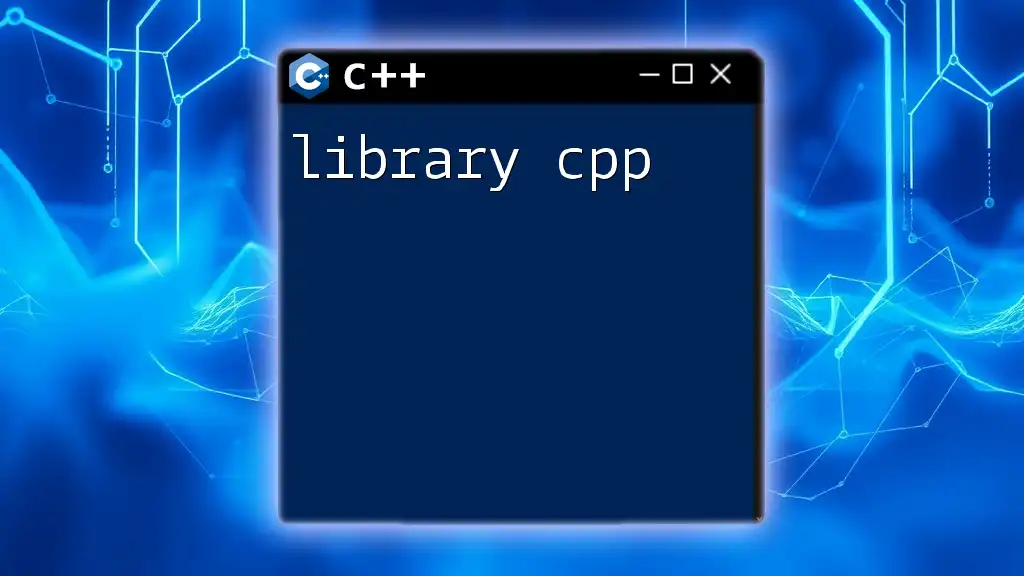
Basic Operations with Sets
Creating a Set
Creating a set in C++ is straightforward. Here’s how you can declare and initialize a set:
std::set<int> mySet; // Declare an empty set
std::set<int> mySet = {1, 2, 3, 4, 5}; // Initialize with values
Inserting Elements
The `insert()` method is how you add elements to your set. Unique elements will be added without duplication:
mySet.insert(6); // Successfully added
mySet.insert(1); // Will be ignored (duplicate)
Accessing Elements
To access elements in a set, you typically iterate through the set using a range-based for loop. This approach allows you to see each element in the ordered collection:
for (const auto& element : mySet) {
std::cout << element << std::endl;
}
Removing Elements
To remove elements from a set, the `erase()` method is used. If the specified element exists, it will be removed; otherwise, no changes will occur:
mySet.erase(4); // 4 will be removed from the set
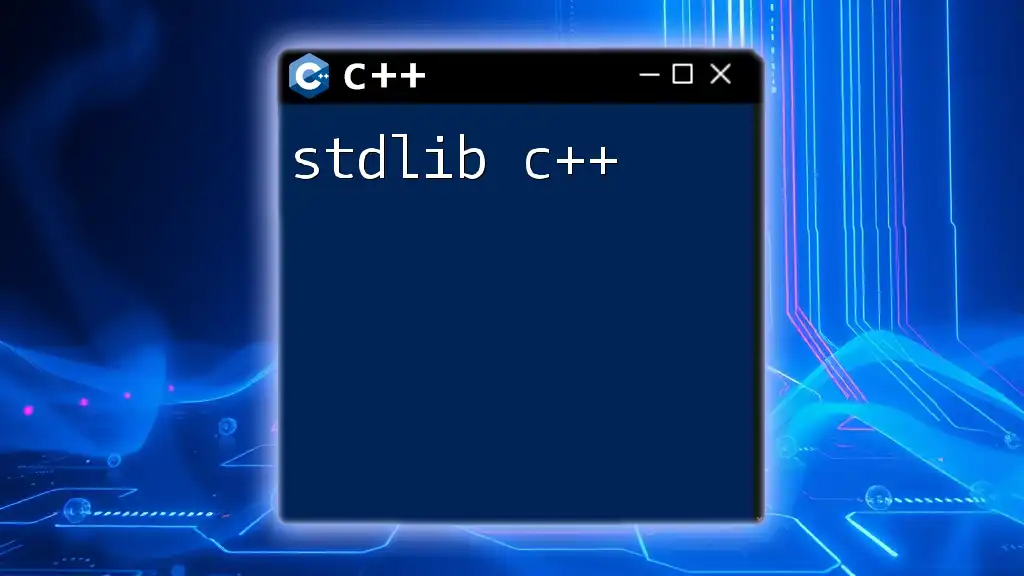
Advanced Operations with Sets
Finding Elements
You can use the `find()` function to check for the existence of an element. If found, it returns an iterator pointing to the element; if not, it returns the end iterator:
auto it = mySet.find(3);
if (it != mySet.end()) {
std::cout << "Found: " << *it << std::endl; // Output: Found: 3
}
Set Size and Clear Operations
Getting the size of a set is simple with the `size()` method:
std::cout << "Size: " << mySet.size() << std::endl; // Outputs the number of unique elements
To clear all elements from a set, use the `clear()` method:
mySet.clear(); // Removes all elements from mySet
Set Modifications
Merging Sets with `insert()`
You can merge two sets using the `insert()` method. For example, if you have two sets, `setA` and `setB`, and you want to combine them:
std::set<int> setA = {1, 2, 3};
std::set<int> setB = {3, 4, 5};
setA.insert(setB.begin(), setB.end()); // Merges setB into setA
Set Intersections and Differences
For tasks like computing intersections or differences between two sets, you can utilize the algorithms `<algorithm>`. Here’s how to get the intersection of two sets:
std::set<int> setA = {1, 2, 3, 4};
std::set<int> setB = {3, 4, 5, 6};
std::set<int> intersection;
// Find intersection
std::set_intersection(setA.begin(), setA.end(),
setB.begin(), setB.end(),
std::inserter(intersection, intersection.begin()));
You can likewise compute differences using `std::set_difference()`.
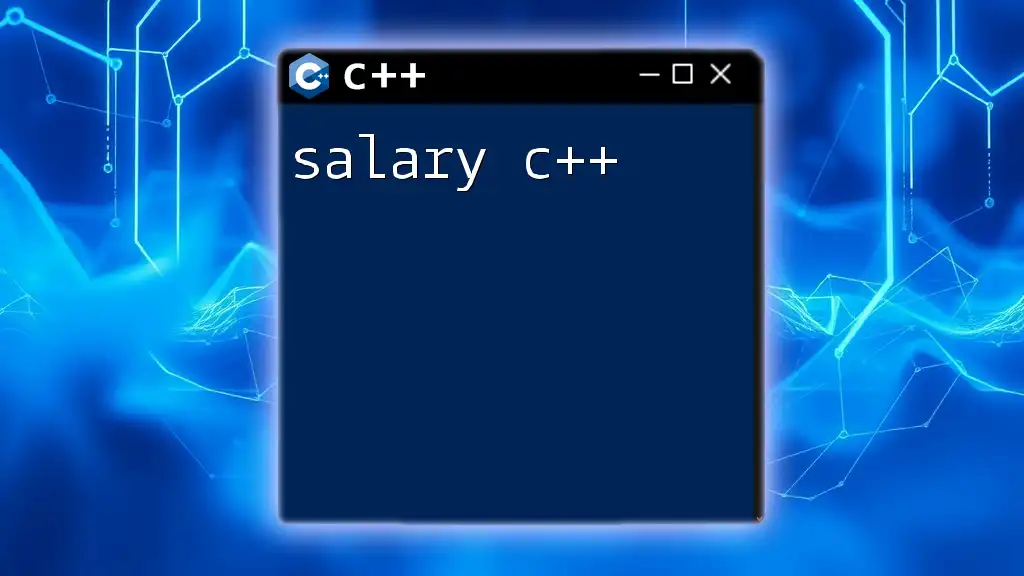
Practical Use Cases for C++ Sets
Use Case 1: Removing Duplicates from a Collection
Sets are fantastic for removing duplicates from a collection. For example, if you start with a vector of integers and want to eliminate duplicates:
std::vector<int> vec = {1, 2, 2, 3, 4, 4, 5};
std::set<int> uniqueSet(vec.begin(), vec.end()); // Automatically removes duplicates
Use Case 2: Storing Unique Users
In applications such as user accounts or registration systems, sets ensure that each user is unique. By storing usernames in a set, you can efficiently prevent duplicates at the time of insertion.
Use Case 3: Membership Testing
A distinctive advantage of sets is their rapid membership testing. When you need to check whether an item is present in a collection, sets provide O(log n) complexity, which is faster than the O(n) complexity of lists or vectors.
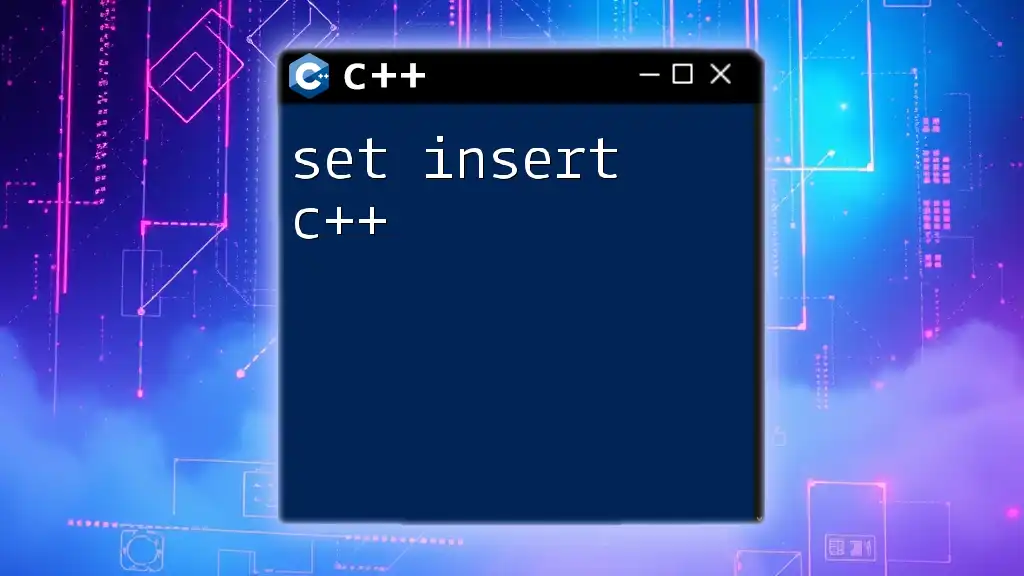
Performance and Complexity
Time Complexity of Set Operations
Operations on sets generally involve logarithmic complexity. Key operations like insertion, deletion, and searching each have average time complexities of O(log n), making sets incredibly efficient for dynamic collections of unique elements.
When to Avoid Using Sets
Despite their advantages, there are instances when you might want to avoid using sets. If ordering of elements is critical or if you often need to access elements by index, a vector or a list might be better suited for your needs. Additionally, if you expect to have a lot of duplicate elements, using a multiset might be more appropriate than a set.
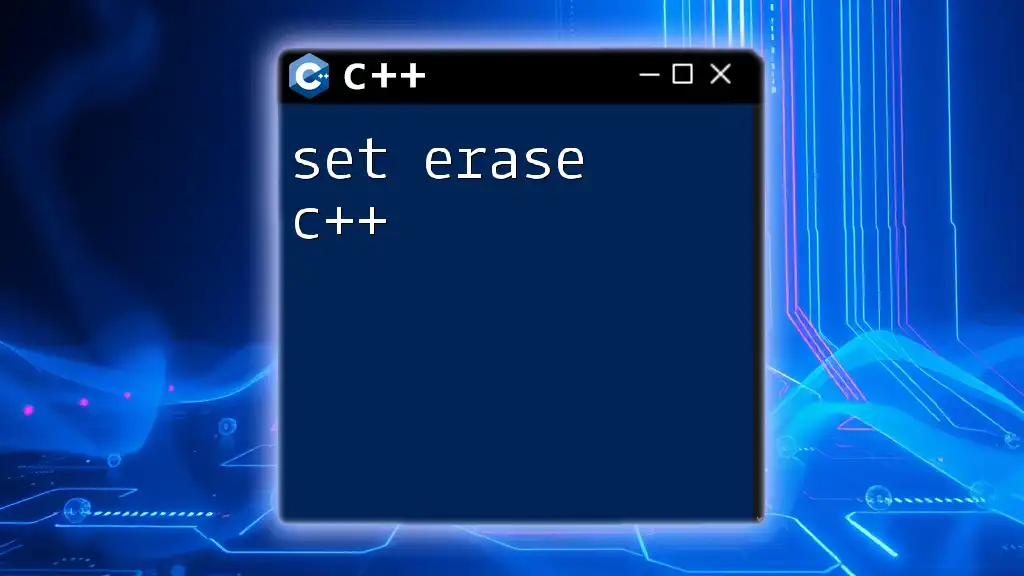
Conclusion
In conclusion, the set library in C++ offers versatile and efficient methods for managing collections of unique elements. With its ability to automatically enforce uniqueness, provide fast access, and support a variety of operations such as intersection and union, the set library is an indispensable tool in the C++ programmer’s toolkit. Experiment with these functionalities, and pair them with other C++ libraries to elevate your programming projects. To further enhance your programming journey, consult the official C++ documentation and recommended learning resources.