Assembly language can be integrated with C++ to allow low-level hardware manipulation and optimization, thereby enabling programmers to write performance-critical code.
Here's a simple example of how to include inline assembly within a C++ program:
#include <iostream>
int main() {
int result;
asm("movl $5, %0" : "=r"(result)); // Move the value 5 into the variable result
std::cout << "The result is: " << result << std::endl;
return 0;
}
Understanding Assembly Language
What is Assembly Language?
Assembly language is a low-level programming language that provides a symbolic representation of a computer's machine code. It serves as a bridge between the human-readable code and the binary instructions executed by the hardware. Unlike higher-level languages that abstract away the hardware specifics, assembly language is closely aligned with the architecture of a computer's CPU, providing developers with precise control over hardware and system resources.
Key Concepts in Assembly Language
Registers and Memory
A fundamental aspect of assembly language is the use of CPU registers—small storage locations within the CPU that provide fast access for the processor. Each register can hold a small amount of data, typically 32 or 64 bits, depending on the architecture.
Memory addressing is another critical concept, referring to how data and instructions are accessed and stored in memory. Understanding how to read from and write to different memory locations is vital for developing efficient assembly code.
Instruction Sets
An instruction set architecture (ISA) defines the set of instructions that a CPU can execute. Different processors have their own unique instruction sets, which dictate how programs interact with the hardware. Common examples include x86 for Intel and AMD processors, and ARM for mobile devices.
Familiarity with an ISA is crucial when integrating assembly language into C++, as it influences how assembly commands are structured and executed.
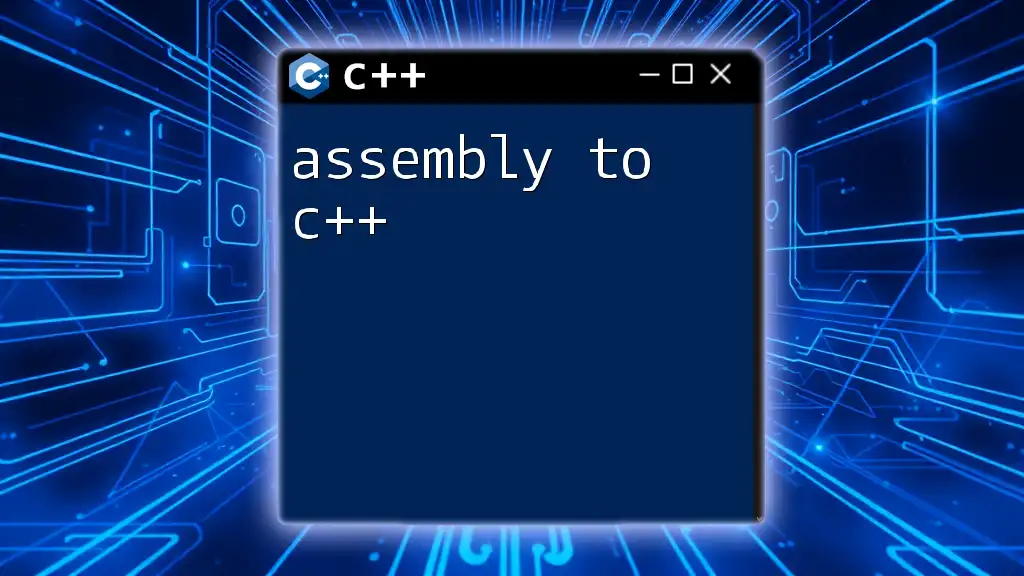
C++ and Assembly Integration
Why Use Assembly in C++?
Using assembly language within C++ programs opens up various performance benefits. Certain computations may not be efficient in high-level languages due to overhead and abstracted processes. By writing critical sections of code in assembly, developers can achieve significant speedups and optimize performance.
Moreover, assembly allows direct hardware manipulation. It enables programmers to utilize specific instructions to interact with hardware components, making it particularly useful in embedded systems and performance-sensitive applications.
When to Use Assembly Language
Identifying the right scenarios to employ assembly language is key. Performance-critical functions, such as algorithm-heavy operations or real-time processing tasks, may greatly benefit from assembly optimizations. Furthermore, in environments where hardware resources are limited—like in embedded or real-time systems—assembly language can be invaluable.
However, developers should also be aware of common pitfalls. Assembly code tends to be less readable and maintainable compared to higher-level code. Therefore, it should be sparingly used to avoid cluttering the codebase and complicating future updates.
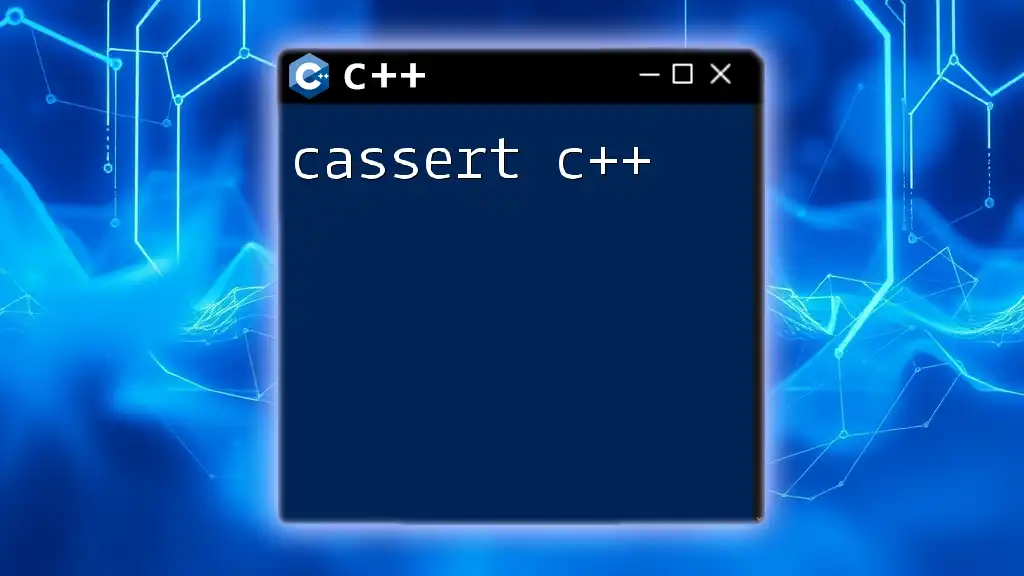
Writing Assembly Code in C++
Inline Assembly
What is Inline Assembly?
Inline assembly refers to embedding assembly language commands directly within C++ code. This technique allows programmers to seamlessly integrate assembly instructions into their C++ routines without needing a separate assembly file, making it easier to maintain.
Syntax and Examples
The syntax for inline assembly varies depending on the compiler, but a common approach in GCC is shown below:
__asm__ __volatile__ (
"movl %eax, %ebx;" // Move contents of eax to ebx
);
In this example, the `movl` instruction moves data from one register to another, directly affecting the execution of the program.
External Assembly Files
How to Link Assembly Code with C++
For more extensive assembly routines, developers often write their code in external files. This approach aids in keeping complex assembly instructions organized and separate from C++ code.
Example Code for Linking
To call an external assembly function from C++, you would declare the function as follows:
extern "C" void my_asm_function();
my_asm_function(); // Call the assembly function
This ensures the C++ compiler knows how to link the assembly code appropriately, preserving the name mangling convention when working with C++.
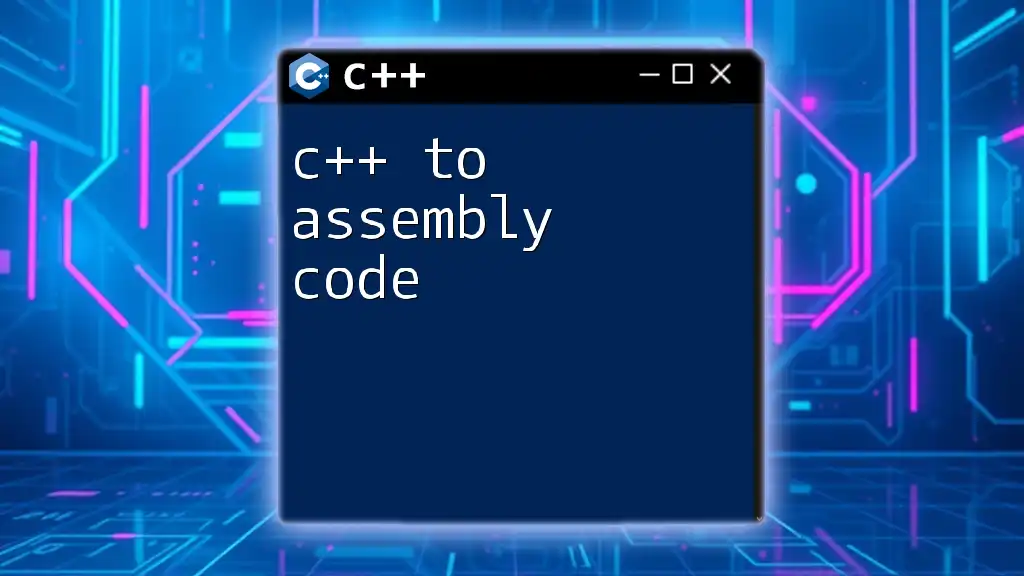
Common Assembly Instructions Used in C++
Basic Instructions
A few fundamental assembly instructions are commonly utilized within C++ integration. Understanding these is crucial for effective assembly programming.
MOV, ADD, and SUB
- MOV: Transfers data from one location to another.
- ADD: Performs addition.
- SUB: Performs subtraction.
Code Examples:
mov eax, 1 ; Move 1 into register eax
add eax, 2 ; Add 2 to the value in eax
sub eax, 1 ; Subtract 1 from the value in eax
These basic operations form the building blocks of more complex logic that can be executed efficiently in assembly.
Control Flow Instructions
Control flow instructions allow developers to direct the flow of execution in their assembly programs.
Jumps and Loops
Jump instructions are pivotal in implementing loops and conditional execution. For instance, the `JMP` instruction enables unconditional branching to a specified label, while conditional jumps, like `JE` (Jump if Equal), control the execution based on specific conditions.
Code Example:
jmp label ; Unconditional jump to label
label: ; Label definition
System Calls and Interrupts
Using assembly language can also enable direct interaction with the operating system through system calls. Understanding how to invoke system calls in assembly allows developers to harness advanced features and capabilities of the OS, essential for systems programming.
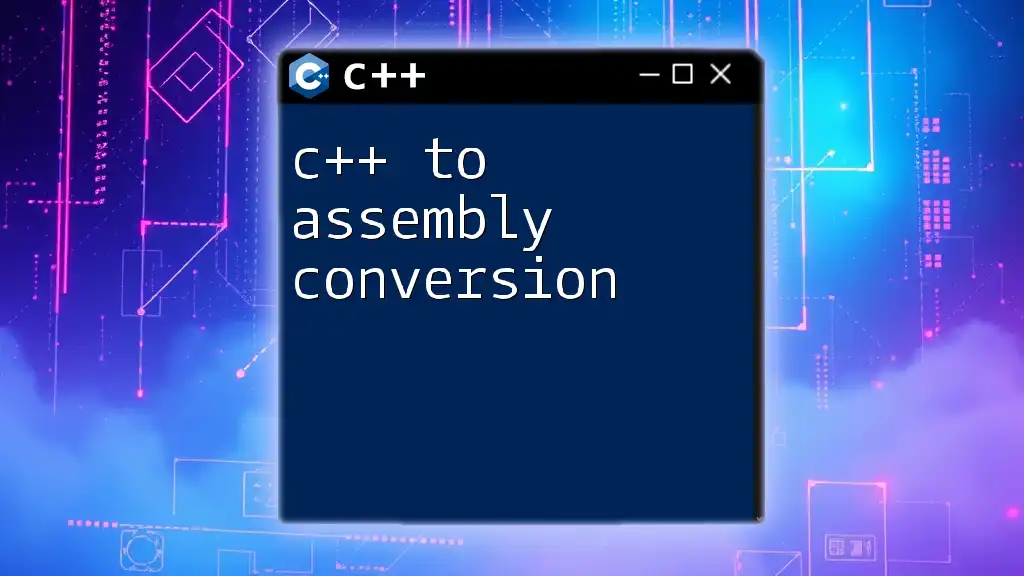
Debugging and Optimization
Debugging Assembly Code
Debugging assembly code can be a challenging aspect of development. However, tools such as gdb (GNU Debugger) are invaluable for tracing the execution of assembly programs, inspecting registers, and identifying bugs. Moreover, inserting debug messages and carefully analyzing CPU states can aid in debugging complex assembly routines.
Performance Optimization
Optimization is critical when writing assembly code. To achieve high performance, developers should employ best practices such as:
- Writing clean code to improve readability and manageability.
- Utilizing loop unrolling to reduce the overhead of loop control.
- Understanding register allocation to minimize memory access time by keeping frequently used variables in registers.
These strategies can significantly enhance the efficiency of the assembly code within a C++ program.
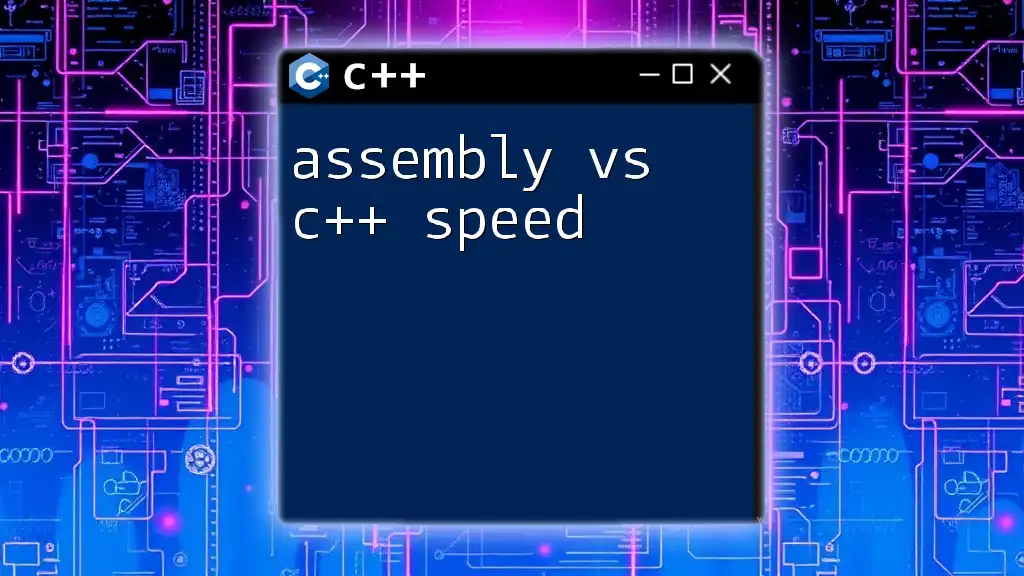
Conclusion
In summary, understanding assembly language and its integration with C++ is crucial for developers looking to optimize their applications for performance and direct hardware control. Although assembly programming can present challenges around readability and maintainability, the performance benefits it offers in specific scenarios can be substantial. By experimenting with both C++ and assembly, developers can greatly enhance their programming skills and produce more efficient software.
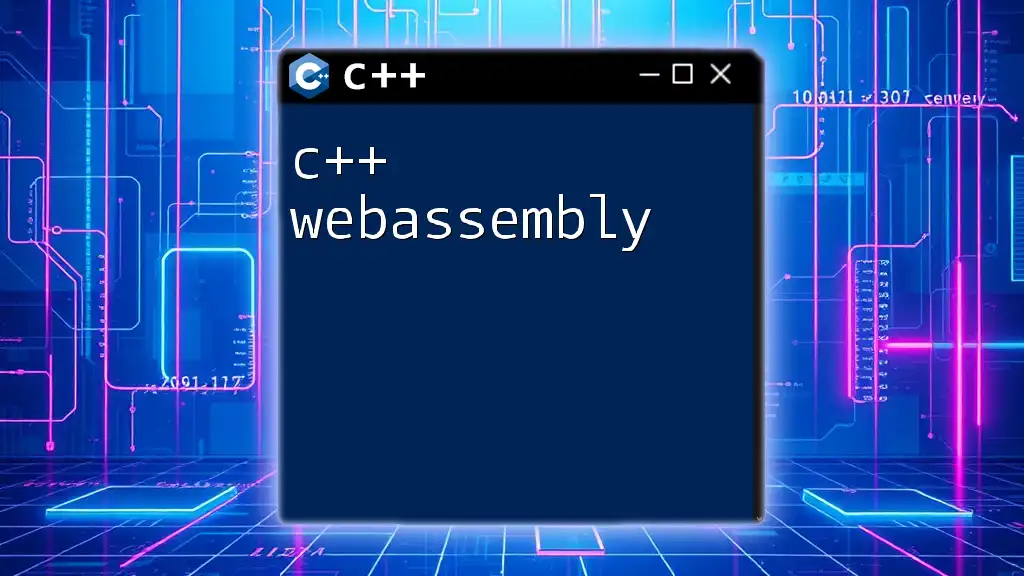
Additional Resources
For those interested in further exploring the intricacies of assembly language and its integration with C++, various books and online tutorials are available that provide detailed insights and hands-on examples. These resources can deepen your understanding and aid in mastering assembly programming.
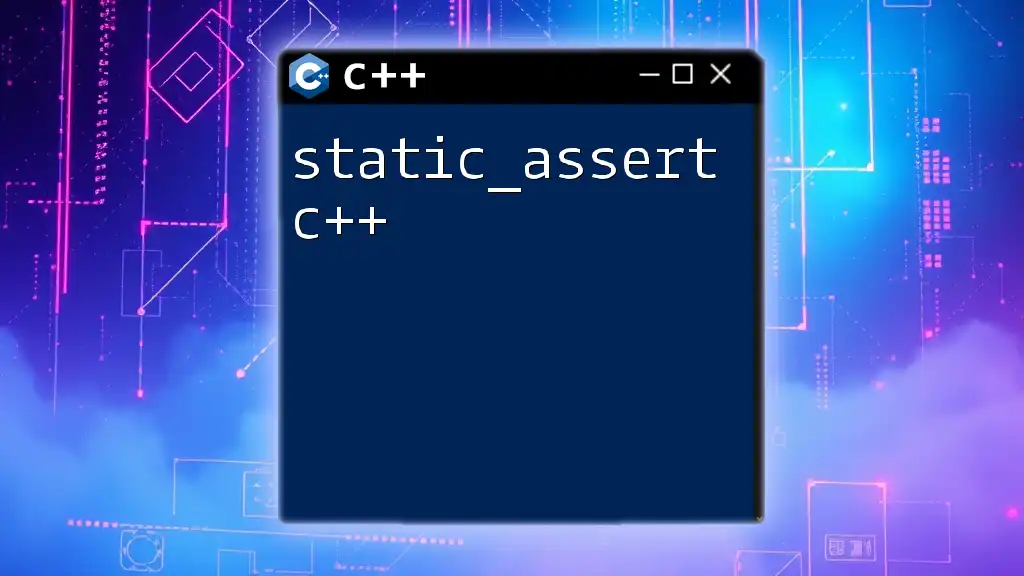
FAQs
-
What are the advantages of using assembly language?
Assembly language allows for highly optimized code and direct hardware manipulation, which can enhance performance in critical applications. -
Can all C++ compilers handle inline assembly?
Not all compilers support inline assembly, and the syntax can vary between compilers, so it's essential to refer to the specific documentation. -
How can I learn more about assembly programming?
You can find extensive resources, including books, online courses, and tutorials, that focus on assembly language concepts and practices.