C++ can be compiled into assembly language, allowing developers to understand the low-level operations performed by their C++ code, as illustrated in the following example.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
The assembly output for this C++ program (generated using a compiler with the `-S` flag) could look something like this:
.LC0:
.string "Hello, World!"
.globl main
.type main, @function
main:
pushq %rbp
movq %rsp, %rbp
leaq .LC0(%rip), %rdi
call _ZSt4cout
call _ZSt4endlIcSt11char_traitsIcEERS1_
movl $0, %eax
popq %rbp
ret
This assembly code represents the low-level instructions generated from the high-level C++ code.
Understanding C++ and Assembly Language
What is C++?
C++ is a versatile and powerful high-level programming language that is widely used in software development. It incorporates features of both procedural and object-oriented programming, which allows developers to create complex applications with a focus on code reuse and efficiency. Key features of C++ include:
- Object-oriented programming: Enables code encapsulation and inheritance.
- Generic programming: Facilitates the creation of functions and classes that work with any data type.
- Standard Template Library (STL): A powerful collection of algorithms and data structures.
The significance of C++ lies in its balance of high-level features and low-level access to memory, making it suitable for systems programming, game development, and performance-critical applications.
What is Assembly Language?
Assembly Language is a low-level programming language that provides a symbolic representation of a computer's architecture. It is considered more machine-oriented than C++, as it closely reflects the underlying hardware operations. Assembly is essential for tasks that require direct hardware manipulation, making it a critical skill for performance optimization and systems programming.
The Relationship between C++ and Assembly
When writing C++, developers create code that is easier to read and maintain. However, before it can be executed by a computer, this high-level code must be translated into machine code, which is what the processor understands. This translation involves several steps, including compilation, which typically generates an Assembly Code as an intermediate step.
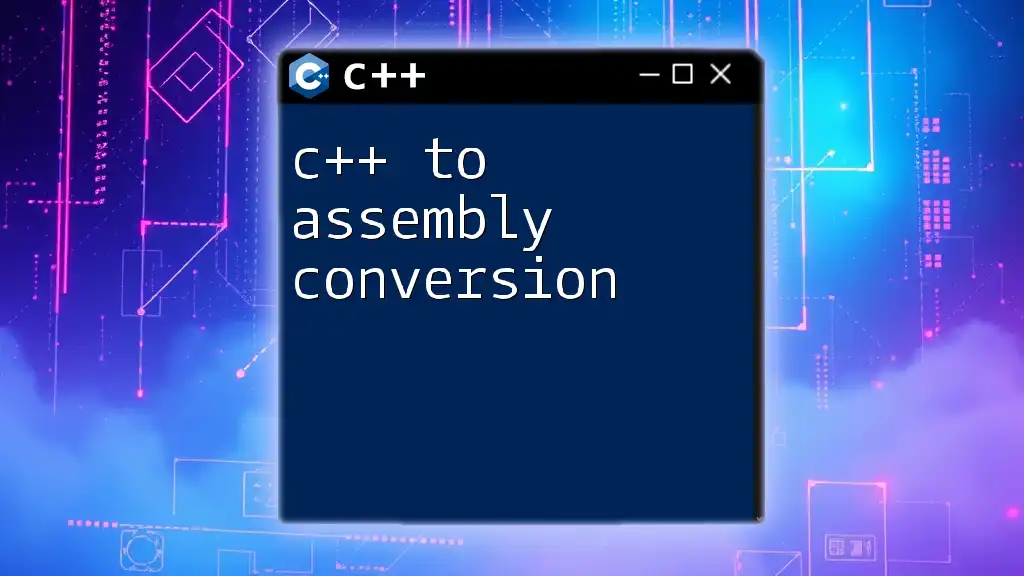
The Compilation Process
Stages of Compilation
The compilation process for C++ to Assembly code consists of several key stages:
- Preprocessing: The preprocessor handles directives such as `#include` and `#define`, preparing the code for compilation.
- Compilation: This stage converts preprocessed code into Assembly language.
- Assembly: The Assembly code is then translated into machine code.
- Linking: Finally, the machine code is linked to libraries and converted into an executable.
Understanding these stages is crucial for optimization, as each step provides opportunities to improve performance and reduce overhead.
Tools for Compilation
There are several C++ compilers available, with GCC (GNU Compiler Collection) and Clang being among the most popular. Compilers often come with various options that allow developers to specify how Assembly code should be generated. Utilizing these tools effectively is critical for learning how to work with C++ to Assembly code.
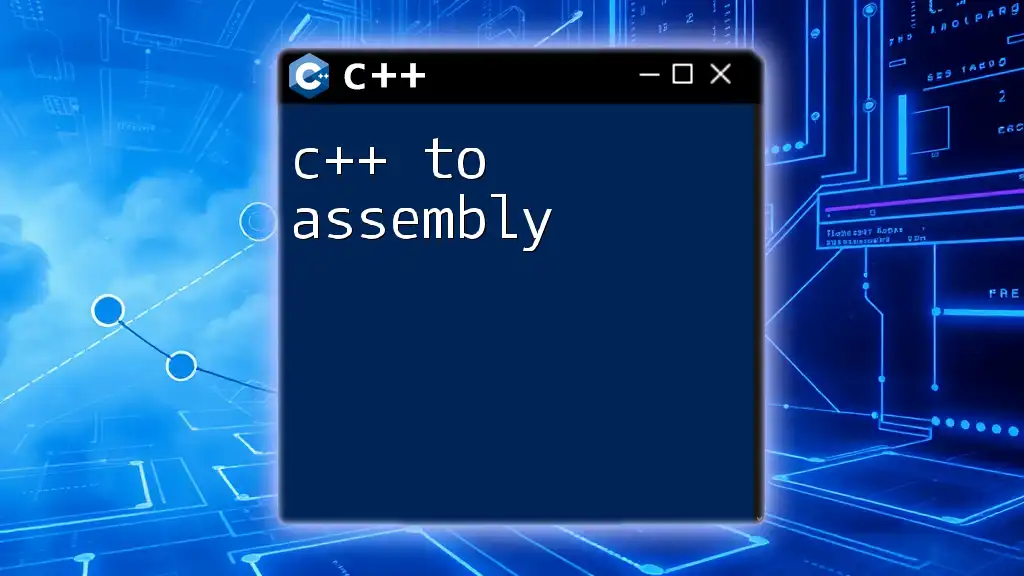
Generating Assembly Code from C++
Writing Simple C++ Code
To illustrate the process of generating Assembly code, let’s look at a simple C++ program that prints "Hello, World!" to the console.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Compiling to Assembly Language
To generate Assembly code from this C++ program, you can use the following command with GCC:
g++ -S hello.cpp -o hello.s
The `-S` flag tells the compiler to compile the code into Assembly language instead of producing an object file.
Understanding the Generated Assembly Code
The generated Assembly code (in the file `hello.s`) will contain several instructions that closely correspond to the operations performed by the C++ code. For example, it will include calls to library functions and assembly instructions for outputting the string to the console.
Understanding this output can deepen your insights into how high-level constructs translate into lower-level operations.
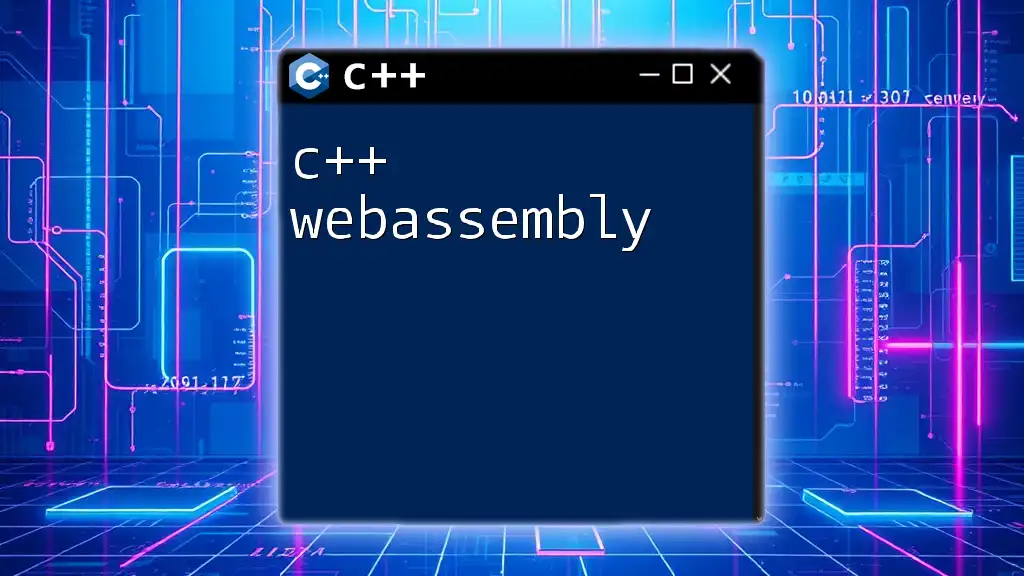
Analyzing Assembly Code
Key Components of Assembly Language
Assembly language comprises several key components:
- Registers: Small storage locations within the CPU that hold data temporarily.
- Instructions: Command-like operations that the CPU can perform (e.g., ADD, SUB).
- Operands: The values or addresses involved in the instructions.
Familiarizing yourself with these elements will enhance your ability to work with C++ to Assembly code effectively.
Example Analysis
Consider a basic addition operation in C++:
int add(int a, int b) {
return a + b;
}
The corresponding Assembly code will demonstrate how the addition operation is implemented through CPU instructions. You'll find that the assembly equivalent likely utilizes registers to perform the arithmetic operation and store the result.
Assembly Code Equivalent
For the `add` function, the generated Assembly might look something like this (simplified for understanding):
mov eax, a ; Move the value of a into register eax
add eax, b ; Add the value of b to eax
ret ; Return the result in eax
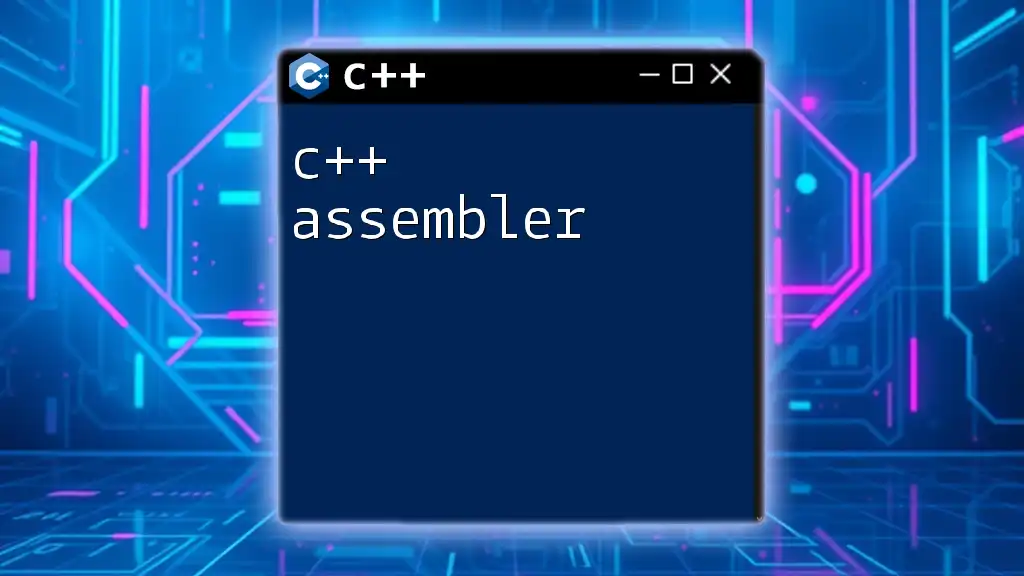
Optimizing C++ for Better Assembly Output
Compiler Optimization Flags
When compiling C++ code, various optimization flags can be applied to enhance performance. Common options include:
- -O1: Basic optimization that enables a set of performance improvements.
- -O2: Focuses on more extensive optimizations.
- -O3: Aggressive optimizations that may increase compilation time but produce the most efficient code.
By leveraging these flags, you can significantly influence the quality of the generated Assembly code.
Case Study: Performance Analysis
To illustrate the impact of optimization, compile the same C++ code with and without optimization flags. Compare execution time and Assembly output to observe how optimizations affect performance and resource usage. Profiling tools can be used to analyze memory and CPU usage statistics.
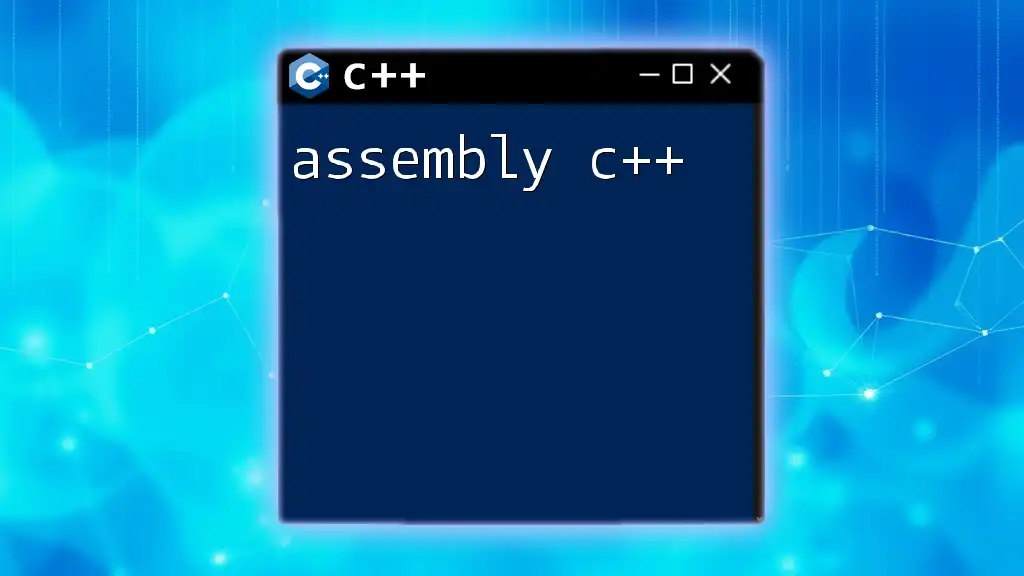
Best Practices for Writing Efficient C++ Code
Code Structure
Organizing your C++ code effectively can facilitate better translation to Assembly code. Focus on modularity by breaking down your code into functions and classes. This approach not only enhances readability but also allows compilers to apply optimizations more effectively.
When to Use Assembly Language
Although Assembly language offers precise control over hardware, it is generally used only in performance-critical scenarios. Examples include graphics engines, real-time systems, and embedded programming. However, it's essential to balance performance with readability, as overly complex Assembly code can become difficult to maintain.
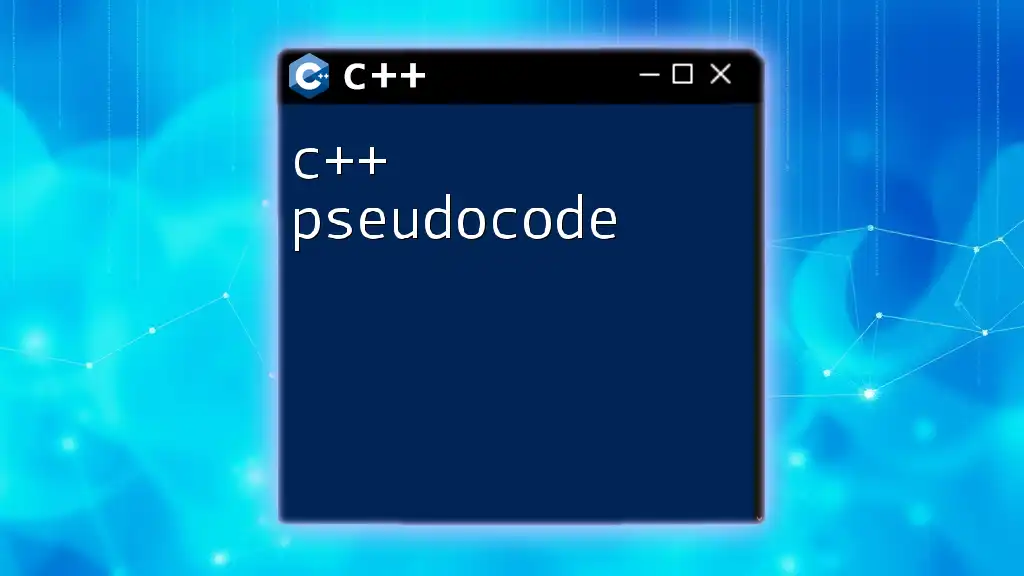
Conclusion
Understanding the transition from C++ to Assembly code is vital for any developer aiming to optimize their applications. By grasping the compilation process, analyzing generated Assembly code, and applying best practices, you position yourself to write performant software.
As you delve deeper into the world of C++ and Assembly, remember that experimentation is key. Try different code structures, compile with various optimization flags, and observe how they change the generated Assembly output. Engaging with a community or a teaching company focused on C++ can further your learning and enhance your skills in this critical area of programming.
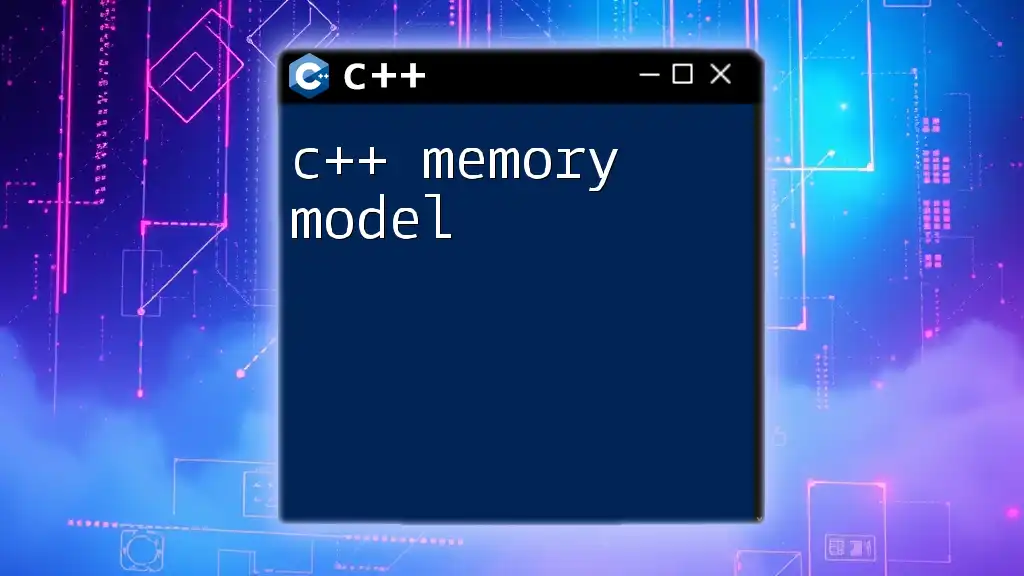
Additional Resources
References and Further Reading
To further explore C++ and Assembly language, consider diving into comprehensive books, online courses, or official documentation that can provide valuable insights and practical experience.
Tools and Compilers
To practice hands-on C++ to Assembly code generation, try using GCC, Clang, or Visual Studio, which all offer extensive documentation and community support for developers at all levels.