C++ in Visual Studio Code (VSCode) allows developers to write, debug, and run C++ programs efficiently with the help of extensions, making it a powerful lightweight option for C++ development.
Here's a simple code snippet demonstrating a basic "Hello, World!" program in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Setting Up Your Environment
Installing Visual Studio Code
VSCode is an essential tool for C++ development, and installing it is the first step toward a seamless programming experience.
To install VSCode, follow these steps:
- Download VSCode from the official site. Select the version compatible with your operating system (Windows, macOS, Linux).
- Run the installer. For Windows, this involves downloading and executing an `.exe` file. On macOS, drag the application to your Applications folder. Linux users can download using a package manager.
- Launch VSCode after installation to explore its Interface.
Installing C++ Compiler
A C++ compiler is crucial for translating your code into executable files. You have several options: GCC, Clang, and MSVC (Microsoft Visual C++ for Windows users).
Choosing a Compiler:
- GCC (GNU Compiler Collection): A versatile and widely-used option, particularly for Linux and macOS.
- Clang: Known for its speed and excellent error messages. Available on multiple platforms.
- MSVC: Integrated with Visual Studio, best for Windows users.
Installation Instructions
Installing GCC on Different Operating Systems:
- Windows: Install MinGW from the official site or the MSYS2 environment. Ensure to add the `bin` directory to your system PATH.
- Linux: Install using the terminal with package managers (`apt`, `yum`, etc.). For example:
sudo apt-get install build-essential
- macOS: Install via Homebrew:
brew install gcc
Using MSVC on Windows:
You can obtain MSVC by downloading the [Visual Studio Installer](https://visualstudio.microsoft.com/downloads/) and selecting the C++ development workload during installation.
Configuring VSCode for C++
Installing the C/C++ Extension
VSCode requires extensions to effectively assist with C++ development. Here's how to install the Microsoft C/C++ extension:
- Open VSCode and navigate to the Extensions view by clicking on the square icon in the sidebar or using the shortcut `Ctrl + Shift + X`.
- Type "C/C++" in the search box and select the Microsoft C/C++ extension.
- Click "Install."
Overview of Useful Extensions
Apart from the primary C/C++ extension, consider adding:
- CMake Tools for better project management.
- Code Runner to quickly execute code files.
- GitLens to improve version control workflows.
Configuring Build Tasks
To compile and run your C++ programs directly in VSCode, you’ll need to set up build tasks using the `tasks.json` configuration file.
Here’s an example of a simple configuration:
{
"version": "2.0.0",
"tasks": [
{
"label": "build",
"type": "process",
"command": "g++",
"args": [
"-g",
"${file}",
"-o",
"${fileDirname}/${fileBasenameNoExtension}.exe"
],
"group": {
"kind": "build",
"isDefault": true
},
"problemMatcher": ["$gcc"]
}
]
}
This configuration compiles the current file and outputs an executable in the same directory.
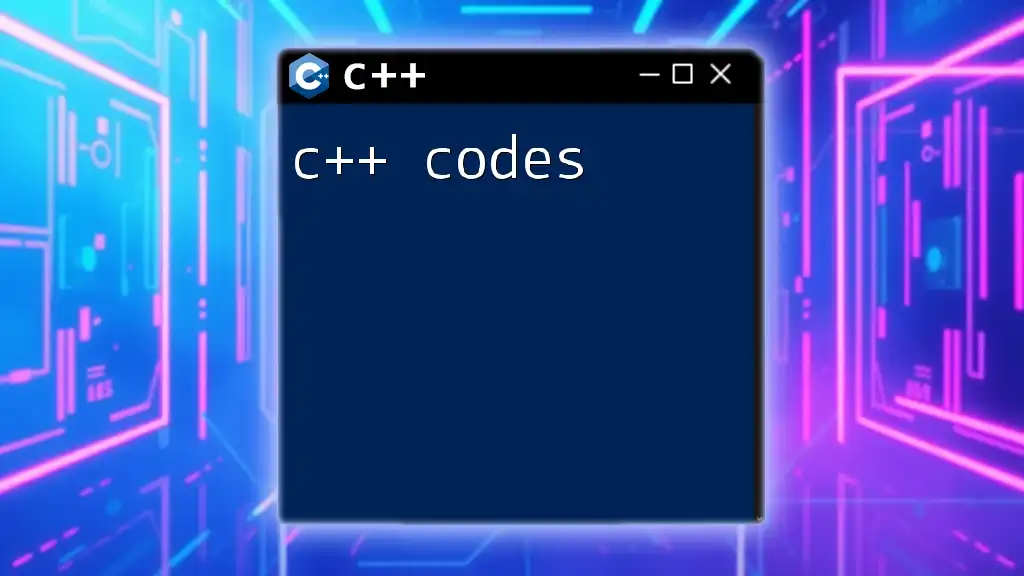
Writing and Structuring Your C++ Code
Creating a New C++ Project
Start by organizing your workspace. It’s vital to establish a logical structure within your project to keep your source code manageable.
Best Practices for Project Structure
- Keep source files (`.cpp`) and header files (`.h`) in separate directories.
- Use a dedicated folder for compiled binaries.
Writing Your First C++ Program
Let’s write a simple C++ program to get you started:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
This program includes the basic `iostream` library and prints "Hello, World!" to the console.
Code Organization with Files
C++ projects often contain numerous files that collaborate to define a complete application. It's essential to manage these files:
Using Multiple Source Files
By splitting your code into several files, you can promote readability and reusability. Here’s an example of a simple multi-file project:
// math_utils.h
#ifndef MATH_UTILS_H
#define MATH_UTILS_H
int add(int a, int b);
#endif
// math_utils.cpp
#include "math_utils.h"
int add(int a, int b) {
return a + b;
}
// main.cpp
#include <iostream>
#include "math_utils.h"
int main() {
std::cout << add(3, 4) << std::endl;
return 0;
}
In this example, `math_utils.h` contains function prototypes, while `math_utils.cpp` provides function implementations. The `main.cpp` file ties everything together.
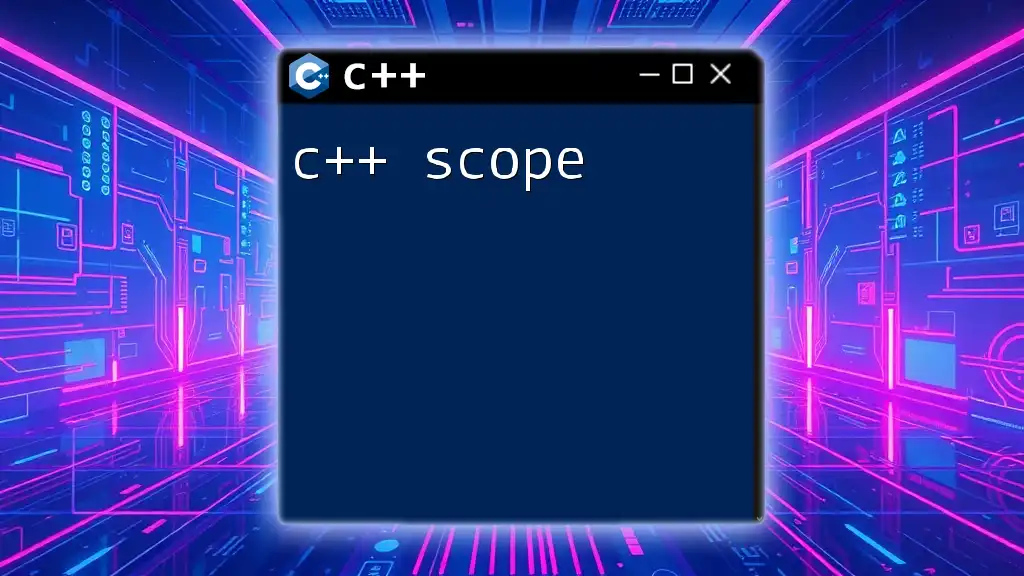
Key Features of VSCode for C++
Code Navigation and IntelliSense
One of the most powerful aspects of VSCode is its IntelliSense feature. This feature provides smart autocompletions based on variable types, function definitions, and imported modules.
Use this feature to speed up coding by reducing the need to remember every syntax detail. For example, typing `std::cout` can suggest the appropriate include directives.
Debugging C++ Code
Debugging is critical for identifying issues in your code. VSCode provides robust debugging tools:
Setting Breakpoints
To set a breakpoint, simply click in the gutter next to the line number where you want to pause execution. Breakpoints allow you to examine variable states and control flow at specific points.
Using the Debugger
Once you have breakpoints set, start debugging using the `Run > Start Debugging` option or the `F5` key. You can step through the code line by line, inspect variable values in real-time, and watch expressions to identify where things might be going wrong.
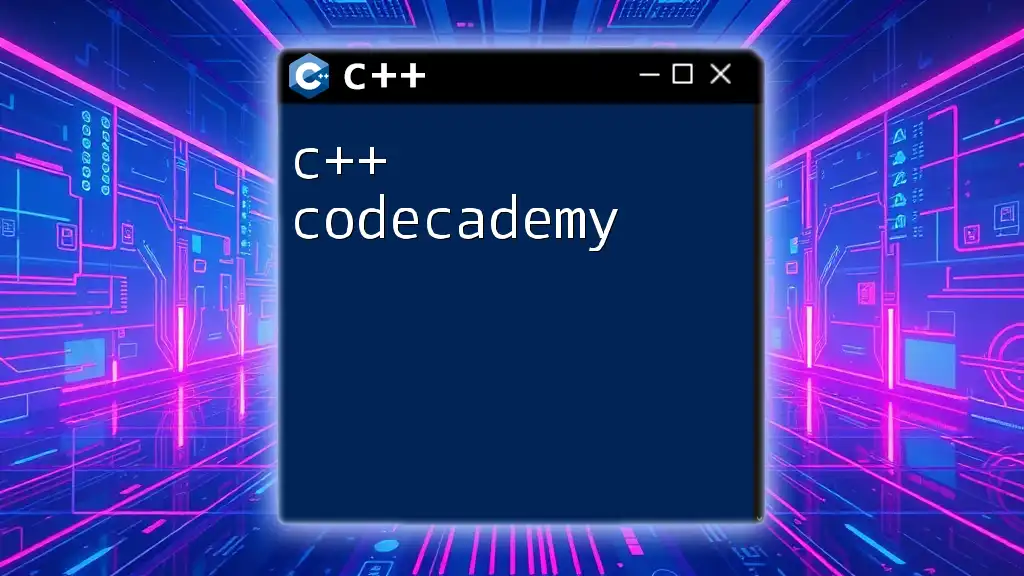
Enhancing Your C++ Workflow
Customizing Your VSCode Environment
Tailoring VSCode to fit your workflow can greatly improve your productivity.
Setting Up Custom Shortcuts
You can create custom keyboard shortcuts to streamline your development process. Access these settings from `File > Preferences > Keyboard Shortcuts`. For example, you could assign `Ctrl + S` to save your file and run specific commands.
Configuring Themes and Layouts
Personalizing your editor’s appearance can reduce eye strain and improve your coding experience. Choose from numerous themes and color schemes in the Extensions marketplace or customize your own in the settings.
Useful Extensions for C++ Development
Consider incorporating additional extensions to enhance your C++ development experience:
- CPplint: A linting tool to enforce coding standards.
- Clang-Format: Automatically formats your code to improve readability.
- Doxygen Documentation Generator: Helps create documentation for your C++ project directly from comments in your code.
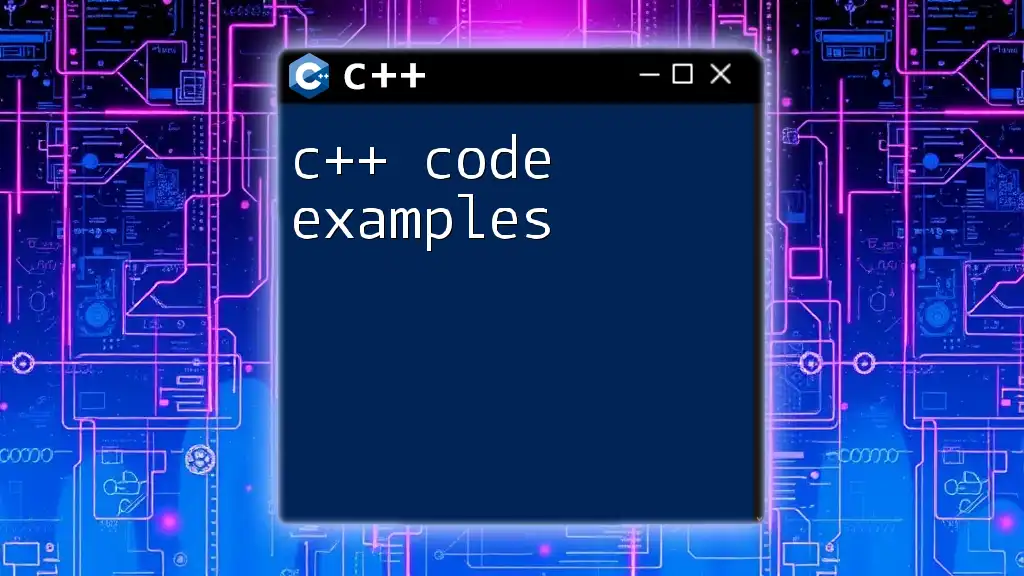
Conclusion
Utilizing C++ in VSCode opens up many avenues for effective programming. The combined power of C++ and the versatile features of VSCode, such as IntelliSense and efficient debugging tools, creates a potent environment for both seasoned developers and beginners.
Explore the features, configurations, and best practices covered in this guide to make the most out of your C++ programming experience. Embrace the journey of learning and mastering your C++ skills, and don’t hesitate to explore further resources in the coding community!