C++ code generation involves automatically creating C++ source code using algorithms or templates to streamline development processes and increase efficiency.
#include <iostream>
// Simple code generation example using macros
#define PRINT_HELLO() std::cout << "Hello, World!" << std::endl;
int main() {
PRINT_HELLO(); // Generates a print command
return 0;
}
What is Code Generation?
C++ code generation refers to the processes and techniques for automatically creating C++ source code, which can significantly streamline development workflows. By generating boilerplate and repetitive code programmatically, developers can maintain focus on the logic that differentiates their applications, leading to more efficient production cycles. Code generation can reduce errors, enhance consistency, and improve productivity.
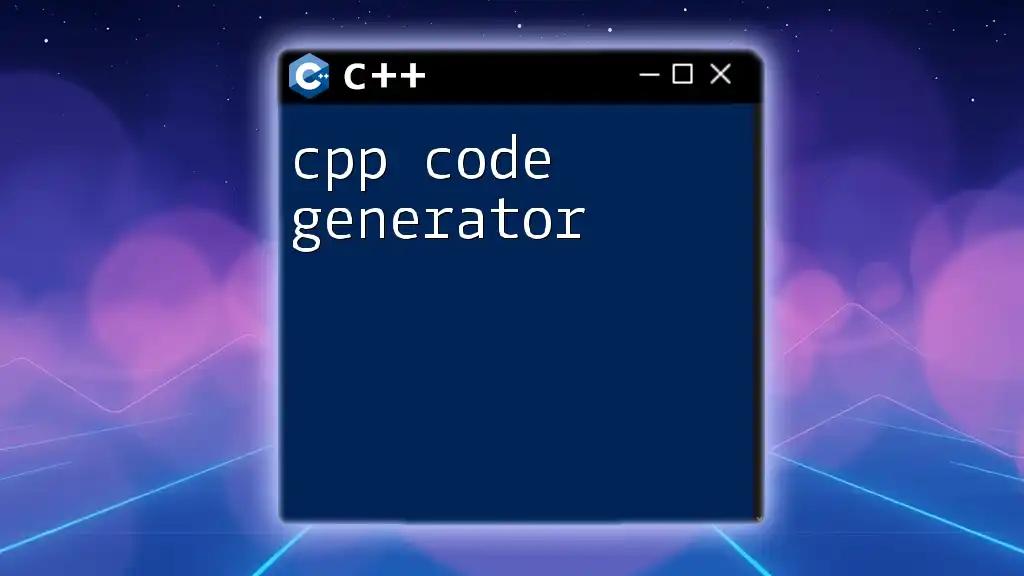
Why Use Code Generation in C++?
Using code generation in C++ is advantageous for several reasons:
-
Efficiency: Automated tools can create large amounts of repetitive code quickly, helping developers save time on mundane tasks.
-
Consistency: Code generators typically follow uniform conventions, producing consistent code structure that adheres to best practices.
-
Reduced Errors: Automated generation minimizes human errors in code writing, providing fewer bugs in the initial implementation.
With these benefits, it is clear that incorporating code generation techniques into C++ development can lead to more robust and scalable applications.
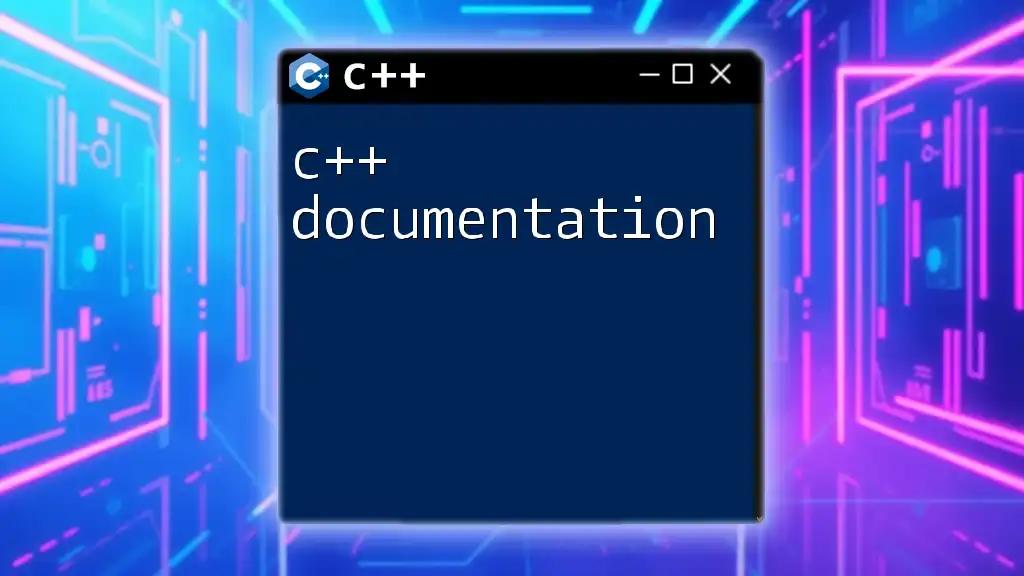
Understanding C++ Code Generation Techniques
Static Code Generation
What is Static Code Generation?
Static code generation occurs during the compilation phase. It involves generating code that is then compiled into the final executable. This method ensures that all the generated code complies with the type system and catches errors at compile time.
Benefits of Static Code Generation
One of the primary benefits of static code generation is increased performance; since the code is generated upfront, the runtime overhead is typically lower. Additionally, static generation allows for thorough compile-time checks, promoting type safety and stability.
Examples of Static Code Generation
A classic example of static code generation involves templates in C++. Here’s a simple code snippet:
template<typename T>
class MyClass {
public:
T value;
MyClass(T val) : value(val) {}
};
In this example, `MyClass` can be instantiated with different data types, leading to the generation of multiple type-specific implementations without unnecessarily duplicating code.
Dynamic Code Generation
What is Dynamic Code Generation?
In contrast, dynamic code generation occurs at runtime. It involves creating or modifying code blocks while the application is running, which provides a high degree of flexibility and adaptability based on user inputs or specific conditions.
Benefits of Dynamic Code Generation
Dynamic code generation allows applications to adjust behavior without the need for recompilation. It can serve to implement features such as plugins, where functionality can be extended or altered based on user requirements.
Examples of Dynamic Code Generation
A typical use case for dynamic code generation can be seen in the utilization of function pointers. Here’s how this can be implemented:
void myFunction() {
// Code for function goes here
}
void (*funcPtr)();
funcPtr = &myFunction; // Assign function to the pointer
funcPtr(); // Call the function dynamically
In this code snippet, we dynamically assign the function to a pointer and invoke it, demonstrating runtime flexibility.
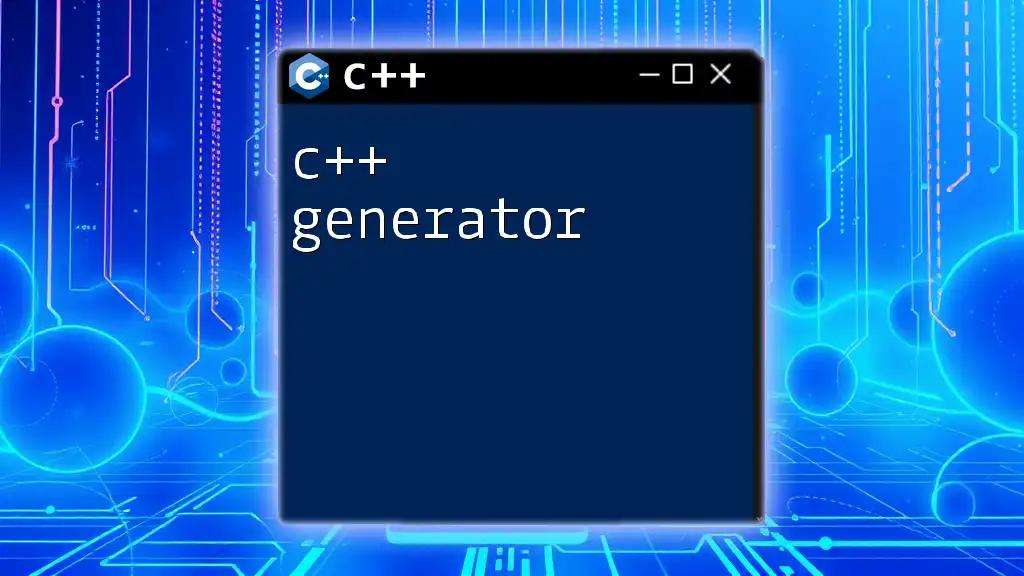
Tools and Libraries for C++ Code Generation
C++ Code Generators
Overview of Popular C++ Code Generators
Various tools assist developers in generating C++ code efficiently. Some prominent code generators include:
- CMake: A cross-platform tool that automates the build process by creating makefiles and project files. It’s suitable for large C++ projects.
- CodeSmith: A template-based code generation tool that can scaffold out code components for various languages, including C++.
How to Use CMake for Code Generation
Setting up a CMake project is straightforward and involves the following steps:
-
Create a CMakeList.txt: This file outlines the project structure and defines how the build process should be conducted.
cmake_minimum_required(VERSION 3.10) project(MyProject) add_executable(myExecutable main.cpp)
-
Generate Makefiles: Once your CMakeList is ready, execute CMake to generate the necessary makefiles by running the command:
cmake .
-
Build the Project: Finally, use the following command to compile the project:
make
Parsing Tools for Code Generation
Introduction to Parsing Libraries
Parsing libraries like ANTLR can be used for developing custom code generators. By defining a grammar, developers can create tools that generate C++ source code based on specific input.
Example of Using ANTLR for C++ Code Generation
To utilize ANTLR in C++, one needs to define the grammar for the desired language syntax. Here is a brief example of what the grammar might resemble:
grammar MyGrammar;
expr : ID '=' INT;
ID : [a-zA-Z]+ ;
INT : [0-9]+ ;
From this grammar, ANTLR can generate a parser that will create the necessary C++ code to handle the defined constructs, streamlining the parsing process significantly.
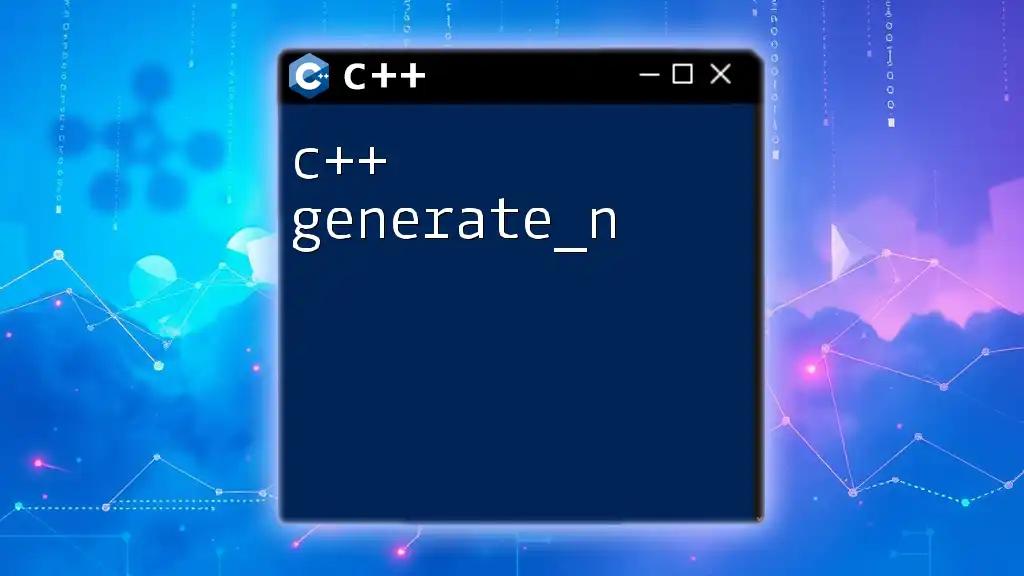
Best Practices in C++ Code Generation
Writing Maintainable Generated Code
Importance of Code Style and Standards
When generating code, adhering to established style guides and coding standards is crucial. This practice not only aids in maintaining uniformity but also improves the overall readability of the codebase.
Techniques for Making Generated Code Readable
- Consistent Naming Conventions: Ensure that classes, methods, and variables adhere to a coherent naming policy throughout the generated code.
- Proper Indentation and Formatting: Utilize tools that format generated code to improve legibility.
- Commenting Generated Code: Include comments that explain the purpose of generated code sections, enhancing maintainability.
Debugging Generated Code
Common Challenges in Debugging
Debugging code that is produced automatically can present challenges, such as the presence of autogenerated comments that offer little contextual information. Additionally, the complexity of macro-generated code may lead to difficulties in tracing back to the source logic.
Tips for Effective Debugging
- Utilize Debugging Tools: Employ debuggers effectively to step through generated code and identify issues.
- Implement Logging: Introduce logging within generated code to gain insights into runtime behavior and errors, making fault detection easier.
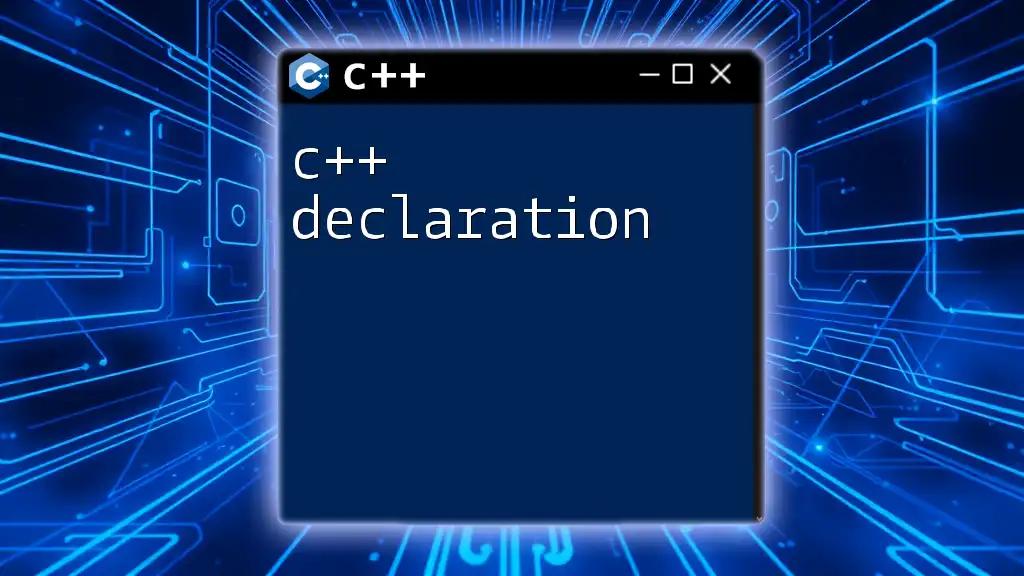
Use Cases of C++ Code Generation
Automated API Generation
C++ code generation can be particularly beneficial for creating API wrappers. By automating the creation of API call structures, developers can easily manage complex integrations with external services, enhancing productivity.
Code Generation in Game Development
Game engines often leverage code generation to manage assets and streamline object creation. By automating processes such as resource loading or level generation, developers can focus on gameplay mechanics more effectively, leading to richer experiences for players.

Conclusion
C++ code generation offers powerful techniques to enhance productivity and maintainability in software development. By leveraging both static and dynamic generation methods, developers can create quality code more efficiently. As technology evolves, the role of code generation in C++ is likely to expand, solidifying its place in modern development workflows.
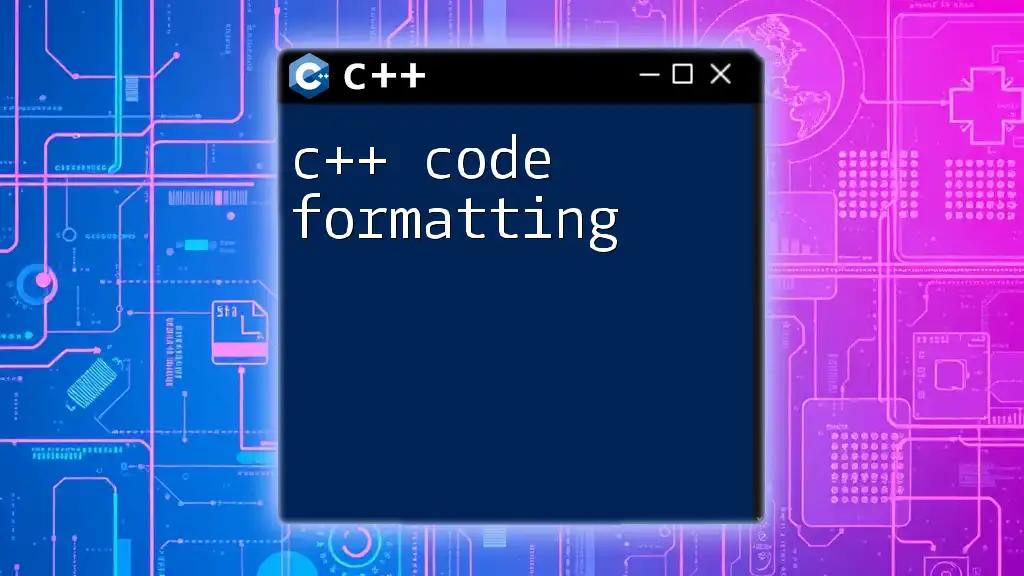
Additional Resources
For further exploration of C++ code generation, consider consulting the following:
- Books and Online Tutorials: Numerous resources can provide insights and deeper dives into advanced code generation techniques.
- Community Forums for C++ Developers: Engaging with the community can offer practical advice and shared experiences that improve your understanding.
- Recommended Tools and Libraries: Familiarize yourself with various libraries and tools that can facilitate effective C++ code generation.