The C++ scope resolution operator `::` is used to define the context in which an identifier (such as a variable, function, or class) is declared, enabling access to global variables or allowing the use of members of a namespace or class.
Here’s a code snippet illustrating its usage:
#include <iostream>
int value = 10; // global variable
namespace MyNamespace {
int value = 20; // namespace variable
}
class MyClass {
public:
static int value; // class variable
};
int MyClass::value = 30; // definition of the class variable
int main() {
std::cout << "Global value: " << ::value << std::endl; // Access global variable
std::cout << "Namespace value: " << MyNamespace::value << std::endl; // Access namespace variable
std::cout << "Class value: " << MyClass::value << std::endl; // Access class static variable
return 0;
}
Understanding Scope Resolution in C++
What is Scope in C++?
In C++, scope refers to the region of the program where a variable or function is accessible. It plays a crucial role in defining the visibility and lifetime of variables, impacting how and where they can be used. Proper understanding of scope is essential for avoiding naming conflicts and ensuring that your code behaves as expected.
Introduction to Scope Resolution Operator
The scope resolution operator in C++ (denoted by `::`) is a vital tool for accessing variables or functions from different scopes, such as global or class scope. Its primary purpose is to specify the context in which an identifier is defined, ensuring that the correct definition is used when there are multiple identifiers with the same name.
The general syntax of the scope resolution operator is as follows:
namespace::identifier
className::member
This allows you to clearly identify and access the correct variable, function, or class member.
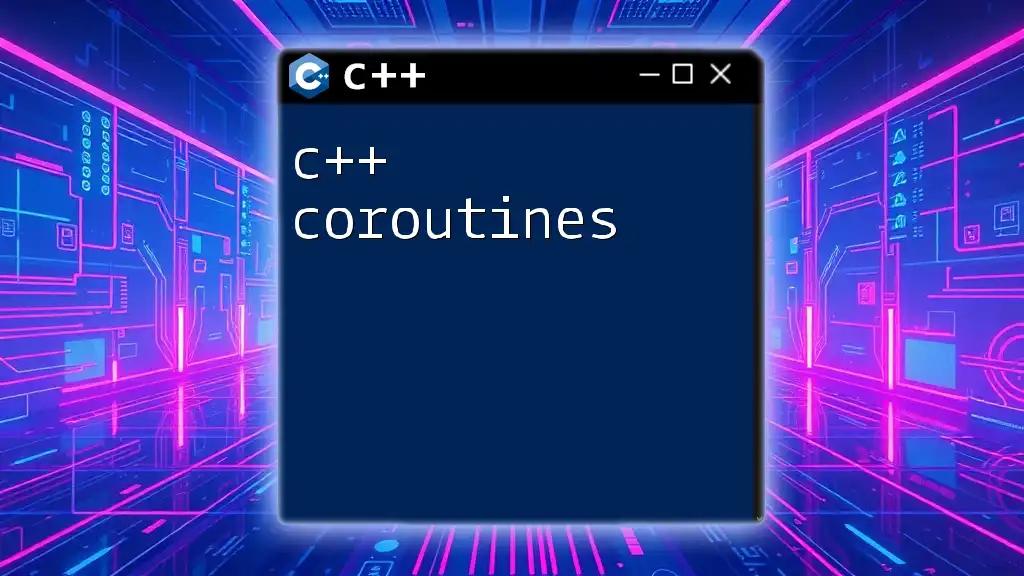
The C++ Scope Resolution Operator
Purpose of the Scope Resolution Operator
The main use of the scope resolution operator is to differentiate between local and global variables. When you declare a variable inside a function (local variable) with the same name as one declared outside it (global variable), the local variable will overshadow it. You can use the scope resolution operator to access the global variable from within the function.
Syntax Breakdown
The syntax for using the scope resolution operator is straightforward, yet powerful. Here’s a simple example illustrating its use:
#include <iostream>
using namespace std;
int value = 10; // Global variable
void display() {
int value = 20; // Local variable
cout << "Local value: " << value << endl;
cout << "Global value: " << ::value << endl; // Accessing global variable
}
int main() {
display();
return 0;
}
In this example, the `display` function has a local variable `value` that overshadows the global variable of the same name. However, by using the scope resolution operator (`::value`), we can still access the global variable.
Accessing Class Members with the Scope Resolution Operator
In the context of classes, the scope resolution operator is pivotal for accessing member variables and functions. It helps in distinguishing between the class members and other identifiers that may share the same name.
For example:
class MyClass {
public:
int myVar;
void setVar(int val) {
myVar = val; // Accessing member variable using implicit 'this' pointer
}
};
int main() {
MyClass obj;
obj.setVar(5);
return 0;
}
In this code, the `setVar` method directly assigns a value to the `myVar` member variable. When needed, you could also explicitly use the scope resolution operator to clarify that you are accessing a class member.
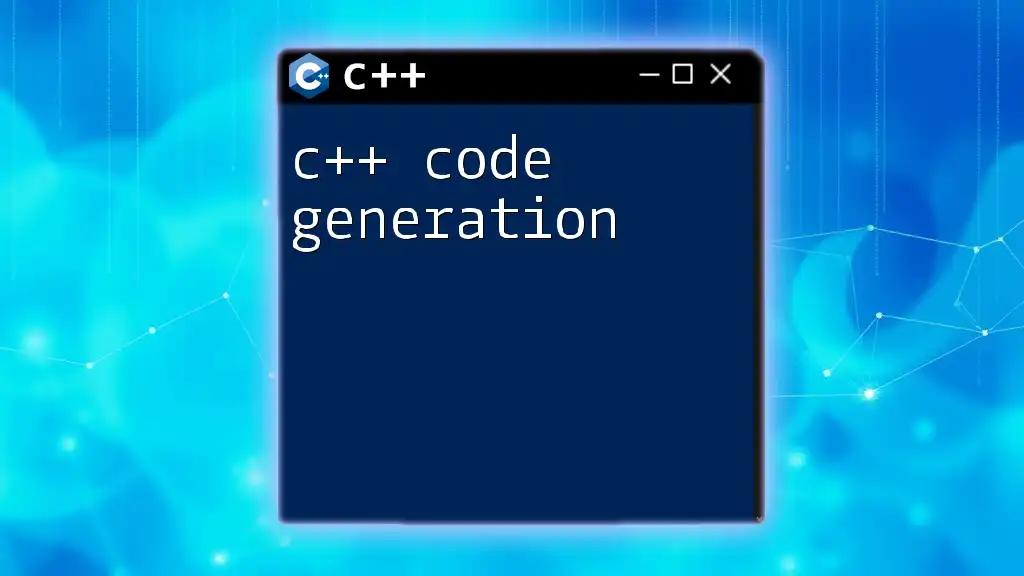
Scope Resolution in Classes
Using Scope Resolution for Static Members
A static member of a class belongs to the class itself rather than any particular object. The scope resolution operator is essential for accessing these static members.
Here’s an example:
class MyClass {
public:
static int staticVal;
static void display() {
cout << "Static Value: " << staticVal << endl;
}
};
int MyClass::staticVal = 10; // Definition of static member outside class
int main() {
MyClass::display(); // Calls static function
return 0;
}
In this case, `staticVal` can be accessed without needing to create an instance of `MyClass`, showcasing the convenience of the scope resolution operator.
Inheriting Classes and the Scope Resolution Operator
When working with inheritance in C++, the scope resolution operator becomes crucial for member access across base and derived classes. It allows you to specify which class's member you are trying to access, thus avoiding ambiguity.
Example: Using Scope Resolution in Base and Derived Classes
Here’s a practical example of using the scope resolution operator when dealing with derived and base class members:
class Base {
public:
void show() {
cout << "Base Class" << endl;
}
};
class Derived : public Base {
public:
void show() {
cout << "Derived Class" << endl;
}
};
int main() {
Derived d;
d.show(); // Calls derived class show
d.Base::show(); // Calls base class show
return 0;
}
By employing the scope resolution operator, you can unambiguously call the `show` method from the `Base` class even when the derived class has overridden it.

Scope Resolution in Namespaces
Understanding Namespaces in C++
Namespaces are a way to group entities in C++, preventing naming conflicts. They are particularly useful in larger projects where identifier collisions are common.
Using the Scope Resolution Operator with Namespaces
You can access functions or variables declared within a namespace using the scope resolution operator. This allows you to maintain clean and organized code.
Example: Namespaces and the Scope Resolution Operator
Here’s an example of using namespaces alongside the scope resolution operator:
namespace MyNamespace {
int x = 10;
}
int main() {
cout << "Namespace Variable: " << MyNamespace::x << endl; // Accessing variable in namespace
return 0;
}
This example demonstrates how easy it is to access variables defined in a namespace using the scope resolution operator.

Additional Features of Scope Resolution in C++
Operator Overloading and Scope Resolution
Operator overloading allows you to define custom behavior for operators when used with user-defined types. The scope resolution operator can clarify which overloaded function you are using.
Using Scope Resolution in Friend Functions
A friend function can access private and protected members of a class. When using friend functions with the scope resolution operator, you can specify which class member you want to access.
class MyClass {
friend void display(MyClass);
private:
int myVar;
public:
MyClass(int val) : myVar(val) {}
};
void display(MyClass obj) {
cout << "Friend Function Access: " << obj.myVar << endl;
}
int main() {
MyClass obj(10);
display(obj); // Correctly accesses private member using friend function
return 0;
}
In this example, the friend function `display` can access the private `myVar` member without needing a public interface.
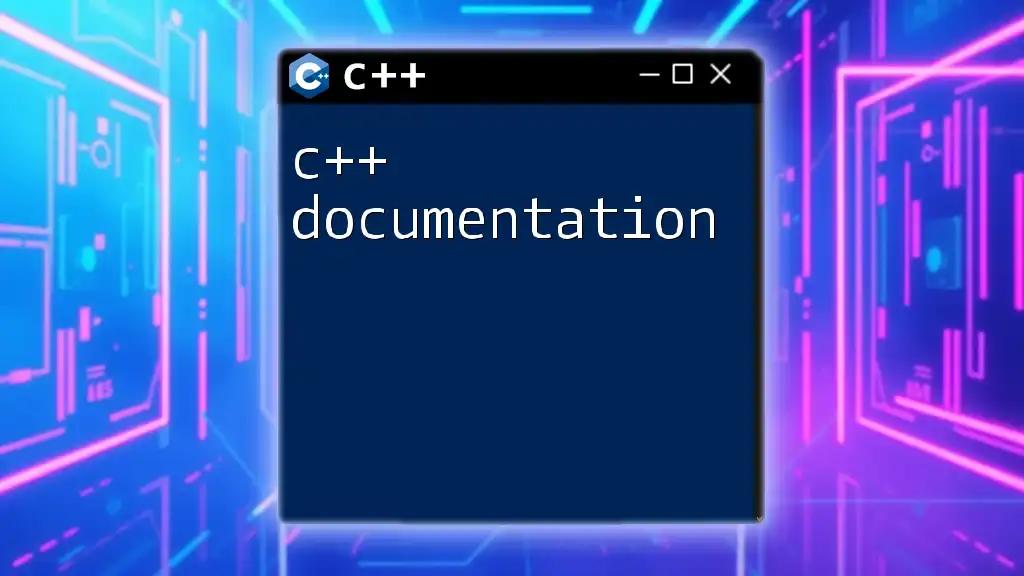
Best Practices for Using the Scope Resolution Operator
When to Use the Scope Resolution Operator
The scope resolution operator should be employed when:
- Accessing a global variable within a local context.
- Differentiating between class members and local identifiers.
- Specifying namespaces in order to improve code organization.
Common Pitfalls to Avoid
- Overuse: Using the scope resolution operator excessively can make code harder to read. Maintain a balance and only use it when necessary.
- Ambiguity: Relying on default accesses might create ambiguity. Always prefer to be explicit about the scope when multiple options exist.
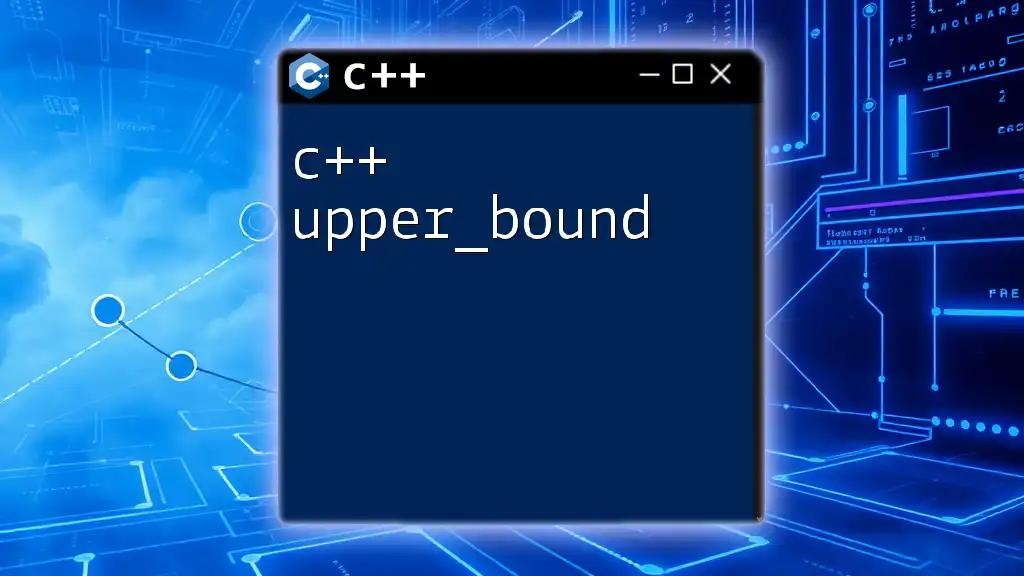
Conclusion
Understanding C++ scope resolution is key to writing clear and effective C++ code. Mastering the scope resolution operator not only enhances your programming efficiency but also establishes a solid foundation for tackling varying complexities in larger applications. As you continue your journey in C++, taking the time to learn and apply the concepts of scope and resolution will pay dividends in creating robust software solutions.
Encouragement for Further Learning
To further deepen your understanding, explore various resources related to advanced C++ topics, such as classes, inheritance, and operator overloading. Practice writing concise code snippets and apply the scope resolution operator to practical examples to reinforce your learning.