The `std::upper_bound` function in C++ returns an iterator to the first element in a sorted range that is greater than a specified value, effectively helping to determine the insertion point for maintaining order.
Here's a code snippet demonstrating its usage:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 3, 5, 7, 9};
int value = 5;
auto it = std::upper_bound(vec.begin(), vec.end(), value);
std::cout << "The upper bound of " << value << " is at position: " << std::distance(vec.begin(), it) << std::endl;
return 0;
}
What is `upper_bound`?
`upper_bound` is a powerful function in the C++ Standard Library that utilizes binary search to locate the position of a specific value within a sorted range. Specifically, it identifies the point in the range where the next element is greater than a given value. This function is integral to optimizing searches in sorted collections, making it a valuable addition to any C++ programmer's toolkit.
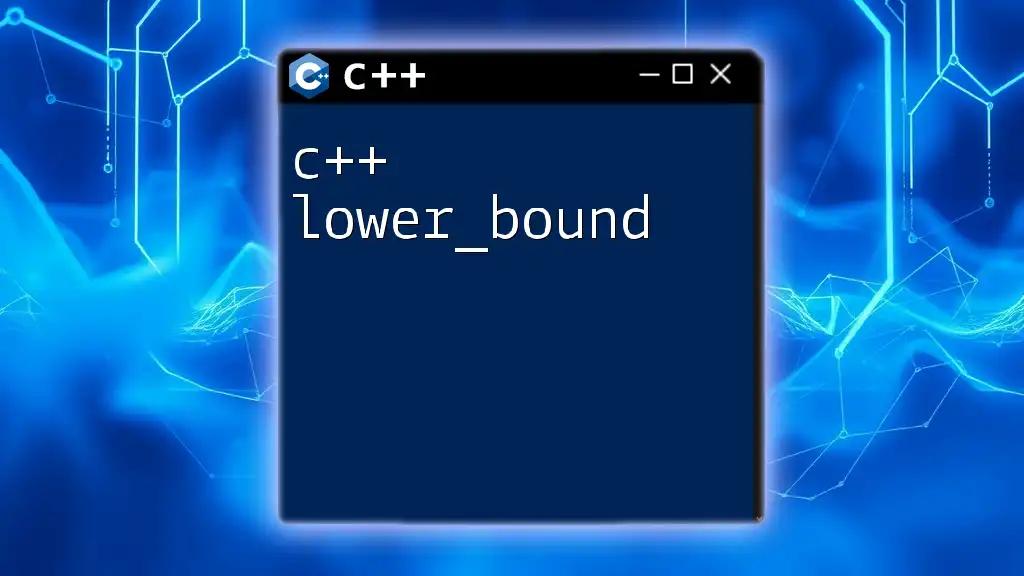
Understanding Binary Search
What is Binary Search?
Binary search is an efficient algorithm designed to locate an item from a sorted collection of items. It operates by repeatedly dividing the search interval in half, effectively honing in on the desired value. The primary requirement for binary search to function correctly is that the data being searched must be sorted.
How `upper_bound` Relates to Binary Search
The `upper_bound` function is a concrete implementation of the binary search algorithm. It takes advantage of the sorted nature of the data to skip over large portions of the collection, drastically improving search efficiency. Instead of checking each element in sequence, which would take linear time, `upper_bound` performs this operation in logarithmic time, O(log n).
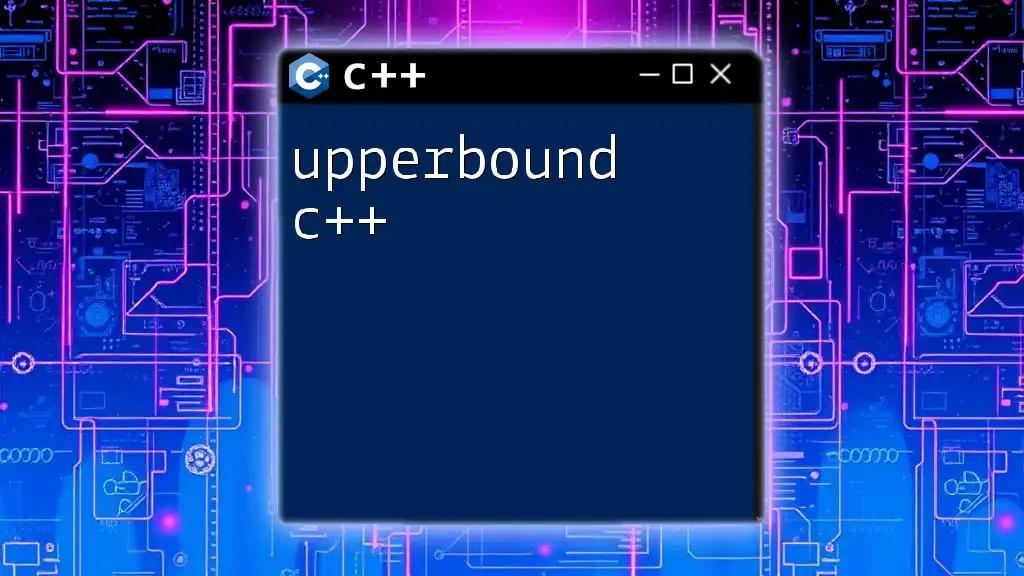
Syntax of `upper_bound`
Function Signature
The syntax for `upper_bound` is defined as follows:
template<class ForwardIt, class T>
ForwardIt upper_bound(ForwardIt first, ForwardIt last, const T& value);
Breakdown of Parameters:
- `ForwardIt first`: This is an iterator that points to the beginning of the range.
- `ForwardIt last`: This is an iterator that points to the end of the range (exclusive).
- `const T& value`: This is the value you want to compare against to find the upper bound.

Key Features of `upper_bound`
Return Value
The `upper_bound` function returns an iterator pointing to the first element in the range that is greater than the specified value. If no such element exists, it returns an iterator to `last`. This means that, if you call `upper_bound` on a sorted array containing `10, 20, 30`, and you search for `20`, the function will return the iterator pointing to `30`.
Complexity
The time complexity of `upper_bound` is O(log n), thanks to its binary search mechanics. This makes it vastly more efficient than a linear search algorithm, especially for large datasets. In contrast, linear search would require O(n) time, making `upper_bound` a more scalable option for seeking elements in sorted collections.
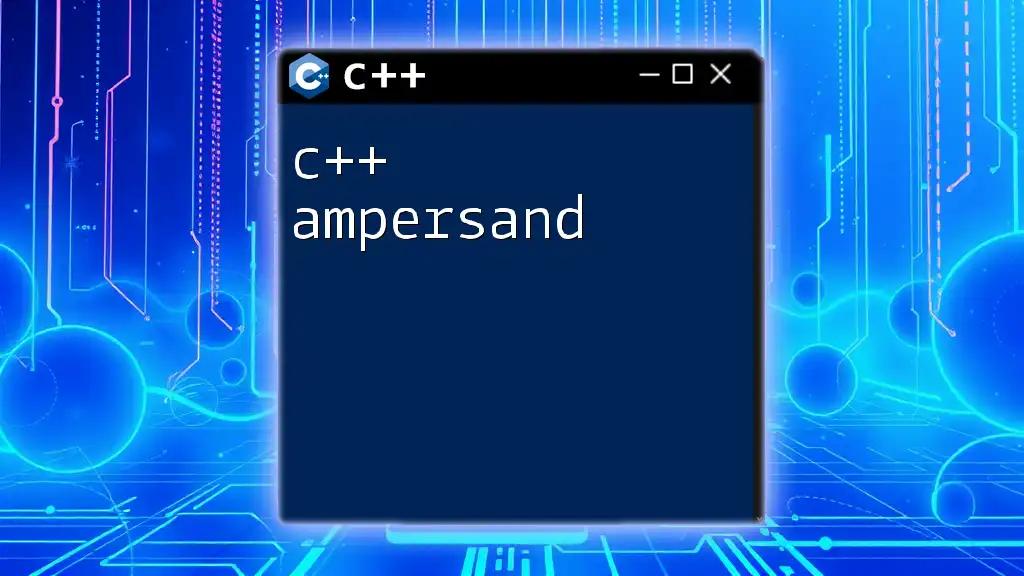
Practical Usage of `upper_bound`
Pre-requisites: Sorted Data
Before using `upper_bound`, it is essential that the data you are searching through is sorted. If the data is not sorted, the results of `upper_bound` will be undefined. To ensure your data is sorted, you can use the `std::sort` algorithm from the `<algorithm>` header in C++.
Example Use Case
Here’s a practical example demonstrating how to utilize `upper_bound`:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {1, 2, 4, 4, 5, 6};
int value = 4;
auto it = std::upper_bound(numbers.begin(), numbers.end(), value);
std::cout << "The next higher number after " << value << " is "
<< (it != numbers.end() ? *it : -1) << std::endl;
return 0;
}
In this example, we create a vector called `numbers` containing sorted integers. We then search for the number `4`. The `upper_bound` function will return an iterator that points to the first element greater than `4`, which is `5`. If `4` were the largest number, the iterator would point to `end()`.
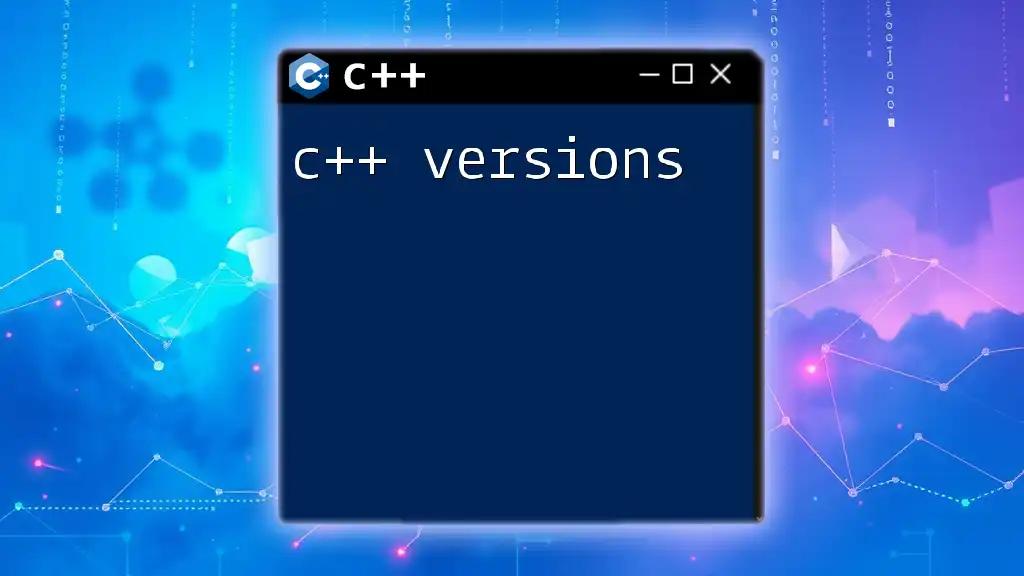
Advanced Scenarios
Using `upper_bound` with Custom Types
`upper_bound` can also be used to search through collections of custom objects. To do this effectively, you will need to define a custom comparator. Below is an example using a custom struct:
#include <iostream>
#include <vector>
#include <algorithm>
struct Point {
int x;
int y;
};
bool operator<(const Point& a, const Point& b) {
return a.x < b.x; // Compares based on x-coordinate
}
int main() {
std::vector<Point> points = {{1, 2}, {2, 3}, {3, 4}};
Point p = {2, 0};
auto it = std::upper_bound(points.begin(), points.end(), p);
if (it != points.end()) {
std::cout << "Next point is (" << it->x << ", " << it->y << ")" << std::endl;
}
return 0;
}
In this scenario, the `Point` struct represents a two-dimensional point. We define a custom comparison operator to compare `Point` objects based on their `x` coordinate. The example shows how to use `upper_bound` to find the next point after the provided point `p`, showcasing the diversity of `upper_bound` in handling user-defined types.
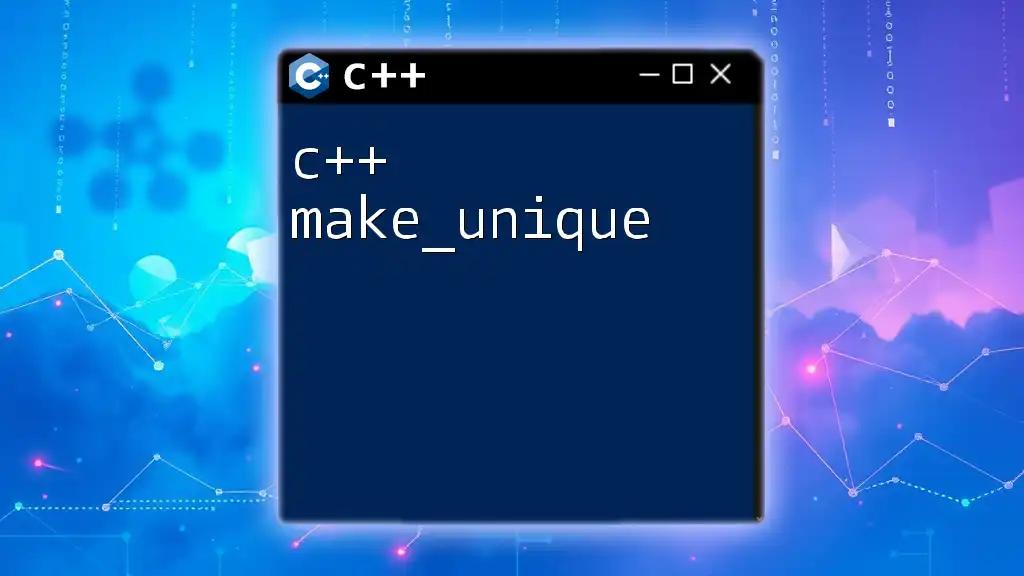
Common Mistakes to Avoid
Misunderstanding Return Behavior
One common mistake is misinterpreting the iterator that `upper_bound` returns. It does not return the element equal to the search value; instead, it finds the position of the next greater element. It’s crucial to understand this distinction to avoid logic errors in your code.
Forgetting Sort Requirement
Another frequent oversight is neglecting the requirement for sorted input. Always ensure that your data is sorted prior to calling `upper_bound`, as operating on unsorted data will yield undefined behavior. Always sort your data first by using `std::sort` like so:
std::sort(numbers.begin(), numbers.end());

Conclusion
In summary, `upper_bound` is a highly efficient function for locating elements within sorted collections in C++. By utilizing binary search, it offers a significant performance advantage, making it a staple for search operations. Understanding how it works and ensuring proper usage will enhance your proficiency in C++ and streamline your code.
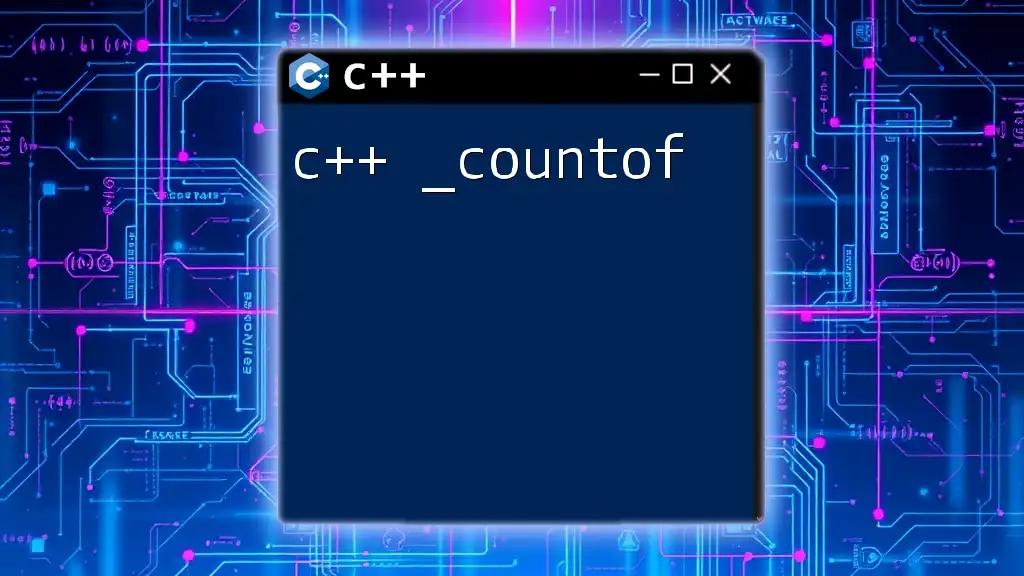
Where to Go Next?
If you're keen to expand your knowledge, consider reading up on other search algorithms, such as `lower_bound`, and experimenting with different data structures in C++.Utilizing these tools will further strengthen your capabilities in the C++ programming language.

Additional Resources
For further reading, you can refer to the official C++ documentation on `upper_bound` available through various online platforms. Books and tutorials focused on advanced C++ features can also provide deeper insights into effectively harnessing the capabilities of the C++ Standard Library.

Call to Action
Now that you’ve learned the ins and outs of `c++ upper_bound`, start practicing! Use it in your own projects, and don't hesitate to share your experiences or pose any questions you may have in the comments section below. Happy coding!