A C++ randomizer allows you to generate random numbers or select random elements from a dataset, enhancing the randomness in your applications.
Here's a simple code snippet demonstrating how to use the C++ `<random>` library to generate a random number between 1 and 100:
#include <iostream>
#include <random>
int main() {
std::random_device rd; // Obtain a random number from hardware
std::mt19937 eng(rd()); // Seed the generator
std::uniform_int_distribution<> distr(1, 100); // Define the range
int random_number = distr(eng); // Generate the random number
std::cout << "Random number: " << random_number << std::endl; // Output the random number
return 0;
}
Understanding Randomness in C++
The Importance of Randomization
Randomization plays a crucial role in many areas of computing and programming. In the world of software development, random numbers can serve vital functions such as simulating various scenarios, ensuring the unpredictability inherent in games, and testing algorithms. The significance of randomness cannot be overstated, as it provides developers with the tools to create more dynamic and engaging programs.
C++ is an excellent choice for random number generation due to its performance and vast ecosystem. It offers powerful libraries and resources that leverage randomness effectively. Utilizing C++ not only allows for high-speed execution but also provides access to robust algorithms, making it ideal for both simple tasks and complex simulations.
Basics of Random Number Generation
To better understand how to work with a C++ randomizer, it’s essential to differentiate between pseudo-random numbers and true random numbers. Pseudo-random numbers are generated using deterministic processes, which means that given the same initial conditions, they will produce the same sequence of numbers. Conversely, true random numbers are far less common and usually obtained from unpredictable physical processes, such as radioactive decay or thermal noise.
Most programming applications, including those in C++, rely on pseudo-random number generation due to its efficiency. Various algorithms exist for this purpose, ranging from linear congruential generators to Mersenne Twister. Among these algorithms, Mersenne Twister is especially popular due to its long period and high-quality output, making it suitable for most applications.
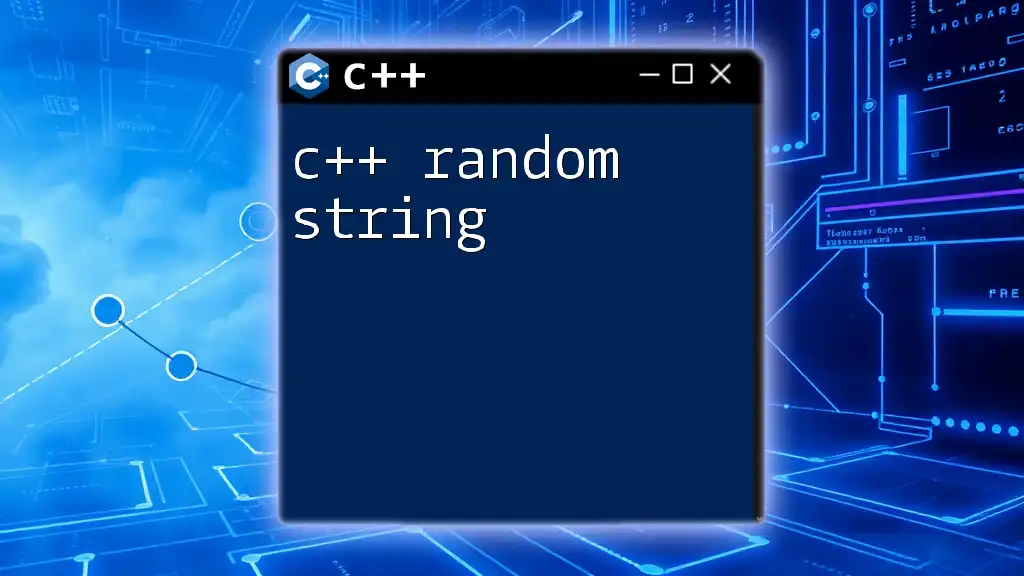
C++ Random Number Generation
The `<random>` Header
The introduction of the `<random>` header in C++11 marked a significant enhancement in random number generation capabilities. Unlike the older `rand()` function provided in `<cstdlib>`, which suffers from limitations like a poor distribution of results, `<random>` provides a more sophisticated approach.
Using `<random>` not only enhances the uniformity of generated numbers; it also allows you to choose from a variety of distributions and engines, thereby tailoring the output to your specific needs.
Components of the `<random>` Library
Random Number Engines
A random number engine is the backbone of random number generation in C++. It produces the raw numbers that can then be shaped into various distributions as per your requirements. Common engines provided in the `<random>` library include:
-
std::default_random_engine: This is a default engine that is typically based on the Mersenne Twister algorithm and offers a balanced trade-off between speed and quality.
-
std::mt19937: An implementation of the Mersenne Twister algorithm, providing excellent quality and a long period of 2^19937−1.
Here is a simple code snippet to illustrate the initialization of a random number engine:
#include <random>
std::default_random_engine generator;
Distributions
Once you have your random number engine, the next step involves defining a distribution. A distribution controls how the generated numbers are spread over a specified range. Some of the commonly used distributions include:
-
Uniform Distribution: Ensures every number within the specified range has an equal chance of being chosen.
-
Normal Distribution: Generates numbers that cluster around a mean value, forming a bell curve.
-
Bernoulli Distribution: Models binary outcomes, ideal for scenarios involving success/failure or yes/no conditions.
To implement a uniform distribution, you can use the following snippet:
std::uniform_real_distribution<double> distribution(0.0, 1.0);
double random_value = distribution(generator);
Creating a Simple Random Number Generator
Creating a simple C++ randomizer is relatively straightforward. The process can be broken down into setting up the random number engine, selecting a distribution, and then generating random numbers.
Here is a complete example that generates five random integers between 1 and 100:
#include <iostream>
#include <random>
int main() {
std::default_random_engine generator;
std::uniform_int_distribution<int> distribution(1, 100);
// Generate 5 random numbers
for(int i = 0; i < 5; ++i) {
std::cout << distribution(generator) << std::endl;
}
return 0;
}
In this example, the code initializes a random number generator and prints five random integers in the defined range. This simple demonstration illustrates the ease of using the `<random>` library to create random outputs.
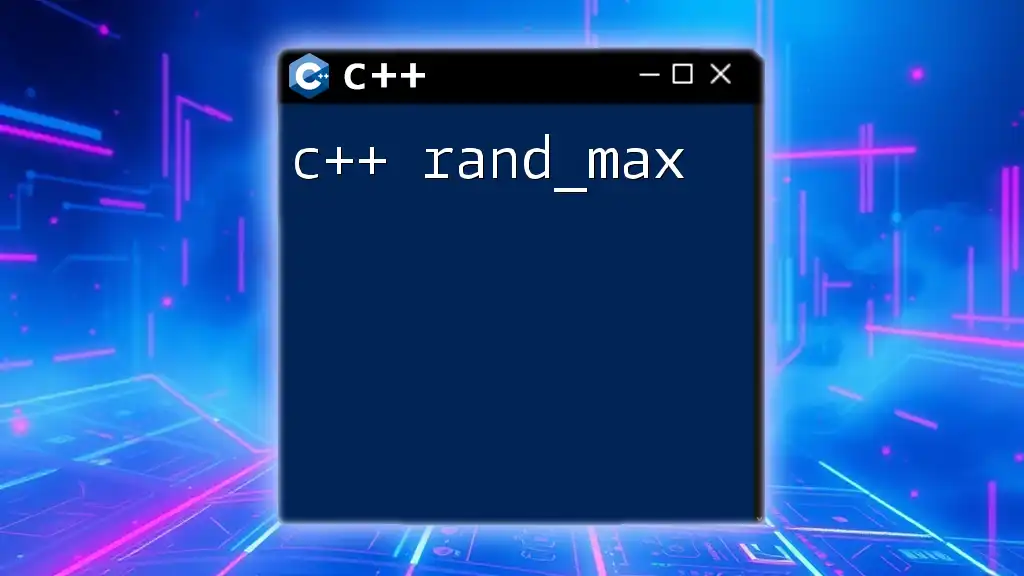
Practical Applications of C++ Randomizers
Randomized Algorithms
Randomized algorithms are a fascinating area in computer science that employ randomness to produce results that may be influenced by chance. Such algorithms can often run faster on average than their deterministic counterparts, leading to improved performance in complex problems.
For instance, Randomized Quick Sort is a well-known sorting algorithm that selects a random pivot. This randomization helps mitigate the worst-case scenario that can occur when data is already sorted or nearly sorted.
Game Development
In game development, randomization is crucial for creating immersive environments. Random elements such as enemy actions, loot drops, or terrain generation add unpredictability, making the gaming experience more engaging.
Here's a simple example that simulates a dice roll in C++:
std::uniform_int_distribution<int> dice(1, 6);
int dice_roll = dice(generator);
std::cout << "Dice Roll: " << dice_roll << std::endl;
With this, each time the dice is rolled, you receive a random number between 1 and 6, enhancing your games' appeal by introducing variability.
Simulations
Randomization is a foundational element in many simulations. One popular application is the Monte Carlo method, which leverages random sampling to obtain numerical results. This technique can be used to model phenomena and assess risks, especially in finance and logistics.
An example code snippet for a Monte Carlo simulation estimating the value of π could look like this:
#include <random>
#include <iostream>
int main() {
std::default_random_engine generator;
std::uniform_real_distribution<double> distribution(0.0, 1.0);
int points_inside_circle = 0;
int total_points = 1000000;
for (int i = 0; i < total_points; ++i) {
double x = distribution(generator);
double y = distribution(generator);
if (x * x + y * y <= 1) {
points_inside_circle++;
}
}
double pi_estimate = 4.0 * points_inside_circle / total_points;
std::cout << "Estimated value of π: " << pi_estimate << std::endl;
return 0;
}
In this example, the program utilizes random coordinates generated within a unit square to approximate the value of π based on the ratio of points inside the unit circle.
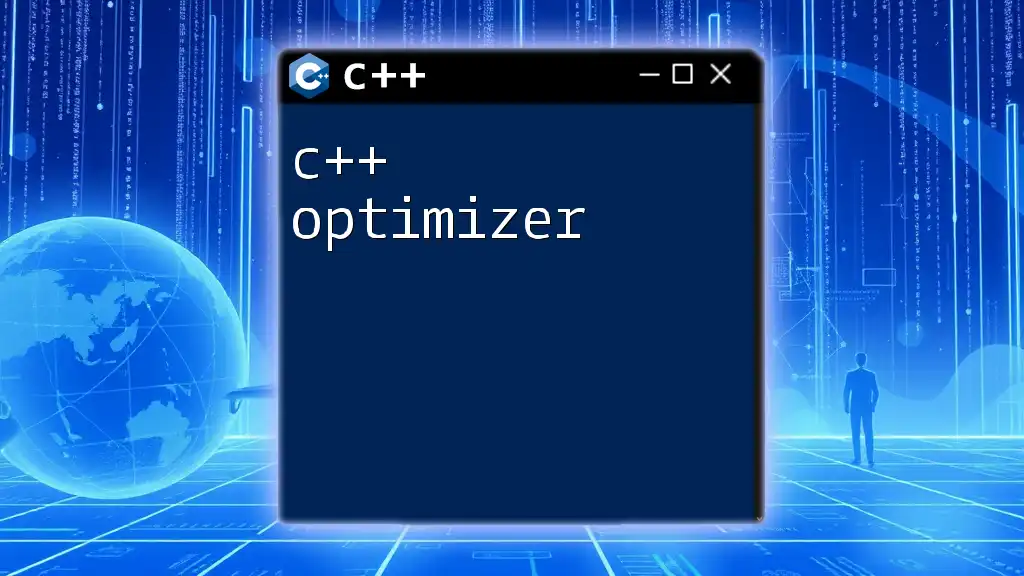
Advanced Techniques
Seeding the Random Number Generator
Seeding is the process of initializing a random number generator to ensure a variety of outputs each time the program runs. By seeding your generator correctly, you can create unpredictable outcomes rather than receiving the same sequence with every execution.
You can seed your random number generator by utilizing the current time:
#include <ctime>
std::default_random_engine generator(static_cast<unsigned int>(time(0)));
Thread Safety in Randomization
As software development increasingly moves towards multi-threading, ensuring thread safety in random number generation has become critical. Concurrent access to a shared random number generator can lead to unpredictable behavior. To avoid such issues, consider using separate generators for each thread or employing synchronization techniques such as mutexes to guard access.
Customized Random Number Generators
Custom random number generators can be designed to meet unique requirements specific to an application. By combining different engines and distributions, you can create a tailored solution that provides desired statistical properties or behavior.
Creating a custom generator may involve defining your logic and integrating it with the C++ random library. Customizing distributions allows you greater flexibility and can lead to more effective simulations or randomized processes.
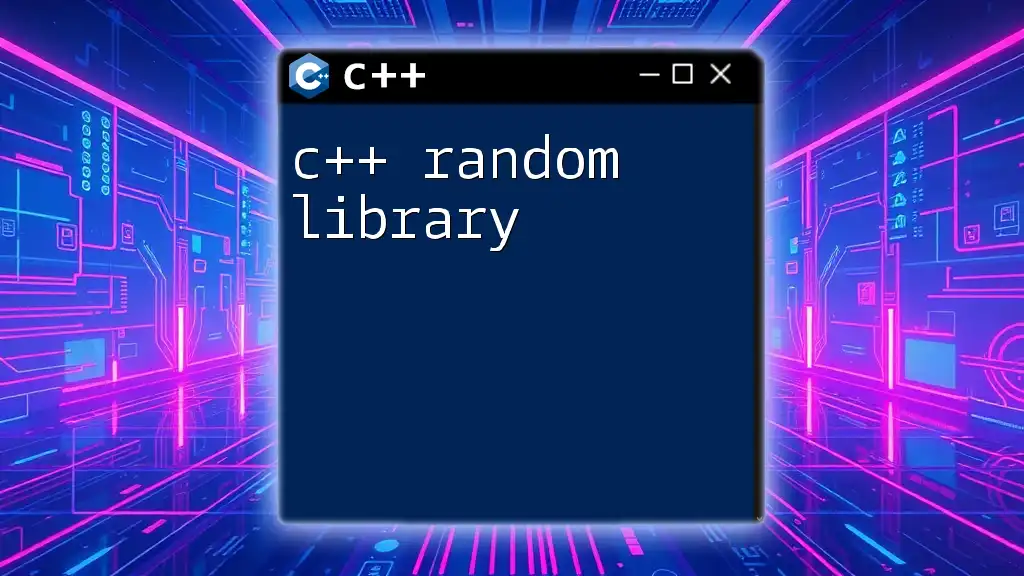
Conclusion
In this exploration of the C++ randomizer, we've delved into the significance of randomness, discussed the components of the `<random>` library, and examined practical applications across various fields.
The versatile capabilities offered by C++ for random number generation enable developers to create dynamic applications that can simulate complex situations or enhance user experiences in games. With its powerful features, the `<random>` library is an invaluable tool for any C++ programmer.
Further Resources
To deepen your understanding of random number generation in C++, consider exploring additional reading materials, code repositories, and forums where you can engage with a community focused on enhancing your skills in this area.
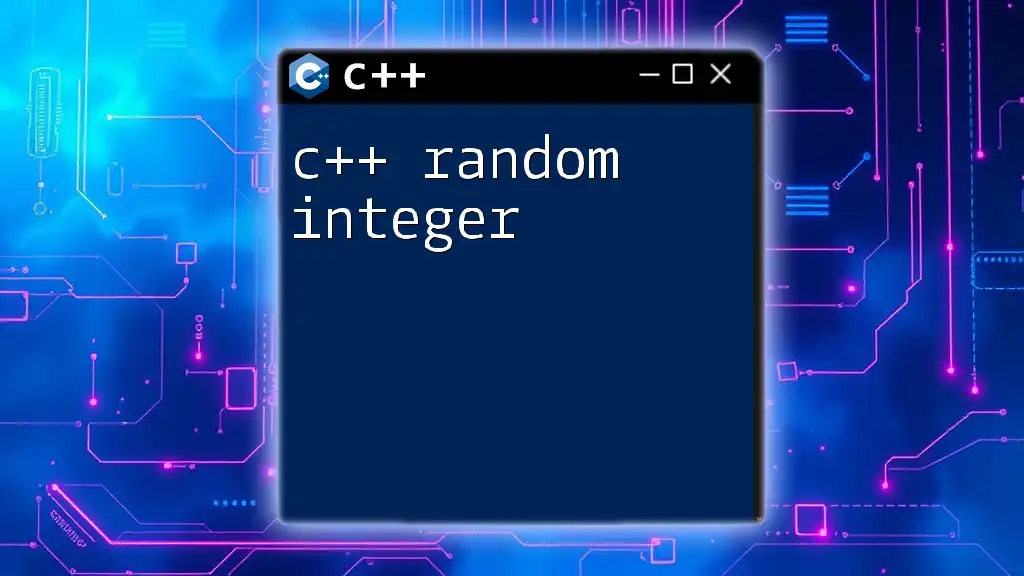
Call to Action
We invite you to share your experiences using C++ for randomization below in the comments. Join our community for more insightful discussions, and don't hesitate to share this article with those eager to learn about C++ programming!