Generating a random string in C++ can be achieved by combining a set of characters with a random selection algorithm to create a desired-length string.
Here’s a simple code snippet to illustrate this:
#include <iostream>
#include <string>
#include <cstdlib>
#include <ctime>
std::string randomString(size_t length) {
const std::string characters = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789";
std::string result;
std::srand(std::time(0)); // Seed for random number generation
for (size_t i = 0; i < length; ++i) {
result += characters[std::rand() % characters.size()];
}
return result;
}
int main() {
std::cout << randomString(10) << std::endl; // Generates a random string of length 10
return 0;
}
Understanding Randomness in C++
What is Randomness?
In the realm of programming, randomness refers to the generation of values that do not follow a predictable pattern. This concept is crucial when you want to introduce variability, such as simulating real-world scenarios or creating unique identifiers. Understanding how to generate random values in C++ is fundamental for many applications, ranging from games to security systems.
The Need for Random Strings
The significance of random strings cannot be overstated, especially in applications where unique and unpredictable data is essential. For instance, when designing a system for user-generated passwords, a strong layer of randomness can prevent unauthorized access. Additionally, random strings can be used in generating test cases or identifiers in databases.

Generating Random Strings in C++
Setting Up the Random Number Generator
To generate a random string in C++, you first need to configure the random number generator. This involves including the appropriate headers and seeding the generator.
Include Necessary Headers
You will need to include the following headers at the start of your code:
#include <iostream>
#include <cstdlib>
#include <ctime>
#include <string>
Seed the Random Number Generator
Seeding the random number generator is vital for ensuring that the sequences of random numbers differ each time your program runs. You can do this using the current time as a seed:
srand(static_cast<unsigned int>(time(0))); // Seed with current time
Creating a Function to Generate Random Strings
Once your random number generator is set up, the next step is to create a function dedicated to generating random strings.
Defining Character Sets
To construct a random string, you must first define the allowed characters. This can include uppercase letters, lowercase letters, digits, and special characters. An example character set might look like this:
const std::string chars = "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789!@#$%^&*()";
Implementing the Function
Creating the function to generate the random string involves using a loop to select a character from the defined set. Here's a simple implementation:
std::string generateRandomString(int length) {
std::string result;
for (int i = 0; i < length; ++i) {
result += chars[rand() % chars.length()];
}
return result;
}
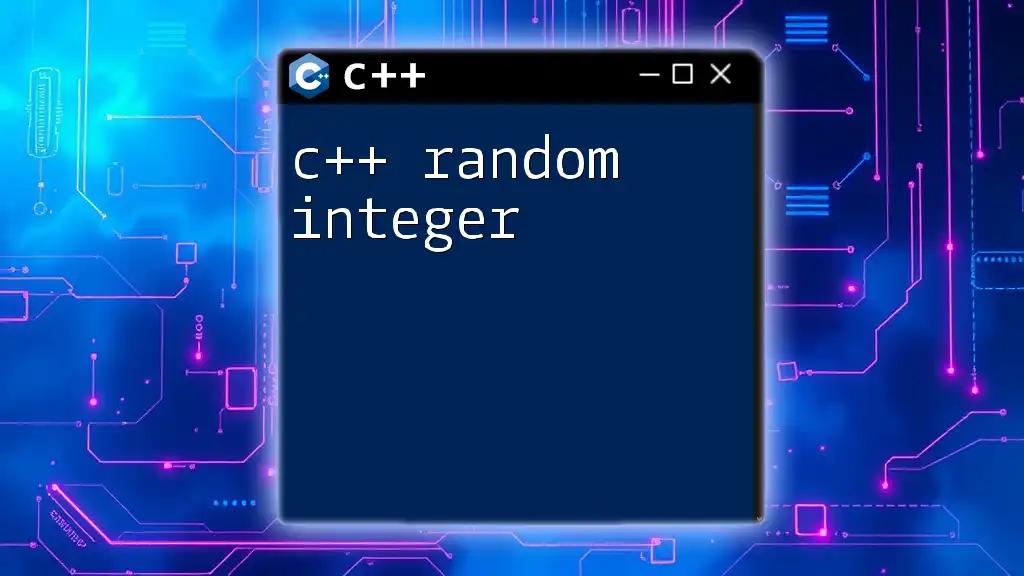
Using the Random String Generator
Practical Examples
Generating a Random Password
One of the most common uses for a random string is as a password. Given the importance of security in today’s digital age, a strong, random password can enhance safety. Here’s how you can generate a random password with the function we created:
std::string password = generateRandomString(12);
std::cout << "Generated Password: " << password << std::endl;
In this example, a password of 12 characters is generated using a mixture of character types, creating a strong security measure.
Creating Unique Identifiers
Unique identifiers are vital in many applications for differentiating records. For instance, in a database, you might want to create unique identifiers for user accounts. You can leverage your random string generator to accomplish this:
std::string uniqueID = generateRandomString(16);
std::cout << "Generated Unique ID: " << uniqueID << std::endl;
In this example, a 16-character unique identifier is produced, which can be useful for tracking users or objects in a system.
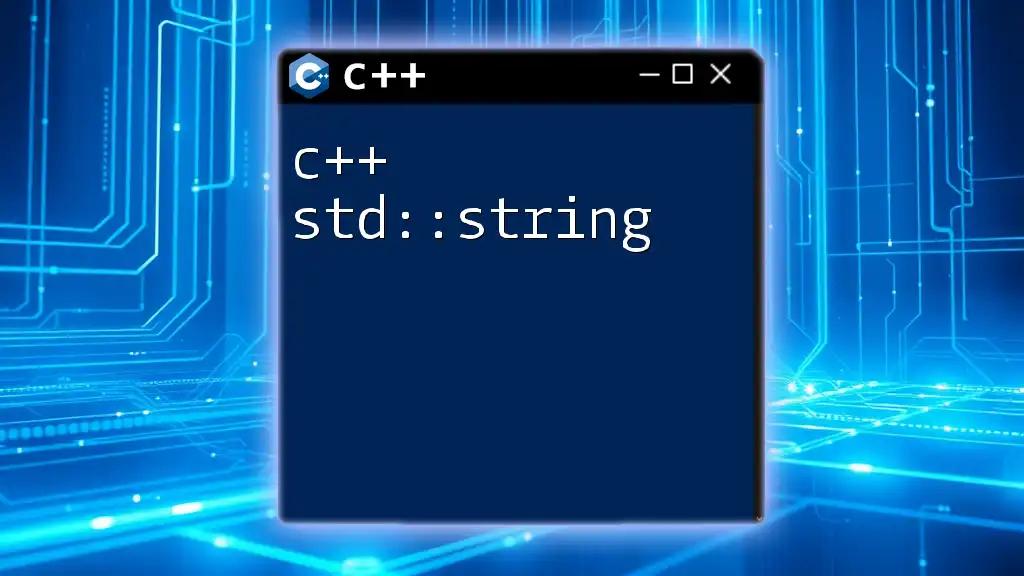
Advanced Techniques
Customizing Character Sets
In some cases, you might want to allow users to define their own character sets for generating random strings. To do this, you can modify the function to accept a second parameter representing the custom character set:
std::string generateRandomString(int length, const std::string& customChars) {
std::string result;
for (int i = 0; i < length; ++i) {
result += customChars[rand() % customChars.length()];
}
return result;
}
This flexibility allows for generating strings with specific requirements, such as alphabetic-only passwords or numeric IDs.
Ensuring Uniqueness
When generating random strings, ensuring uniqueness becomes essential, especially for identifiers. Using a `std::set`, you can store generated strings and check for duplicates:
std::set<std::string> uniqueStrings;
while (uniqueStrings.size() < desiredCount) {
uniqueStrings.insert(generateRandomString(length));
}
Here, you continuously generate random strings until your set reaches a desired count of unique entries, helping you avoid any duplicates.
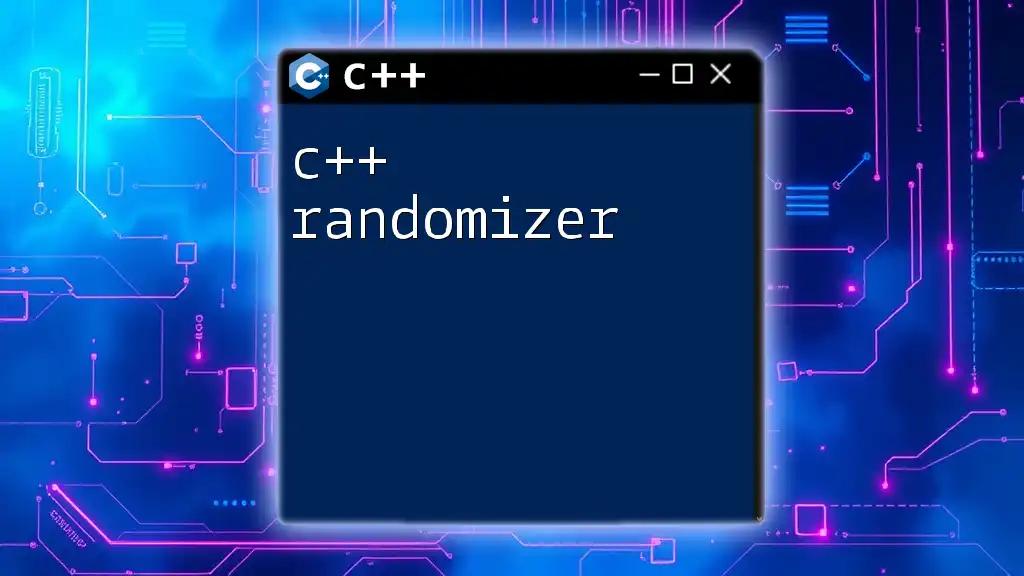
Common Pitfalls and Mistakes
Misunderstanding Randomness
A common misconception is that using a random number generator guarantees perfectly random strings. It’s crucial to understand that the randomness generated by `rand()` is pseudorandom. If you do not use proper seeding, you may end up with the same sequence every time your program runs.
Overreliance on `rand()`
Though `rand()` can be useful, it may not provide sufficient randomness for all applications. For more robust implementations, consider using `std::random_device` and `std::mt19937`:
std::random_device rd;
std::mt19937 gen(rd());
std::uniform_int_distribution<> dis(0, chars.length() - 1);
Using these modern approaches enhances the quality of the randomness in your strings.

Conclusion
By mastering the generation of C++ random strings, you can create secure passwords, unique identifiers, and add variability to your applications. Remember to experiment with different character sets, string lengths, and techniques to customize your solutions. Each concept discussed here opens a new avenue for creativity in your programming projects. Don’t hesitate to share your implementations or thoughts in the comments!