In C++, you can concatenate strings using the `+` operator, allowing you to easily combine multiple strings into one.
#include <iostream>
#include <string>
int main() {
std::string str1 = "Hello, ";
std::string str2 = "world!";
std::string result = str1 + str2;
std::cout << result << std::endl; // Outputs: Hello, world!
return 0;
}
Understanding String Data Types in C++
In C++, strings can be represented using two main types: C-style strings and the more commonly used `std::string`.
- C-style strings are arrays of characters terminated by a null character (`'\0'`). They require manual memory management and often lead to complexities when it comes to string manipulation.
- `std::string`, part of the C++ Standard Library, provides a more flexible and user-friendly interface. It automatically manages memory and supports various operations, making it easier to work with strings.
Using `std::string` is beneficial for string concatenation, as it simplifies tasks such as adding strings together.
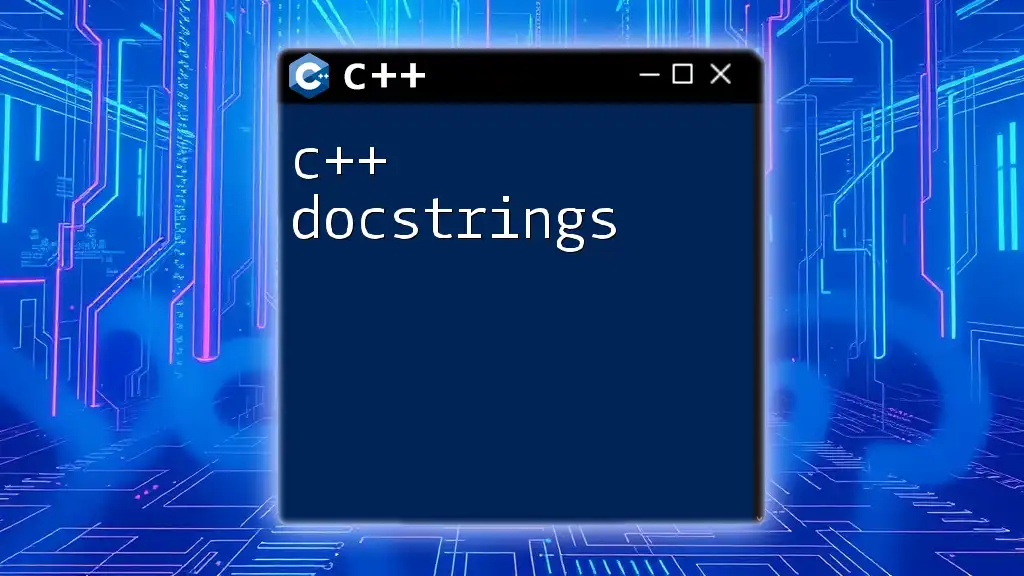
The Basics of String Concatenation in C++
What is String Concatenation?
String concatenation involves combining two or more strings into a single string. It is an essential operation in many programming scenarios, such as constructing messages, generating output, or building user interfaces. Understanding how to effectively c++ add strings will empower you to write more efficient and readable code.
Using the `+` Operator to Concatenate Strings
In its simplest form, you can use the `+` operator to concatenate strings in C++. This is intuitive and straightforward.
Here’s an example of how it works:
std::string str1 = "Hello, ";
std::string str2 = "world!";
std::string result = str1 + str2;
In this example, `result` will hold the value `"Hello, world!"`. The `+` operator invokes the overloaded operator function for `std::string`, which creates a new string by combining the two.
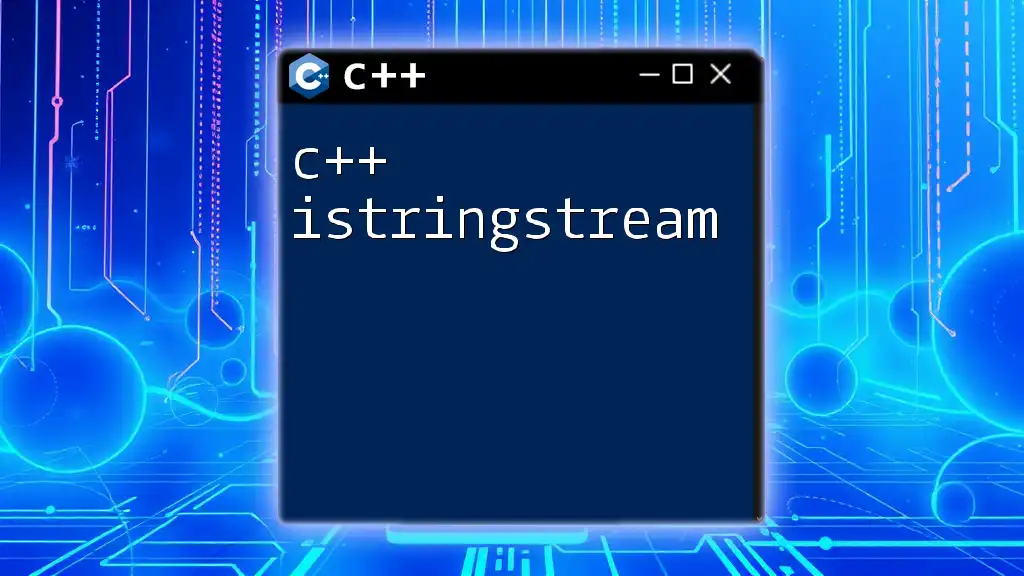
Exploring String Concatenation Functionality
The `append()` Method for Concatenation
The `append()` method is another robust solution for string concatenation. It modifies the string in place by adding another string to it.
Consider the following example:
std::string str = "Hello, ";
str.append("world!");
After executing this code, `str` will now contain `"Hello, world!"`.
Using `append()` can be advantageous when you need to concatenate multiple parts to the same string gradually, as it often can be more efficient than using the `+` operator repeatedly.
The `+=` Operator for Concatenation
The `+=` operator is a shorthand for appending strings, combining functionality for both concatenation and assignment. It enhances code readability.
For instance:
std::string str = "Hello, ";
str += "world!";
The result will again be `"Hello, world!"`. The `+=` operator simplifies the expression, covering both the addition of a string and its assignment back to `str`.
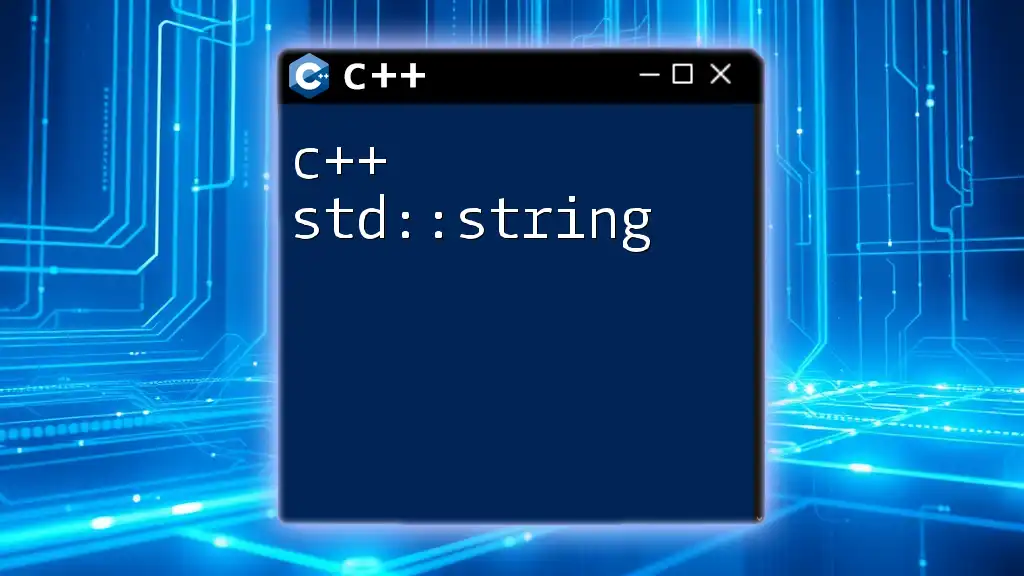
Advanced String Concatenation Techniques
Concatenating Multiple Strings Using `std::ostringstream`
When dealing with complex string constructions, you might prefer using `std::ostringstream` from the `<sstream>` library. This technique is particularly effective for concatenating multiple strings or different data types into a single output.
Here’s how you can do it:
#include <sstream>
std::ostringstream oss;
oss << "Hello, " << "world!";
std::string result = oss.str();
In this case, you've streamed multiple pieces into `oss`, and the final string is extracted using `oss.str()`. This method is efficient for larger or varied data types since it minimizes temporary string creation during the process.
Using `std::vector` for Dynamic String Concatenation
When you need to concatenate multiple strings stored in a collection, consider using a `std::vector<std::string>`. This allows for dynamic string assembly, as shown in the following example:
#include <vector>
#include <numeric> // for std::accumulate
std::vector<std::string> strings = {"Hello, ", "world!", " Welcome to C++!"};
std::string result = std::accumulate(strings.begin(), strings.end(), std::string());
Here, `std::accumulate` iteratively combines each string in the vector into a single result. This approach is particularly powerful for handling larger datasets or dynamically generated strings.
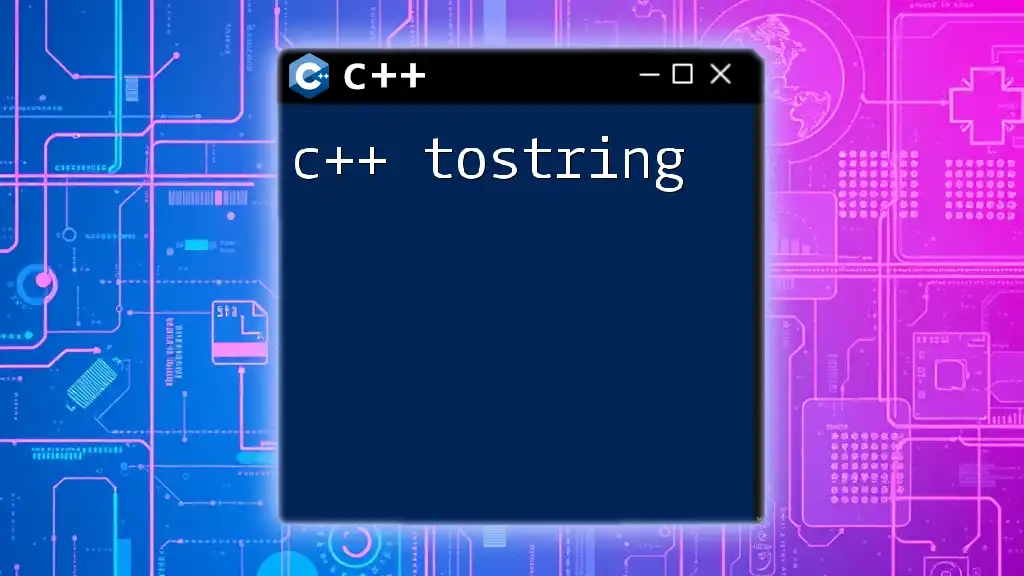
Handling Edge Cases in String Concatenation
Concatenating Empty Strings
When working with strings, it’s essential to understand how empty strings can influence your concatenation results.
For instance:
std::string str1 = "";
std::string str2 = "world!";
std::string result = str1 + str2; // result is "world!"
In this example, the empty string `str1` does not affect the outcome, demonstrating that concatenation handles empty inputs gracefully.
Concatenating Special Characters and Whitespace
Strings may sometimes contain special characters or whitespace, and understanding how to handle these cases can prevent unexpected issues.
Here’s an example:
std::string str = "C++";
std::string special = "@2023!";
std::string result = str + " " + special; // result is "C++ @2023!"
By incorporating whitespace, you can format your output neatly.
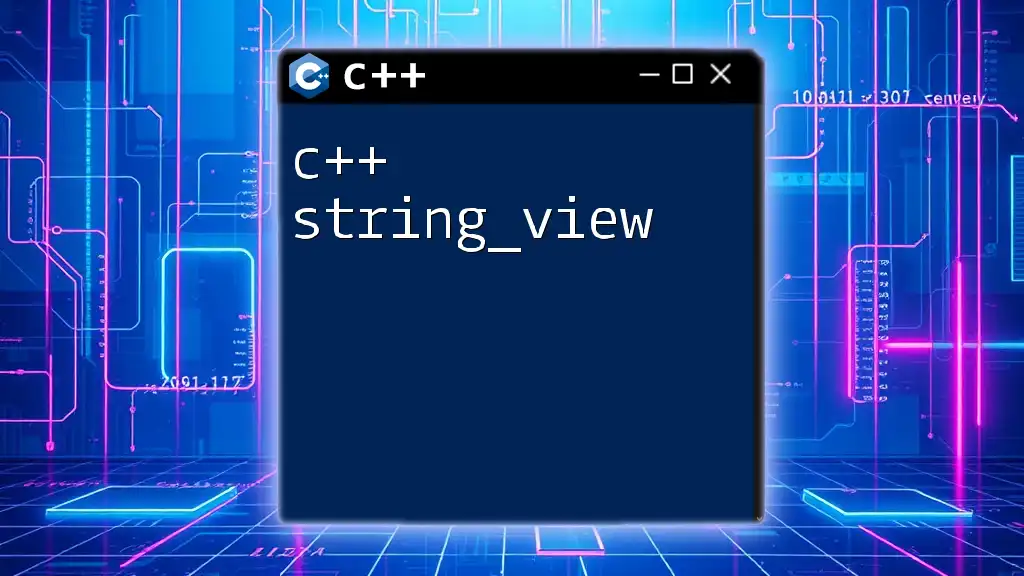
Performance Considerations in C++ String Concatenation
Time Complexity of Various Concatenation Methods
When you c++ add strings, it's crucial to consider the time complexity of different methods.
- Using the `+` operator can lead to O(n^2) complexity in scenarios involving repeated concatenation, as it may create a new string for each operation.
- The `append()` method usually operates in O(1) for adding to the end, making it preferable when concatenating multiple strings iteratively.
- Leveraging `std::ostringstream` is generally more efficient in terms of performance, especially when assembling larger strings.
Memory Management with Strings
Understanding memory allocation is vital as it directly impacts your program's efficiency. `std::string` automatically manages memory for you, but inadequate handling of string sizes during concatenation can lead to performance bottlenecks.
When concatenating strings, it’s wise to pre-allocate memory for your final result when you know the expected size. This minimizes the need for multiple memory re-allocations, enhancing performance.
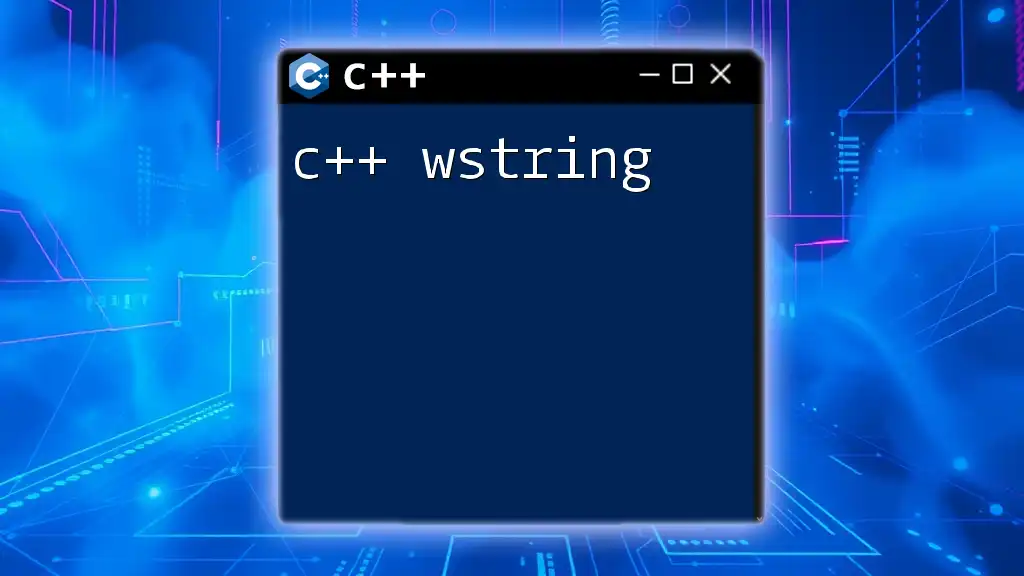
Conclusion
In summary, c++ add strings effectively requires an understanding of the tools and techniques available in the C++ language. Using `+`, `append()`, `+=`, and advanced solutions like `std::ostringstream` or `std::vector` opens up a world of possibilities for manipulating string data efficiently. By paying attention to potential edge cases and performance considerations, you can significantly improve your C++ applications.
Now is the time to practice these techniques with the various examples provided and see firsthand how string concatenation can enhance your development workflow.