C++ docstrings, typically used as comments or in documentation tools, help explain the purpose and usage of code elements such as functions or classes in a clear manner.
Here's an example of a C++ function with a docstring:
/**
* @brief Calculates the factorial of a number.
* @param n The number to calculate the factorial for.
* @return The factorial of the given number.
*/
int factorial(int n) {
if (n <= 1) return 1;
return n * factorial(n - 1);
}
What Are Docstrings?
C++ docstrings are special comments embedded within the source code that serve as documentation for functions, classes, methods, and modules. Unlike regular comments, which primarily serve to explain code to human readers, docstrings are designed to facilitate automated documentation generation and provide a clear and structured way of describing the function's purpose, parameters, return values, and more.
Why Use Docstrings in C++
Incorporating docstrings in your C++ code brings several notable benefits:
-
Enhanced Readability: With clear explanations embedded in the code, other developers (or even yourself in the future) can easily understand what a function does without digging through its implementation.
-
Maintainability: Well-defined docstrings help ensure that as functionality changes or grows, the documentation evolves alongside it, reducing the time and effort needed for future updates.

Understanding Docstrings in C++
The Basics of Docstrings
The primary distinction between docstrings and regular comments is structure. Docstrings follow a specific syntax that allows documentation tools to parse them effectively. They provide a consistent format for conveying information, making it easier to generate external documentation, such as manuals or online guides.
Where to Place Docstrings in Your Code
Placing docstrings correctly is crucial for maximizing their effectiveness. Typically, a docstring should be positioned immediately before the definition of a function, class, or complex logic. This strategic placement provides context right where it is needed.
When deciding between inline comments (short comments within code blocks) and block comments (longer explanations before a function or class), prefer using docstrings for larger entities, as they cater to comprehensive documentation and clarity.
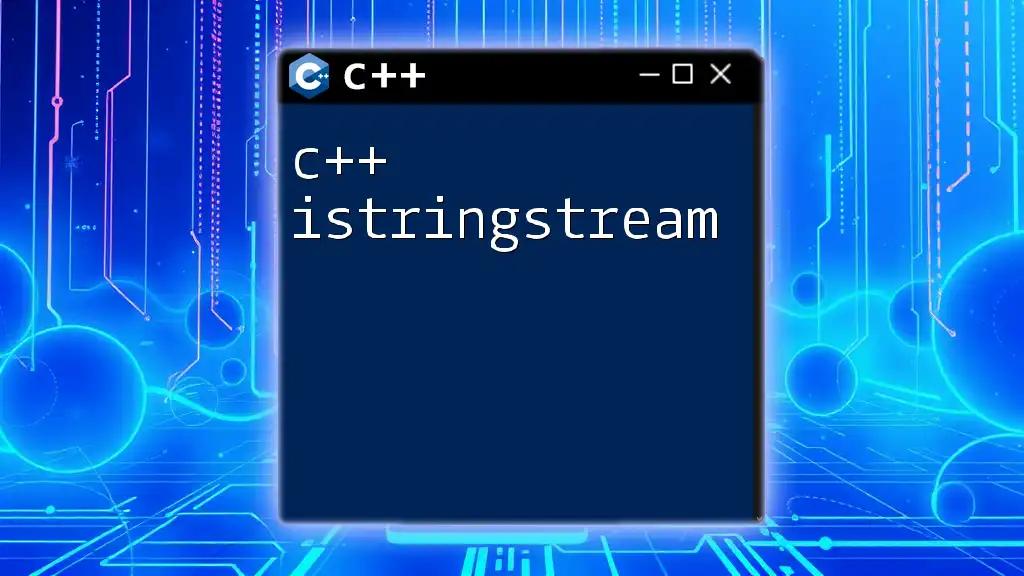
Creating Effective Docstrings
Elements of a Good Docstring
For docstrings to be effective, they should encompass several key elements:
-
Title of the Function or Class: A succinct title that immediately conveys what the function or class is about.
-
Description of Functionality: A brief explanation detailing what the function accomplishes. It should capture the intent without going into excessive detail.
-
Parameters: A clear statement about the input arguments, including their names and types, such as:
void calculateArea(double radius); // radius: The radius for which area is calculated.
-
Return Values: Describe what the function returns and its expected type. For example:
double calculateArea(double radius); // Returns the area of the circle.
-
Exceptions: Note any exceptions that the function might throw, ensuring that users understand potential pitfalls.
Example of a Well-Written Docstring
Here is an example of a well-structured docstring:
/**
* Calculates the factorial of a given integer.
*
* @param n The integer for which the factorial is to be calculated. Must be non-negative.
* @return Returns the factorial of the input integer n.
* @throw std::invalid_argument if n is negative.
*/
int factorial(int n) {
if (n < 0) {
throw std::invalid_argument("n must be non-negative");
}
// Implementation...
}
This example includes all essential elements, clearly outlining what the function does, the parameters it takes, the return value, and the exceptions it may throw.
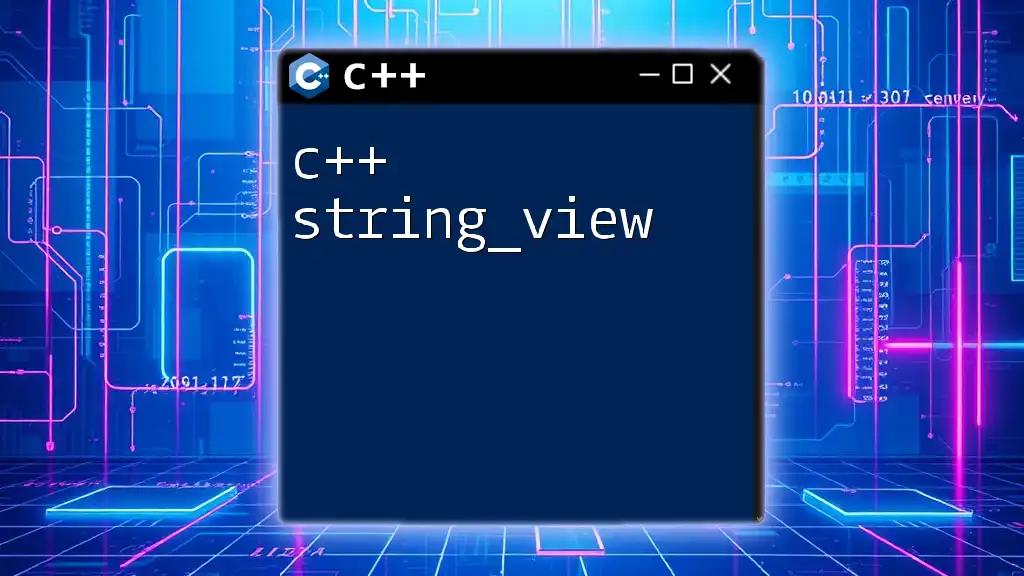
Tools for Generating and Using Docstrings
Popular C++ Documentation Generators
Several tools can help in generating structured documentation from your C++ docstrings, with Doxygen and Sphinx being the most widely used. These tools parse the docstrings in your code and create well-formatted documentation sites or manuals.
Integrating Docstring Generators into Your Workflow
Integrating documentation generators into your development setup is straightforward. For Doxygen, you typically need to configure a Doxyfile, which outlines the project's source files and documentation preferences.
Here’s a simple command to generate documentation with Doxygen:
doxygen Doxyfile
This command processes the specified files and generates documentation in your chosen format, such as HTML or LaTeX.

Best Practices for Writing Docstrings
Consistency in Style and Format
To maximize clarity, it’s essential to maintain a consistent style and format across your project. Adopting a standard documentation style, like that of Doxygen or Javadoc, provides a uniform method of presenting function descriptions, making it easier for others to follow.
Clarity and Conciseness
When writing docstrings, aim for clarity and conciseness. Avoid using overly complex terminology that could confuse readers. Documentation should be accessible and straightforward, showcasing the functionality clearly.
Keeping Docstrings Updated
An essential practice is to keep your docstrings updated as the code evolves. Outdated documentation can lead to discrepancies between the implementation and its explanation, which can confuse users and other developers. A regular auditing process can help maintain accurate documentation.
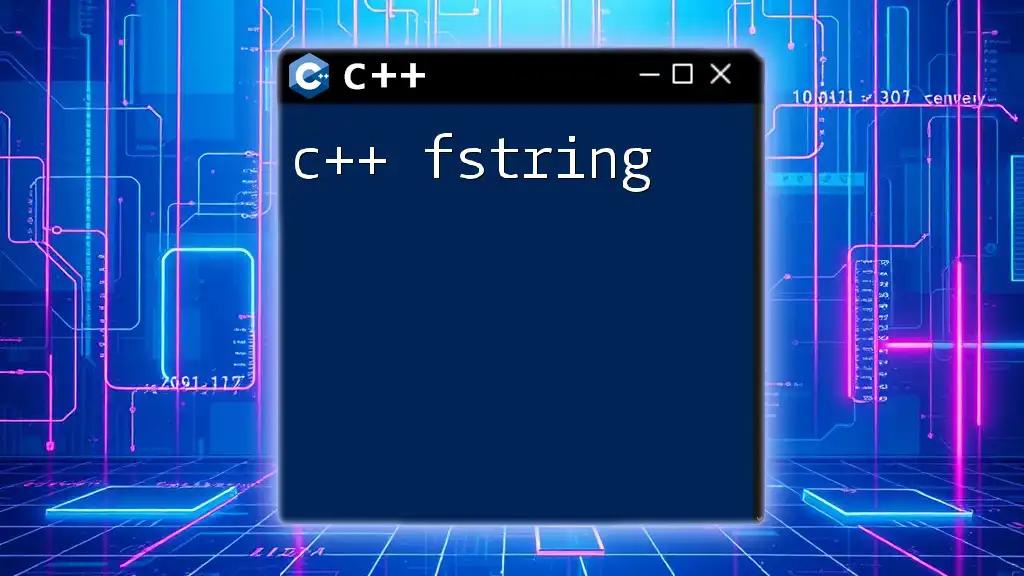
Advanced Usage of Docstrings
Use Cases for Docstrings in Large Projects
In large codebases, well-crafted docstrings play a crucial role in ensuring efficient collaboration among teams. They provide context and understanding, allowing new developers to get up to speed quickly and reducing the learning curve associated with complex systems.
Automating Documentation Creation
Consider leveraging automation tools and scripts to generate or update docstring documentation. For instance, some projects use continuous integration (CI) tools to automatically parse and check for accurate comments. By establishing a routine documentation update, you can ensure that your docstrings are consistently up-to-date.
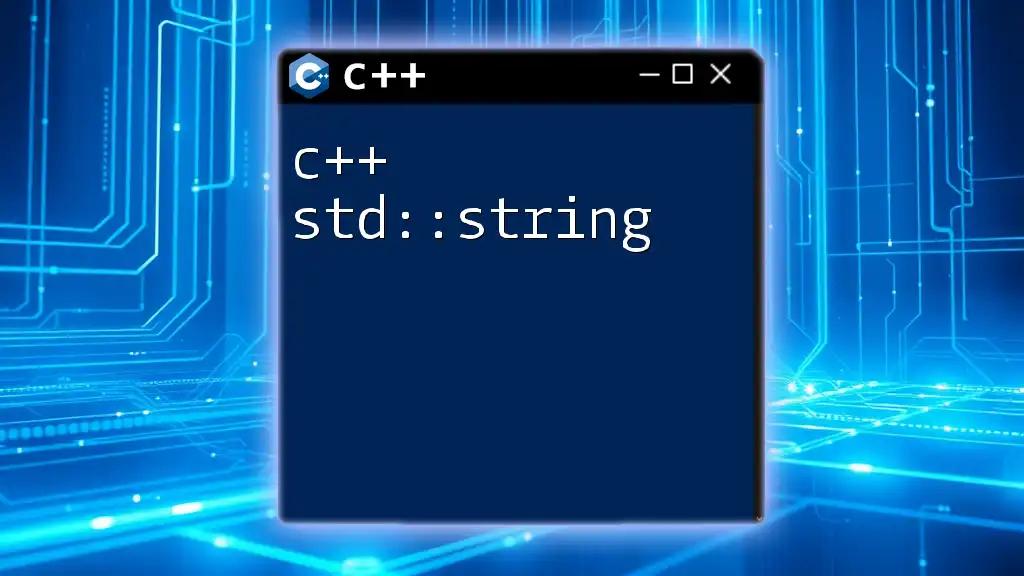
Common Pitfalls and How to Avoid Them
Mistakes in Writing Docstrings
Several common pitfalls can undermine the effectiveness of docstrings. Overly complex wording or jargon can alienate readers. Additionally, neglecting to include critical arguments or return types leads to misunderstandings about a function’s use.
Ignoring Docstring Maintenance
Failing to update docstrings leads to inaccurate documentation. This negligence can be detrimental, especially when multiple developers utilize the codebase. Establishing a routine for reviewing and updating documentation as part of your development cycle helps maintain accuracy.
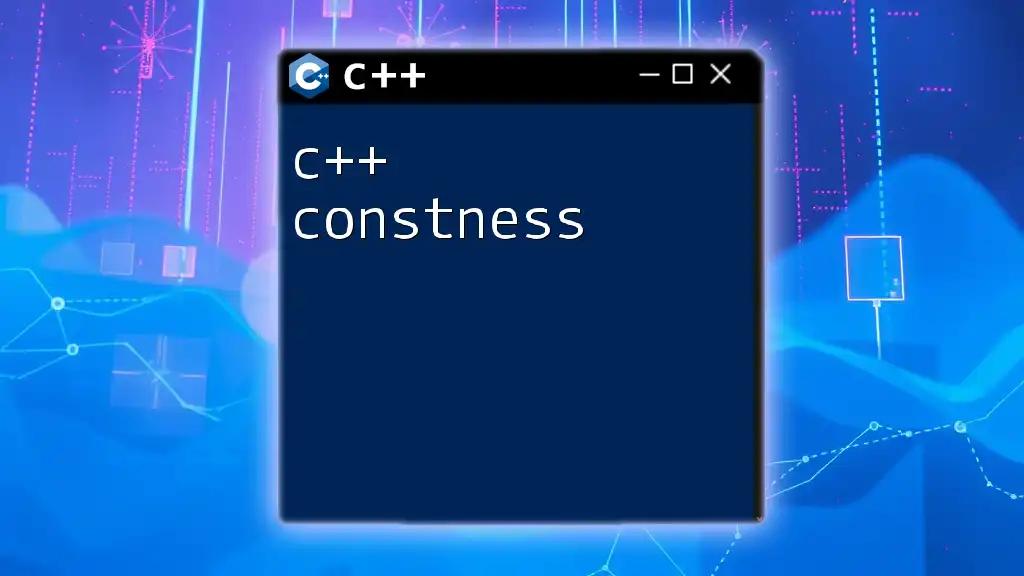
Conclusion
Incorporating C++ docstrings into your development process significantly enhances code readability and maintainability. By clearly documenting your functions and classes, you support collaborative efforts and simplify future development tasks. Embracing the practice of writing consistent and effective docstrings will ultimately benefit both you and the wider programming community.

Further Reading and Resources
To deepen your understanding of C++ documentation practices, consider exploring literature that emphasizes the importance of documentation. Engaging with community forums where developers discuss their experiences and share tools can also offer valuable insights.