In C++, the term "redistribute" often refers to the process of redistributing the elements of a container or array, which can be done using algorithms from the Standard Library, such as `std::rotate` to change the order of elements.
Here's a code snippet that demonstrates how to use `std::rotate` to redistribute elements in a vector:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
std::rotate(vec.begin(), vec.begin() + 2, vec.end()); // Rotate elements
for (int num : vec) {
std::cout << num << " "; // Output: 3 4 5 1 2
}
return 0;
}
What are C++ Redistributables?
C++ redistributables are essential software components that package together the libraries and runtime files required by applications developed in C++. These redistributables ensure that end users can run C++ applications without needing the entire development environment installed on their machines. Understanding these components is crucial for anyone involved in C++ software development.
Redistributables primarily handle dependency management, allowing developers to package their applications without bundling the entire Visual Studio environment. When a C++ application is compiled, it often relies on specific libraries and runtime features. Redistributables serve as a bridge, enabling the application to call upon these libraries, which are otherwise not present on the user's machine.
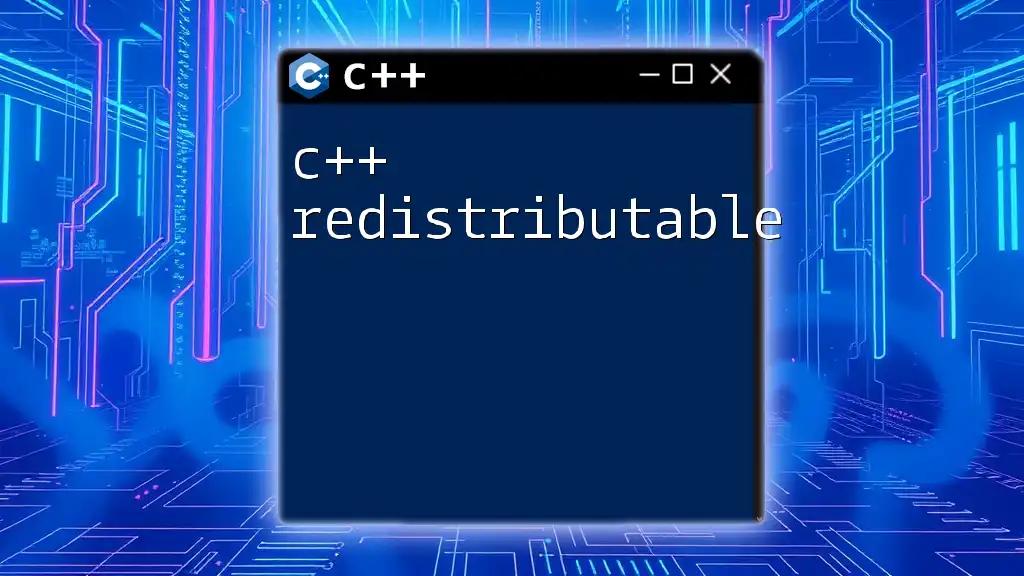
Visual C++ Redistributables
Overview of Visual C++
Microsoft Visual C++ is a widely used integrated development environment (IDE) for C++ applications, providing powerful tools and libraries. It enables developers to create applications for Windows platforms efficiently. However, applications compiled in Visual C++ require specific runtime libraries to function correctly on end-user systems.
What are Visual C++ Redistributable Packages?
Visual C++ Redistributable Packages are a collection of runtime components that allow applications built with Visual C++ to run on a machine where the full Visual Studio environment is not installed. These packages contain essential runtime libraries, including C Runtime (CRT), Standard C++ Library, and others, which are necessary for the execution of C++ applications. Without these packages, users may encounter errors such as "The program can't start because msvcp140.dll is missing from your computer."
Key Features of Visual C++ Redistributables
Understanding the key features can greatly enhance the effectiveness of managing dependencies:
-
Runtime Libraries Provided: Visual C++ Redistributables include various components for applications built with different versions of Visual Studio. For example, Visual C++ 2015 Redistributable is crucial for applications that use the libraries from the 2015 version of Visual Studio.
-
Version Compatibility: Developers can target specific versions of redistributables, making it easy to maintain control over application behavior concerning certain library versions.
-
Platform Support: Visual C++ Redistributables support both x86 and x64 architectures, providing flexibility when deploying applications on different system configurations.

Installing Visual C++ Redistributables
How to Download and Install
To ensure your application runs smoothly on target machines, you'll need to download the correct Visual C++ Redistributables. Follow these steps:
- Visit the Official Microsoft Download Page: Navigate to the Visual C++ Redistributable download section on Microsoft's official website.
- Choose the Correct Version: Select the version corresponding to your application's requirements (e.g., Visual C++ 2015, 2017, 2019).
- Download the Installer: Click on the download link for the x86 or x64 version, depending on your application's architecture.
- Run the Installer: Follow the prompts in the installation wizard to complete the installation.
Common Installation Issues
While installing redistributables is generally straightforward, users might encounter some common issues:
-
Error Messages: Some users may see messages indicating that they do not have permission to install the redistributable. This usually requires running the installer as an administrator.
-
Missing Dependencies: If the installer reports missing dependencies or conflicts, ensure that no prior versions of the redistributable are active. Uninstall any old versions before proceeding.

Using Visual C++ Redistributables in Your Projects
Linking with Visual C++ Redistributables
To make sure your C++ application utilizes the redistributables correctly, proper linking is essential. Here’s how to configure your development environment:
- Open Your Project in Visual Studio.
- Navigate to Project Properties: Right-click your project in the Solution Explorer and choose "Properties."
- Set C/C++ Dependencies: Under "Configuration Properties," ensure the appropriate Included Directories point to the redistributables.
Here’s an example of how a simple C++ program might look:
#include <iostream>
#include <vector>
// Using Standard Template Library (STL) requires Redistributable
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
std::cout << num << std::endl;
}
return 0;
}
Packaging Your C++ Application
When deploying your application, consider bundling the redistributables required by your software. Here are some best practices:
- Incorporate the right packages: Ensure that you include the correct version of the redistributables that your application depends on.
- Automate the installation process: Create a script or installer that checks for existing redistributables and installs them if absent. Tools like Inno Setup or NSIS can assist with this.
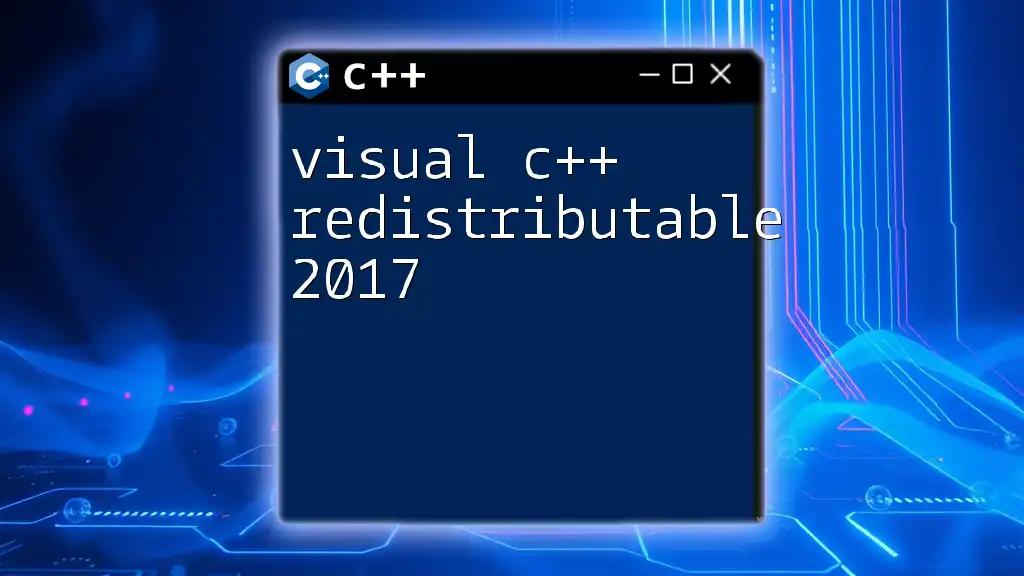
Verifying Redistributable Installation
Confirming Installation on a Windows System
To ensure that the Visual C++ Redistributables are correctly installed on a Windows system, do the following:
-
Control Panel: Go to `Control Panel > Programs > Programs and Features`. Look for the Visual C++ Redistributable entries in the list to confirm installation.
-
Command-Line Options: Use PowerShell or Command Prompt to check installed packages. You can run:
wmic product get name
Ensuring Correct Version Usage
To confirm that your application is using the correct version of the redistributable, consider using the Dependency Walker tool. This utility enables you to check the dependencies of your executable and see whether the correct version of the redistributables is being utilized.

Alternatives to Visual C++ Redistributables
Other C++ Libraries and Distributions
While Visual C++ Redistributables are integral, there are alternatives developers may consider, especially for cross-platform applications. Libraries such as Qt or Boost provide robust functionalities and can sometimes serve as substitutes.
Comparing Visual C++ Redistributables with Other Options
When choosing libraries, it’s important to weigh the benefits and drawbacks:
-
Pros: Visual C++ Redistributables are highly compatible with Windows applications, more straightforward for Windows-only development, and provide extensive support.
-
Cons: For cross-platform projects, relying solely on Visual C++ Redistributables might not be ideal, as they bind your application to the Windows runtime environment.
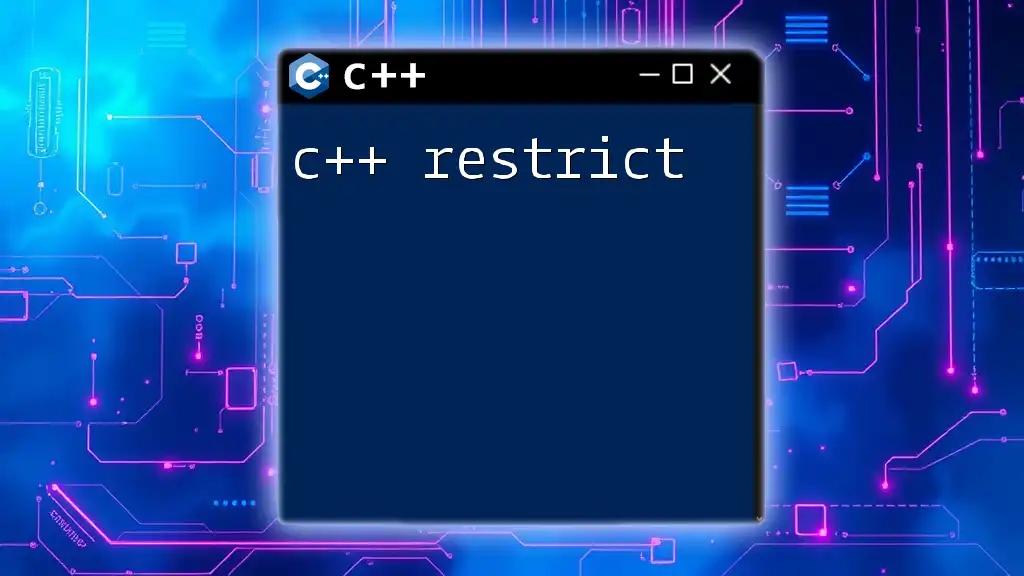
Conclusion
Understanding and properly managing C++ redistributables is essential for any developer aiming to ensure that their applications run seamlessly on users' systems. As highlighted, Visual C++ Redistributables play a pivotal role in linking necessary libraries and ensuring compatibility. By following the guidelines outlined in this article, developers can enhance the reliability and user experience of their C++ applications.

Additional Resources
- Official Microsoft Documentation: Essential for the latest updates on redistributables and troubleshooting.
- Further Reading: Explore articles on C++ libraries, development practices, and dependency management.
- Tools and Utilities: Familiarize yourself with utilities that can aid in C++ project management, such as Dependency Walker and packaging tools.