In C++, an `enum` is a user-defined type that consists of a set of named integral constants, and you can convert enums to strings using a function for better readability and debugging.
Here's a simple example:
#include <iostream>
#include <string>
enum Color { Red, Green, Blue };
std::string colorToString(Color color) {
switch (color) {
case Red: return "Red";
case Green: return "Green";
case Blue: return "Blue";
default: return "Unknown Color";
}
}
int main() {
Color myColor = Green;
std::cout << "The color is: " << colorToString(myColor) << std::endl;
return 0;
}
Understanding Enums in C++
What is an Enum?
An enum (short for enumeration) in C++ is a user-defined type that consists of a set of named integer constants. It allows developers to represent a set of related values in a more readable and organized manner compared to using plain integers. This leads to better code clarity and type safety, reducing the likelihood of errors caused by invalid values.
Basic Syntax of Enums
Declaring an enum is straightforward. You define it using the `enum` keyword followed by the name of the enumeration and its possible values enclosed in curly braces. Here is a simple example:
enum Color {
RED,
GREEN,
BLUE
};
In this example, `Color` is the enum type, and it can take on the values `RED`, `GREEN`, or `BLUE`. By default, the values start from 0 and increment by 1, so `RED` is 0, `GREEN` is 1, and `BLUE` is 2.
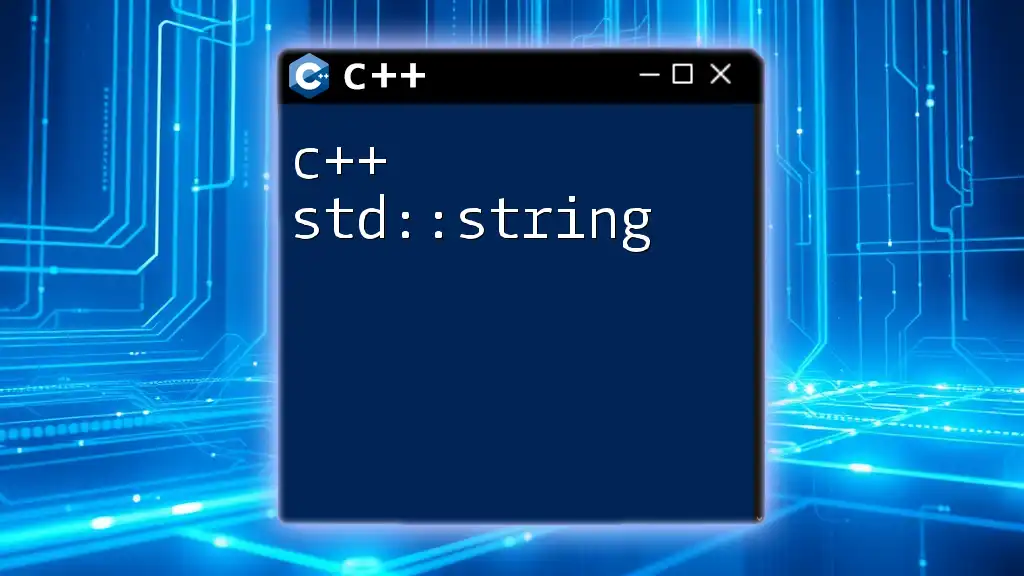
C++ Enum to String Conversion
Why Convert Enums to Strings?
Converting enums to strings can be incredibly useful for debugging and logging purposes. When you print an enum's value, it might not be readily understood; however, converting it to a string provides a more user-friendly display. This can enhance the clarity of output, especially in graphical user interfaces (GUIs) or logs.
Simple Enum to String Function
A basic way to convert an enum to its string representation is by using a function with a switch case. Here is how you can implement it:
const char* enumToString(Color color) {
switch (color) {
case RED: return "Red";
case GREEN: return "Green";
case BLUE: return "Blue";
default: return "Unknown Color";
}
}
In this function, when you pass a `Color` value, the function uses a switch case to return its corresponding string name. If an unknown value is passed, it returns "Unknown Color". This straightforward method is easy to understand and implement.
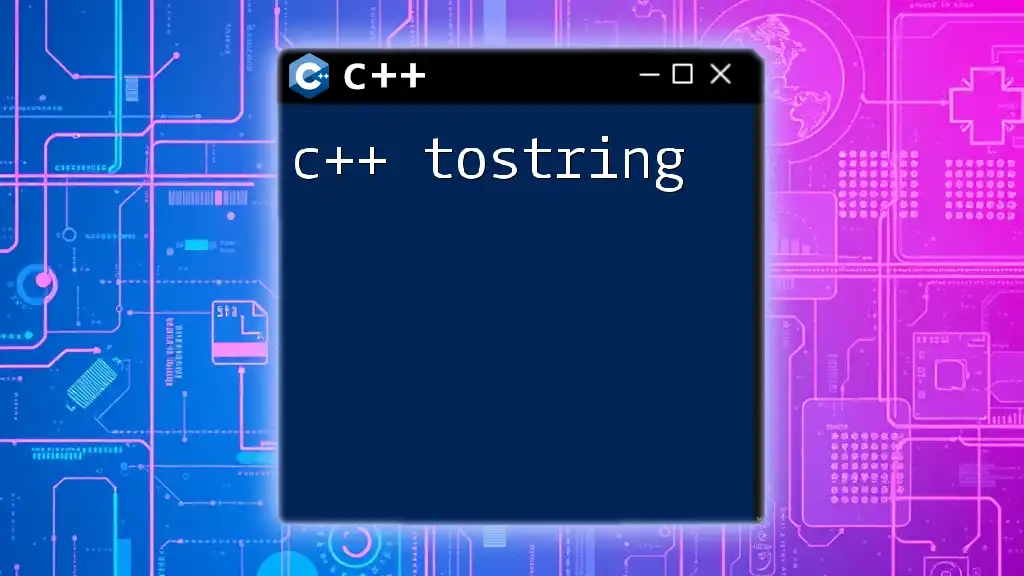
Advanced Enum to String Techniques
Using `std::map` for Enum-to-String Mapping
For larger enums or when you want more scalability, using a `std::map` can be a more elegant solution. This approach decouples enum values from their string counterparts, making it easy to modify, add, or remove mappings. Here’s how you can do it:
#include <map>
const std::map<Color, std::string> colorToString = {
{RED, "Red"},
{GREEN, "Green"},
{BLUE, "Blue"}
};
std::string enumToString(Color color) {
auto it = colorToString.find(color);
return (it != colorToString.end()) ? it->second : "Unknown Color";
}
In this code snippet, a `std::map` is used to map each enum value to its corresponding string. The `enumToString` function then simply looks up the value and returns the associated string. This method is more maintainable, especially when dealing with larger enums.
Utilizing C++17 Features for Enums
C++17 introduced several features that can enhance how we handle enums and string conversion. One significant addition is `std::optional`, which allows for safer handling of values that might not exist.
Here’s an implementation that uses `std::optional`:
#include <optional>
std::optional<std::string> enumToString(Color color) {
switch (color) {
case RED: return "Red";
case GREEN: return "Green";
case BLUE: return "Blue";
default: return std::nullopt; // Or use an error message
}
}
In this case, if the color is unknown, the function returns `std::nullopt`, indicating an absence of a value. Using `std::optional` improves the robustness of your code by explicitly handling cases where the provided enum does not match any expected values.
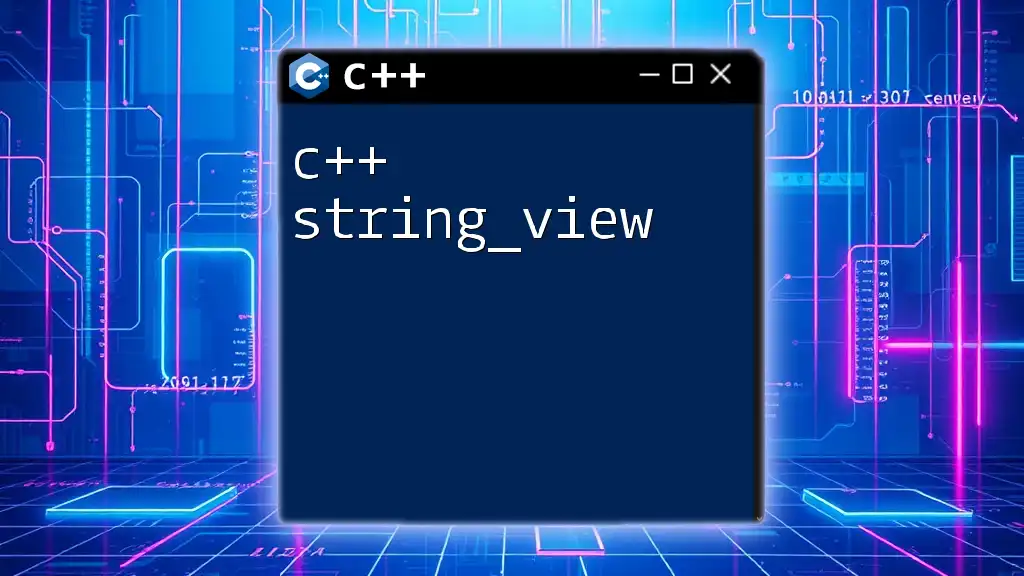
Best Practices for C++ Enum String Usage
Consistency in Enum Declaration
When working with enums, it is crucial to maintain consistent naming conventions and organization in your codebase. This might involve naming all enum values in uppercase or using a prefix indicating the type, such as `COLOR_RED`, `COLOR_GREEN`, etc.
Documentation for Enums
Thorough documentation for your enums is essential. It not only aids in understanding but makes it easier to maintain the code over time. Clearly document what each enum value represents, especially if they are part of a larger system. This would help new developers quickly comprehend the structure and usage.
Using Enum String Conversion in Real-world Scenarios
Enum-to-string conversions find their applications in various real-world scenarios, particularly in logging and error handling. Here’s a simple output example that displays the current color:
Color currentColor = RED;
std::cout << "Current Color: " << enumToString(currentColor) << std::endl;
This displays: `Current Color: Red`. Such clarity in output can greatly facilitate debugging processes and enhance user experience.
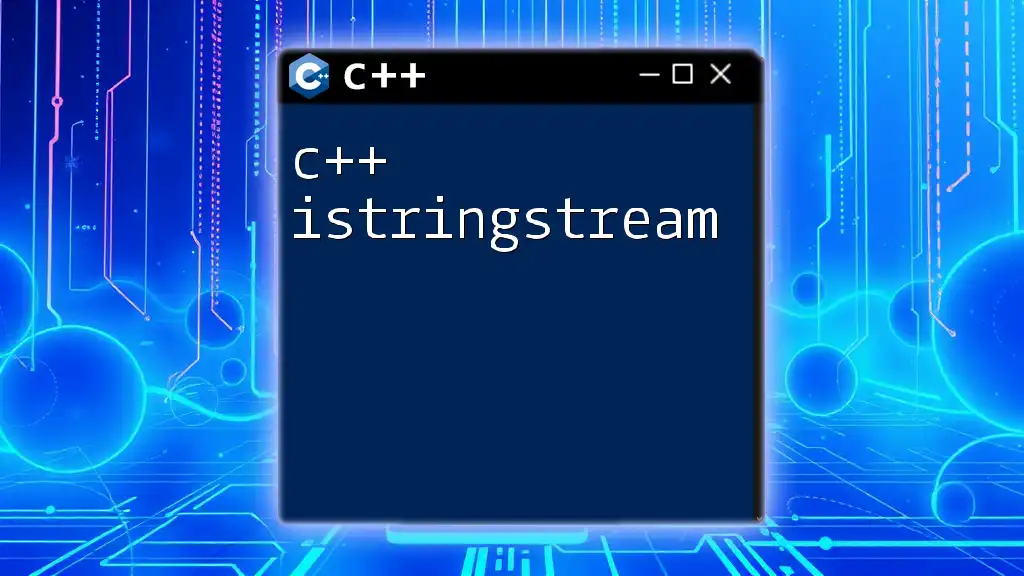
Conclusion
In this guide, we've walked through the concept of C++ enums and various techniques for converting them to their string representations. From basic switch-case methods to more advanced mappings and C++17 enhancements, understanding these concepts is crucial for writing clean, efficient, and maintainable C++ code. By embracing these practices, you can significantly improve the readability and manageability of your codebase.