In C++, an enum can be defined within a class to group related constants, improving code readability and maintainability.
class Color {
public:
enum ColorEnum { RED, GREEN, BLUE };
};
Color::ColorEnum myColor = Color::RED;
Understanding Enums in C++
What is an Enum?
An enum (short for enumeration) is a user-defined data type in C++ that consists of a set of named integral constants. By grouping related constants under a single name, enums enhance code readability and maintainability. An enum is declared using the `enum` keyword followed by the name of the enumeration and a list of its possible values.
For instance, consider the basic syntax for declaring an enum:
enum Color {
RED,
GREEN,
BLUE
};
In this example, `Color` is an enumeration type that can take the values RED, GREEN, or BLUE.
Why Use Enums?
Using enums in your code comes with several advantages:
- Improve Code Clarity: Enums make your code easier to understand. Instead of using arbitrary numbers, you can refer to meaningful names that represent the values.
- Type Safety: Enums are type safe, meaning they provide a distinct type that helps avoid errors involving mismatched values.
- Easier Maintenance: When changes are required, you only need to adjust the enum definition rather than finding and replacing multiple occurrences in the code.
Enums also present a clearer alternative to `#define` constants or plain integer values. While `#define` constants are preprocessor directives and lack type safety, enums are scoped and type-checked by the compiler.
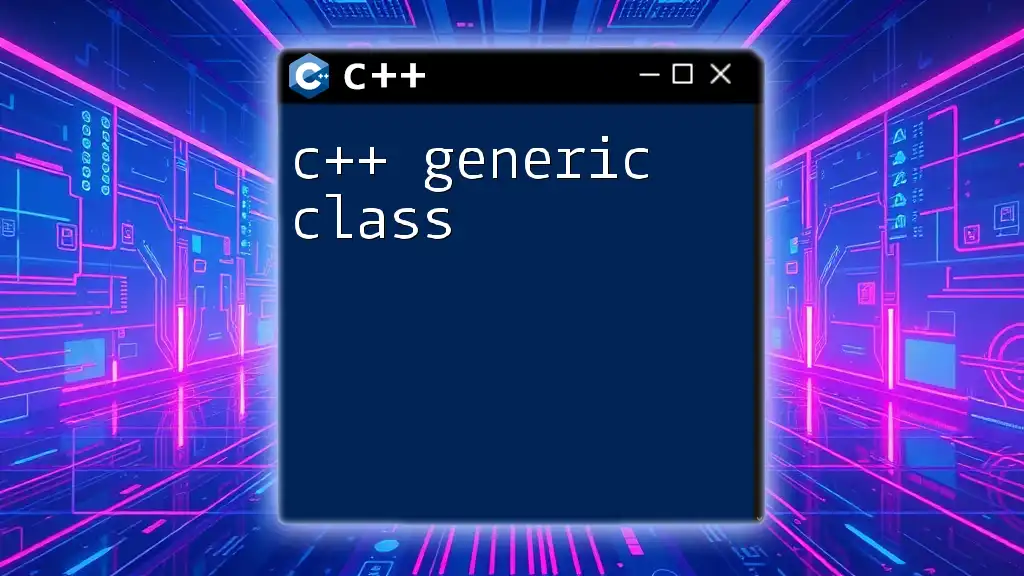
Declaring Enums Within a Class
Syntax of Enums in a Class
When declaring an enum within a class, the syntax is straightforward. Here’s an example:
class Vehicle {
public:
enum Type {
CAR,
TRUCK,
MOTORCYCLE
};
};
In this case, `Type` is an enumeration inside the `Vehicle` class, providing well-defined options for the vehicle type.
Accessing Enum Values
Accessing enum values in a class involves using the class name followed by the scope resolution operator `::`. For example:
Vehicle::Type myVehicle = Vehicle::CAR;
This line sets `myVehicle` to the CAR value defined within the `Vehicle` class.
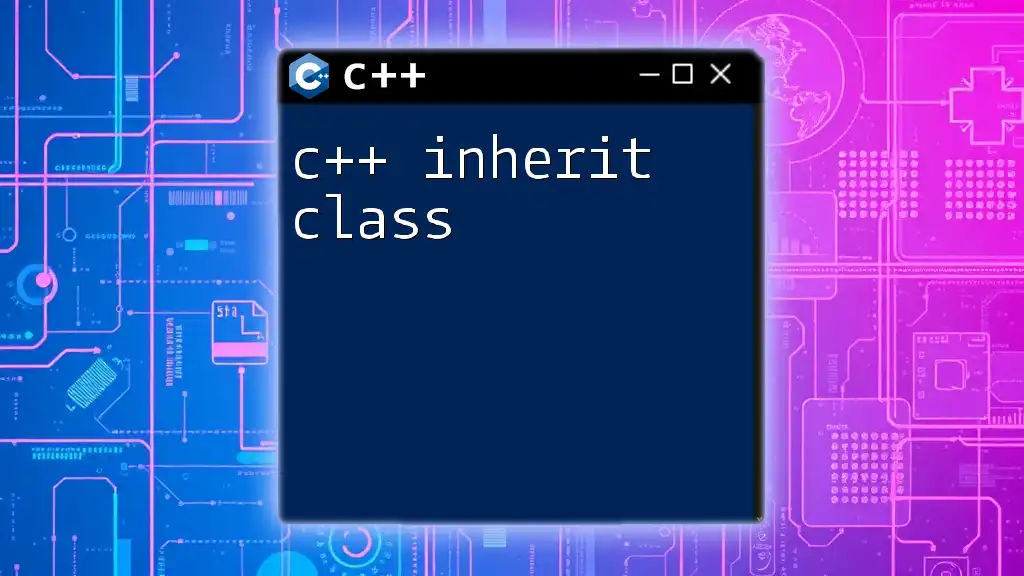
Practical Examples of Enums in Classes
Example 1: State Management
Enums are particularly useful in managing the state of an object. Consider a simple `LightBulb` class where an enum is used to define possible states:
class LightBulb {
public:
enum State {
ON,
OFF,
DIMMED
};
State currentState;
void switchOn() {
currentState = ON;
}
void switchOff() {
currentState = OFF;
}
void dim() {
currentState = DIMMED;
}
};
In this example, `State` allows for clear and concise management of a light bulb's status, making the code easily understandable.
Example 2: Traffic Light System
Another illustrative example is a traffic light system where states can easily be defined using an enum:
class TrafficLight {
public:
enum Color {
RED,
YELLOW,
GREEN
};
Color currentColor;
void changeColor(Color newColor) {
currentColor = newColor;
}
};
In this `TrafficLight` class, the `Color` enum represents the possible colors of the traffic light, demonstrating how enums can help maintain clarity in state management.
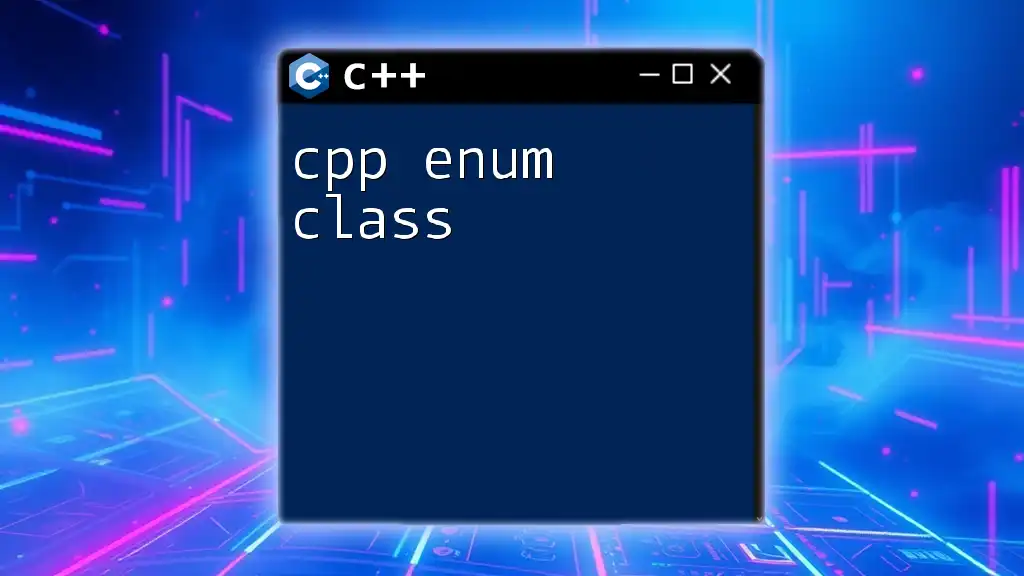
Best Practices for Using Enums in Classes
Keep Enums Scoped
Keeping enums scoped within classes is crucial to avoid naming conflicts and maintain organization. Scoped enums help to encapsulate constants and make the code more readable, ensuring that enum values don't clash with those from other classes or namespaces.
Use Strongly Typed Enums (C++11 and later)
With C++11, you can utilize strongly typed enums (also called `enum class`), which offer better type safety compared to traditional enums. Here's an example:
class Game {
public:
enum class State {
START,
PAUSE,
GAME_OVER
};
State currentState;
};
Using `enum class` avoids implicit conversions and keeps the enum members isolated from the global namespace. This way, you must prefix enum values with their enum type, enhancing clarity.
Consider Using Namespaces
In cases where you have multiple enums that could overlap, using namespaces can be a prudent choice. By encapsulating enums within a namespace, you can differentiate them clearly:
namespace VehicleType {
enum Type {
CAR,
TRUCK
};
}
namespace AnimalType {
enum Type {
DOG,
CAT
};
}
Here, the `VehicleType` and `AnimalType` namespaces prevent any conflicts and keep the code organized.
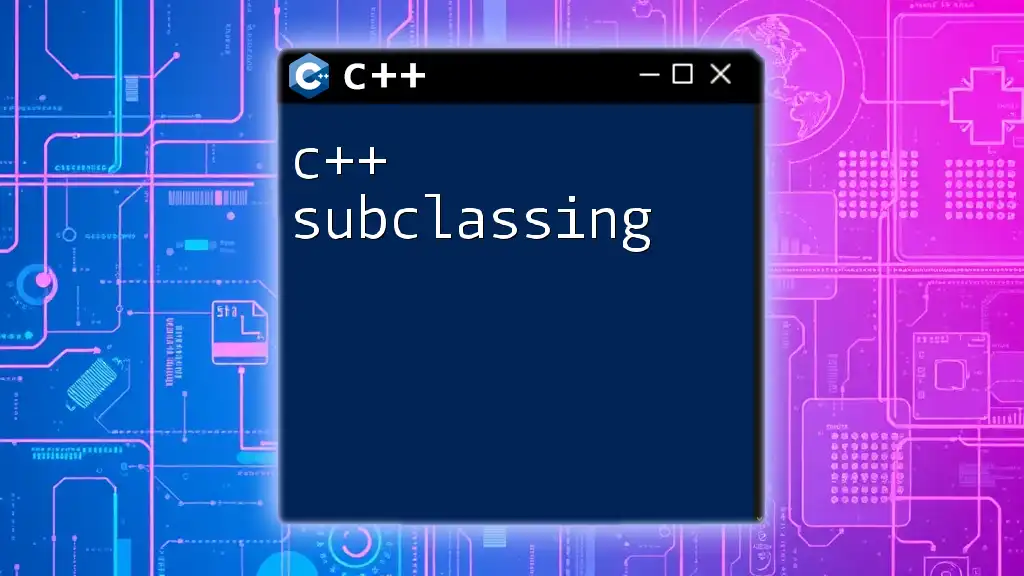
Common Mistakes to Avoid
Forgetting to Initialize Enum Variables
One common mistake is neglecting to initialize enum variables. If an enum variable is not set to one of its valid states, it could lead to undefined behavior. For example:
LightBulb bulb; // Uninitialized
if (bulb.currentState == LightBulb::ON) {
// This may yield unexpected results
}
Always ensure that enum variables have a defined state before usage.
Overusing Enums for Everything
While enums are powerful, relying on them for every scenario isn't advisable. Enums work best for a fixed set of values. For instance, using enums to represent values that should change frequently or dynamically can lead to code that becomes difficult to manage. In such cases, consider using other data structures, such as classes or structs.
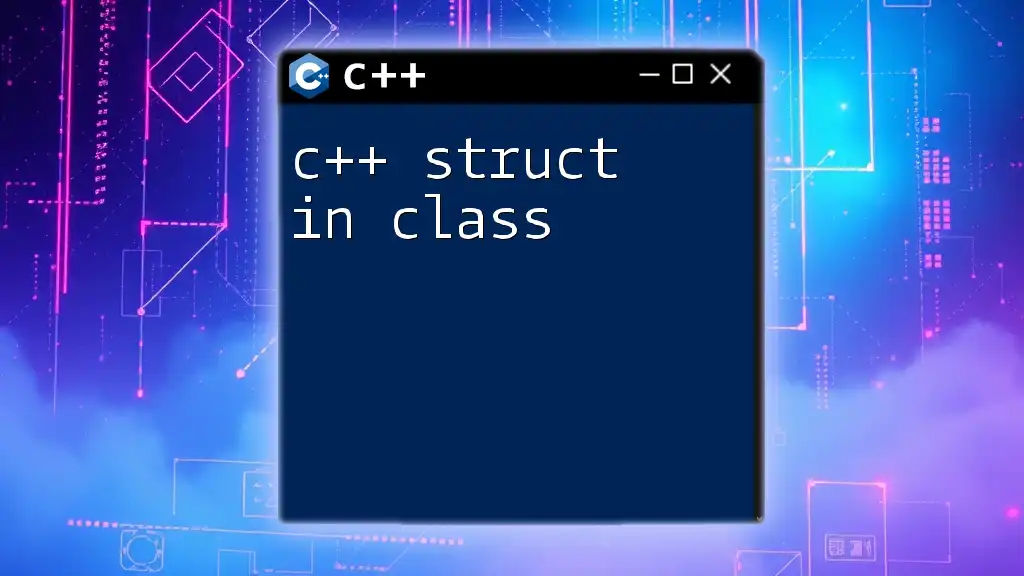
Conclusion
Incorporating C++ enums in classes elevates your coding practices by promoting clarity, safety, and maintainability. By using enums smartly within your classes, you not only improve the readability of your code but also make it easier for others to collaborate and understand your intentions.
Experiment with the code snippets provided, and get creative with your own implementations of enums in classes. The versatility and power of enums will undoubtedly enhance your C++ projects.