C++ compilers translate C++ code into machine code so that it can be executed by a computer, allowing developers to run their programs efficiently.
Here’s a simple example demonstrating a C++ program that outputs "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is a C++ Compiler?
A compiler is a specialized program that translates source code written in a programming language into machine code, bytecode, or another programming language. In the context of C++, a compiler transforms your human-readable C++ code into an executable program that can run on your machine.
Compilers vs Interpreters
The distinction between compilers and interpreters is crucial in software development. While a compiler translates the entire code and generates an executable file, an interpreter translates code line by line during execution. This fundamental difference affects performance, error handling, and usage contexts. For instance, C++ compilers are preferred for performance-heavy applications due to their efficiency in generating optimized binaries, making them ideal for production environments.

Types of C++ Compilers
C++ Compiler Categories
Native Compilers produce code that runs natively on the operating system for which they are compiled. This leads to faster execution, which is ideal for performance-critical applications.
Cross-Compilers allow developers to compile code on one platform, targeting another. This is useful when developing applications for embedded systems or any scenario where the development environment differs from the deployment environment.
Just-In-Time (JIT) Compilers compile code dynamically at runtime, which can optimize performance based on the current execution context. While JIT compilers are more common in languages like Java, certain C++ implementations may benefit from similar techniques.
Popular C++ Compilers
GCC (GNU Compiler Collection) is one of the most widely used C++ compilers. It supports several programming languages and is available on multiple platforms. To get started with GCC, you can use the following command to compile a simple program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
After saving this code in a file called `hello.cpp`, you can compile it using:
g++ hello.cpp -o hello
Clang is another popular compiler recognized for its fast compilation speed and excellent diagnostics. It's particularly favored in macOS development. Like GCC, you can compile programs easily, for instance:
clang++ hello.cpp -o hello
Microsoft Visual C++ (MSVC) is the primary compiler for Windows developers, bundled with Microsoft Visual Studio. It offers integrated development environment (IDE) support, which streamlines the development process, allowing for quick testing and debugging.
When choosing a compiler, consider the strengths and weaknesses of each. GCC is known for portability, Clang for performance and readability of error messages, and MSVC for its integration with Windows development tools.
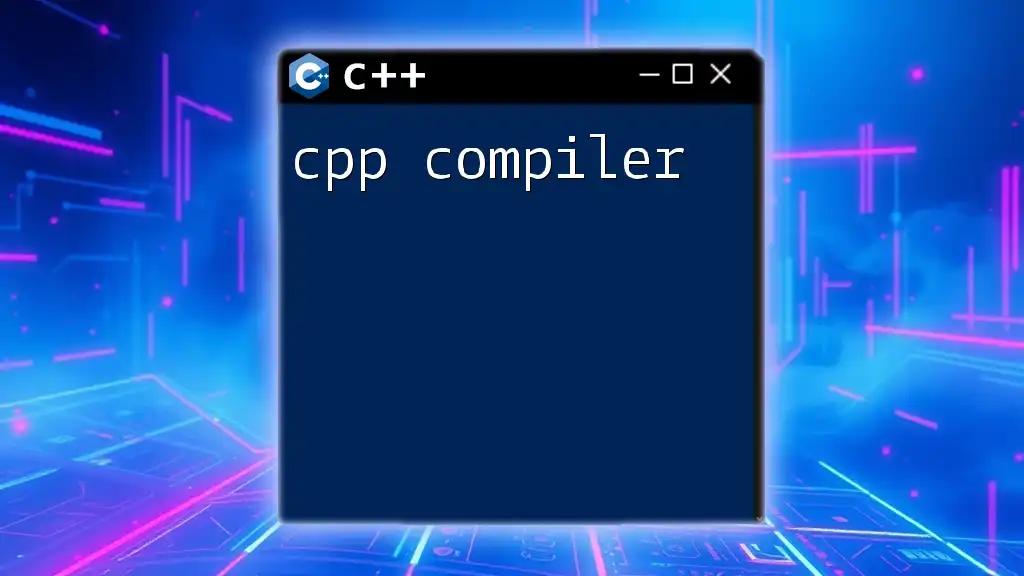
How to Choose the Right C++ Compiler
Factors to Consider
Operating System Compatibility is a primary factor. Ensure that the compiler you intend to use supports your preferred OS—GCC for Linux, Clang for macOS, and MSVC for Windows.
Project Requirements can significantly influence the choice of a compiler. For instance, you might need specific optimizations or features that are only available in certain compilers.
Community and Support are vital. A strong community can provide resources, tutorials, and help when you encounter issues. Selecting a well-supported compiler can make your development process smoother.
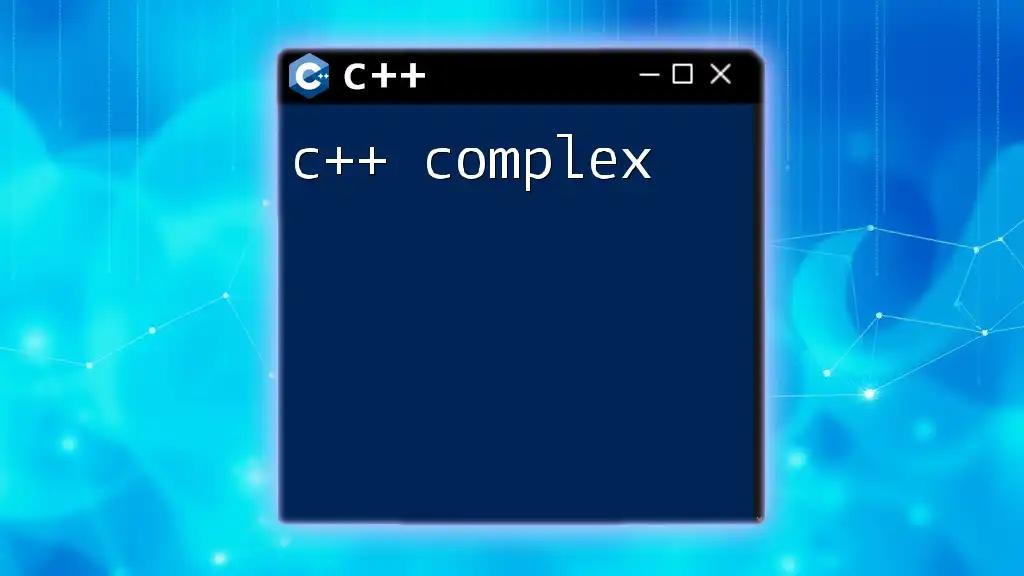
Setting Up a C++ Compiler
Installation Guide for GCC
To install GCC on Ubuntu-based systems, use:
sudo apt install g++
On macOS, it can be installed via Homebrew:
brew install gcc
Once installed, you can verify it with:
g++ --version
Setting Up Clang
To install Clang on macOS, it usually comes pre-installed with Xcode. For Linux, you can install it via package managers. For Ubuntu:
sudo apt install clang
You can test the installation with:
clang++ --version
Using MSVC
To set up MSVC, download and install Visual Studio from the Microsoft website, choosing the "Desktop development with C++" workload. Once installed, you can create a new C++ project, write your code, and directly compile and run it via the IDE interface.

Compiling C++ Code: Step-by-Step Process
Writing a Simple C++ Program
Here’s a simple program demonstrating the essentials of C++ syntax:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, C++ Compilers!" << endl;
return 0;
}
Compilation Process Explained
The compilation process in C++ occurs in several stages:
Preprocessing is the first step where the preprocessor directives (`#include`, `#define`) are handled. For example, the `#include <iostream>` directive fetches the declarations from the iostream library necessary for input and output functionalities.
Compilation follows, converting the preprocessed code into object code—an intermediate representation of the source code. This phase checks for syntax errors and generates error messages if present.
Linking is the final stage where the object code is linked with libraries required for functions not defined in the user code, ultimately producing an executable file.
Running the Compiled Program
To run your compiled program, simply execute the generated binary. For instance, on Linux/macOS:
./hello
On Windows, just type:
hello.exe

Common Compiler Errors and Warnings
Understanding Compiler Output
Compiler outputs consist of errors and warnings.
Syntax Errors are the most common, arising from incorrect code structure. For example, forgetting a semicolon at the end of a line will trigger an error that indicates a missing declaration.
Linker Errors occur when the compiler cannot find the definitions for declared functions or variables. This often happens when not including necessary libraries.
Warning Messages are non-critical issues that may not stop the compilation but indicate potential problems. Addressing warnings improves code quality.
Using Compiler Flags
Utilizing compiler flags can help improve code quality and highlight errors. Some useful flags include:
- `-Wall`: Enables warnings about all constructs that are not strictly standard.
- `-O2`: Applies optimized settings to improve performance.
You can add these flags when compiling your code like so:
g++ -Wall -O2 hello.cpp -o hello
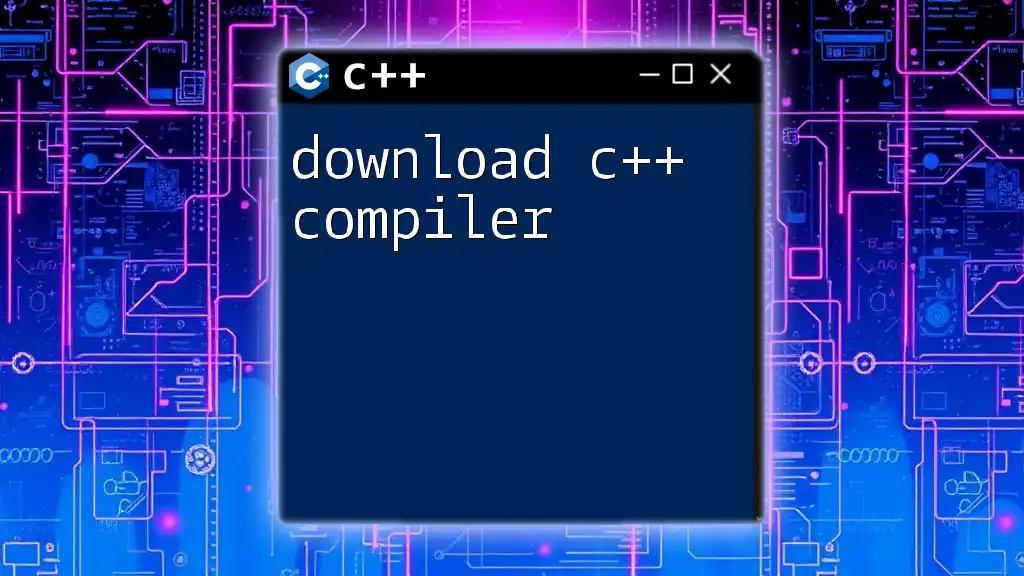
Advanced Topics in C++ Compiling
Compiler Optimization Techniques
C++ compilers offer various optimization levels that can significantly impact performance:
- Level 0: No optimization
- Level 1: Basic optimizations that don't change program behavior.
- Level 2: Allows gradual optimization that may improve runtime without significant increases in compile time.
For example, compiling with optimizations can be done with:
g++ -O2 hello.cpp -o hello
Cross-Platform Compilation
Using cross-compilers allows you to compile code for different platforms without switching your development environment. Tools like CMake facilitate this by providing configurations that define how to build your project across various platforms.
For example, if using CMake, you might set up a `CMakeLists.txt` that specifies:
cmake_minimum_required(VERSION 3.10)
project(HelloCrossPlatform)
add_executable(hello hello.cpp)
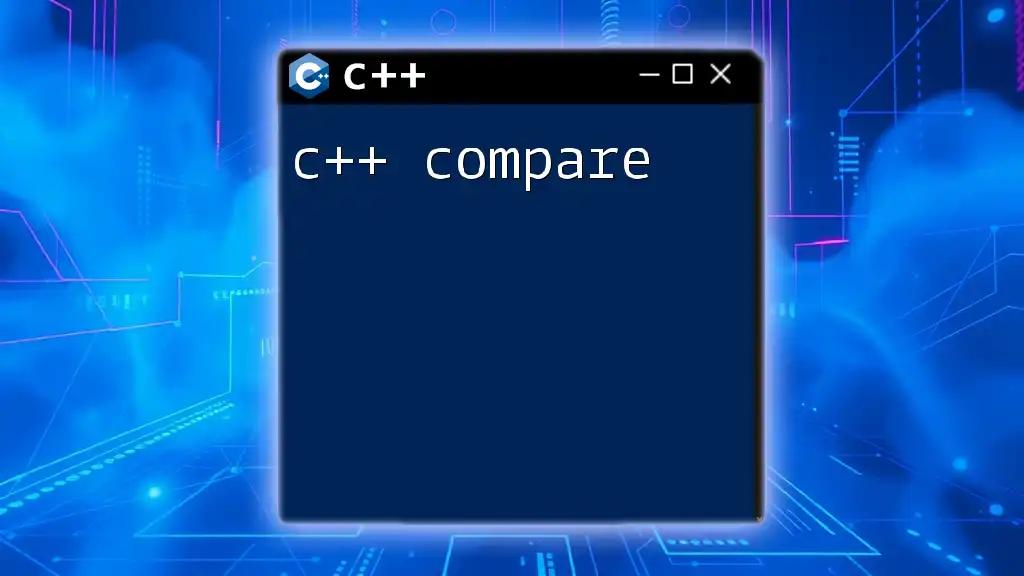
Conclusion
Understanding the intricacies of C++ compilers is a foundational step in mastering C++. From selecting the right compiler for your needs, to installing and using it effectively in your projects, each step lays the groundwork for successful application development. Experimenting with different compilers and leveraging their unique features can significantly enhance your experience and proficiency in C++ programming.

Additional Resources
Online Documentation and Tutorials
- GCC Documentation: Comprehensive guide to using the GCC compiler.
- Clang Documentation: Detailed resources for working with Clang.
- MSVC Documentation: Official guide for using Visual Studio with C++.
Community Forums and Support
Participating in communities such as Stack Overflow or the C++ Reddit can provide answers to common queries and solutions to challenging programming problems, fostering a supportive learning environment.