The "C++ Complete Reference" serves as an essential guide to the C++ programming language, providing clear explanations and examples of its syntax, commands, and features to enhance your coding skills.
Here’s a simple C++ program that demonstrates the use of the `iostream` library to output "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding the Basics
What is C++?
C++ is an object-oriented programming language that was developed by Bjarne Stroustrup in the late 1970s as an extension of the C programming language. It blends low-level memory manipulation with high-level abstraction, making it a versatile tool for various types of software development, including systems software, application software, and game development. Its features allow programmers to express complex ideas in a concise manner.
Setting Up Your C++ Environment
Before diving into coding, one must have the right environment set up.
IDE Choices
Choosing an Integrated Development Environment (IDE) can significantly enhance your coding experience. Some popular IDEs include:
- Microsoft Visual Studio: A powerful IDE mainly for Windows users, providing extensive features for C++ development.
- Code::Blocks: An open-source IDE that supports multiple platforms.
- CLion: A cross-platform IDE from JetBrains that provides smart assistance and powerful debugging capabilities.
Compilers
C++ requires a compiler to translate your code into machine-readable instructions. Notable compilers include:
- G++: A part of the GNU Compiler Collection, ideal for Linux users and supports a wide range of standards.
- Clang: An LLVM-based compiler with exceptional performance and error diagnostics.
- MSVC: Microsoft's Visual C++ compiler, commonly used in Windows development.
Installation Tips
Follow simple steps to get started with C++:
- Download your preferred IDE and install it.
- If needed, install a compiler. Most IDEs come with one pre-integrated.
- Configure your IDE settings to recognize the compiler paths.
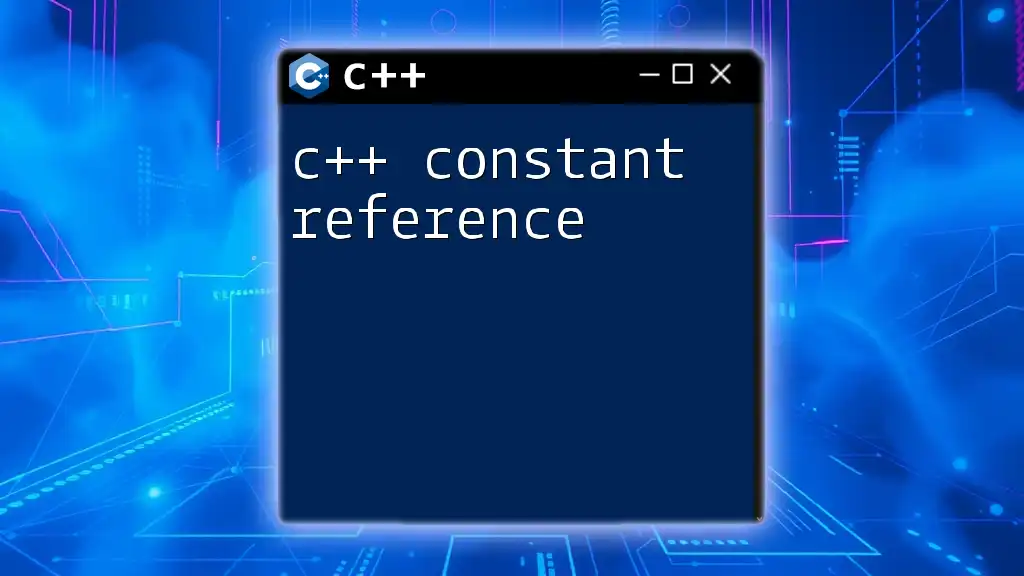
Core Concepts of C++
Variables and Data Types
In C++, variables act as containers for storing data, and data types determine what kind of data can be stored.
Basic Data Types
C++ supports several built-in data types, including:
- int: Represents integers.
- float: Used for single-precision floating-point numbers.
- double: For double-precision floating-point numbers.
- char: Represents a single character.
- bool: Represents Boolean values (true or false).
A quick example:
int number = 10;
float pi = 3.14;
Type Modifiers
Type modifiers allow you to modify the size of data types to better utilize memory. They include `signed`, `unsigned`, `short`, and `long`, each accommodating various range and size conditions. Selecting the most appropriate type is crucial for optimizing efficiency.
Control Structures
Conditional Statements
Conditional statements enable you to execute code based on certain conditions. The most common are `if`, `else`, and `switch`.
For example:
if (number > 0) {
cout << "Positive";
} else {
cout << "Non-positive";
}
Loops
Loops, including `for`, `while`, and `do-while`, allow for repeated execution of code blocks. Here’s a quick example of a for loop iterating through an array:
int arr[] = {1, 2, 3, 4, 5};
for (int i = 0; i < 5; i++) {
cout << arr[i] << " ";
}
Functions in C++
Functions are essential for structuring your code logically. They allow for reusable blocks that perform specific tasks.
Function Declaration and Definition
A function must be declared before it’s used. Here’s a simple example:
void greet() {
cout << "Hello, world!";
}
Function Overloading
C++ allows function overloading, meaning you can create multiple functions with the same name as long as their parameter lists differ. This feature aids readability and reduces naming complexity.
int add(int a, int b) {
return a + b;
}
double add(double a, double b) {
return a + b;
}
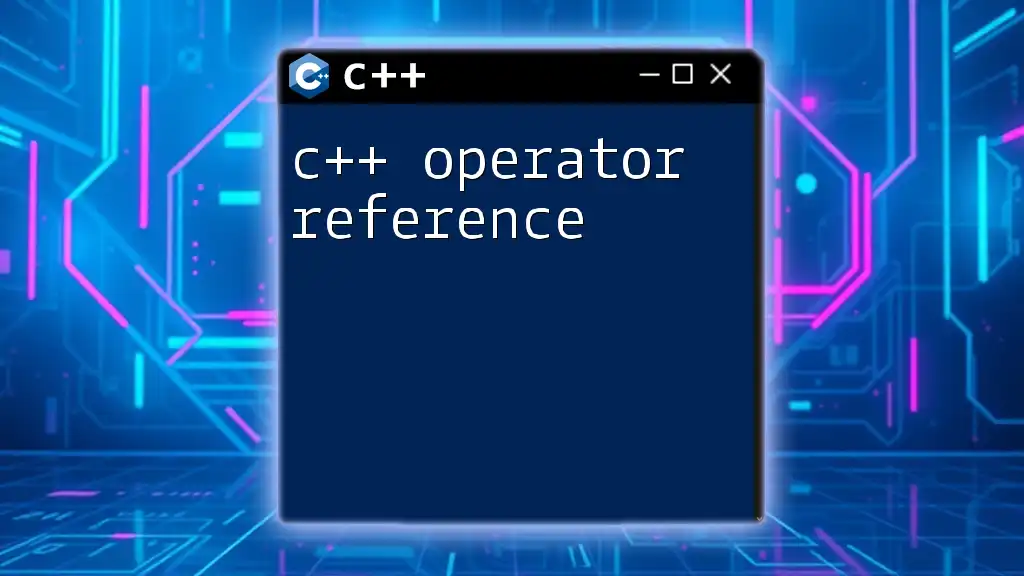
Object-Oriented Programming in C++
Understanding OOP Concepts
C++ is fundamentally built on the principles of Object-Oriented Programming (OOP), which promotes greater organization and reliability in code.
Classes and Objects
A class serves as a blueprint for creating objects. Here’s a simple class `Dog` example:
class Dog {
public:
void bark() {
cout << "Woof!";
}
};
Encapsulation
Encapsulation restricts access to the inner workings of a class. By utilizing private and public access specifiers, you control how data is accessed and modified.
Inheritance
Inheritance allows classes to inherit characteristics from parent classes, promoting code reuse. C++ supports both single and multiple inheritance.
Here’s a basic snippet illustrating inheritance:
class Animal {
public:
void eat() {
cout << "Eating...";
}
};
class Dog : public Animal {
public:
void bark() {
cout << "Woof!";
}
};
Polymorphism
Polymorphism allows methods to do different things based on the object that it is acting upon. It can be classified into runtime and compile-time polymorphism.
An example of runtime polymorphism is using virtual functions:
class Animal {
public:
virtual void sound() {
cout << "Some sound";
}
};
class Dog : public Animal {
public:
void sound() override {
cout << "Woof!";
}
};
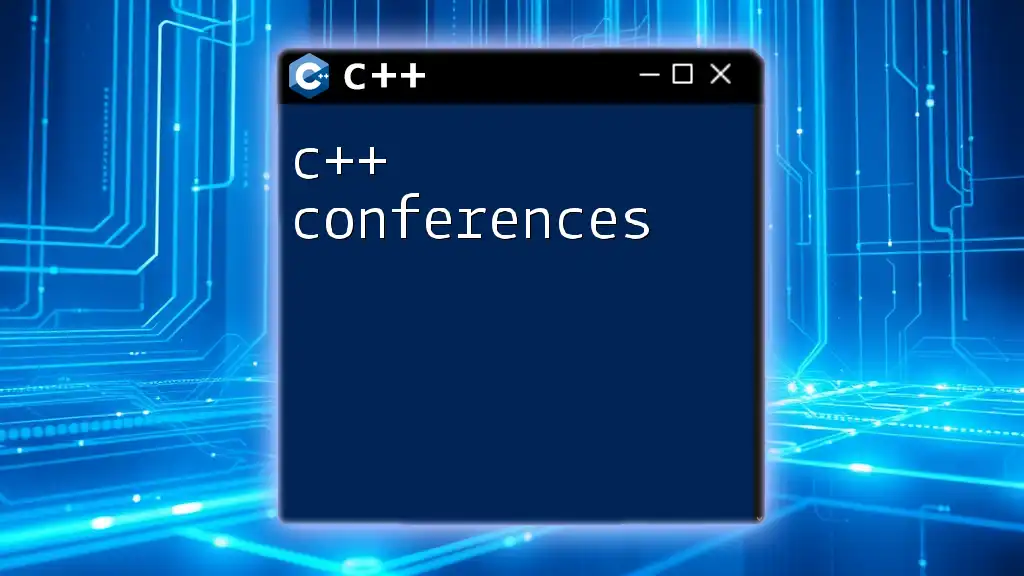
Advanced C++ Features
Templates
Templates enable writing generic and reusable code.
Function Templates
A function template allows you to create a function that operates on any data type:
template <typename T>
T add(T a, T b) {
return a + b;
}
Class Templates
Similar to function templates, class templates allow building a class that can work with any data type, making your classes more flexible.
template <typename T>
class Box {
public:
T content;
void setContent(T item) {
content = item;
}
};
Exception Handling
Exception handling in C++ is a mechanism to handle runtime errors:
Try, Catch, and Throw
By wrapping code that may throw exceptions in a `try` block, you can catch and handle errors gracefully.
try {
int x = 0;
if (x == 0) throw "Division by zero!";
} catch (const char* msg) {
cout << msg;
}
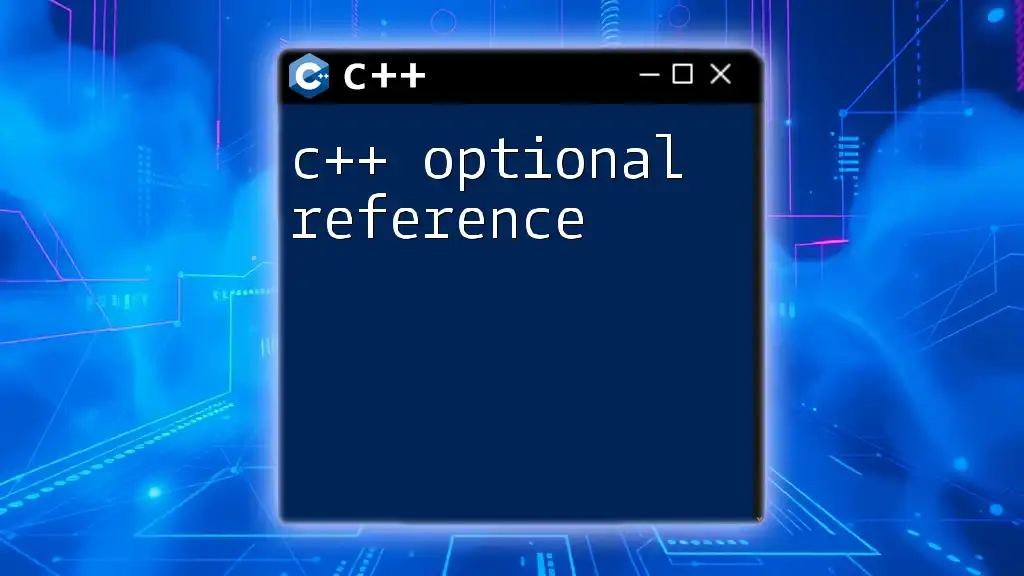
Standard Template Library (STL)
The Standard Template Library (STL) contains a powerful set of C++ template classes for data structures and algorithms.
Overview of STL
STL offers key components such as containers, algorithms, and iterators, promoting efficient programming and code reuse.
Common Containers
Some commonly used containers include:
- Vector: A dynamic array that can resize itself.
- List: A doubly linked list that allows for fast insertions and deletions.
- Map: An associative array that stores pairs of keys and values.
Example of basic operations using vectors:
#include <vector>
vector<int> numbers;
numbers.push_back(1);
numbers.push_back(2);
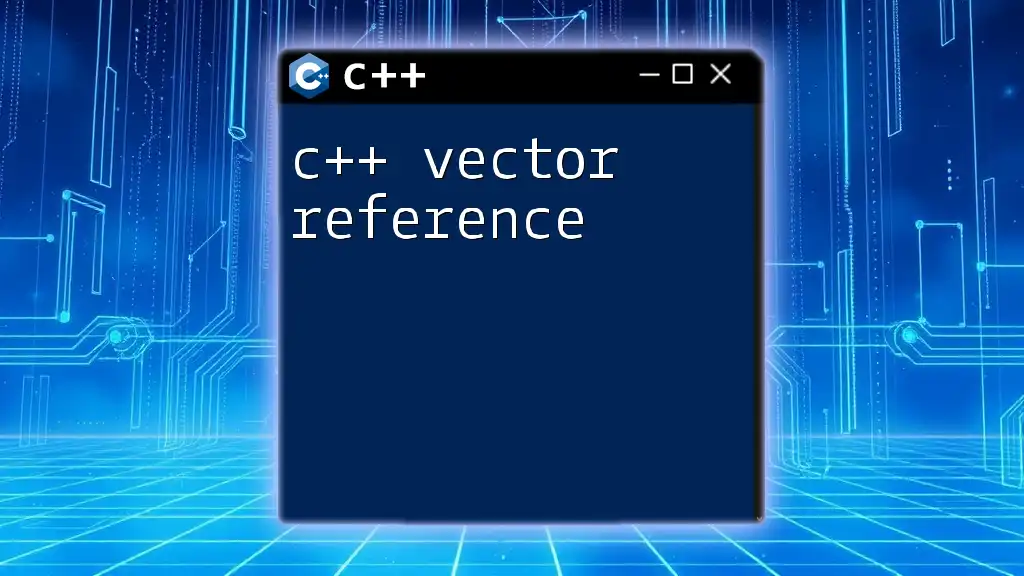
Best Practices in C++
Coding Standards
Maintaining a consistent coding style makes your code more readable and manageable.
Naming Conventions
Use meaningful names for functions and variables that reflect their purpose. For example, prefer `calculateSum` over `cs`.
Commenting Code
Effective commenting aids in understanding the intent behind code sections. Document complex logic and algorithms for future reference.
Debugging and Optimization
Ensuring your code runs efficiently can significantly improve performance.
Common Debugging Techniques
Utilize debugging tools such as breakpoints and watches to track errors. Familiarize yourself with your IDE's debugging features for a smoother experience.
Performance Optimizations
Implement techniques like improving algorithm efficiency and using `std::move` to manage resources finely, which can lead to vast performance improvements.
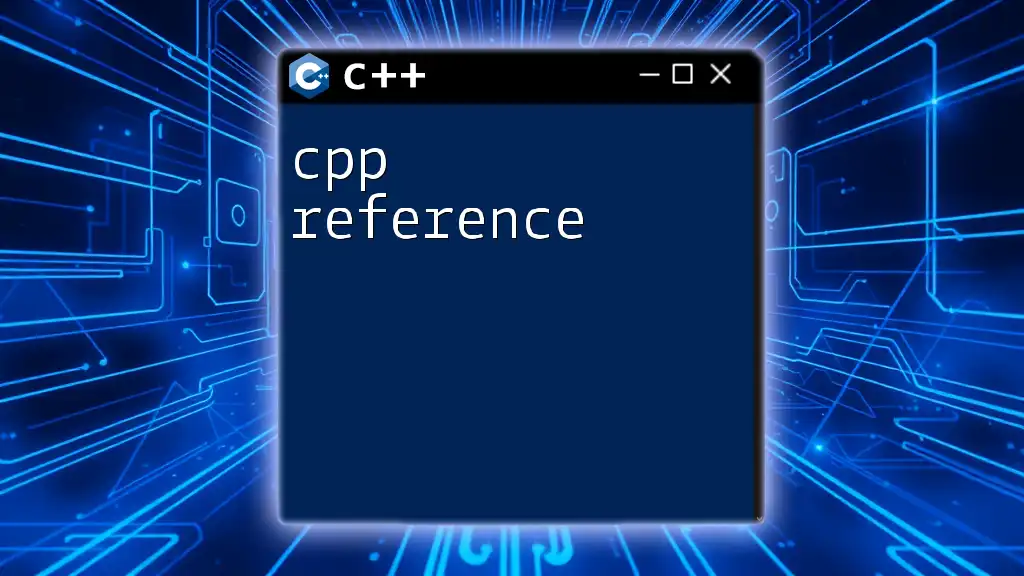
Conclusion
Mastering C++ is not just about understanding syntax; it involves grasping complex concepts such as OOP, templates, and STL. As you delve deeper into the language, apply these principles to create efficient and robust software.
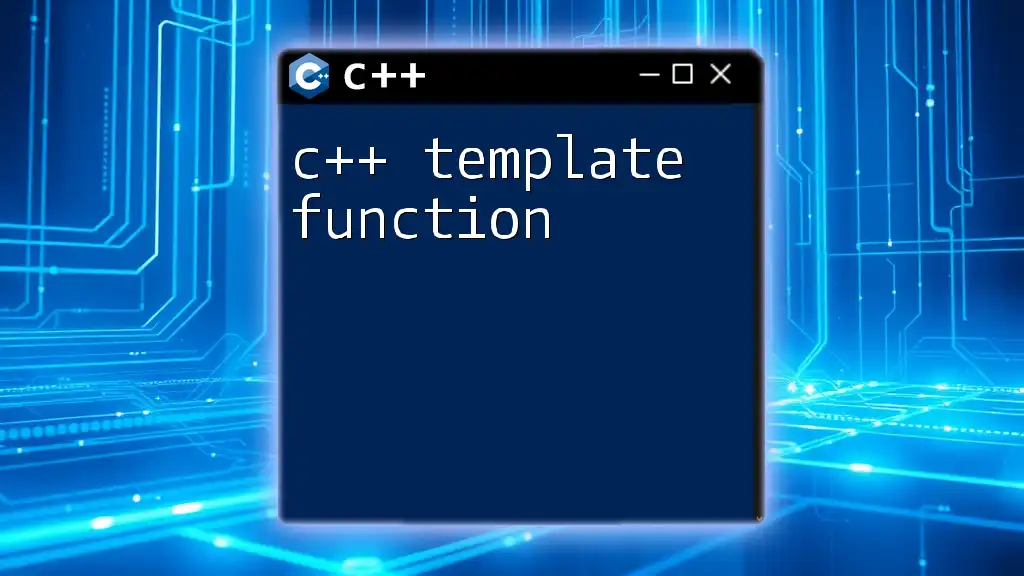
Call to Action
Join our C++ community! Connect with fellow learners through online platforms, participate in webinars, and attend workshops to enhance your C++ skills and knowledge.