In C++, a const reference allows you to refer to an object without modifying it, providing both a performance benefit by avoiding unnecessary copies and ensuring the original object remains unchanged.
Here's an example:
#include <iostream>
void printValue(const int& value) {
std::cout << "Value: " << value << std::endl;
}
int main() {
int num = 42;
printValue(num); // Passing num as a const reference
return 0;
}
Understanding References in C++
What is a Reference?
In C++, a reference is an alias for another variable. It allows you to refer to the same memory location without creating a new variable. The primary syntax for creating a reference is as follows:
int x = 10;
int& ref = x; // ref is a reference to x
This means that any change to `ref` will affect `x`, as both refer to the same memory address.
Types of References
C++ has two main types of references: lvalue references and rvalue references.
-
Lvalue References: These are what we typically think of when referencing variables. An lvalue reference can bind to an lvalue (an object that has an identifiable location in memory).
int a = 5; int& lvalueRef = a; // Valid
-
Rvalue References: Introduced in C++11, rvalue references can bind to temporary objects (rvalues). They are denoted using `&&`.
int&& rvalueRef = 20; // Valid, binding to a temporary
The distinction between lvalue and rvalue references is crucial, particularly when optimizing resource management and avoiding unnecessary copies.

Const References
What is a Const Reference?
A constant reference is a type of reference that does not allow the object it refers to be modified. The syntax for declaring a const reference is as follows:
const int& constRef = x;
In this example, any attempt to alter the value of `constRef` will result in a compilation error, as it is bound to a constant reference.
Why Use Const References?
Using const references provides several benefits:
- Preventing Modification: They ensure that the referenced variable cannot be altered through the reference, thereby maintaining the integrity of the data.
- Avoiding Unnecessary Copies: When passing large objects (like complex data structures) to functions, using a const reference avoids the overhead of copying while still providing read-only access.
Overall, const references optimize both performance and safety within your C++ programs.

When to Use Const References
Function Parameters and Return Types
Const references are particularly useful in function parameters, especially when dealing with large objects.
void processObject(const MyClass& obj) {
// Read-only access to obj
}
In the above example, `obj` can be accessed without the overhead of copying. It maintains clarity, ensuring that `processObject` cannot modify the original object, thus enhancing the function's safety.
Using const references in return types can also be advantageous:
const MyClass& getObject() {
static MyClass obj; // Ensure the object remains alive
return obj; // Return a const reference
}
This allows for efficient access to large objects while preventing modification from the outside scope, showcasing effective use of memory resources.
Working with Standard Library and Containers
When working with standard library collections like `std::vector` or `std::string`, employing const references can make a significant difference:
#include <vector>
#include <iostream>
void printVector(const std::vector<int>& vec) {
for (const int& num : vec) {
std::cout << num << " "; // Read access only
}
}
Using const references here allows the function to handle large vectors without copying, leading to better performance, particularly when the size of the vector is substantial.
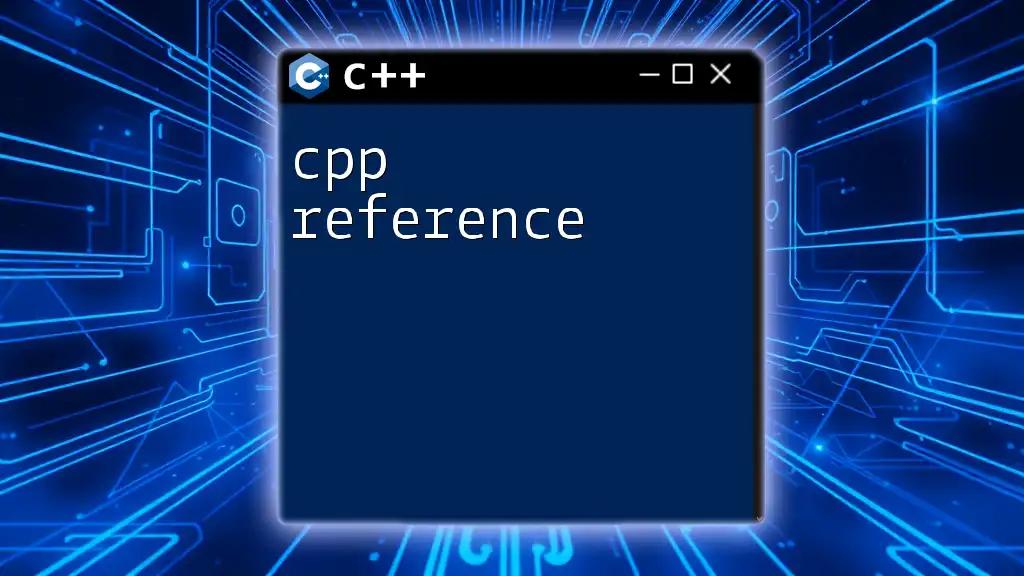
Pitfalls of Const References
Common Mistakes
One common mistake when using const references is attempting to modify the referenced object:
const int& constRef = x;
constRef = 20; // Compiler error: cannot modify a const reference
This showcases the fundamental property of const references — any attempt to change them will result in a compilation error, making it clear that mutation is unwanted.
Lifespan Considerations
Another important aspect to consider is the lifespan of the object being referenced. A const reference can lead to dangling references when the original object goes out of scope:
const int& danglingRef = someLocalFunction(); // Dangerous if local function returns a reference
To avoid this, ensure that the object referenced by a const reference remains in scope for as long as the reference is needed.
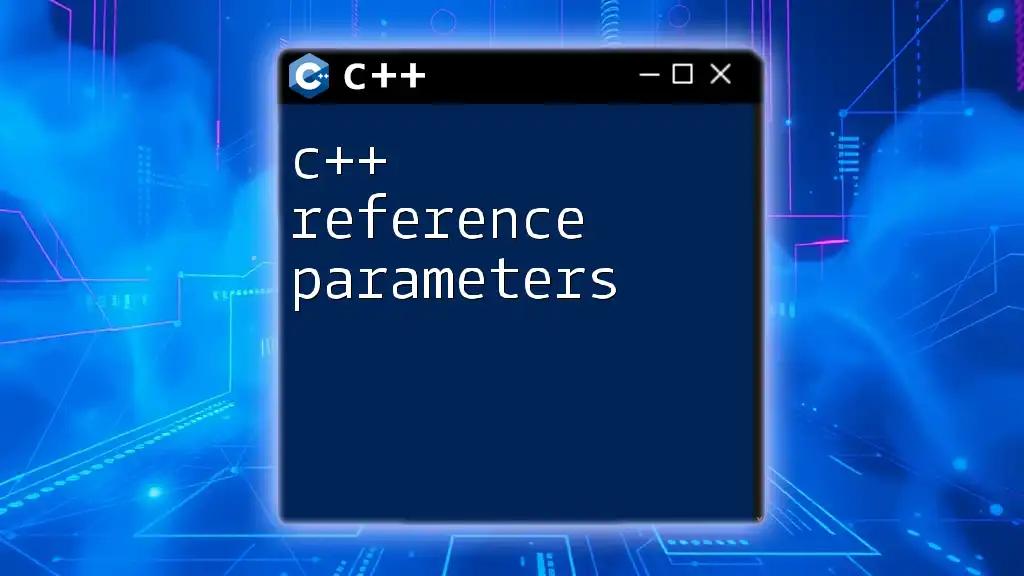
Best Practices for Using Const References
General Guidelines
When deciding whether to use const references or pointers, consider using const references when:
- You need read-only access to an object.
- The size of the object is large enough to warrant avoiding copies.
In cases where a pointer may be required (like for dynamic memory allocation), carefully weigh the trade-offs of clarity and performance against the necessity of mutability.
Code Readability and Maintenance
Employing const references enhances the readability of your code. They clearly communicate to other developers that the referenced variable will not be altered by your function, a sign of well-structured and maintainable code.
void displayValue(const int& value) {
std::cout << "Value: " << value << std::endl; // Clearly indicates intent
}
Using comments to document the purpose of const references can further enhance understanding and maintainability.

Real-world Applications of Const References
Case Study: Using Const References in Large Data Structures
Consider a function that processes large datasets, such as images or 3D graphics.
class LargeDataSet {
public:
void analyze() const { /* Function logic */ }
};
void processData(const LargeDataSet& data) {
data.analyze(); // Efficiently access without copying large data
}
Using const references enables the function to access large datasets without copies, resulting in efficient memory and performance.
Advanced Usage with Templates
Const references shine in template programming as well. They can help maintain type safety while providing flexible and reusable functions.
template<typename T>
void handleData(const T& data) {
// Process data without modifying it
}
In this example, `handleData` works with any type, ensuring that the input data remains unchanged, all while avoiding unnecessary copies.
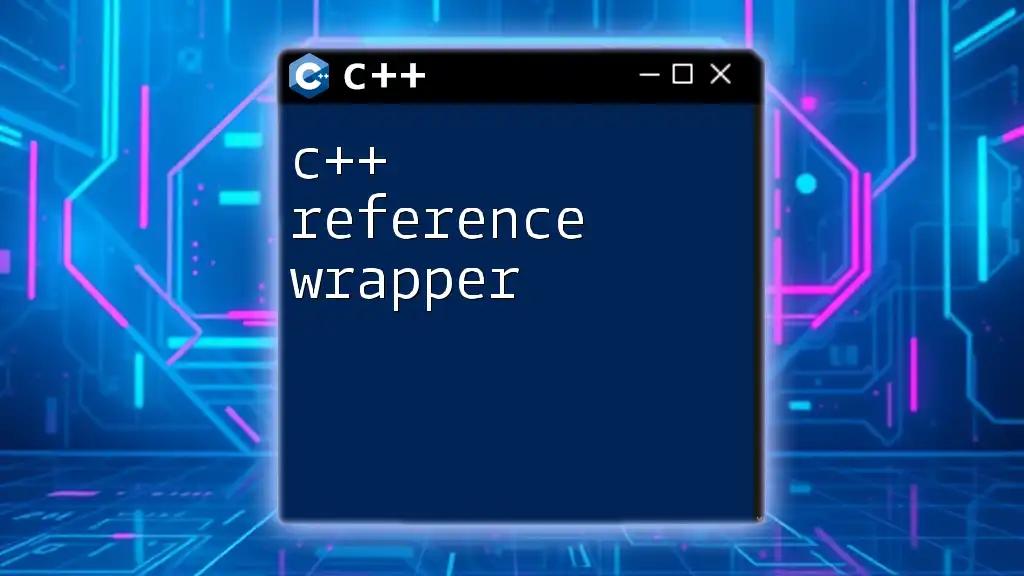
Conclusion
The use of const references in C++ is a powerful technique that enhances performance and safety. By ensuring variables remain read-only and avoiding unnecessary copies, const references are a crucial aspect of efficient C++ programming. Practicing and implementing const references in personal projects can significantly improve code quality and performance.

Additional Resources
For those interested in deepening their understanding of const references and C++ in general, several resources are recommended. Explore books, online courses, and community forums to further hone your C++ skills.
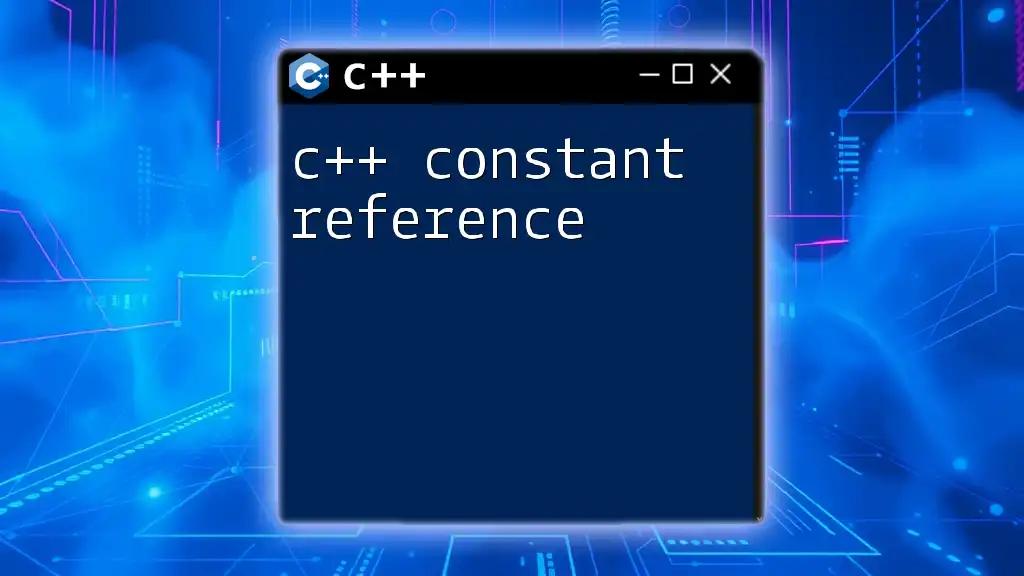
FAQs about Const References
Visitors frequently ask questions around const references, such as their behavior in various contexts and common use cases. Addressing these can clarify misunderstandings and highlight the utility of const references in everyday programming scenarios.