In C++, returning a reference allows a function to give back a reference to an existing variable, enabling direct modification of the variable without copying; this is useful for efficiency and working with large objects.
Here's a simple example:
#include <iostream>
int& getReference(int& x) {
return x; // Return a reference to the passed variable
}
int main() {
int a = 10;
int& ref = getReference(a);
ref = 20; // Modify 'a' through the reference
std::cout << a; // Outputs: 20
return 0;
}
What is a Reference in C++?
In C++, a reference is an alias for another variable. It allows you to refer to the original variable without making a copy. Unlike pointers, which can point to different objects over their lifetime, a reference is bound to a variable once it’s initialized and cannot be changed to reference another variable. The syntax for defining a reference involves using the ampersand (`&`) symbol.
Advantages of Using References
References provide several key advantages:
- Performance Benefits: When large objects are involved, returning by reference avoids the overhead of copying. Instead of creating a new instance of the object, a function can directly work with the existing data.
- Readability: Functions that use references are often easier to read and understand, as they closely resemble regular variable usage without the additional syntax of pointers.
- Functionality Enhancement: References allow functions to modify the original variable directly, which can simplify certain operations and lead to cleaner code.

Returning by Reference in C++
Syntax for Returning a Reference
To create a function that returns a reference, the declaration must specify a type followed by an ampersand. Here is a basic example:
int& returnReference() {
static int variable = 42;
return variable;
}
In this example, the function `returnReference` returns a reference to a static variable `variable`. Static variables maintain their state between function calls, which ensures that the reference remains valid even after the function exits.
Example: Simple Function Returning a Reference
Consider the following code example, which illustrates a function returning a reference to a variable:
int& getReferenceExample() {
static int value = 10;
return value; // Returning a reference to a static variable
}
int main() {
int& ref = getReferenceExample();
ref += 5; // Modify value through the reference
std::cout << getReferenceExample(); // Outputs 15
return 0;
}
In this example, when we call `getReferenceExample`, we alter the value of the static variable `value` through the reference `ref`.

When to Return a Reference
Use Cases for Returning by Reference
Returning by reference can be particularly useful in scenarios where modifying existing data is necessary. Some common use cases include:
- Getter methods in classes that return class member variables.
- Accessing elements of a container, such as arrays or vectors, which allows for direct modification of these elements without additional overhead.
Common Scenarios
An excellent illustration of a getter that returns a reference can be found in the following class structure:
class MyClass {
public:
int& getValue() { return value; }
private:
int value = 20;
};
int main() {
MyClass obj;
int& ref = obj.getValue();
ref += 5; // Modifies the original `value` in MyClass
std::cout << obj.getValue(); // Outputs 25
return 0;
}
In this scenario, `getValue` returns a reference to the `value` member of the class, allowing the caller to modify `value` directly.

Risks of Returning a Reference
Challenges and Dangers
While returning references can be powerful, it comes with some caveats. One of the most significant risks is returning a reference to a local variable. When a function returns a reference to a local variable, that reference points to a memory location that goes out of scope when the function exits, leading to undefined behavior.
Example of Dangling Reference
Consider the following example that demonstrates the issue with dangling references:
int& dangerousFunc() {
int localVar = 10;
return localVar; // This will lead to undefined behavior
}
int main() {
int& ref = dangerousFunc(); // ref now points to a local variable
std::cout << ref; // Undefined behavior
return 0;
}
In this case, `localVar` is destroyed when `dangerousFunc` finishes executing, which means the reference `ref` is left dangling and accessing it can lead to unpredictable results.
Best Practices for Safe Reference Returns
To mitigate the risks associated with dangling references:
- Always return references to static, global variables, or class member variables where their lifetime is guaranteed.
- Ensure that the lifetime of any referenced data exceeds the usage of the reference in calling functions.

Returning a Reference in C++: Additional Features
Const References
Const references are references that cannot be modified. They can be particularly useful as they allow you to pass large objects without making copies while ensuring that the object remains unchanged:
const int& getConstReference() {
static int value = 10;
return value;
}
int main() {
const int& ref = getConstReference();
// ref = 20; // Not allowed: cannot modify a const reference
std::cout << ref; // Outputs 10
return 0;
}
This practice is especially common in function parameters when passing large objects, as it prevents unnecessary copying while maintaining encapsulation.
Overloading Operators with References
Returning a reference is also useful when overloading operators in C++. Here’s a brief illustration:
class MyClass {
public:
MyClass& operator=(const MyClass& other) {
// Assignment logic goes here
return *this; // Returning reference to the current object
}
};
In this operator overload, returning `*this` as a reference allows subsequent chaining of assignments (e.g., `a = b = c;`).

Conclusion and Key Takeaways
Understanding how to return a reference in C++ is fundamental for effective programming in this language. Returning by reference allows you to work directly with existing data, enhancing performance and simplifying syntax. However, it’s crucial to be mindful of the risks involved, particularly when dealing with lifetimes of variables. By adhering to best practices and leveraging features like const references and operator overloading, you can write safe and efficient C++ code.
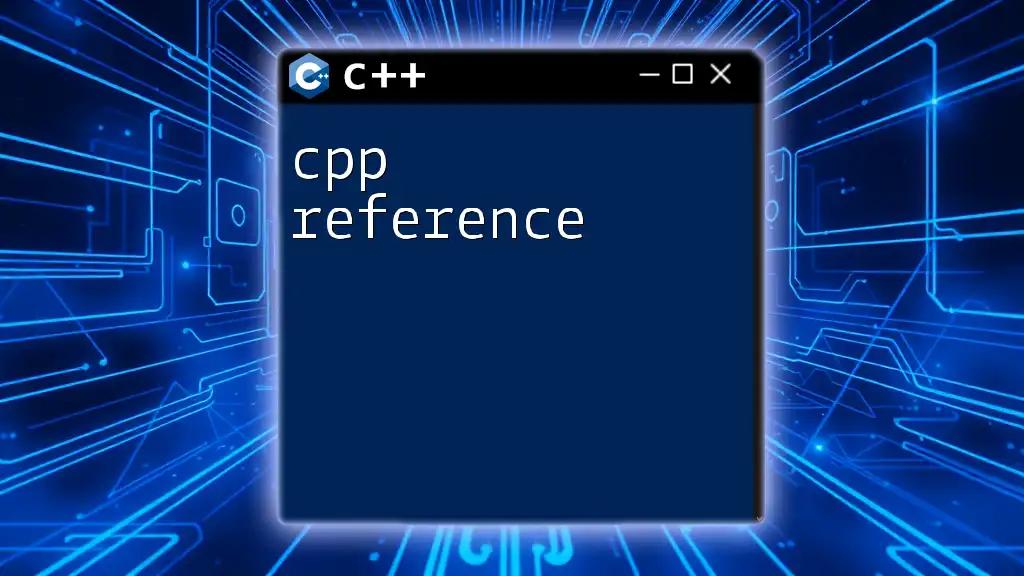
Additional Resources
For further reading, consider exploring advanced C++ topics and the official documentation on references. Engaging in community forums can also provide valuable insights and answer any lingering questions on this essential subject.
Call to Action
If you want to dive deeper into the intricacies of C++ commands and improve your coding skills, consider joining our courses or workshops designed to empower you with concise and effective programming techniques.