To reverse an array in C++, you can use a simple loop to swap elements from both ends of the array until you reach the middle. Here's a code snippet demonstrating this:
#include <iostream>
using namespace std;
void reverseArray(int arr[], int n) {
for(int i = 0; i < n / 2; i++) {
swap(arr[i], arr[n - i - 1]);
}
}
int main() {
int arr[] = {1, 2, 3, 4, 5};
int n = sizeof(arr) / sizeof(arr[0]);
reverseArray(arr, n);
for(int i = 0; i < n; i++)
cout << arr[i] << " ";
return 0;
}
Understanding Arrays in C++
What is an Array?
An array is a collection of items stored at contiguous memory locations. It can be thought of as a container that holds a fixed number of values of a single type. In C++, arrays are crucial for efficient storage and processing of data. They simplify access to related data elements through indexing while enabling easy management of grouped elements.
Example of declaring and initializing an array:
int arr[5] = {1, 2, 3, 4, 5};
In this example, we create an integer array named `arr`, holding five elements.
Types of Arrays in C++
C++ supports various types of arrays:
-
Single-dimensional Arrays: These are the most common arrays, holding a linear sequence of elements. For example, an array of integers can be created to store student grades.
-
Multi-dimensional Arrays: These arrays extend the concept of a single array by allowing indexes to reference multiple levels of data, like a matrix in mathematics. An example would be:
int matrix[2][3] = {{1, 2, 3}, {4, 5, 6}};
Understanding these array types lays the groundwork for manipulating data effectively, particularly when it comes to reversing an array in C++.

Methods to Reverse an Array in C++
Manual Reversal Method
One widely used technique for reversing an array is the two-pointer technique. This method involves using two pointers to swap elements until you have traversed half the array.
Here's a concise code snippet to demonstrate this method:
void reverseArray(int arr[], int size) {
int start = 0;
int end = size - 1;
while (start < end) {
std::swap(arr[start], arr[end]); // Swapping elements
start++; // Move forward from the start
end--; // Move backward from the end
}
}
Detailed Breakdown:
- `std::swap` is a function from the <algorithm> header that swaps two elements.
- The starting pointer (`start`) begins at index 0 while the ending pointer (`end`) begins at the last index of the array. They move toward each other until they meet.
Using the Standard Library
Utilizing `std::reverse`
C++ offers built-in functionality that can simplify the process of reversing an array. The `std::reverse` function from the `<algorithm>` library is one such tool that promotes code efficiency and readability.
Here’s how you can use `std::reverse`:
#include <algorithm>
#include <iostream>
void reverseWithStd(int arr[], int size) {
std::reverse(arr, arr + size); // Reverse the array in place
}
Using standard library functions brings benefits such as reduced coding errors, increased efficiency, and clearer intent, leading to better maintainability.

Reversing an Array C++: Edge Cases
Handling Empty Arrays
When dealing with an empty array, it is crucial to ensure that your code can gracefully manage such scenarios. An empty array should not trigger errors or undesired behavior:
void reverseEmptyArray(int arr[], int size) {
// No action needed, just return
}
No elements to swap means the function exits immediately without any operations.
Working with Odd and Even Length Arrays
The behavior of reversal differs based on whether the array has an odd or even number of elements. When reversing an odd-length array, the middle element will remain in place, while for an even-length array, all elements will swap positions completely.
Example of an Odd Length Array:
int oddArr[5] = {1, 2, 3, 4, 5}; // Becomes: {5, 4, 3, 2, 1}
Example of an Even Length Array:
int evenArr[4] = {1, 2, 3, 4}; // Becomes: {4, 3, 2, 1}
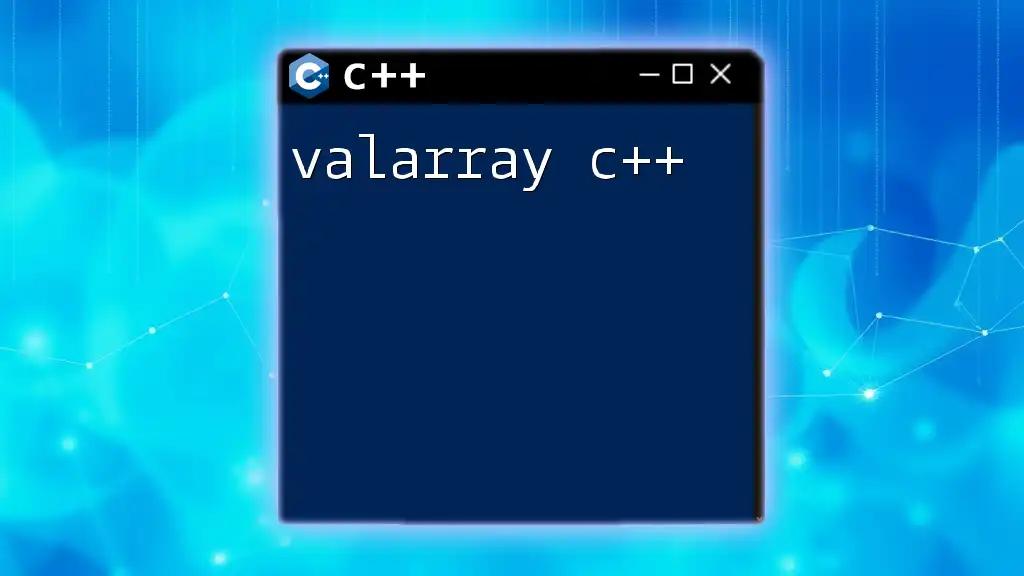
Common Mistakes to Avoid When Reversing an Array in C++
Inefficient Algorithms
While brainstorming ways to reverse an array, many novice programmers might resort to inefficient algorithms that run in O(n^2) time complexity. Such approaches involve nested loops to swap elements., which drastically reduce performance.
Pointer Offsets
Mismanagement of array indices can lead to critical issues, including memory access violations. When swapping elements, ensure that the pointers correctly reference the intended array elements. Always validate array bounds, particularly in dynamic contexts.
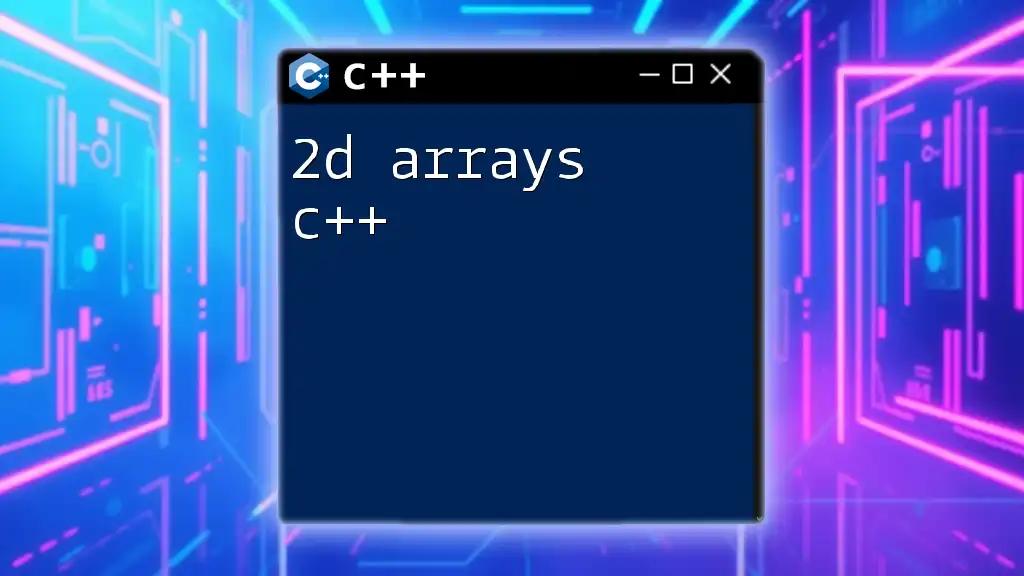
Best Practices for Reversing an Array C++
Choosing the Right Method
When considering how to reverse an array, it's important to evaluate the trade-offs between manual methods versus using the standard library. Using `std::reverse` is usually preferred due to its simplicity and robustness, especially in larger projects.
Code Maintainability
Practicing clean code is paramount. Keep code readable by:
- Commenting crucial areas
- Structuring code logically
- Following established naming conventions to enhance clarity

Conclusion
In summary, understanding how to reverse an array in C++ opens the door to effective data management and manipulation in programming. Familiarity with various methods, identifying edge cases, and adhering to best practices will significantly a programmer's efficiency. So, practice these strategies to become proficient in using C++ arrays!

Additional Resources
To further develop your understanding, you may explore authoritative C++ documentation, recommended books, and online platforms that offer practice exercises. These can significantly enhance your skills in contemporary C++ programming.

Call to Action
What techniques have you found useful in reversing an array in C++? Share your experiences and tips in the comments! Don't forget to subscribe for more insights and strategies on effectively utilizing C++ commands.