In C++, an array is a fixed-size collection of elements of the same type, whereas a vector is a dynamic array that can grow or shrink in size, providing more flexibility.
#include <iostream>
#include <vector>
int main() {
// Array example
int arr[5] = {1, 2, 3, 4, 5};
// Vector example
std::vector<int> vec = {1, 2, 3, 4, 5};
vec.push_back(6); // Vectors can change size
return 0;
}
Understanding Arrays in C++
What is an Array?
An array in C++ is a collection of elements, all of the same type, stored in contiguous memory locations. Each element can be accessed using an index, with the first element at index 0. Here’s how you can declare and initialize an array:
int numbers[5] = {1, 2, 3, 4, 5};
Benefits of Using Arrays
Arrays have certain advantages:
- Memory Efficiency: Since the size is fixed at compile-time, arrays can be more memory efficient compared to vectors, especially if the size is known beforehand.
- Quick Access: You can access any element in an array directly using its index, leading to O(1) time complexity for access operations.
Consider a scenario where you need to store a known number of temperature readings for a week. An array is ideal here because the size doesn’t change.
Limitations of Arrays
However, arrays have notable limitations:
- Fixed Size: Once declared, the size of an array cannot be changed, leading to wasted space if the storage requirement is overestimated, or overflow errors if underestimated.
- Lack of Built-in Functions: Arrays do not come with built-in methods for resizing or manipulating elements. You need to implement such functionalities manually, which can lead to more coding overhead.
For instance, if you wish to add an element beyond the fixed size of an array, it will cause complications leading to runtime errors.

Understanding Vectors in C++
What is a Vector?
A vector in C++ is a dynamic array that can grow and shrink during its runtime. Vectors belong to the standard template library (STL) and offer various utilities that make them versatile and powerful. You can initialize a vector as follows:
#include <vector>
std::vector<int> numbers = {1, 2, 3, 4, 5};
Benefits of Using Vectors
Vectors provide several advantages over arrays:
- Dynamic Resizing: Vectors automatically handle memory allocation, allowing them to grow and shrink based on the elements added or removed.
- Convenient Functions: Vectors come equipped with a rich set of member functions, such as `push_back` to add elements, `pop_back` to remove the last element, and many more that make data manipulation straightforward.
For example:
numbers.push_back(6);
In this case, the vector dynamically increases its size to accommodate the new element.
Limitations of Vectors
While vectors are flexible, they also have some downsides:
- Slight Overhead: The process of dynamic memory allocation can introduce overhead in terms of performance compared to static arrays, due to the potential need for reallocating memory.
- Performance in Specific Scenarios: In situations requiring constant-time complexity, such as when using array indexes directly, arrays might outperform vectors.
Comparing Arrays and Vectors
Syntax Differences
A direct comparison of array and vector syntax reveals their essential differences. Here’s a side-by-side look:
// Array
int arr[5] = {1, 2, 3, 4, 5};
// Vector
std::vector<int> vec = {1, 2, 3, 4, 5};
Memory Management
The memory management strategies of arrays versus vectors differ fundamentally:
- Arrays: Memory for arrays is allocated statically, meaning the size must be known at compile-time. This can be efficient in terms of access speed, but it does limit flexibility.
- Vectors: Memory for vectors is allocated dynamically. This allows for elements to be added or removed without needing to worry about exceeding an initial size.
Performance Considerations
In terms of performance:
- Access Speed: Both arrays and vectors offer O(1) access time. However, vectors might have additional overhead during insertions or deletions due to dynamic resizing.
- Real-world Examples: In applications where performance is critical, such as real-time systems or specific algorithms, arrays might be favored. Conversely, for general use where flexibility is more important, vectors are often preferred.
// Example of timing access
#include <chrono>
#include <vector>
#include <iostream>
int main() {
std::vector<int> vec(1000000, 0);
int sum = 0;
auto start = std::chrono::high_resolution_clock::now();
for (size_t i = 0; i < vec.size(); ++i) {
sum += vec[i];
}
auto end = std::chrono::high_resolution_clock::now();
std::cout << "Time taken: "
<< std::chrono::duration_cast<std::chrono::milliseconds>(end - start).count()
<< " ms" << std::endl;
return 0;
}
Built-in Functions and Usability
Vectors come with numerous built-in functions which simplify common tasks such as inserting, deleting, and resizing elements, while arrays will require manual handling for these operations.
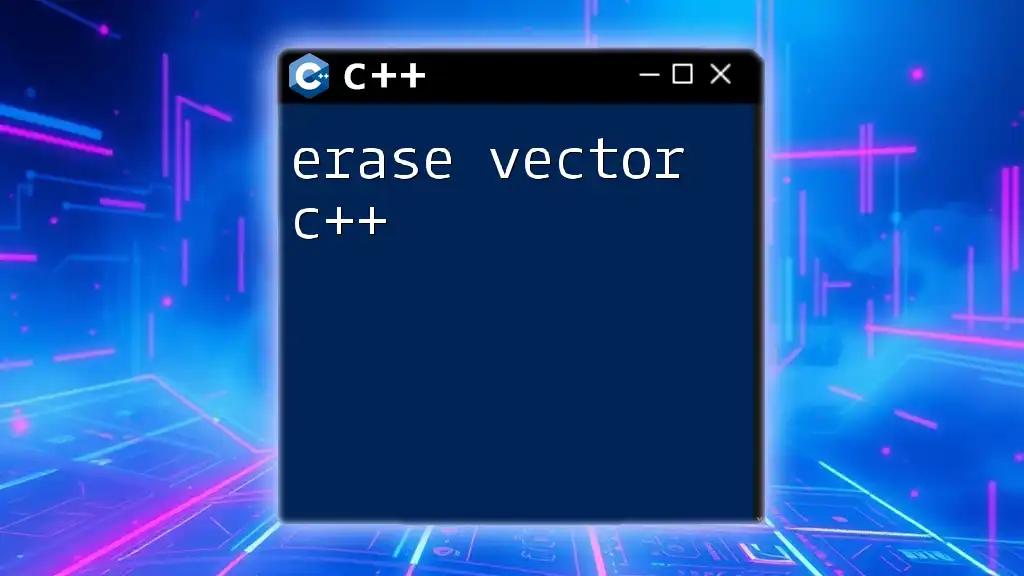
Use Cases for Arrays and Vectors
When to Use Arrays
Arrays can be a great choice when:
- The data size is known beforehand.
- Performance and memory efficiency are critical, such as in embedded systems.
For example, if a program needs to store scores for a fixed number of players, an array would suffice:
int scores[10] = {0}; // scores for 10 players
When to Use Vectors
Vectors, on the other hand, are more suitable when:
- The number of elements is not fixed or during runtime.
- You need to frequently add or remove elements from the collection.
For instance, when creating a list of user inputs where the size is unpredictable, vectors shine:
std::vector<std::string> names;
std::string input;
while (std::cin >> input) {
names.push_back(input); // dynamic addition
}
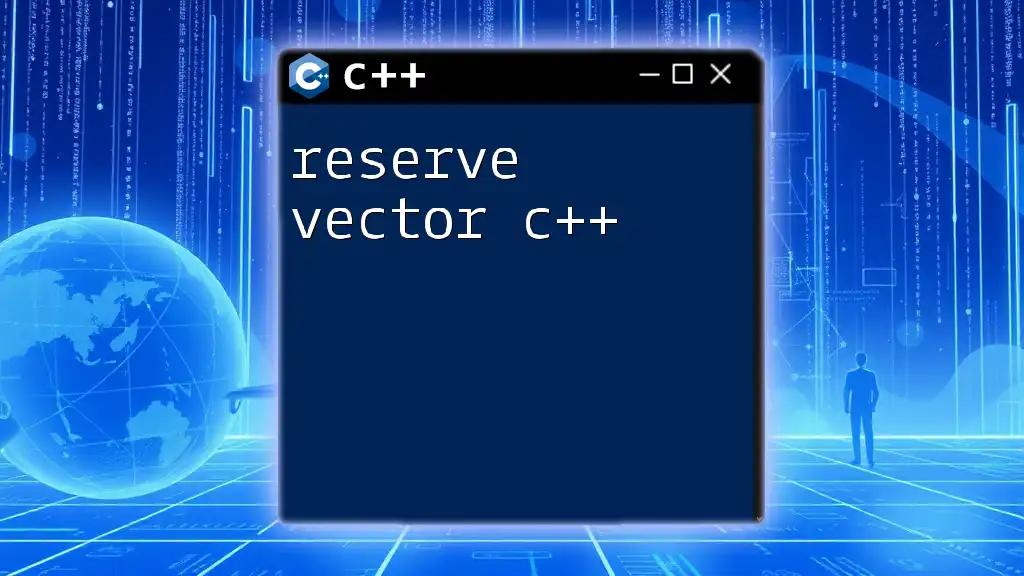
Conclusion
In summary, understanding the differences between arrays and vectors in C++ is crucial for effective programming. Arrays provide speed and efficiency when the size is known and fixed, while vectors offer flexibility and convenience for dynamic data management. The choice between them comes down to the specific requirements of your application. Experiment with both and see which best fits your needs as a developer.