An array of vectors in C++ allows you to create a collection of vector containers, where each vector can store a dynamic array of elements, as demonstrated in the following code snippet:
#include <iostream>
#include <vector>
int main() {
std::vector<int> arrOfVectors[3]; // Array of 3 vectors
// Adding elements to each vector
arrOfVectors[0].push_back(1);
arrOfVectors[1].push_back(2);
arrOfVectors[2].push_back(3);
// Printing elements
for (int i = 0; i < 3; ++i) {
std::cout << "Vector " << i << ": " << arrOfVectors[i][0] << std::endl;
}
return 0;
}
Understanding Vectors in C++
What is a Vector?
A vector in C++ is a dynamic array that can grow or shrink in size as needed, allowing for flexible data management. Unlike traditional arrays, which have a fixed size, a vector can adjust its capacity automatically. This makes vectors particularly powerful for storing collections of data where the number of elements can vary.
Vectors possess several key characteristics:
- They offer dynamic resizing, so you don’t have to specify the size at the time of declaration.
- They provide an intuitive way to access elements using indices similar to arrays.
- They come equipped with several built-in member functions like `push_back()`, `pop_back()`, and `size()`.
For example, consider this simple use of a vector:
#include <iostream>
#include <vector>
int main() {
std::vector<int> myVector = {1, 2, 3, 4, 5};
for (int i : myVector) {
std::cout << i << " ";
}
return 0;
}
Basic Operations with Vectors
Vectors support a variety of operations that can facilitate data manipulation:
-
Initialization: Vectors can be initialized using initializer lists or constructors.
std::vector<int> myVector = {1, 2, 3};
-
Adding and Removing Elements: The `push_back()` method allows you to append elements to the end of the vector, while `pop_back()` removes the last element.
myVector.push_back(4); // Now myVector contains {1, 2, 3, 4} myVector.pop_back(); // Now myVector contains {1, 2, 3}
-
Accessing Elements: You can access elements using `indexing` or the `.at()` method, which avoids out-of-bound errors by performing checks.
std::cout << myVector.at(1); // Outputs 2
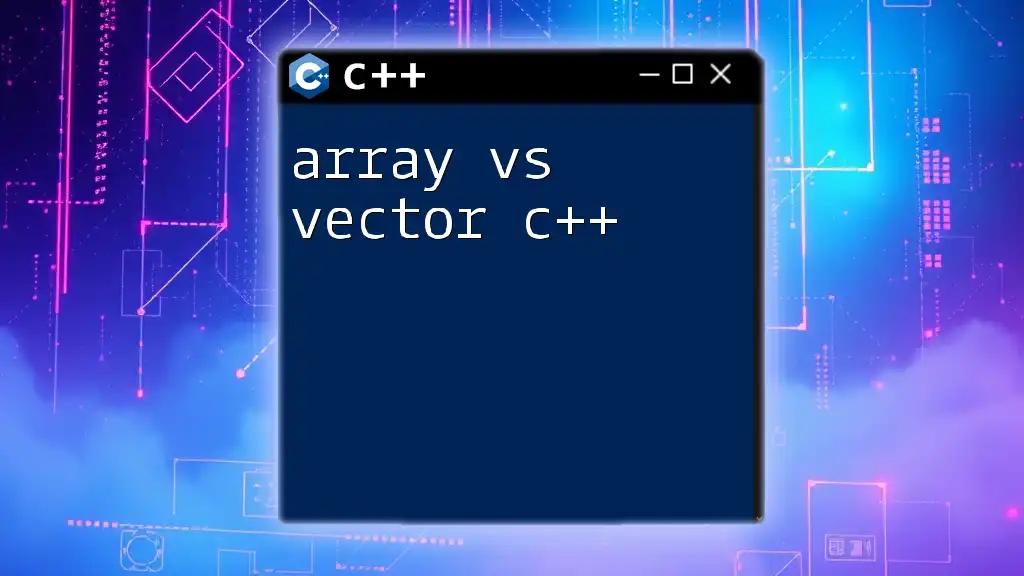
Introducing Arrays of Vectors
What is an Array of Vectors?
An array of vectors is a collection where each element is a vector. This structure allows you to utilize the benefits of both arrays and vectors - the static nature of arrays with the dynamic capabilities of vectors. This is particularly useful when you need to manage collections of different sets of data that have a variable size.
Syntax and Declaration
Declaring an array of vectors in C++ is straightforward. You can use the following syntax:
std::vector<int> arrOfVectors[5]; // Declares an array of 5 vectors
This creates an array named `arrOfVectors` that can store 5 individual vectors, each capable of dynamically holding integers.
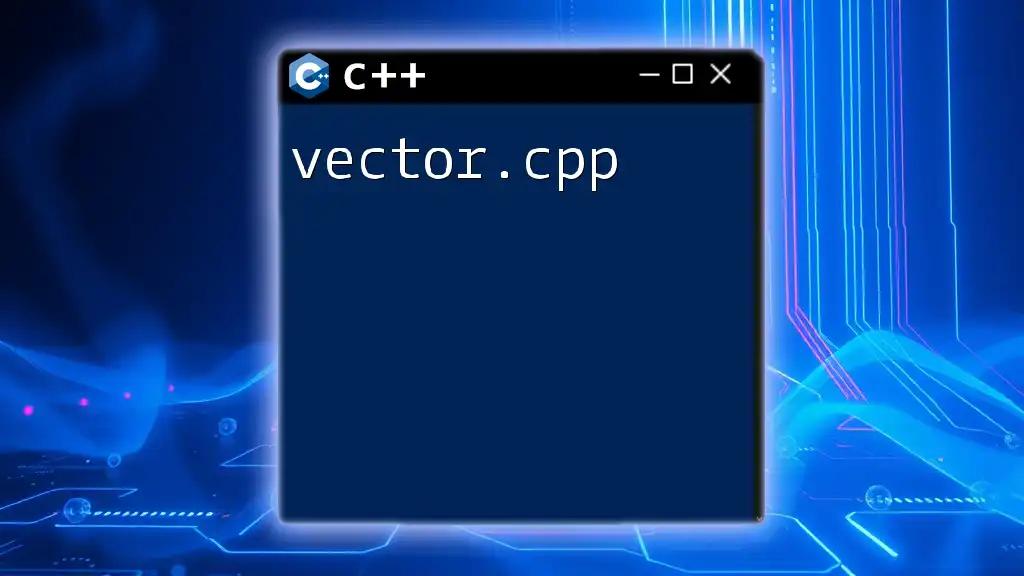
Working with Arrays of Vectors
Adding Elements to the Array of Vectors
To populate the vectors within the array, iterate through each vector and use the `push_back()` method. This is how you can do it:
for (int i = 0; i < 5; ++i) {
arrOfVectors[i].push_back(i + 1); // Adds numbers 1 to 5 to each vector
}
This loop initializes each vector with a unique set of integers.
Accessing and Modifying Elements
Accessing elements in an array of vectors requires you to specify both the vector index and the element index. For example:
arrOfVectors[2][1] = 99; // Modifies the second element of the third vector
This is a powerful feature, as it allows you to manipulate data stored in complex structures easily.
Iterating Through the Array of Vectors
To process or display contents of the array of vectors, you typically use nested loops:
for (int i = 0; i < 5; ++i) {
for (int j = 0; j < arrOfVectors[i].size(); ++j) {
std::cout << arrOfVectors[i][j] << " "; // Outputs all the elements
}
}
This nested loop structure gives you the flexibility to deal with each vector's contents individually.
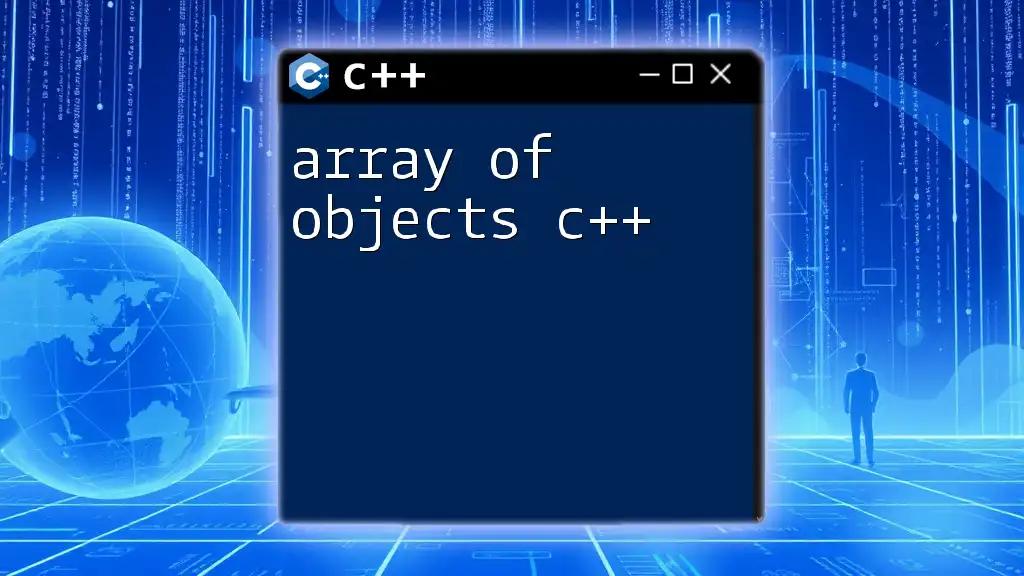
Practical Applications of Array of Vectors
Use Case in Data Structures
A common application for an array of vectors is in implementing adjacency lists for graph structures. Each vector in the array represents a node in the graph and contains a list of its adjacent nodes. Using this method is space-efficient compared to using a matrix for sparse graphs.
Use in Game Development
In game development, arrays of vectors can be used to manage collections of game objects. For instance, you might have an array representing different types of enemies, where each vector holds the coordinates of enemy instances in the game world. This helps in organizing and processing large quantities of entities efficiently.
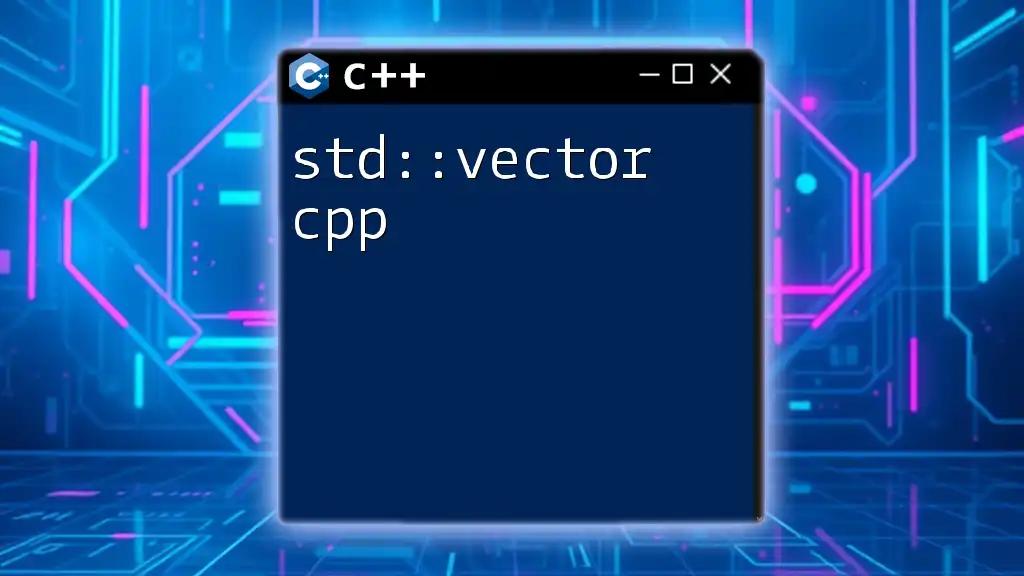
Best Practices for Using Arrays of Vectors
Memory Management Considerations
While vectors manage memory automatically, poor use of arrays of vectors can lead to excessive memory consumption. Be mindful of memory allocation and ensure you free up resources appropriately when not needed. The dynamic nature can cause memory fragmentation if vectors are not trimmed or cleared effectively.
Performance Optimization Tips
Choosing between an array of vectors and other data structures should be based on your needs:
- Use an array of vectors when you need dynamic sizing but also want to maintain categorization across distinct groups of data.
- Consider accessing patterns; if random access is frequent, ensuring that the inner vectors are well-balanced in size can optimize performance.
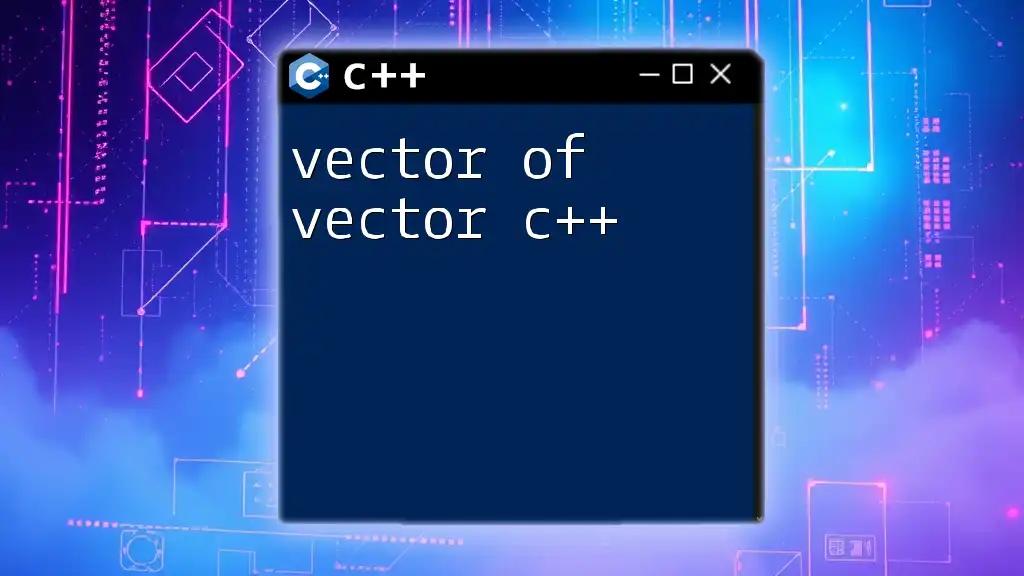
Conclusion
The array of vectors in C++ presents a versatile and efficient way to manage collections of data. By comprehending their use and structure, developers can implement robust applications across various programming scenarios. Whether you're handling data structures in algorithms or managing complex game object lists, mastering arrays of vectors will expand your programming capabilities and enhance your efficiency.
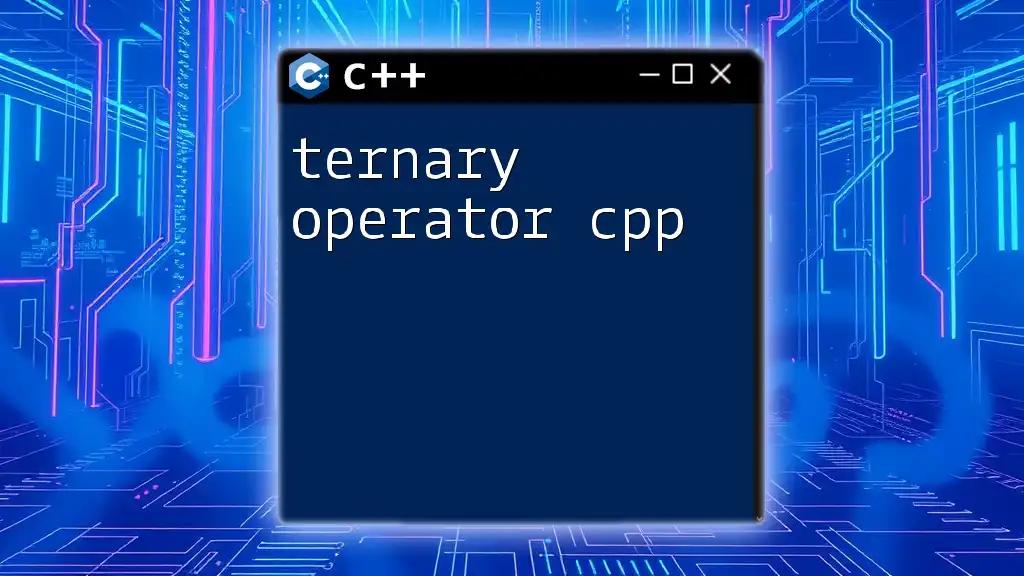
Additional Resources
For further exploration, consider referring to the official C++ documentation or online coding platforms that offer interactive learning modules. Additionally, books focused on C++ programming will deepen your understanding and introduce you to more advanced concepts, such as templates and advanced data structures.