The `std::vector` in C++ is a dynamic array that can resize itself automatically as elements are added or removed, providing flexibility in memory management and data manipulation.
Here's a simple example of using `std::vector` to store and display integers:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
What is std::vector?
`std::vector` is a part of the Standard Template Library (STL) in C++, serving as a dynamic array. Unlike traditional arrays, vectors can change in size, adapting as elements are added or removed. This flexibility is crucial for developers who need to manipulate datasets without worrying about memory allocation details.
When evaluating STL containers, vectors stand out for their ease of use and powerful functionalities. For example, while arrays have a fixed size, vectors can grow or shrink dynamically, making them more adaptable. Lists and other containers may offer different performance characteristics, but `std::vector` often excels in terms of speed for most indexing operations due to its contiguous memory allocation.
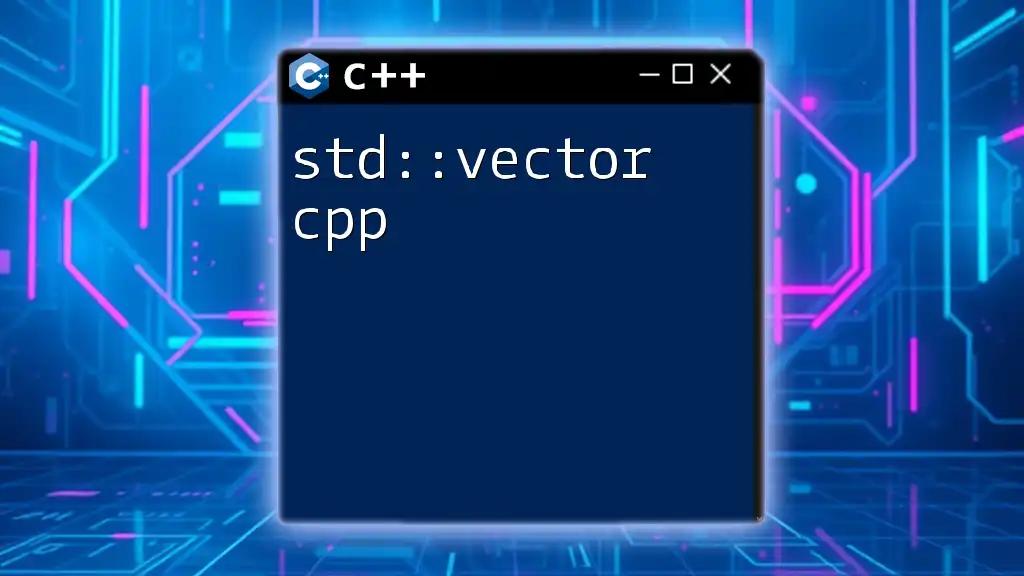
Benefits of Using std::vector
Using `std::vector` brings several advantages:
- Dynamic Sizing: Vectors automatically adjust their size. You do not have to specify an explicit size upfront, which can save time and reduce errors.
- Contiguous Memory Allocation: Elements are stored in a contiguous block of memory, which can improve cache performance during iteration.
- Rich Functionality: Vectors provide a variety of member functions such as sorting, modifying, and accessing elements, all of which contribute to cleaner and more efficient code.
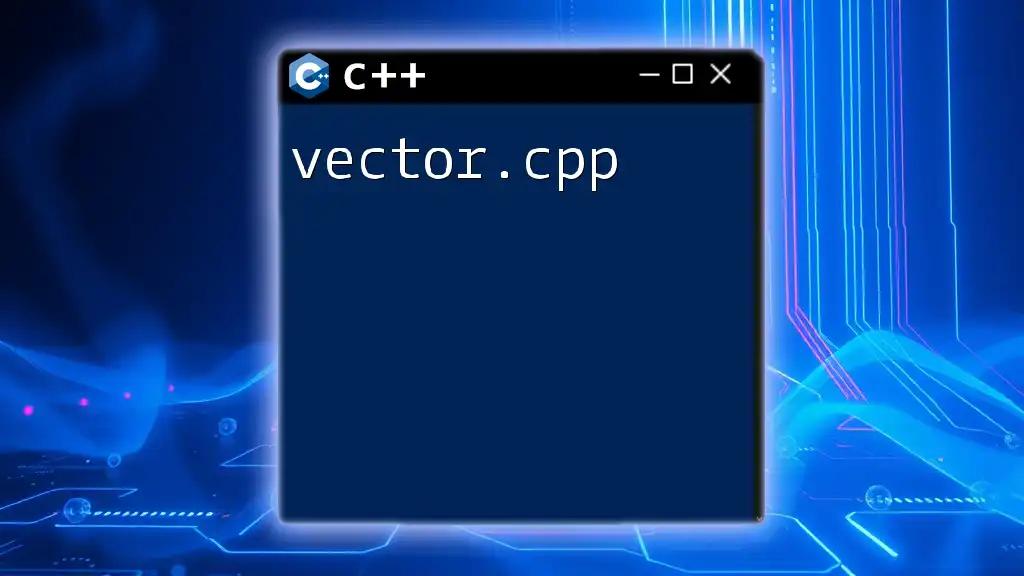
Creating a std::vector
Declaring and Initializing Vectors
Declaring a vector is straightforward. The syntax is as follows:
std::vector<int> myVector; // Declaration
std::vector<int> myVector = {1, 2, 3}; // Initialization
In the initialization example, the vector is populated with three integers. You can create vectors for various data types, including strings or custom user-defined types.
Specifying Size and Default Values
You can specify the size of a vector during declaration and set default values for each element. The following example demonstrates this:
std::vector<int> myVector(5, 10); // Five elements, all initialized to 10
In this code, a vector with five elements is created, each initialized to the value of 10. This feature is particularly useful when you want a vector pre-filled with placeholder values.
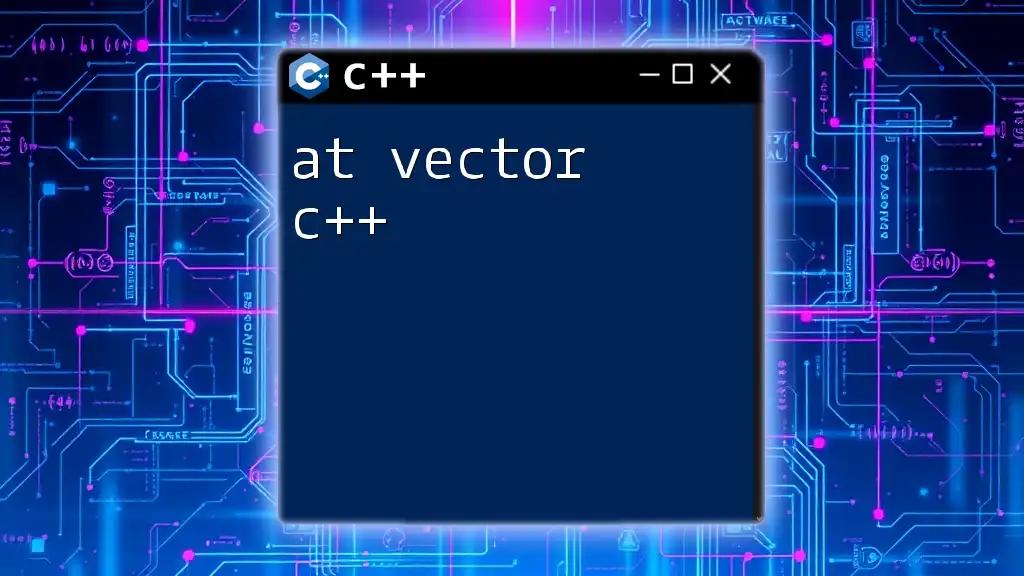
Accessing Elements in std::vector
Using the `at()` Method
The `at()` method provides safe access to vector elements, including bounds checking. This means that if you try to access an index out of range, it throws an `out_of_range` exception, helping you catch errors.
std::vector<int> myVector = {1, 2, 3};
std::cout << myVector.at(1); // Outputs: 2
This approach is beneficial in production code, where error prevention is crucial.
Using the Index Operator []
Although convenient, the index operator `[]` doesn't perform bounds checking. Using it on an out-of-bounds index results in undefined behavior, which can cause difficult-to-diagnose bugs.
std::cout << myVector[3]; // Undefined behavior if out of range
While this method is faster, it is advisable to use it carefully.
Iterating Over Elements
Iterating through a vector is simple and can be accomplished in multiple ways. The range-based for loop is modern and expressive:
for (auto& val : myVector) {
std::cout << val << " ";
}
Alternatively, a traditional for loop can give you more control over the index:
for (size_t i = 0; i < myVector.size(); ++i) {
std::cout << myVector[i] << " ";
}
Both methods effectively allow you to access each element in the vector.
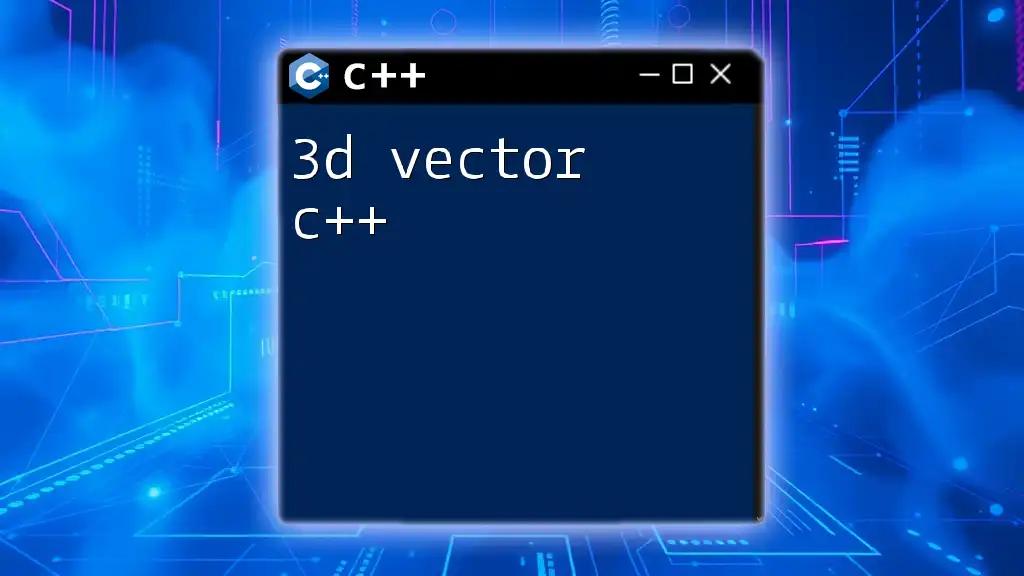
Modifying std::vector
Adding Elements
Adding new elements can be done with the `push_back()` method. This adds an element to the end of the vector, dynamically increasing its size.
myVector.push_back(4);
Inserting Elements
If you need to insert an element at a specific position, you can use the `insert()` method.
myVector.insert(myVector.begin() + 1, 5); // Inserts 5 at index 1
This versatility makes it easy to tailor the data structure to your requirements.
Removing Elements
Removing elements is equally straightforward. You can use the `pop_back()` method to remove the last element or employ `erase()` to remove elements at specific indices.
myVector.pop_back(); // Removes last element
To clear the entire vector, `clear()` can be invoked, erasing all elements and resetting its size to zero.
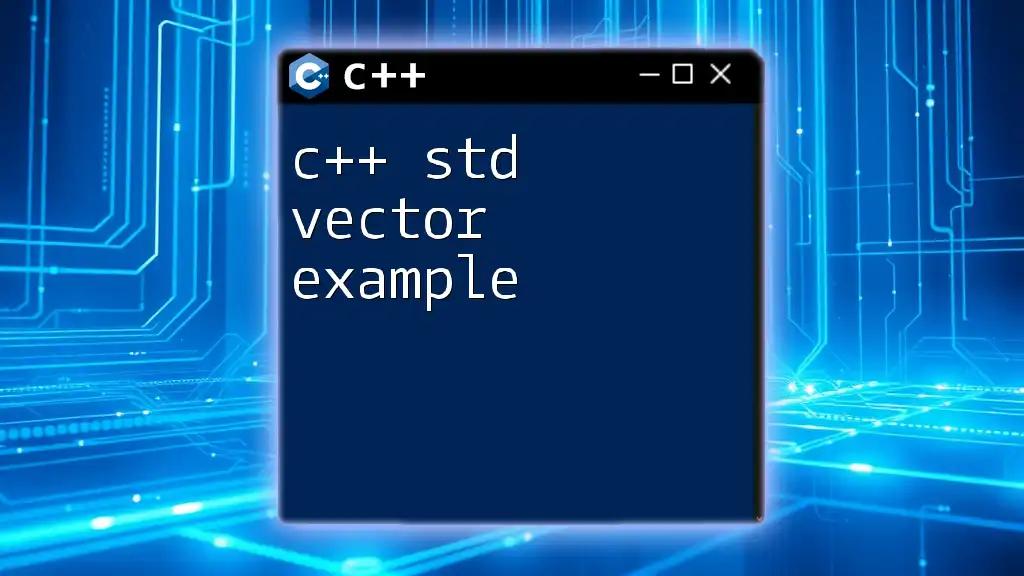
Resizing and Capacity
Resizing a Vector
Vectors can be resized on-demand using the `resize()` method. This may increase the size of the vector or reduce it, filling new elements with default values if necessary.
myVector.resize(10); // Increases size, defaults to 0 for new elements
Capacity Management
Understanding vector capacity is fundamental. The `size()` method gives the current number of elements, while `capacity()` indicates the total available space. You can also call `shrink_to_fit()` to reduce capacity to match size and free up unused memory.
std::cout << "Size: " << myVector.size() << ", Capacity: " << myVector.capacity();
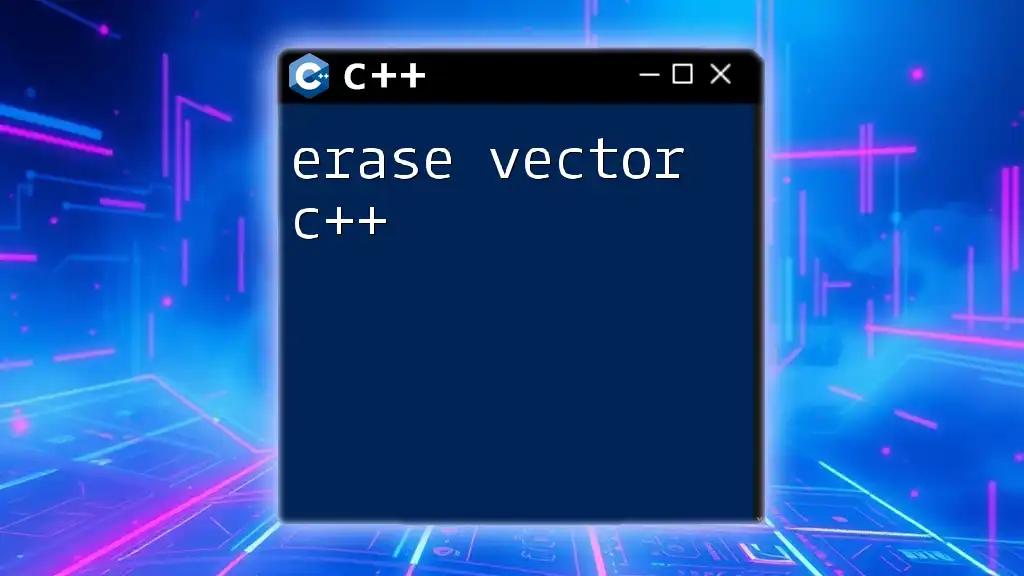
Common Operations
Sorting a Vector
Vectors can be sorted using the `std::sort()` function from the `<algorithm>` header. This function provides an efficient way to organize elements.
#include <algorithm>
std::sort(myVector.begin(), myVector.end());
Finding Elements
To find an element efficiently, utilize `std::find()`, which returns an iterator pointing to the element or to the end if not found.
auto it = std::find(myVector.begin(), myVector.end(), 2);
Modifying Elements
To modify all elements easily, consider `std::for_each`, which allows you to apply a function to every element in the vector.
std::for_each(myVector.begin(), myVector.end(), [](int& n) { n += 1; });
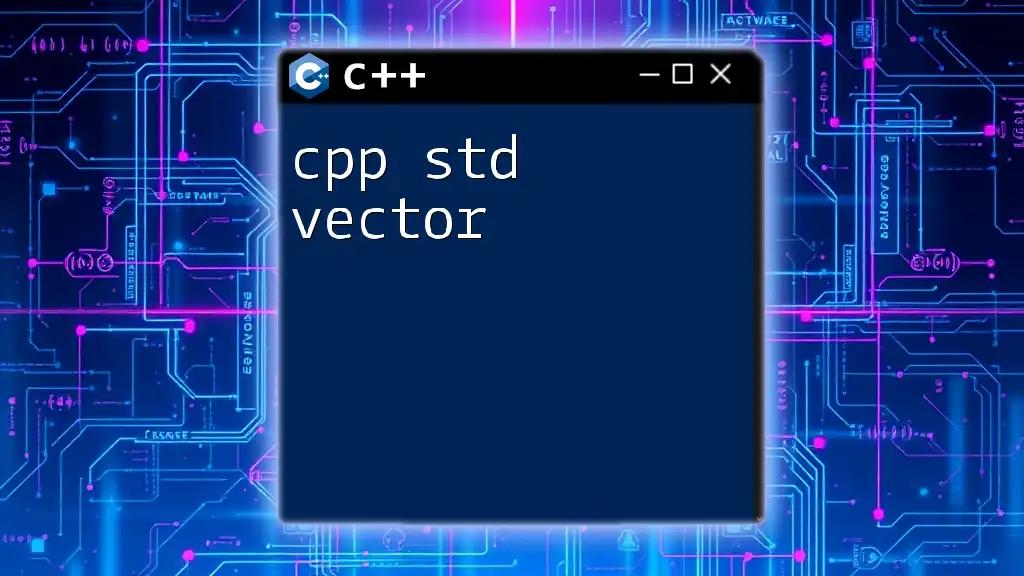
Advanced Topics
Passing std::vector to Functions
When passing vectors to functions, it's essential to consider whether to pass by reference or by value. Passing by reference (`std::vector<int>& vec`) allows for modifications without copying the entire vector, enhancing performance.
void processVector(std::vector<int>& vec);
Nested std::vector
Using nested vectors allows for the creation of multi-dimensional data structures, commonly used in applications like matrices.
To create a 2D vector:
std::vector<std::vector<int>> matrix(3, std::vector<int>(4));
Each element corresponds to a row in the matrix.
Performance Considerations
Each operation on `std::vector` has distinct time complexities. Preparing for performance concerns is vital. For instance, inserting at the beginning can be costly (O(n)), while accessing elements is O(1). Thus, understanding your use case is essential to make the most optimal choices.
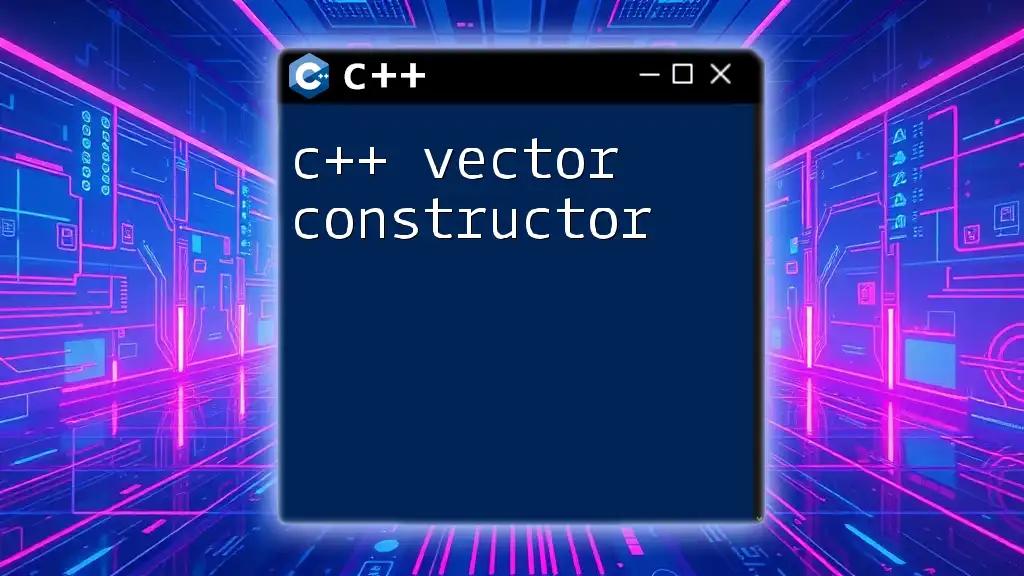
Conclusion
Mastering `std::vector` in C++ is invaluable for modern programming. Its flexibility, ease of use, and built-in functionalities make it an excellent data structure for managing dynamic arrays. By grasping how to initialize, manipulate, and access vectors efficiently, you lay a solid foundation for developing robust C++ applications.
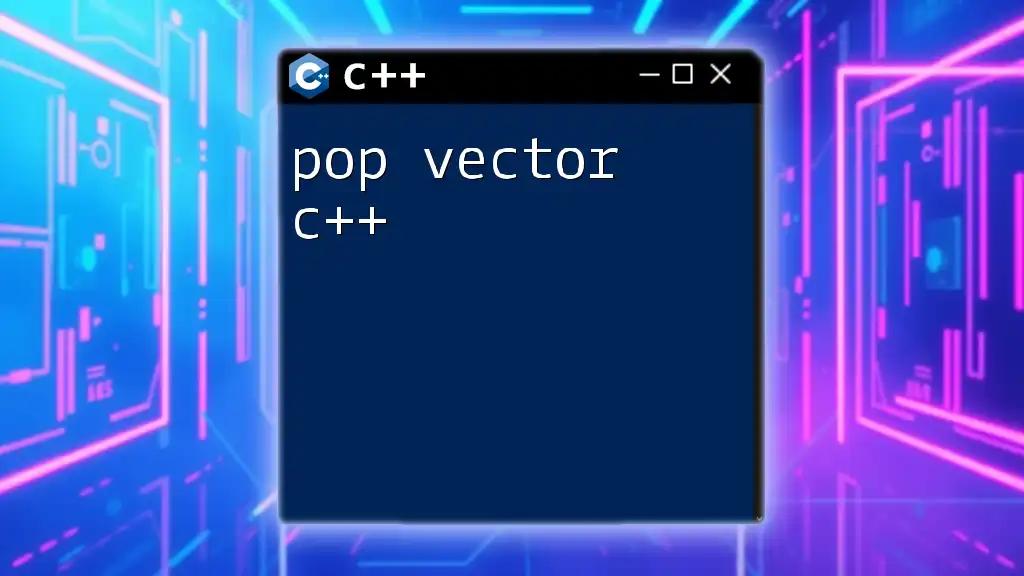
Additional Resources
For further deep dives, consult the official documentation of the C++ STL or engage with complementary books and online courses focused on mastering C++ and its data structures.
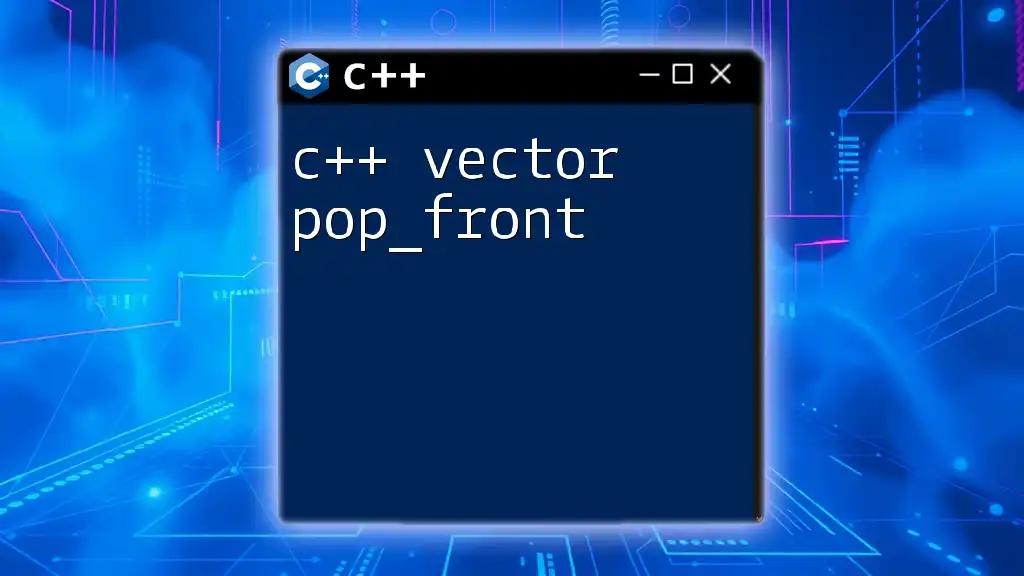
FAQ Section
Explore common questions developers encounter while using `std::vector`. Understanding these can help troubleshoot common errors effectively.