In C++, appending an element to a `std::vector` can be accomplished using the `push_back()` method, which adds the specified value to the end of the vector.
#include <vector>
std::vector<int> numbers;
numbers.push_back(10); // Appending the value 10 to the vector
What is a C++ Vector?
Definition of a C++ Vector
A C++ vector is part of the Standard Template Library (STL) and is a dynamic array that can grow and shrink in size as elements are added or removed. Unlike static arrays, vectors provide greater flexibility as they can adapt their size during runtime. This makes vectors a highly useful data structure when working with collections of data whose size can change.
Key Properties of Vectors
Vectors possess several properties that contribute to their versatility:
- Dynamic Sizing: Unlike fixed-size arrays, vectors automatically resize themselves when elements are added or removed.
- Memory Management: Vectors manage their own memory, which simplifies code management for developers.
- Type Safety: Vectors are strongly typed and can store elements of a single type, enhancing type safety and reducing errors.

Understanding Vector Append in C++
What Does It Mean to Append to a Vector?
Appending to a vector refers to the process of adding new elements to the end of the vector. This operation is crucial for data manipulation, allowing programmers to manage collections of data efficiently. With vectors, appending doesn't necessitate the manual resizing needed with traditional arrays.
Benefits of Using Vectors for Appending
The use of vectors for appending offers an array of advantages:
- Flexibility of Storage: Vectors automatically expand to accommodate new elements, eliminating the need to allocate additional memory manually.
- Efficient Memory Management: When a vector reaches capacity, it typically allocates more memory (often double the size) to minimize the frequency of reallocations, leading to efficient memory handling.
- Ease of Use: With intuitive member functions like `push_back()` and `insert()`, appending elements becomes a straightforward task.

C++ Vector Append Methods
Using `push_back()`
The `push_back()` function is a straightforward method for appending elements to the end of a vector. This method does not require you to specify the index or handle resizing manually, as the vector handles this internally.
Example:
#include <iostream>
#include <vector>
int main() {
std::vector<int> myVector;
myVector.push_back(10);
myVector.push_back(20);
std::cout << "Vector contents: ";
for (int i : myVector) {
std::cout << i << " ";
}
return 0;
}
In the example above, the vector starts empty. The `push_back()` method adds 10 and then 20 to the end. The resulting output demonstrates how the vector contains the appended values: 10 20.
Using `insert()`
The `insert()` method provides more flexibility, allowing you to insert an element at any position or a range of elements from one vector into another.
Example:
#include <iostream>
#include <vector>
int main() {
std::vector<int> myVector = {1, 2, 3};
myVector.insert(myVector.end(), 4);
std::cout << "Vector contents: ";
for (int i : myVector) {
std::cout << i << " ";
}
return 0;
}
This code appends 4 to the end of the vector initialized with elements 1, 2, and 3. The output will show that the vector now contains: 1 2 3 4. Using `insert()` allows you to specify `myVector.end()`, meaning you are appending just as you would with `push_back()`, but with more options available.
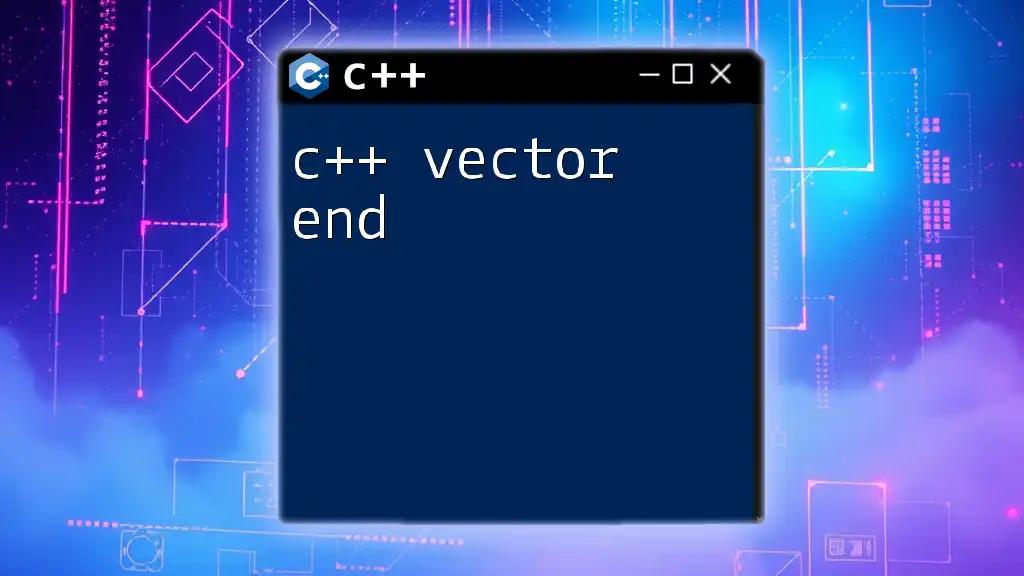
Appending a Vector to Another Vector
Using `insert()` for Vector to Vector Append
You can easily append one vector to another using the `insert()` method. This is particularly useful when you want to merge datasets.
Example:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vector1 = {1, 2, 3};
std::vector<int> vector2 = {4, 5, 6};
vector1.insert(vector1.end(), vector2.begin(), vector2.end());
std::cout << "Combined Vector: ";
for (int i : vector1) {
std::cout << i << " ";
}
return 0;
}
In this case, elements from vector2 are appended to vector1. The output displays the merged vector: 1 2 3 4 5 6. The important takeaway here is that by specifying the beginning and end of vector2, you can control which elements you wish to append.
Using `insert()` with Range
In certain scenarios, you may want to append only a specific subset of elements from a vector. The `insert()` function allows you to do this easily.
Example:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
std::vector<int> toAppend = {6, 7, 8};
vec.insert(vec.end(), toAppend.begin(), toAppend.begin() + 2); // Appends only first two elements
for (int i : vec) {
std::cout << i << " ";
}
return 0;
}
In the example above, only the first two elements from toAppend (i.e., 6 and 7) are appended to vec. The final output reveals the contents of vec: 1 2 3 4 5 6 7. Utilizing ranges allows you to have tight control over which elements to append, enhancing efficiency and customization.
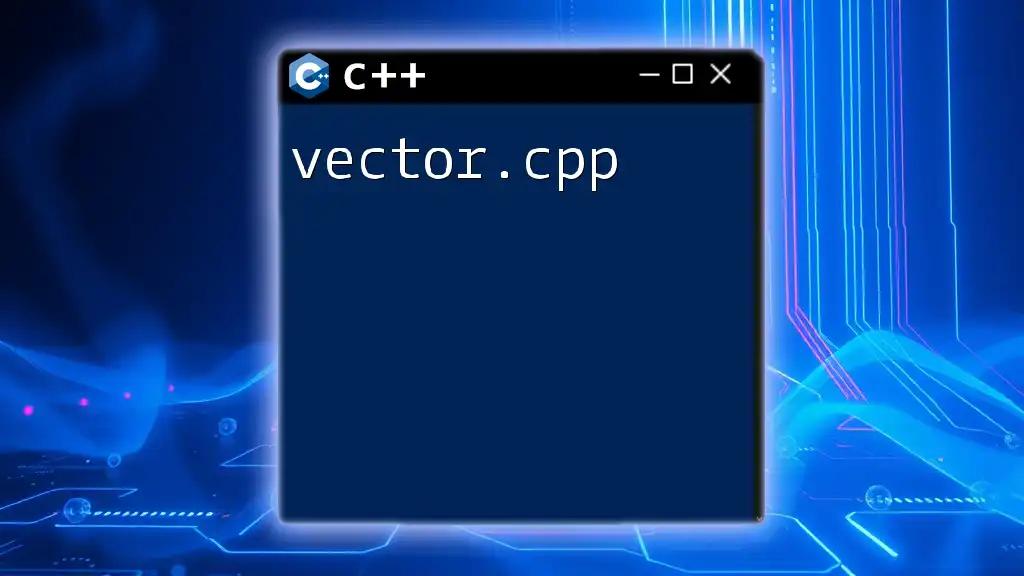
Performance Considerations
Efficiency of Vector Append Operations
When considering vector append operations, it is essential to understand their time complexity. The `push_back()` operation generally runs in O(1) time unless the vector needs to reallocate memory, in which case it runs in O(n) (where n is the current size of the vector). The `insert()` function typically has a time complexity of O(n) since it may need to shift elements to accommodate the new ones.
Best Practices for Efficient Appending
To maximize efficiency:
- Batch Insertions: If anticipating adding many elements at once, consider reserving memory in advance using the `reserve()` function. This approach minimizes costly reallocations.
- Minimize Reallocation: Call `reserve()` with a generous estimate of the total number of elements needed to avoid growing beyond capacity frequently.
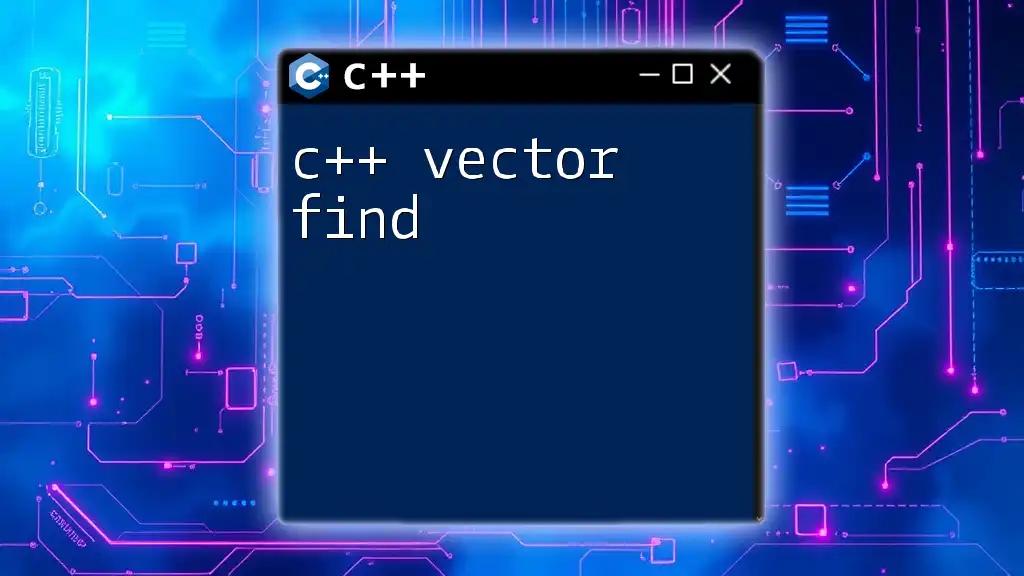
Conclusion
Mastering how to perform a cpp vector append is a vital skill in C++ programming. Understanding the various methods to append elements and the performance implications can significantly impact your program's efficiency. By effectively utilizing vectors’ flexibility, you can streamline your data management processes. Remember to practice these techniques regularly to get comfortable with manipulating vectors in your projects.
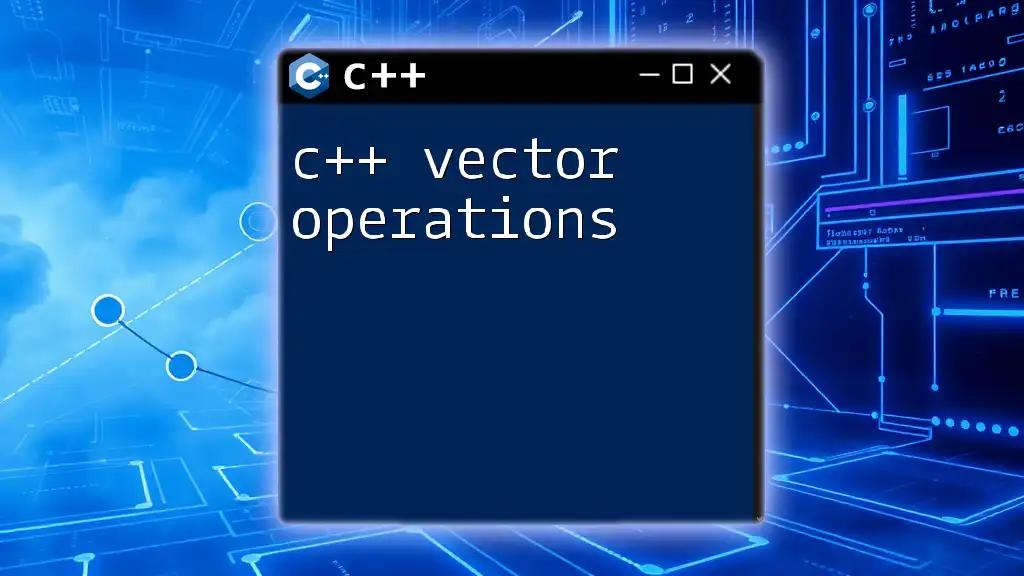
Call to Action
If you found this guide helpful, consider subscribing for more insightful C++ tutorials. Joining programming forums can also enhance your learning journey, allowing you to ask questions, share knowledge, and connect with other developers passionate about C++.

Additional Resources
For further reading on C++ vectors and the Standard Template Library (STL), consider consulting the official C++ documentation and exploring community resources available online.