In C++, the `std::sort` function in combination with vectors allows you to easily sort elements in a vector in ascending order.
Here’s a code snippet demonstrating how to sort a vector of integers:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {5, 2, 8, 1, 4};
std::sort(vec.begin(), vec.end());
for (int n : vec) {
std::cout << n << " ";
}
return 0;
}
Introduction to C++ Vector Sort
Understanding Vectors in C++
In C++, a vector is a dynamic array provided by the Standard Template Library (STL). `std::vector` enables developers to manage collections of data efficiently, as it can resize automatically when elements are added or removed. This versatility makes vectors a preferred choice for handling lists of data where the size may change.
What is Sorting?
Sorting is a fundamental operation in computer science where data elements are arranged in a specific order—usually in ascending or descending order. This task is essential for optimizing data retrieval processes and enhancing the performance of search algorithms. A variety of sorting algorithms exist, such as Quick Sort, Merge Sort, and Bubble Sort. However, for C++ vector sort, we primarily utilize the built-in STL sorting functions, which provide robust performance with convenience.
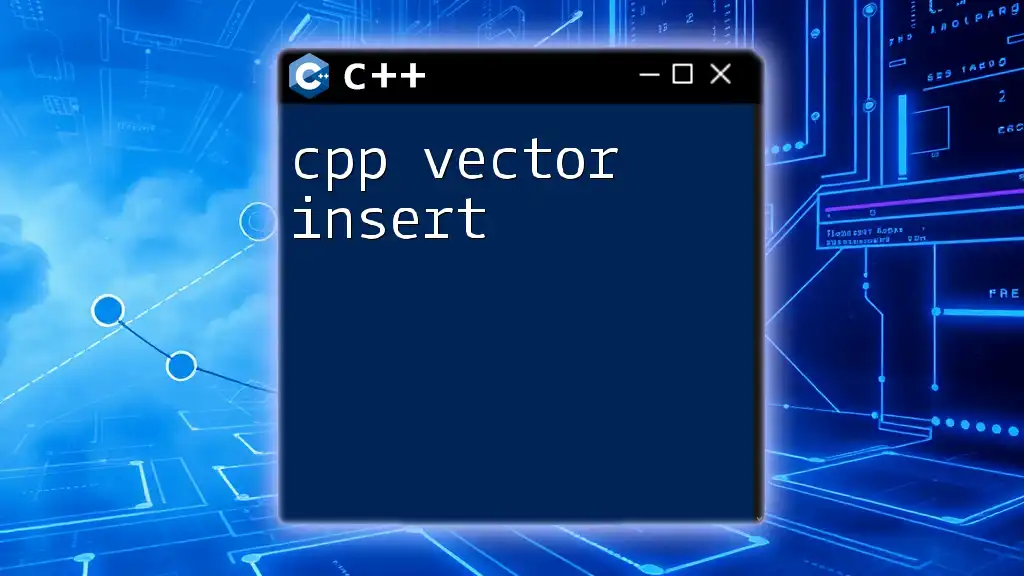
Overview of std::sort in C++
What is std::sort?
`std::sort` is a built-in function in the C++ Standard Library that efficiently sorts a range of elements using the IntroSort algorithm, which combines Quick Sort, Heap Sort, and Insertion Sort. This blend achieves optimal performance across various data sizes and distributions.
Basic Syntax of std::sort
The basic syntax for using `std::sort` requires specifying the range of elements to sort, defined by two iterators, and an optional comparison function.
For example:
#include <vector>
#include <algorithm> // For std::sort
...
std::vector<int> vec = {3, 1, 4, 1, 5};
std::sort(vec.begin(), vec.end());
In this example, `vec.begin()` and `vec.end()` specify the start and end of the vector to be sorted. By default, `std::sort` arranges the elements in ascending order.
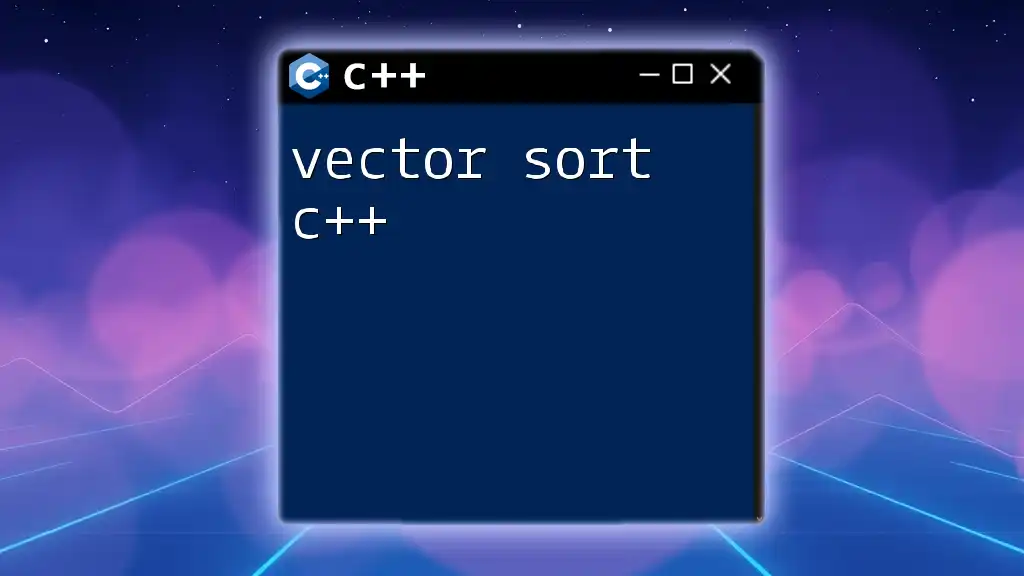
How to Sort a Vector in C++
Sorting a Numeric Vector
Sorting a vector of numbers is straightforward. The `std::sort` function's default behavior sorts numbers in ascending order. Consider the following example:
std::vector<int> numbers = {5, 2, 9, 1, 5, 6};
std::sort(numbers.begin(), numbers.end());
// Output: 1, 2, 5, 5, 6, 9
In this code, the vector `numbers` is sorted in place, with the values rearranged from least to greatest.
Sorting a Vector of Strings
Sorting a vector of strings works similarly. The `std::sort` function compares strings based on lexicographical order (dictionary order). Here's an example:
std::vector<std::string> fruits = {"banana", "apple", "cherry"};
std::sort(fruits.begin(), fruits.end());
// Output: apple, banana, cherry
After executing `std::sort`, the `fruits` vector is sorted, with `"apple"` appearing first due to its lexicographical precedence.
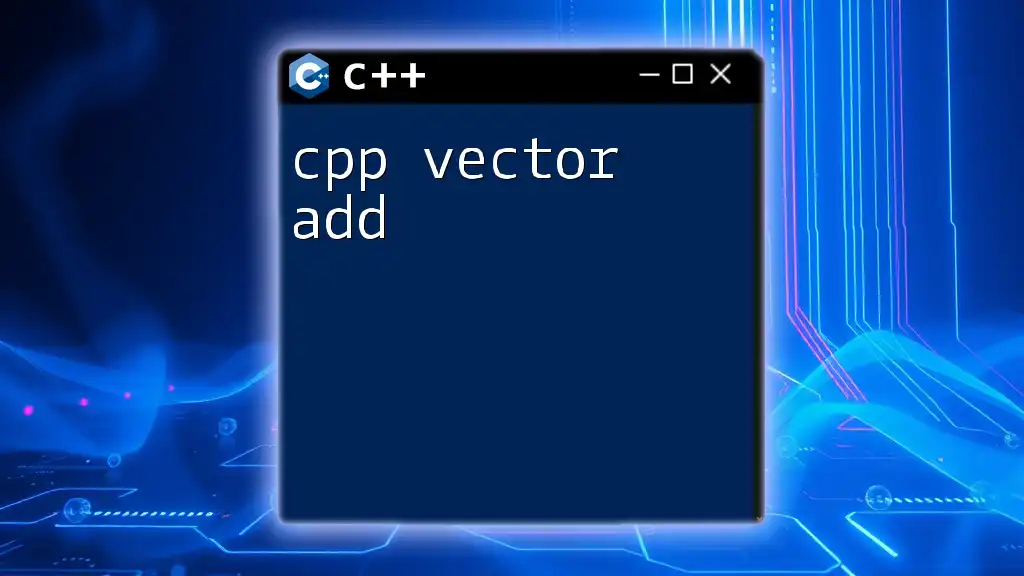
Custom Sorting with std::sort
Using a Custom Comparator
In some cases, the default sorting order might not meet your requirements. When you need a different sorting criterion, you can use a custom comparator function. Here's an example of sorting integers in descending order:
auto descending = [](int a, int b) { return a > b; };
std::sort(numbers.begin(), numbers.end(), descending);
This code snippet defines a lambda function that specifies the order, allowing flexibility in how elements are sorted.
Sorting Complex Data Types
Vectors can also store complex data types like structs or classes. When sorting such vectors, you can define custom comparators that reference the structure's fields. For example:
struct Person {
std::string name;
int age;
};
std::vector<Person> people = { {"Alice", 30}, {"Bob", 25} };
std::sort(people.begin(), people.end(), [](const Person &a, const Person &b) {
return a.age < b.age;
});
In this code, the vector `people` is sorted by age, allowing for clarity when handling more intricate data types.
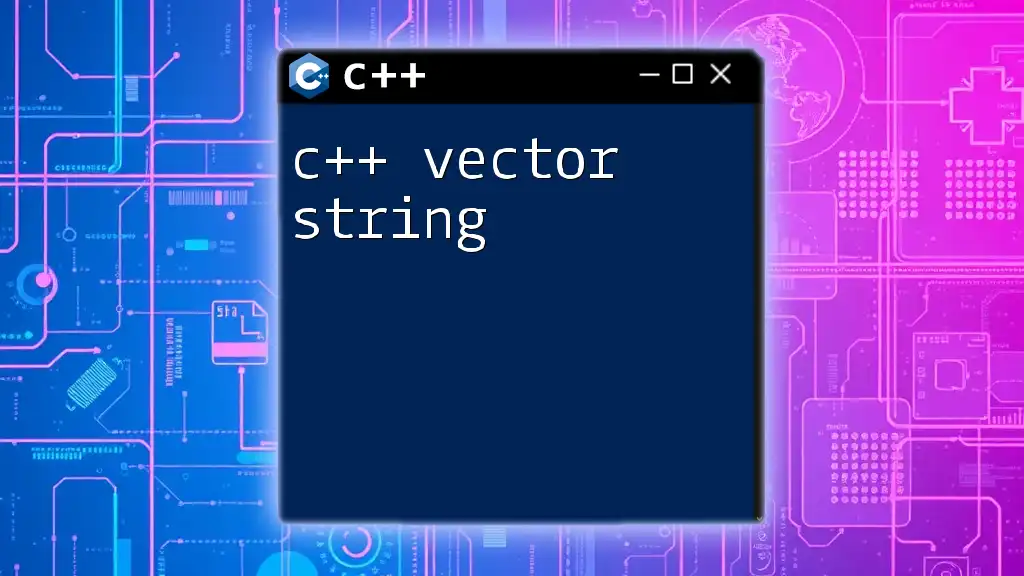
Best Practices for C++ Vector Sorting
Choosing the Right Sort Algorithm
Although `std::sort` is typically reliable, knowing when to prefer one algorithm over another is crucial for optimizing performance. `std::sort` is generally efficient for small to medium-sized datasets. For larger collections that require a stable output, consider `std::stable_sort`.
Stability of Sorting
Sorting stability refers to maintaining the relative order of records with equal keys. In scenarios where this attribute is critical, prefer `std::stable_sort`, which guarantees that equal elements retain their original order. This is particularly useful when sorting multi-field datasets.
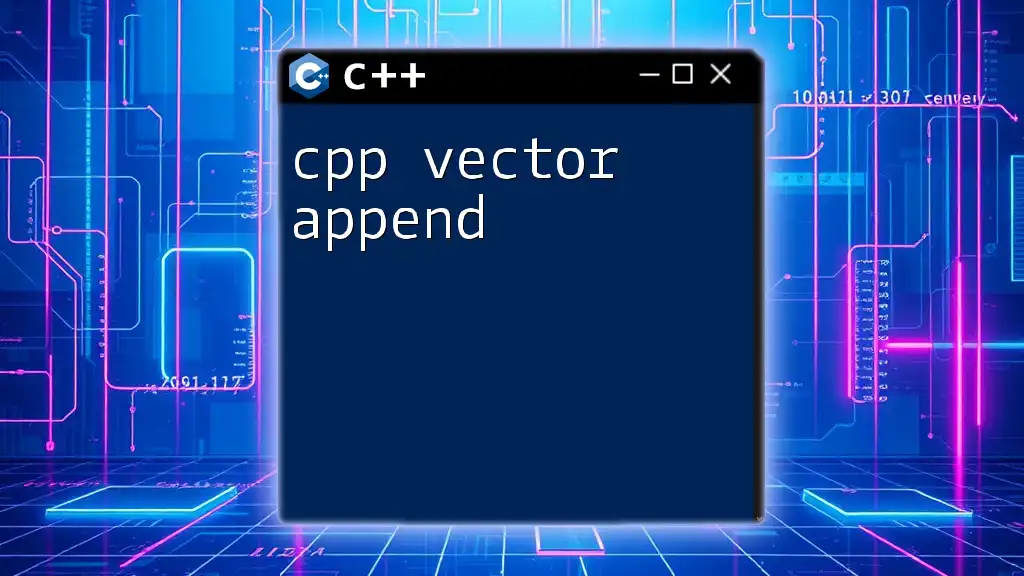
Advanced Sorting Techniques
Sorting with std::stable_sort
For cases where stable sorting is required, use `std::stable_sort`. Here’s how it can be applied:
std::stable_sort(people.begin(), people.end(), [](const Person &a, const Person &b) {
return a.name < b.name;
});
By employing `std::stable_sort`, you ensure that the order of individuals sharing the same name remains unchanged after sorting.
Multi-criteria Sorting
When it comes to sorting by multiple fields, you can define a more complex comparator. For instance, sorting by age and then by name can be accomplished with the following code:
std::sort(people.begin(), people.end(), [](const Person &a, const Person &b) {
return a.age < b.age || (a.age == b.age && a.name < b.name);
});
This approach enables you to make sophisticated arrangements based on multiple attributes.
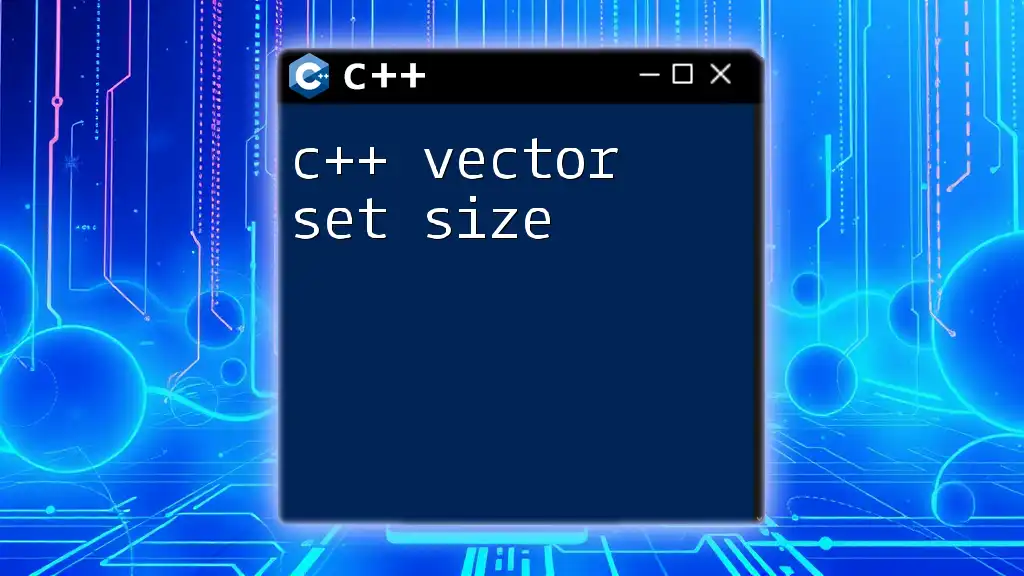
Debugging and Performance Tuning
Common Issues with Sorting Vectors
When using `std::sort`, one common issue may arise from incorrect iterator ranges. It’s crucial to check that the iterators are well-defined; otherwise, you might encounter runtime errors or unexpected results.
Performance Tips
To improve sorting speed, ensure that your data sizes suit the algorithm. For large datasets, consider pre-sorting operations or moving data into a more suitable structure, like a linked list, if frequent insertions or deletions are expected.
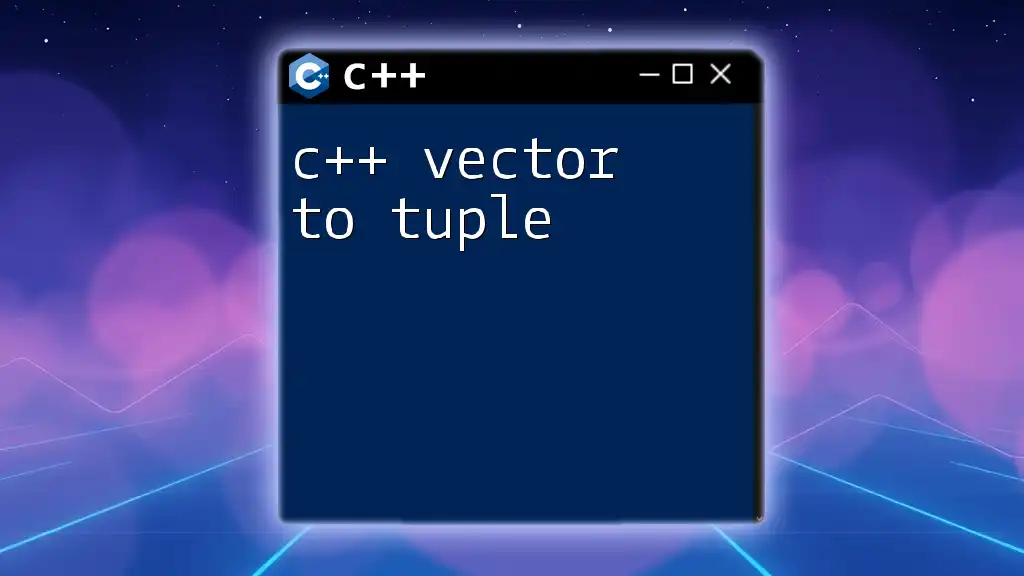
Conclusion
Sorting vectors in C++ is essential for efficient data manipulation and retrieval. Mastering the techniques of `cpp vector sort` empowers you to handle datasets more effectively, whether through simple numeric sorts or complex multi-field custom arrangements. As you dive deeper into sorting algorithms and their applications, continue exploring the vast resources available in the C++ community to keep refining your skills.