C++ performance can be significantly enhanced through efficient resource management, optimal algorithms, and minimizing memory overhead, as demonstrated in the following code snippet that utilizes pointers for faster data access.
#include <iostream>
void optimizePerformance(int* arr, int size) {
for (int i = 0; i < size; ++i) {
arr[i] *= 2; // Example of modifying array elements in place
}
}
int main() {
int arr[] = {1, 2, 3, 4, 5};
int size = sizeof(arr) / sizeof(arr[0]);
optimizePerformance(arr, size);
for (int i = 0; i < size; ++i) {
std::cout << arr[i] << " "; // Output: 2 4 6 8 10
}
return 0;
}
Understanding Performance Metrics
What is performance in C++? Performance refers to the efficiency of a C++ program in terms of its execution time and resource utilization. It is crucial to understand that performance can be affected by various factors, such as algorithms, data structures, memory management, and even compiler optimizations. High-performance applications often use C++ to get the most out of system resources.
Key Metrics to Measure Performance
-
Execution Time: The time taken by a program or a specific function to complete its tasks. Utilize tools such as `std::chrono` or `clock()` to measure execution time effectively.
-
Memory Usage: The amount of memory used by your program. Memory profiling is essential, as inefficient memory use can lead to slower performance. Tools like Valgrind can aid in identifying memory leaks and usage patterns.
-
CPU and Memory Bottlenecks: Identifying where your application is slowing down can significantly impact performance. Common bottlenecks can be in CPU cycles or memory bandwidth.
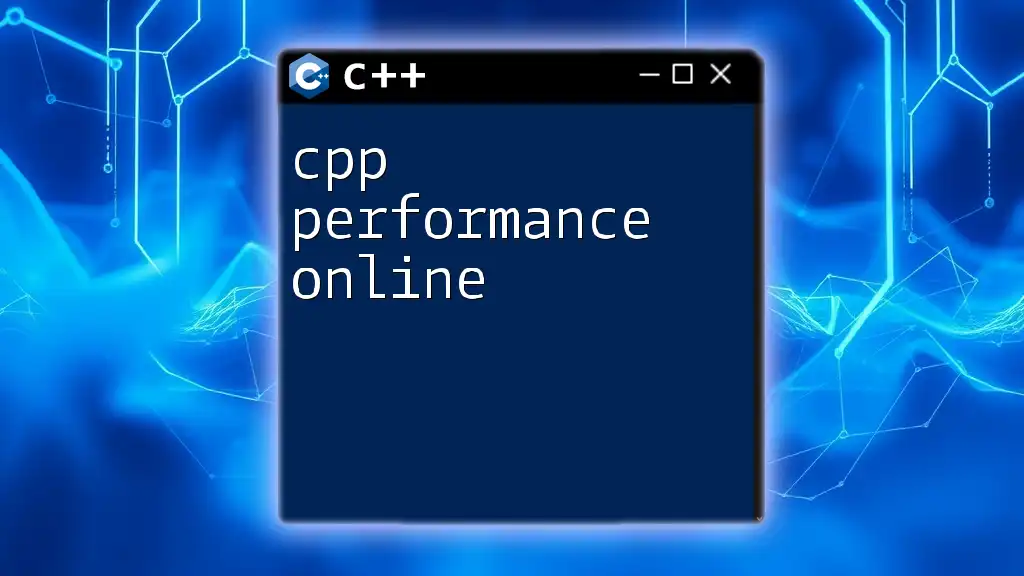
Code Efficiency
Writing efficient code is fundamental to achieving optimal C++ performance. What makes code efficient? It involves choosing appropriate data structures and leveraging algorithms that minimize resource consumption and maximize speed.
Choosing the Right Data Structures
Different data structures serve different needs, impacting performance:
-
`std::vector`: Excellent for dynamic arrays, with fast random access but inefficient insertions/deletions at arbitrary positions.
-
`std::list`: Suitable for frequent insertions/deletions, but slower for random access.
-
`std::map`: Efficient for key-value pair storage with log(n) search time, but more memory-intensive.
Example: Choosing Data Structures
In this example, we will test performance using `std::vector` and `std::list`:
#include <vector>
#include <list>
#include <chrono>
#include <iostream>
void vector_vs_list() {
const int size = 1000000;
// Measure time for std::vector
std::vector<int> vec;
vec.reserve(size);
auto start = std::chrono::high_resolution_clock::now();
for (int i = 0; i < size; ++i) {
vec.push_back(i);
}
auto end = std::chrono::high_resolution_clock::now();
std::cout << "Vector time: " << std::chrono::duration_cast<std::chrono::milliseconds>(end - start).count() << " ms\n";
// Measure time for std::list
std::list<int> lst;
start = std::chrono::high_resolution_clock::now();
for (int i = 0; i < size; ++i) {
lst.push_back(i);
}
end = std::chrono::high_resolution_clock::now();
std::cout << "List time: " << std::chrono::duration_cast<std::chrono::milliseconds>(end - start).count() << " ms\n";
}
Algorithm Analysis
Understanding the complexity of algorithms is crucial. Big O notation provides a high-level understanding of an algorithm's efficiency as input size grows.
For example:
- A linear search has a time complexity of O(n).
- A binary search, on the other hand, operates at O(log n) but requires a sorted array.
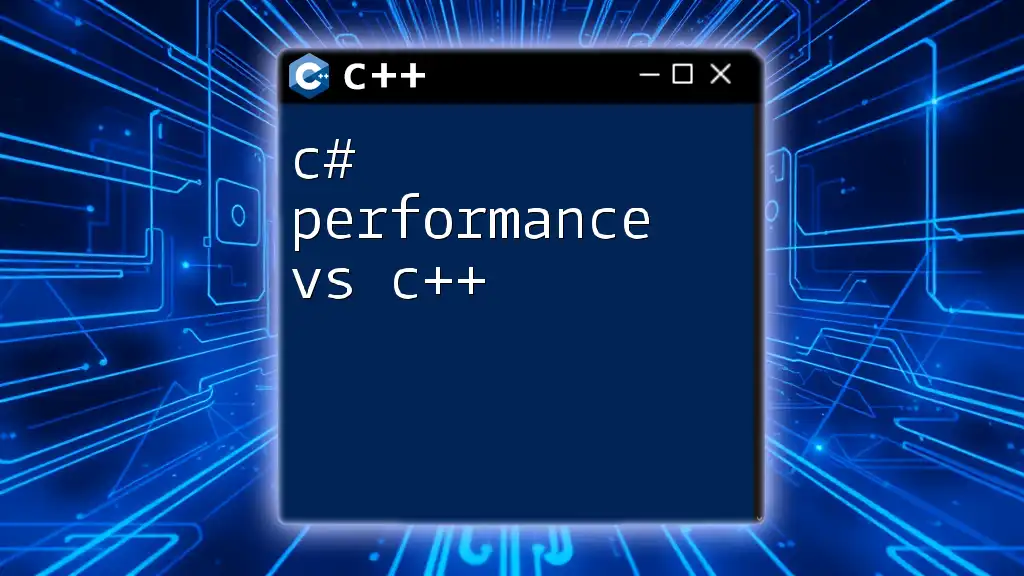
Memory Management
Efficient memory management optimizes C++ performance significantly. C++ allows both static and dynamic memory allocation.
Static vs. Dynamic Memory Allocation
Static allocation allocates memory at compile time, making it faster, whereas dynamic allocation, done at runtime using `new` and `delete`, is flexible but can introduce overhead and fragmentation.
Smart Pointers
Utilizing smart pointers, such as `std::unique_ptr` and `std::shared_ptr`, can help manage memory efficiently. They automatically handle memory deallocation, reducing the risk of memory leaks.
Example: Using Smart Pointers
#include <memory>
#include <iostream>
void smart_pointer_example() {
std::unique_ptr<int> ptr = std::make_unique<int>(42);
std::cout << "Value: " << *ptr << std::endl;
// Automatically deallocates memory when ptr goes out of scope
}
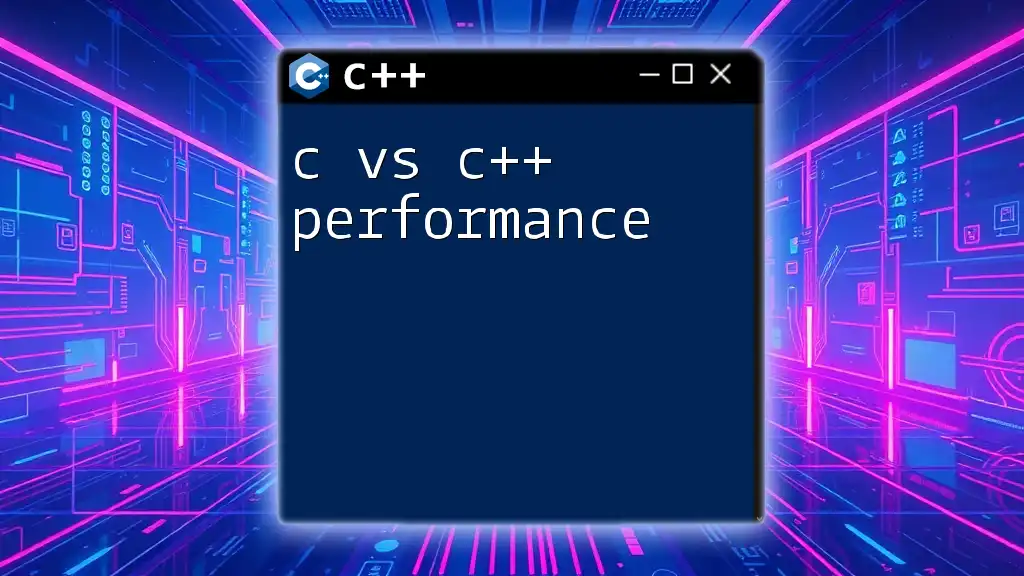
Compiler Optimization
Compiler optimizations are powerful tools for enhancing C++ performance. When compiling code, certain flags can significantly affect the final executable's efficiency.
Enabling Compiler Optimization
When you compile your C++ code, you can enable optimizations using flags like `-O2`, `-O3`, or `-Os`. Higher optimization levels can considerably reduce code execution time but may result in longer compile times.
In-depth Look at Compiler Optimizations
-
Loop Unrolling: This optimization technique reduces the overhead of loop control. By expanding the loop body, performance can be improved, especially in computationally intensive tasks.
-
Inlining Functions: Marking a function with the `inline` keyword allows the compiler to replace calls to that function with the function's body, reducing function-call overhead.
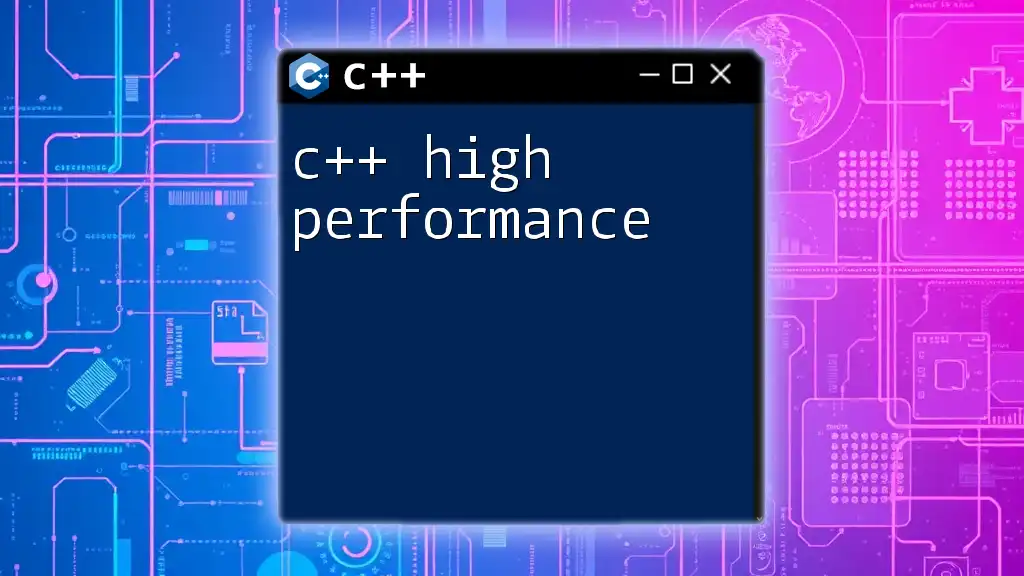
Multithreading and Concurrency
In today’s computing landscape, utilizing multiple cores is essential for maintaining C++ performance. Multithreading can lead to significant performance gains for applications that can perform parallel computing.
The Importance of Multithreading
Multithreading is ideal for tasks that can be divided into smaller, independent operations. For example, data processing tasks can often benefit from parallel execution.
C++ Standards and Threading Libraries
C++11 introduced a standard threading library, allowing developers to manage threads easily without needing low-level threading APIs. The `std::thread`, `std::async`, and `std::mutex` classes are crucial for developing concurrent applications.
Example: Basic Multithreading
#include <iostream>
#include <thread>
void printMessage() {
std::cout << "Hello from thread!" << std::endl;
}
void thread_example() {
std::thread myThread(printMessage);
myThread.join(); // Wait for thread to finish
}
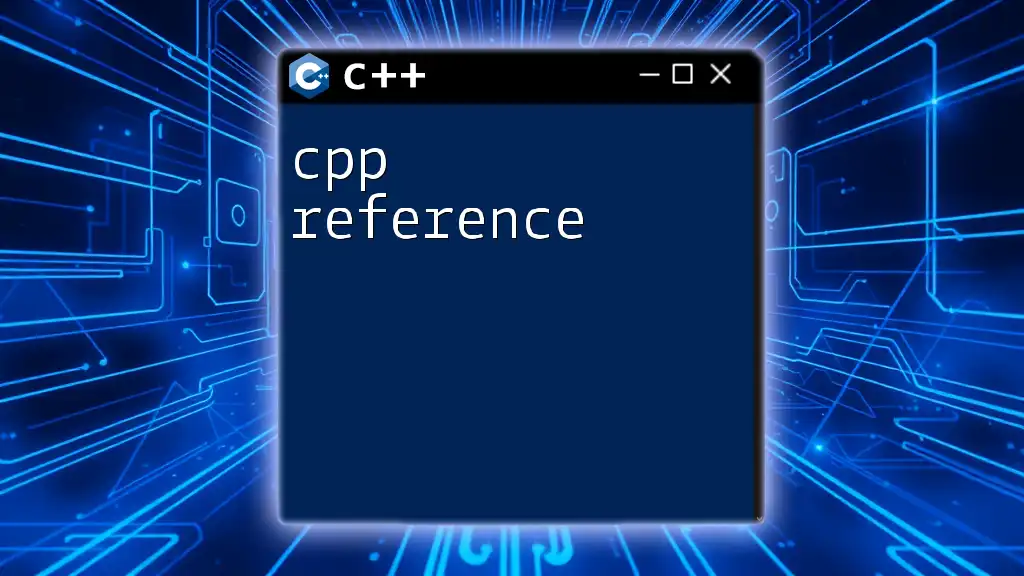
Profiling and Analysis
Profiling your application gives insights into where optimizations are needed. Various profiling tools, such as Valgrind, gprof, and Visual Studio Profiler, can identify performance bottlenecks.
Analyzing Performance Bottlenecks
Profiling tools will provide you with detailed metrics on function call durations, memory usage, and execution paths. Understanding these metrics is essential for making informed optimization decisions.
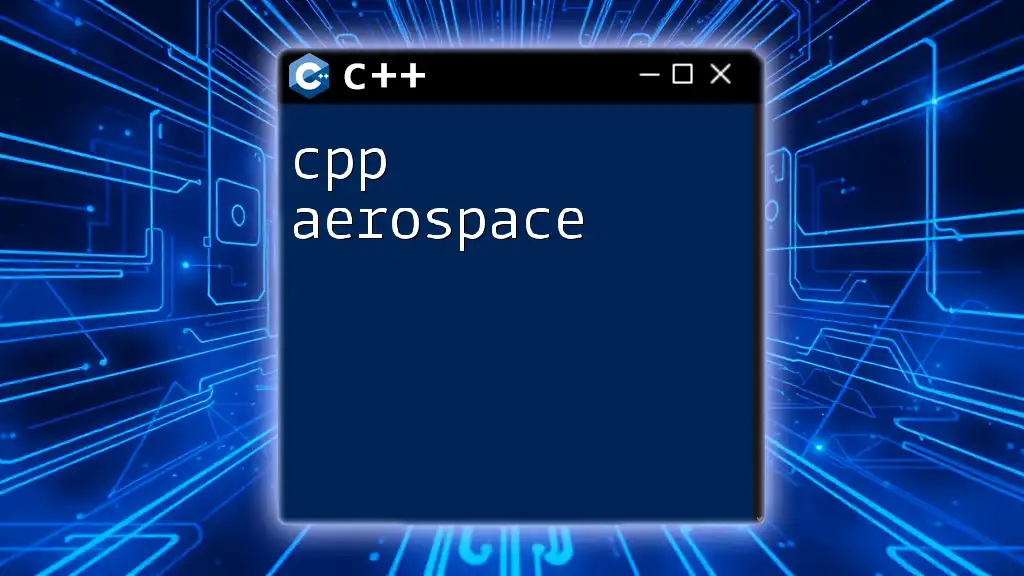
Advanced Performance Techniques
For developers looking to squeeze even more out of their C++ performance, advanced techniques such as template metaprogramming and inline assembly might come into play.
Template Metaprogramming
Template metaprogramming enhances performance by allowing computations to occur at compile time rather than runtime, yielding faster execution times in cases such as type manipulations.
Inline Assembly
While typically avoided, inline assembly can be used for performance-critical sections of code that require highly optimized machine instructions to achieve specific performance gains.
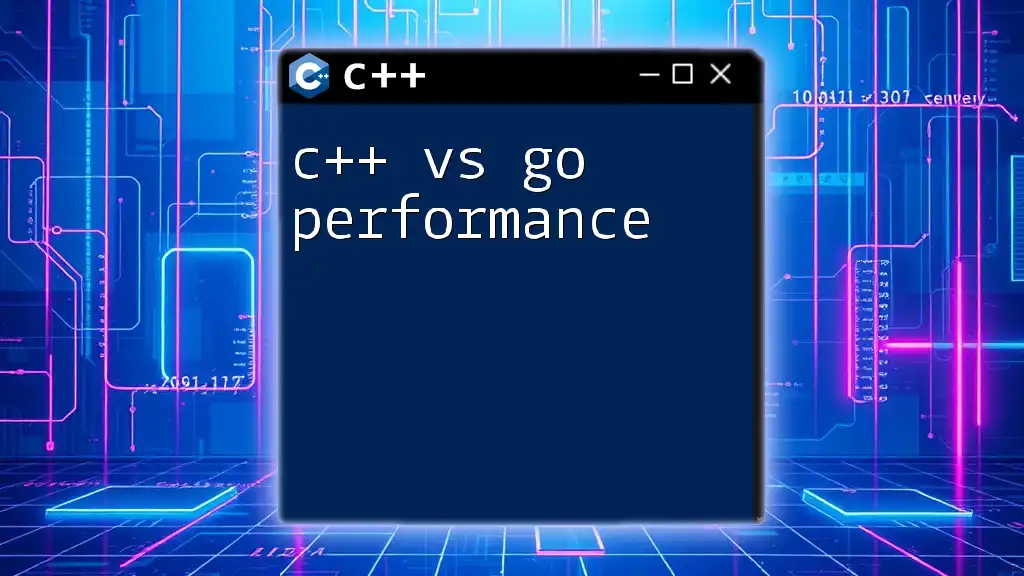
Conclusion
C++ performance is not just about writing code that works—it's about writing code that works efficiently. By understanding fundamental concepts, employing the right techniques, and using appropriate tools, C++ developers can enhance the performance of their applications significantly. Continuous learning and experimentation in performance optimization will yield better results in software development, leading to faster, more efficient applications.