C++ offers high-performance capabilities for financial systems by utilizing its efficient memory management and real-time processing features, which are crucial for tasks such as algorithmic trading and risk analysis.
Here’s a simple code snippet illustrating how to use C++ for a high-frequency trading strategy by executing a basic market order:
#include <iostream>
#include <string>
class MarketOrder {
public:
MarketOrder(const std::string& symbol, int quantity)
: symbol(symbol), quantity(quantity) {}
void execute() {
// Here we would connect to a trading API to execute the order
std::cout << "Executing market order for " << quantity << " shares of " << symbol << std::endl;
}
private:
std::string symbol;
int quantity;
};
int main() {
MarketOrder order("AAPL", 100);
order.execute();
return 0;
}
Understanding Financial Systems
What is a Financial System?
A financial system encompasses various institutions, instruments, and markets that facilitate the exchange of funds. It includes banks, stock exchanges, investors, and borrowers, working together to manage liquidity, risk, and capital across the economy. The efficiency and speed of transactions within this system are paramount; delays or inefficiencies can result in significant financial losses and missed opportunities.
The Role of C++ in Financial Systems
C++ has established itself as a leading programming language in the finance sector for several key reasons:
- Performance: With its low-level capabilities, C++ enables high-speed execution, which is critical in environments where milliseconds matter.
- Control: The language offers fine-grained control over system resources, allowing developers to optimize applications for performance.
- Versatility: Its extensive libraries and frameworks support various financial models and algorithms, ensuring C++ can handle diverse tasks.
When compared to other programming languages, such as Python or Java, C++ often provides superior performance, making it the language of choice for high-frequency trading (HFT) and risk management systems.

Key Features of C++ for High-Performance Applications
Memory Management
Efficient memory management is at the core of C++'s capability for high performance. The choice between manual and automatic memory management can drastically impact speed and resource use.
In C++, developers typically manage memory manually using `new` and `delete`, which provides greater flexibility but requires careful handling to avoid memory leaks. Here’s a simple example of dynamic memory allocation:
#include <iostream>
int main() {
int* arr = new int[100]; // Dynamic allocation
// Perform operations on arr
delete[] arr; // Memory deallocation
return 0;
}
Understanding and implementing efficient memory practices can lead to significant performance improvements in complex financial systems.
Multi-threading Capabilities
C++ offers robust support for multi-threading, enabling the simultaneous execution of operations. This is particularly beneficial in processing vast amounts of financial data where latency is detrimental.
Using threads in C++, developers can execute multiple tasks concurrently, thus improving throughput. Below is an example of how threads can be utilized to process transactions:
#include <iostream>
#include <thread>
void processTransaction(int transactionId) {
std::cout << "Processing transaction " << transactionId << std::endl;
}
int main() {
std::thread t1(processTransaction, 1);
std::thread t2(processTransaction, 2);
t1.join(); // Wait for t1 to finish
t2.join(); // Wait for t2 to finish
return 0;
}
By leveraging multi-threading, financial applications can enhance their processing capabilities, ultimately leading to faster decision-making and operational efficiency.
Performance Optimization Techniques
Compiler Optimizations
C++ compilers utilize several optimization techniques that can significantly enhance the performance of the final executable. Familiarizing oneself with optimization flags (e.g., `-O2`, `-O3` in GCC) can lead to a tangible performance increase, especially in compute-intensive applications.
Inlining Functions
Function inlining can improve performance by reducing the overhead of function calls, especially when dealing with small functions where call overhead is significant. By marking a function as `inline`, the compiler is encouraged to replace a function call with the actual function body.
Here's an example of an inline function:
inline int add(int a, int b) {
return a + b;
}
In real-world financial systems, inlining frequently-used functions can result in performance gains, particularly in calculations involving trading algorithms.

Design Patterns in C++
Understanding Common Design Patterns
Design patterns offer proven solutions for common problems in software design and can enhance clarity and performance in financial applications. Some notable patterns include:
- Singleton Pattern: Useful for managing shared resources, such as database connections or configuration settings.
- Factory Pattern: Facilitates the creation of complex objects, promoting code reuse and scalability.
- Observer Pattern: Beneficial for managing real-time data updates, especially in applications that rely on stock prices or trading signals.
Implementing Singleton Pattern
The Singleton pattern restricts the instantiation of a class, ensuring only one instance exists throughout the application's lifecycle. This is particularly useful in managing resources that should be shared, such as database connections.
Here’s how to implement a Singleton in C++:
class Database {
public:
static Database& getInstance() {
static Database instance; // Guaranteed to be destroyed, instantiated on first use
return instance; // Reference to the singleton instance
}
private:
Database() {} // Private constructor
};
By implementing the Singleton pattern, financial systems can maintain strict control over resource management, ensuring efficiency and consistency.

Real-World Applications
High-Frequency Trading (HFT)
In the realm of high-frequency trading, where speed is everything, C++ shines. Financial firms leverage C++ to create low-latency trading systems that can process thousands of transactions per second. The language’s performance capabilities allow traders to execute strategies faster than their competitors, capitalizing on fleeting market opportunities.
Risk Management Systems
Risk management relies heavily on complex financial models, simulations, and the ability to process large datasets efficiently. C++ is often employed for Monte Carlo simulations that assess risk by simulating numerous scenarios. Its speed ensures that quant models run effectively, providing critical insights for risk assessment and decision-making.

Practical Example: Building a Simple Financial Application
Overview of the Application
Let's explore building a basic account management application that encapsulates key features, such as depositing, withdrawing, and viewing an account balance. This example will serve to illustrate C++’s capabilities within a financial context.
Basic Structure of the Application
We begin with a simple class design for our bank account:
class BankAccount {
public:
void deposit(double amount) {
balance += amount;
}
void withdraw(double amount) {
if (amount <= balance) {
balance -= amount;
} else {
// Handle insufficient funds
}
}
double getBalance() const {
return balance;
}
private:
double balance = 0.0; // Initial balance set to zero
};
This class encapsulates the basic functionality needed for managing a bank account within a financial application. It emphasizes control over the account state and integrates safety checks for crucial operations like withdrawals.
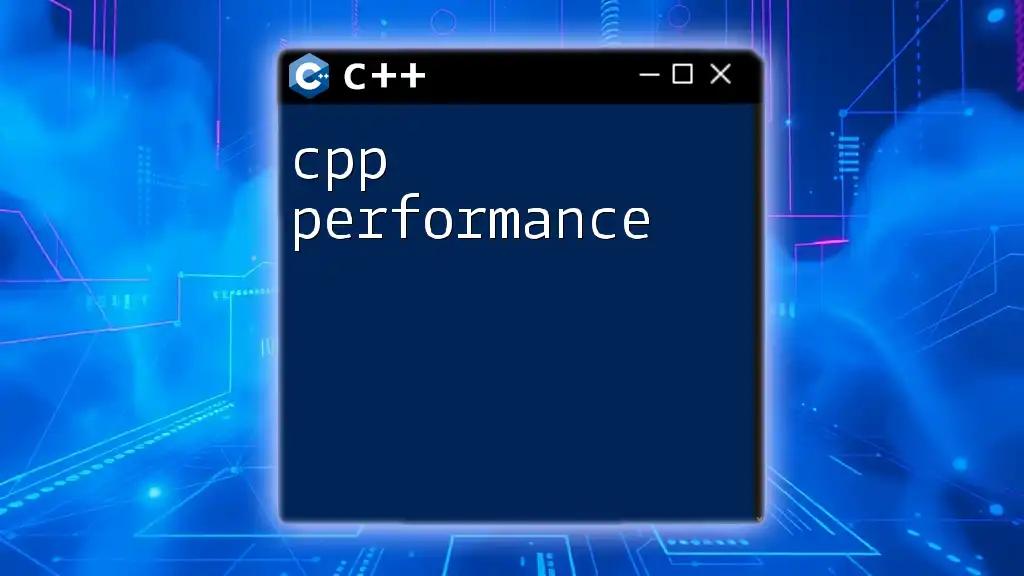
Testing and Validation
Importance of Testing in Financial Systems
Testing in finance is indispensable due to the high stakes involved in financial transactions. Bugs can lead to considerable financial repercussions, making rigorous testing essential for ensuring system reliability.
Tools and Libraries for Testing C++ Applications
Utilizing libraries like Google Test and Catch2 can simplify the process of writing and executing tests, thereby enhancing application reliability. Below is a basic example of a unit test for our bank account class:
#include <gtest/gtest.h>
TEST(BankAccountTest, Deposit) {
BankAccount account;
account.deposit(100);
EXPECT_EQ(account.getBalance(), 100);
}
This unit test ensures that the deposit functionality of our bank account works as intended, laying the groundwork for systematic validation of our financial applications.

Conclusion
Utilizing C++ high performance for financial systems offers numerous advantages, including speed, resource control, and versatility. By understanding the unique features of C++, applying appropriate design patterns, and implementing rigorous testing practices, developers can create robust financial applications capable of meeting the demands of modern finance.
As the finance industry evolves, the possibilities of C++ might lead to even more sophisticated financial solutions. For those eager to dive deeper into C++, continual learning and hands-on experience remain critical to mastering high-performance financial system development.