Private inheritance in C++ allows a derived class to inherit from a base class, where the members of the base class become private members of the derived class and cannot be accessed by any class outside of it.
Here's a simple code snippet demonstrating private inheritance:
class Base {
public:
void display() {
std::cout << "Base class display function." << std::endl;
}
};
class Derived : private Base {
public:
void show() {
display(); // Accessible within Derived
}
};
int main() {
Derived obj;
obj.show(); // Works fine, calls display()
// obj.display(); // Error: 'display' is inaccessible
return 0;
}
Understanding Inheritance in C++
What is Inheritance?
Inheritance is a fundamental concept in object-oriented programming (OOP) that allows one class (derived class) to inherit the attributes and methods of another class (base class). This mechanism fosters relationships between classes, enabling them to share functionality and data.
There are three primary types of inheritance in C++: public, protected, and private. Each type determines how the members of the base class can be accessed by the derived class and outside components.
Benefits of Using Inheritance
Using inheritance provides several advantages:
- Code Reusability: You can encapsulate common functionalities in a base class, allowing derived classes to reuse that code without having to rewrite it.
- Extensibility of Classes: You can create new functionalities by extending existing classes instead of modifying them.
- Polymorphism: Inheritance allows classes to be treated as instances of their base class, enabling more flexible and reusable code.
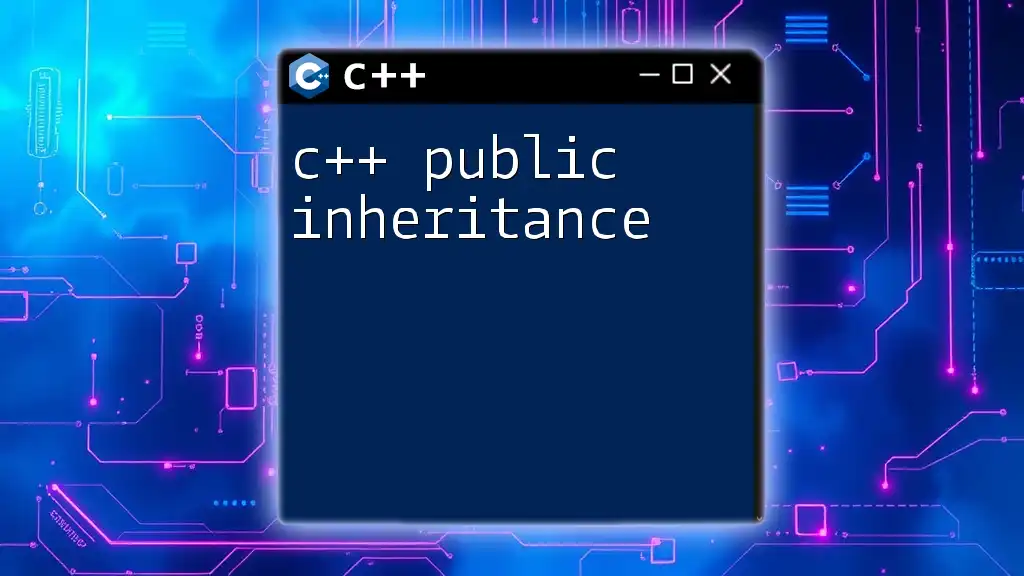
Private Inheritance: An Overview
Definition of Private Inheritance
Private inheritance means that the base class's public and protected members become private members of the derived class. This means that while the derived class can access these members, they cannot be accessed directly through an instance of the derived class from outside the class hierarchy.
When to Use Private Inheritance
Private inheritance is particularly useful in certain scenarios, such as:
- Private Implementation Hiding: It helps to hide the details of implementation, thereby offering a clear boundary between an interface and its implementation.
- Limiting Interface Exposure: By using private inheritance, you can prevent other classes from depending on the base class's interface, which can lead to tighter control over the architecture.
Real-world applications for private inheritance might include situations where a class needs to functionally extend another class but shouldn't expose its interface.
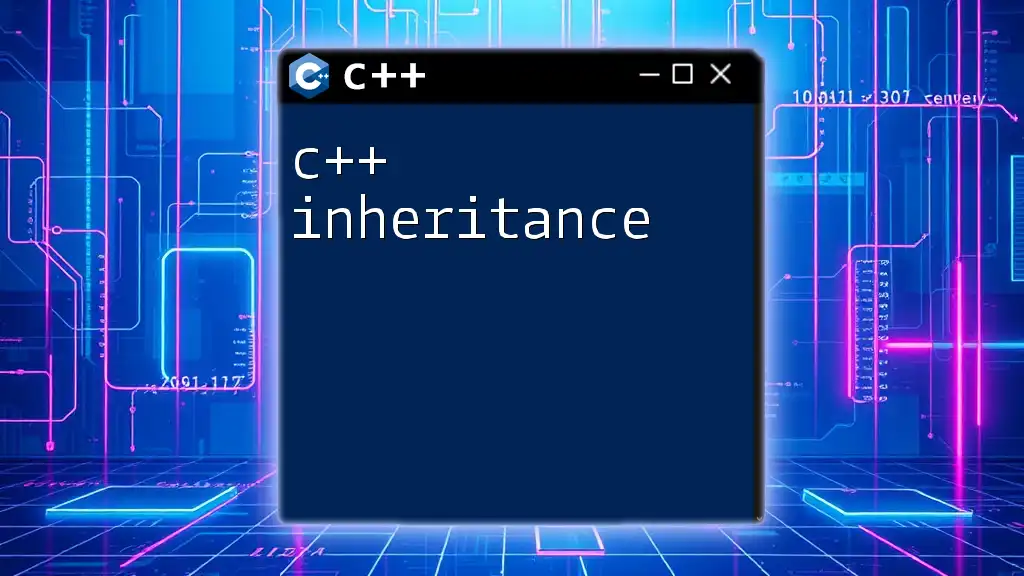
Syntax and Implementation of Private Inheritance
Basic Syntax
The syntax for private inheritance in C++ is fairly straightforward. You can declare it by placing the keyword `private` before the base class name in the derived class definition. Here’s an example:
class Base {
public:
void display() { std::cout << "Base class display." << std::endl; }
};
class Derived : private Base {
public:
void show() { display(); } // Use of Base's method in Derived
};
Example of a Private Inheritance Scenario
Let's look at a real-world example where a specialized class needs to inherit from a base class privately.
class Engine {
public:
void start() { std::cout << "Engine started." << std::endl; }
};
class Car : private Engine {
public:
void ignite() { start(); } // Car can use Engine's start method
};
In this example, the `Car` class inherits privately from the `Engine` class. While `Car` can use the `start` method, any outside classes cannot directly access an `Engine` object through a `Car` instance. This design helps in implementing a specific behavior without exposing the base class's interface.
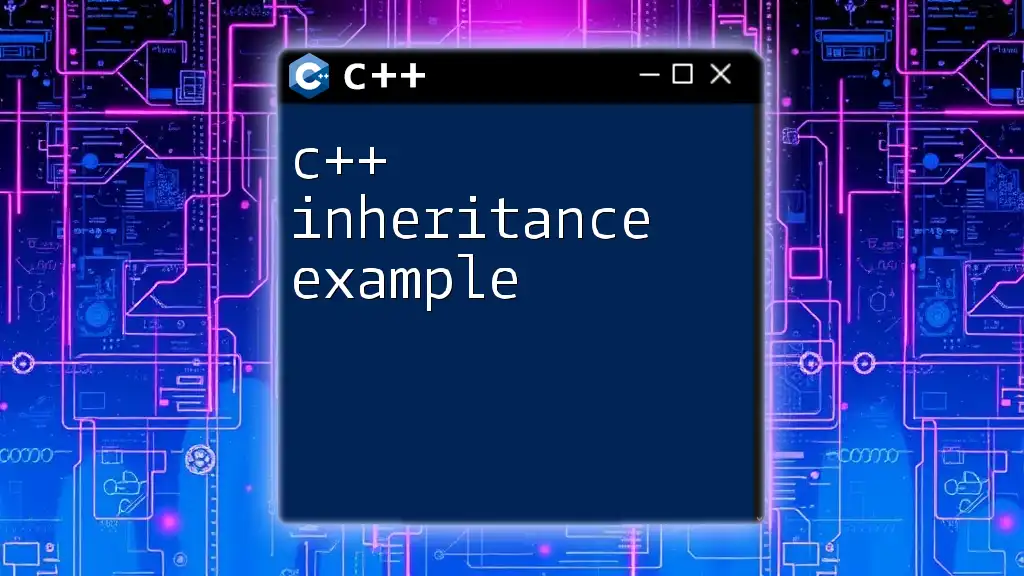
Access Control with Private Inheritance
Accessing Base Class Members
Private inheritance significantly changes the accessibility of base class members. In private inheritance, all members of the base class are treated as private in the derived class. This strict encapsulation means they cannot be accessed from outside the derived class.
Example of Access Control
Consider this code snippet to illustrate access control:
class A {
public:
void funcA() {}
};
class B : private A {
public:
void funcB() {
funcA(); // Accessible, since funcA is accessed within B
}
};
// Class C cannot access funcA directly
class C : public B {
public:
void funcC() {
// funcA(); // Error: funcA is not accessible
}
};
Here, class `B` privately inherits from class `A`. `B` can access `funcA`, but class `C`, which derives from `B`, cannot. This illustrates how private inheritance effectively restricts the visibility of the base class members.
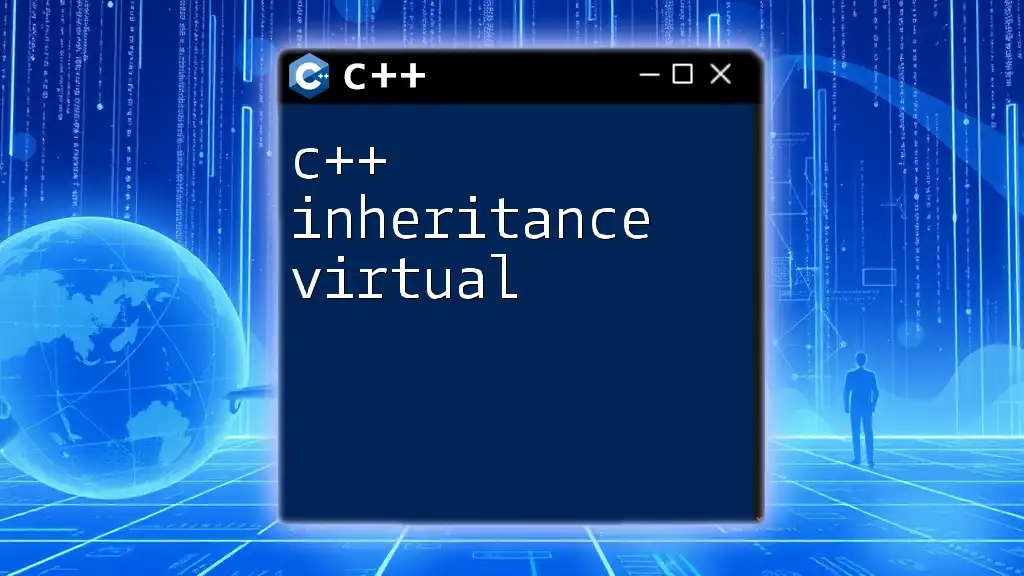
Advantages of Private Inheritance
Encapsulation and Flexibility
Private inheritance enhances encapsulation by restricting access to the base class members. This increased encapsulation leads to higher flexibility in class design, as changes in the base class do not necessarily affect external classes.
Avoiding Fragile Base Class Problem
The fragile base class problem occurs when changes to a base class inadvertently affect dependent derived classes. Private inheritance can mitigate this issue since the derived class does not expose the base class publicly, thus protecting the derived classes' implementations from unintended dependencies.
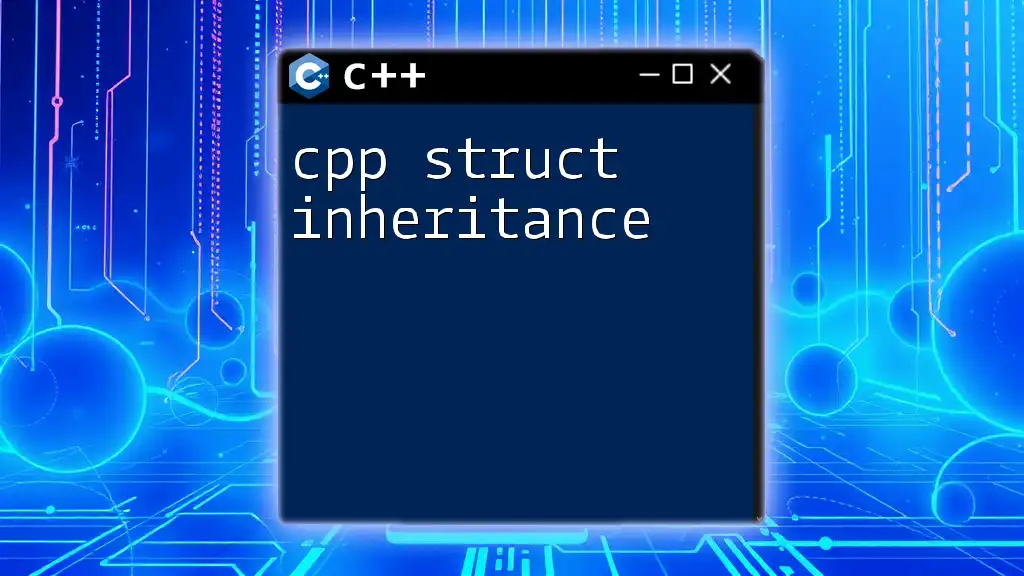
Limitations of Private Inheritance
Reduced Polymorphism
One drawback of private inheritance is that it hampers polymorphic behavior. Because the base class's interface is not exposed, external code cannot take advantage of polymorphism through the derived class. This limitation can lead to inefficiencies if polymorphism is a design goal.
Potential for Misuse
While private inheritance can provide significant benefits, it can also be misused. Developers might inadvertently create a complex hierarchy that is difficult to manage. Careful consideration should be given to the relationship between classes to prevent over-engineering.
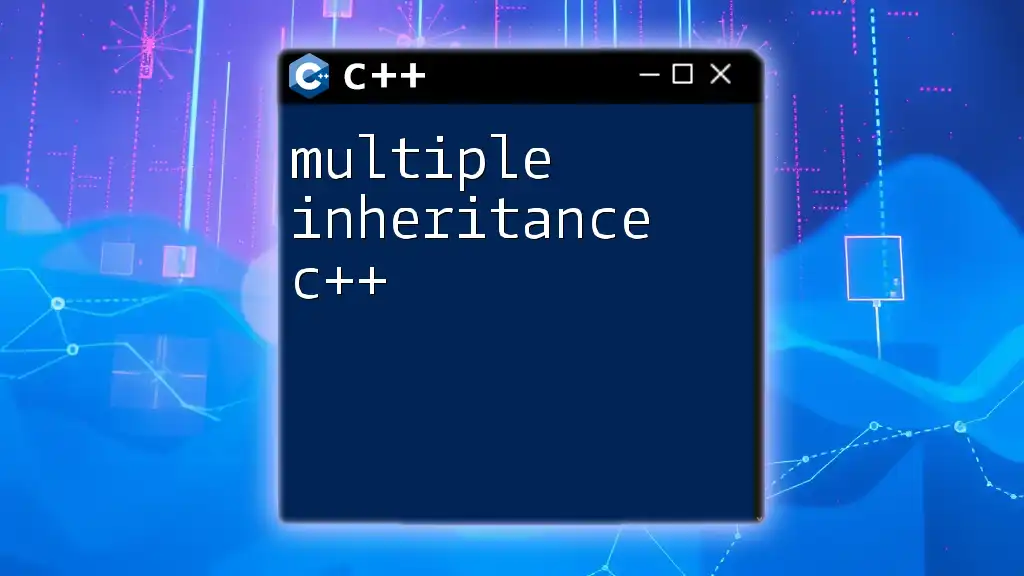
Conclusion
In summary, C++ private inheritance is a powerful feature that allows developers to define strong relationships between classes while maintaining encapsulation. Understanding its mechanics, appropriate usage scenarios, and its advantages and limitations is essential for effective class design. As with many programming principles, experimentation and careful consideration are keys to leveraging private inheritance successfully in real-world applications.
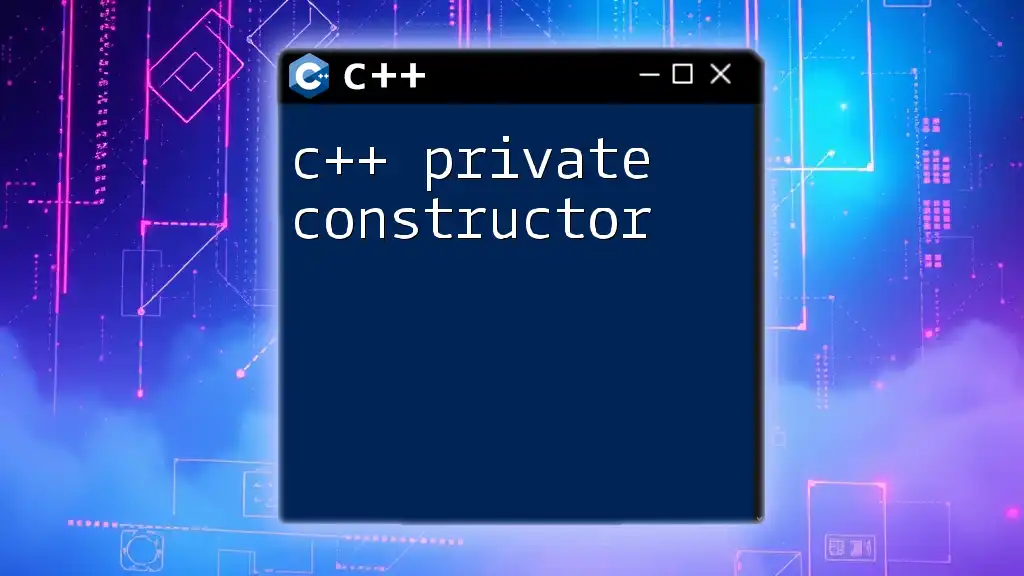
Call to Action
I encourage you to experiment with the concepts discussed in this article. Try implementing private inheritance in your projects and observe how it impacts your code structure and design. Share your experiences and questions to continue the learning process!