Protected inheritance in C++ allows a derived class to inherit the base class's public and protected members but makes them accessible only to the derived class and its further derived classes, not to the outside world.
class Base {
protected:
int protectedValue;
public:
Base() : protectedValue(10) {}
};
class Derived : protected Base {
public:
void showValue() {
// Accessing protected member from Base class
std::cout << "Protected Value: " << protectedValue << std::endl;
}
};
Understanding Protected Inheritance
Definition of Protected Inheritance
Protected inheritance in C++ serves as a special access control mechanism, determining how members of a base class can be accessed by derived classes. In protected inheritance, the public and protected members of the base class become protected members for the derived class. This means that while the derived class can access the base class's public and protected members, these members are not accessible from outside the derived class hierarchy.
To illustrate this concept better, consider the following simple structure. Here's the syntax used to declare protected inheritance:
class Base {
protected:
int protectedValue;
};
class Derived : protected Base {
public:
void setValue(int value) {
protectedValue = value; // Allowed
}
};
In this example, `protectedValue` is accessible within `Derived`, but it is hidden from users of `Derived`, providing a layer of encapsulation.
Syntax of Protected Inheritance
The syntax for protected inheritance is straightforward, utilizing the `protected` keyword while defining the derived class. The derived class inherits from the base class in a way that modifies access permissions, as shown in the example above.
Differences Between Protected and Public Inheritance
Public Inheritance
Public inheritance is the most common form of inheritance in C++. Here, the public members of the base class remain public in the derived class. This type of inheritance is used when you want to express an "is-a" relationship that is open for all users.
class Base {
public:
int publicValue;
};
class Derived : public Base {
public:
void setPublicValue(int value) {
publicValue = value; // Allowed
}
};
In this code snippet, `publicValue` is available to all users of `Derived`, allowing unrestricted access to its value.
Comparison of Access Rights
When contrasting protected inheritance with public inheritance, the key difference lies in the control of access. In protected inheritance, derived classes can still access protected and public members, but any external code that tries to access them will face restrictions. This is particularly useful in scenarios where internal class structure should remain hidden while allowing derived classes to function normally.
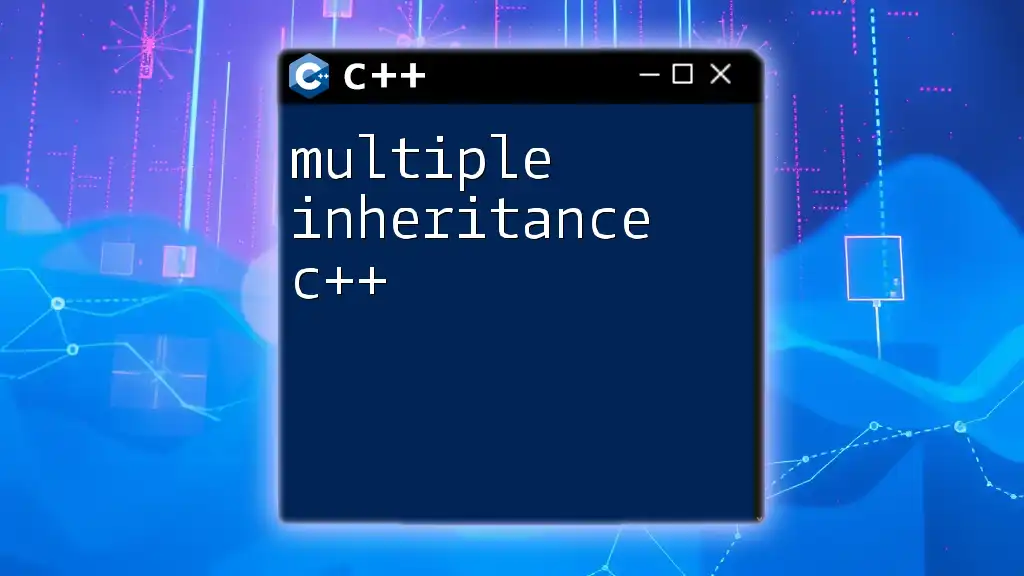
When to Use Protected Inheritance
Use Cases for Protected Inheritance
Protected inheritance is beneficial in several scenarios:
- Subclassing Frameworks: When creating a framework where base classes define general behavior and derived classes implement specific details, protected inheritance ensures derived classes can access the behavior without exposing it to users.
- Controlled Access: In cases where you want to allow derived classes to modify base class states while preventing users from manipulating those states directly, protected inheritance acts as a safeguard.
Best Practices for Implementing Protected Inheritance
When employing protected inheritance, developers should remember a few best practices:
- Be Intentional: Use protected inheritance only when it genuinely fits the intended design. If public inheritance is more suitable, opt for it.
- Avoid Overcomplication: If the hierarchy becomes too complex with protected inheritance, consider refactoring the design to enhance clarity.
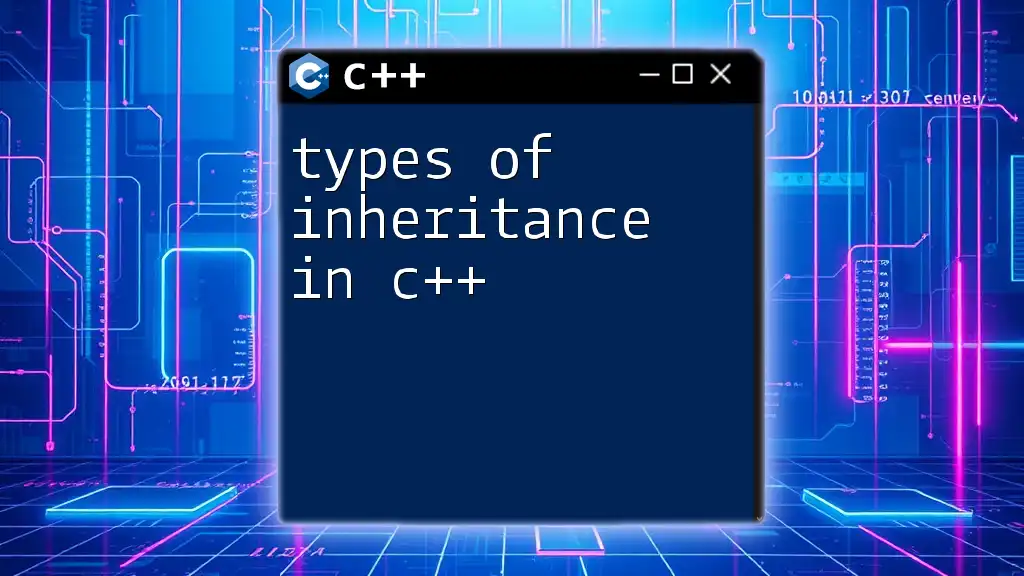
Code Examples and Scenarios
Example of Protected Inheritance in Action
To demonstrate how protected inheritance can be implemented effectively, consider the following example:
class Base {
protected:
int value;
};
class Derived : protected Base {
public:
void initializeValue(int val) {
value = val; // Allowed
}
};
class FurtherDerived : public Derived {
public:
void showValue() {
// value is not accessible here
// This will cause a compilation error
}
};
In this example, `FurtherDerived`, while inheriting from `Derived`, cannot access `value`, even though it is a part of the class's parentage due to the protected nature of the inheritance. This illustrates the encapsulation provided by protected inheritance.
Practical Scenario Comparisons
A practical comparison of protected versus public inheritance can be instrumental in understanding their effects. For instance, if you are designing a library where a base class exposes functionality to clients but should restrict some details, using protected inheritance can limit visibility while still allowing derived classes to utilize functional components.
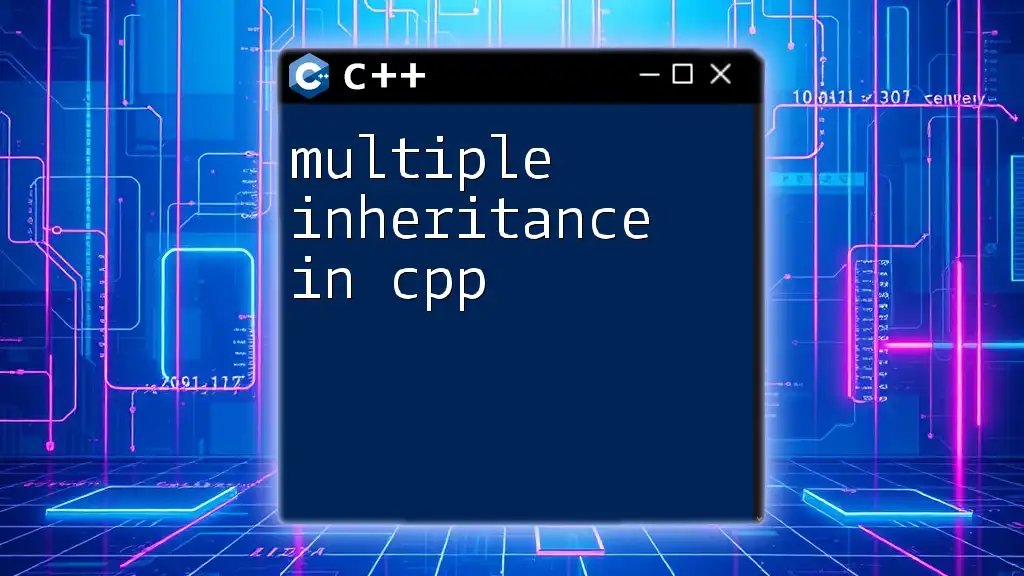
Common Misconceptions about Protected Inheritance
A prevalent misconception is that protected inheritance is synonymous with private inheritance. While both restrict access from outside the derived class, protected inheritance still allows derived classes within the same hierarchy to access base class members. Clarifying these distinctions can prevent confusion and promote better design choices.
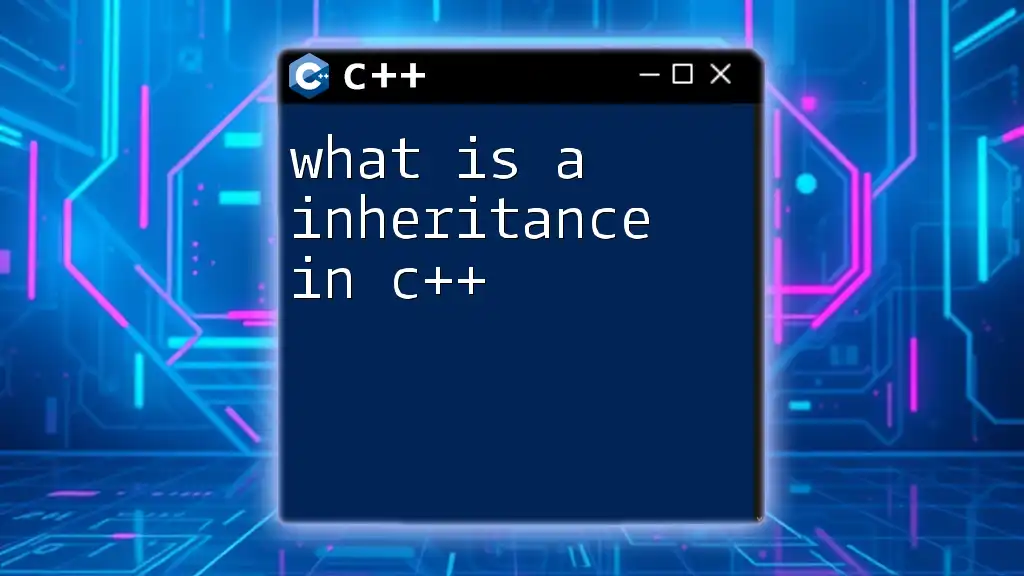
Conclusion
In summary, protected inheritance in C++ plays a significant role in managing access levels between base and derived classes. By modifying the visibility of inherited members, it ensures controlled interactions and enhances encapsulation. When used appropriately, protected inheritance can be a powerful tool in an object-oriented programmer's toolkit, emphasizing design clarity and structural integrity. It's crucial for developers to grasp these concepts to build robust and maintainable code architectures. Engage with your examples and deepen your understanding of C++ to reach your full programming potential!
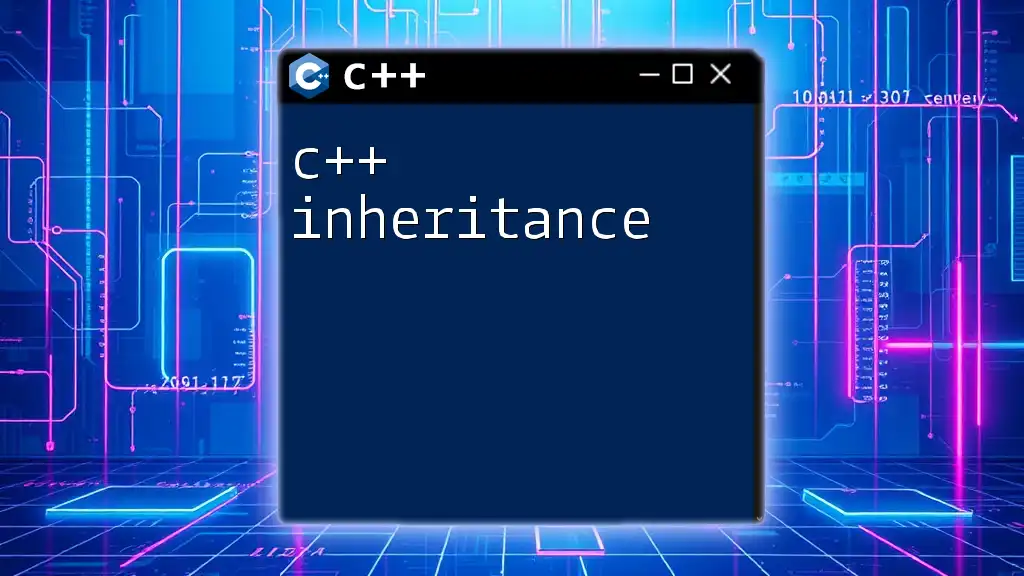
Additional Resources
For those looking to further their understanding of protected inheritance and other object-oriented principles in C++, consider exploring related literature, online courses, and forums dedicated to deepening your programming expertise.