In C++, "composition over inheritance" refers to a design principle where objects are composed of other objects instead of being derived from a parent class, promoting flexibility and reusability.
Here's a simple code snippet to illustrate the concept:
#include <iostream>
#include <string>
class Engine {
public:
void start() { std::cout << "Engine started." << std::endl; }
};
class Car {
private:
Engine engine; // Composition: Car has an Engine
public:
void drive() {
engine.start(); // Using Engine's functionality
std::cout << "Car is driving." << std::endl;
}
};
int main() {
Car car;
car.drive();
return 0;
}
Understanding Composition and Inheritance
What is Inheritance?
Inheritance is a fundamental concept in Object-Oriented Programming (OOP) that allows a class to derive properties and behaviors (methods) from another class. The class that is being inherited from is known as the base class, while the class that inherits is called the derived class. Inheritance promotes code reuse and establishes hierarchical relationships between classes, which can lead to a more intuitive understanding of the relationships within your code.
For instance, if you have a base class `Animal`, derived classes like `Dog` and `Cat` can be created, inheriting common properties like `name` and behaviors like `speak()`.
What is Composition?
Composition is another OOP design principle where a class is composed of one or more objects from other classes. Instead of inheriting from a base class, a class can include instances of other classes as members. This leads to a more flexible design as the behavior of a class can change simply by changing or replacing the components it relies on.
For example, a class `Car` might use `Engine`, `Wheels`, and `Chassis` as parts of its composition, allowing each part to be defined and modified independently.
Difference Between Composition and Inheritance
When evaluating composition and inheritance, it's essential to recognize their fundamental differences:
- Inheritance establishes an "is-a" relationship (e.g., a `Dog` is an `Animal`).
- Composition establishes a "has-a" relationship (e.g., a `Car` has an `Engine`).
Use Cases:
- Inheritance is suitable when the derived class shares a common behavior or characteristic of the base class.
- Composition shines in scenarios where classes need to cooperate but don't necessarily form a hierarchy.
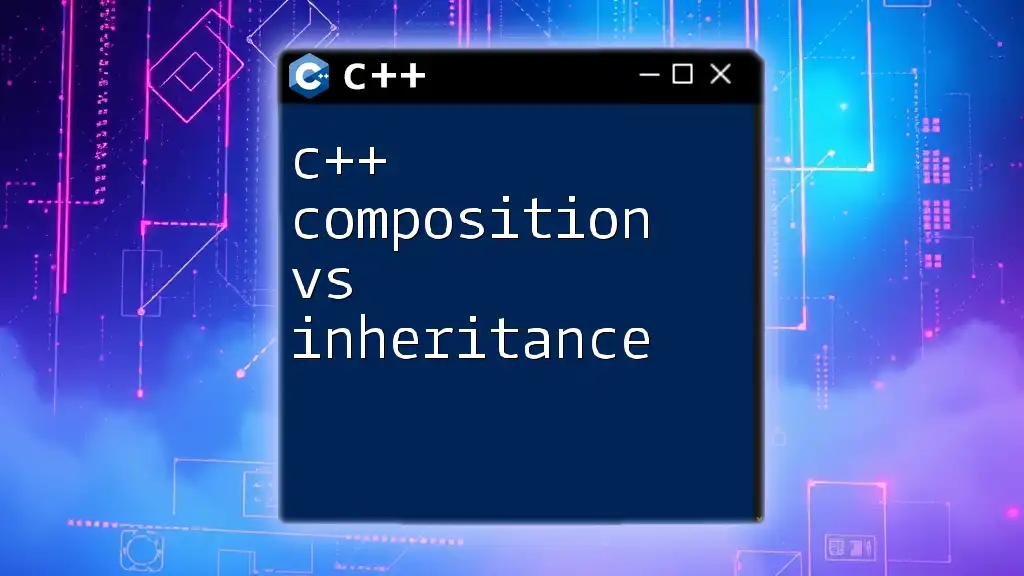
Advantages of Composition Over Inheritance
Flexibility and Adaptability
One of the greatest advantages of composition is its inherent flexibility. With composition, you can easily modify a class's behavior by swapping components. For example, if you want to change the `Engine` of a `Car` class, you can create a new `Engine` class and replace the existing one without affecting the rest of the codebase.
Reduced Coupling
Composition helps achieve loose coupling, meaning that classes are more independent from one another. In contrast, inheritance can lead to tight coupling where changes to a base class could inadvertently affect all derived classes.
For example, consider the following composition:
class Engine {
public:
void start() {...}
void stop() {...}
};
class Wheels {
public:
void rotate() {...}
};
class Car {
private:
Engine engine; // Composition relationship
Wheels wheels; // Composition relationship
public:
void startCar() {
engine.start();
wheels.rotate();
}
};
In this case, the `Car` class can function properly regardless of how the `Engine` and `Wheels` classes are implemented, as long as they adhere to the expected interface.
Prevention of Inheritance Pitfalls
Utilizing composition can significantly mitigate common pitfalls associated with inheritance, such as the fragile base class problem and the diamond problem.
-
Fragile Base Class Problem: This occurs when changes in a base class inadvertently break derived classes. For instance, if you refactor a method in a base class, all derived classes might need updates to align with the changes.
-
Diamond Problem: In languages that allow multiple inheritance, a diamond problem arises when two classes derive from the same base class, leading to ambiguity concerning which base class method gets called.
By using composition, these issues can be circumvented. Each class operates independently, and the complexity is managed within each contained component.
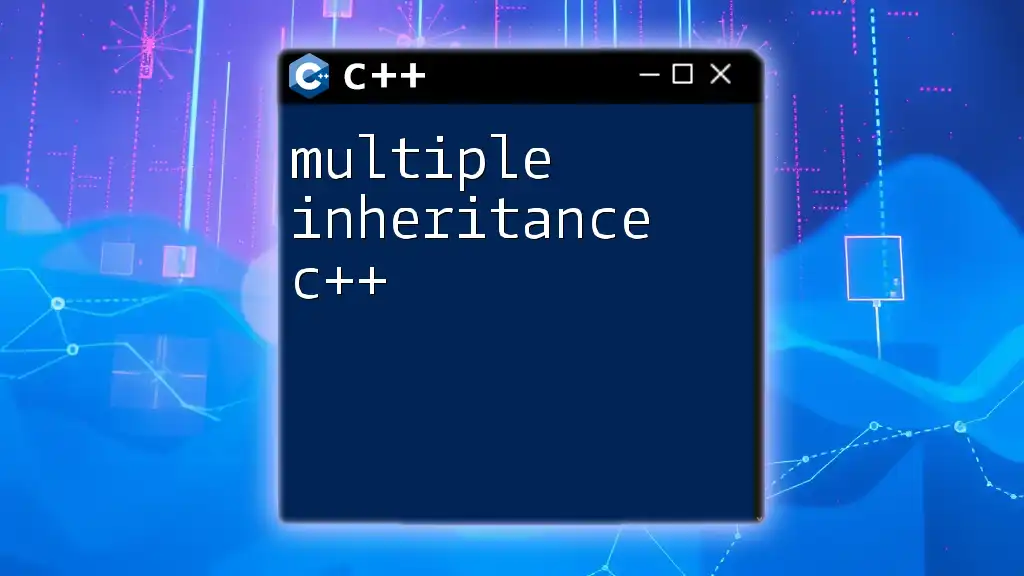
Practical Examples of Composition in C++
Real-World Scenario
Let's consider a real-world application of composition by modeling a `Car`. In this case, we utilize multiple classes representing the different functional components of the car, such as `Engine`, `Wheels`, and `Chassis`.
class Engine {
public:
void start() {
// Start the engine logic
}
void stop() {
// Stop the engine logic
}
};
class Wheels {
public:
void rotate() {
// Logic for rotating wheels
}
};
class Chassis {
public:
void hold() {
// Logic to hold the car parts together
}
};
class Car {
private:
Engine engine; // Composition of engine
Wheels wheels; // Composition of wheels
Chassis chassis; // Composition of chassis
public:
void startCar() {
engine.start();
wheels.rotate();
// Additional logic to start driving
}
};
Expanding Functionality with Composition
One of the strengths of composition is its ability to add or modify functionality dynamically. Suppose we want to incorporate a GPS system into our `Car`. Instead of creating a new class hierarchy, we can simply include a new component:
class GPSSystem {
public:
void navigate() {
// Logic for GPS navigation
}
};
class CarWithGPS {
private:
Engine engine;
Wheels wheels;
Chassis chassis;
GPSSystem gps; // New functionality added through composition
public:
void startCarWithGPS() {
engine.start();
gps.navigate();
}
};
In this case, you've incorporated a new `GPSSystem` into the existing `Car` class simply by adding it as a composition. This approach allows you to expand the functionality of your classes efficiently, reflecting the true potential of composition.
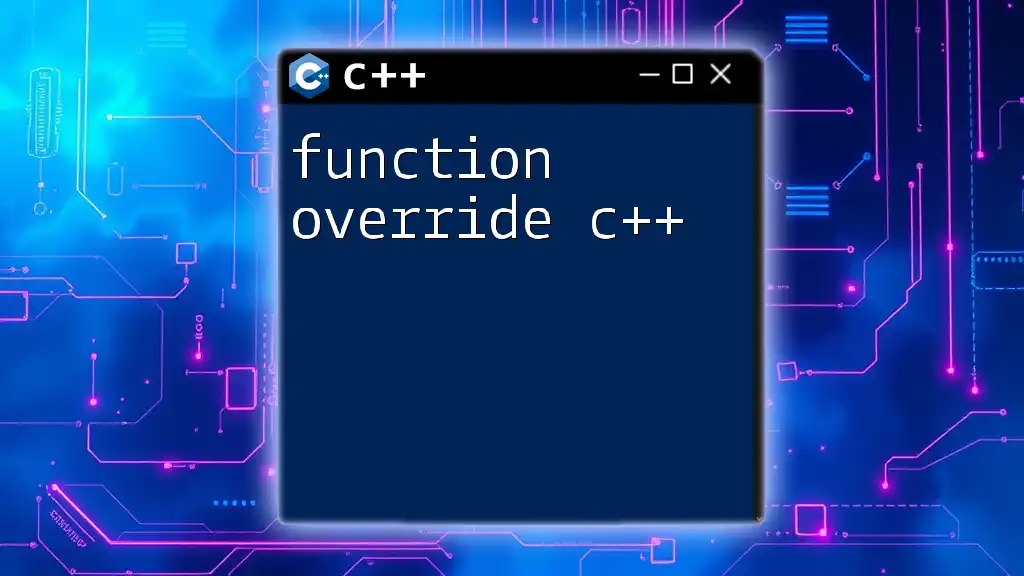
When to Use Composition
Decision Criteria
Choosing between composition and inheritance can sometimes be nuanced, but here are some guidelines:
- Use composition when you need to model relationships where one class is not a special type of another.
- Favor inheritance when a subclass is fundamentally an extension of a base class with shared behavior.
Best Practices
To maximize the benefits of composition in your C++ applications, consider the following best practices:
- Avoid deeply nested hierarchies: They can become hard to manage and maintain.
- Define interfaces to specify expected behaviors, allowing different classes to be substituted flexibly.
- Use composition for building flexible, reusable code, making it easier to adapt to changing requirements.
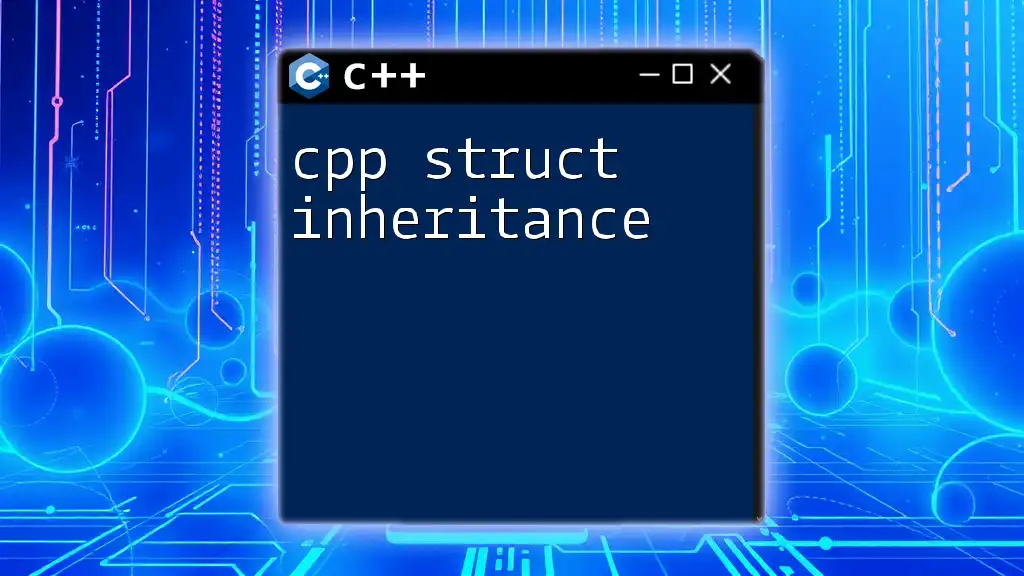
Conclusion
To summarize, composition over inheritance in C++ provides significant advantages in flexibility, reduced coupling, and prevention of common pitfalls associated with traditional inheritance. By opting for composition, developers can create systems that are not only easier to manage but also promote cleaner, more modular code.
As you embark on your C++ programming journey, embrace the power of composition and apply it to your projects. Share your experiences or questions in the comments below as we explore this vital area of object-oriented design together!
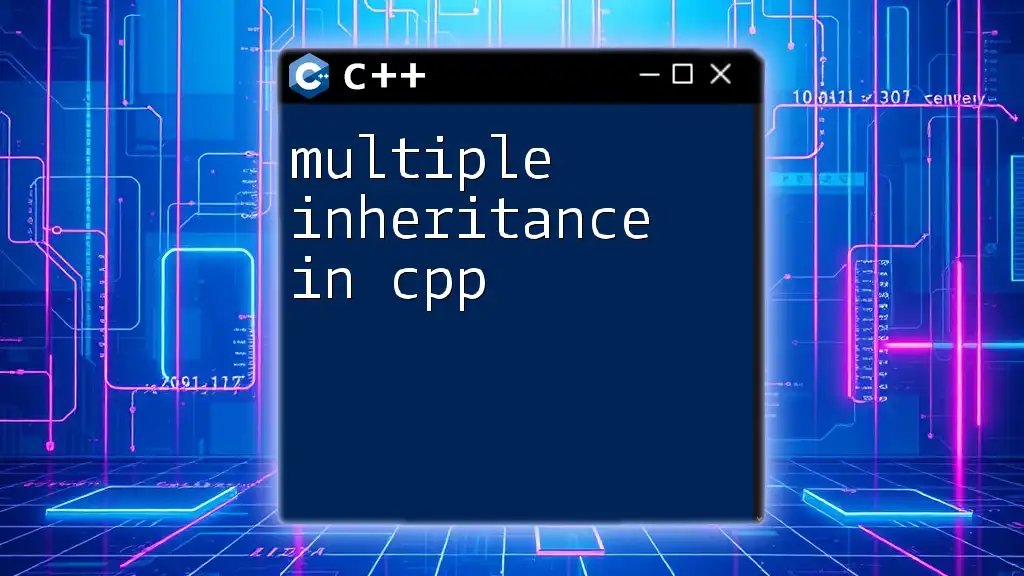
Further Reading
For those eager to delve deeper into advanced C++ topics and design patterns, consider exploring:
- Books on Object-Oriented Programming design patterns.
- Online C++ courses emphasizing hands-on practice with class composition.
- Official C++ documentation focusing on best practices and new features.
Learn, experiment, and build excellent software using the principles of composition over inheritance!