In C++, struct inheritance allows one struct to inherit the properties and methods of another struct, promoting code reuse and organization.
Here's a code snippet demonstrating struct inheritance:
#include <iostream>
struct Base {
void show() {
std::cout << "Base struct" << std::endl;
}
};
struct Derived : Base {
void display() {
std::cout << "Derived struct" << std::endl;
}
};
int main() {
Derived obj;
obj.show(); // Inherited method from Base
obj.display(); // Method from Derived
return 0;
}
What is Struct Inheritance?
Struct inheritance in C++ allows a programmer to define new structs based on existing ones, facilitating the extension of functionality and reuse of code. Structs in C++ are similar to classes but have some key differences, notably the default access modifier, which is public for structs and private for classes. This distinction makes structs particularly suited for simple data structures.
Understanding struct inheritance is crucial for developers looking to take advantage of code reusability and data organization, allowing them to build on existing entities without rewriting code.
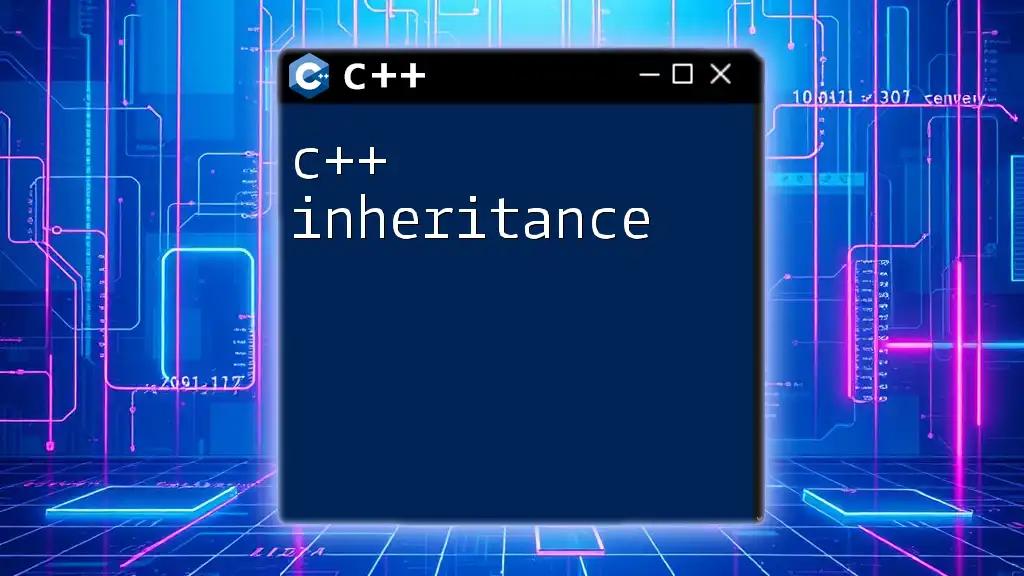
Benefits of Using Struct Inheritance
Using struct inheritance offers several advantages:
-
Code Reusability: By creating derived structs that inherit attributes from base structs, developers reduce redundancy and make it easier to manage updates.
-
Enhanced Data Organization: Struct inheritance enables the logical grouping of data, facilitating better management and understanding of complex data types.
-
Simplification of Complex Data Types: Struct inheritance allows for the abstraction of complex behaviors through derived structs, keeping implementations clean and maintainable.
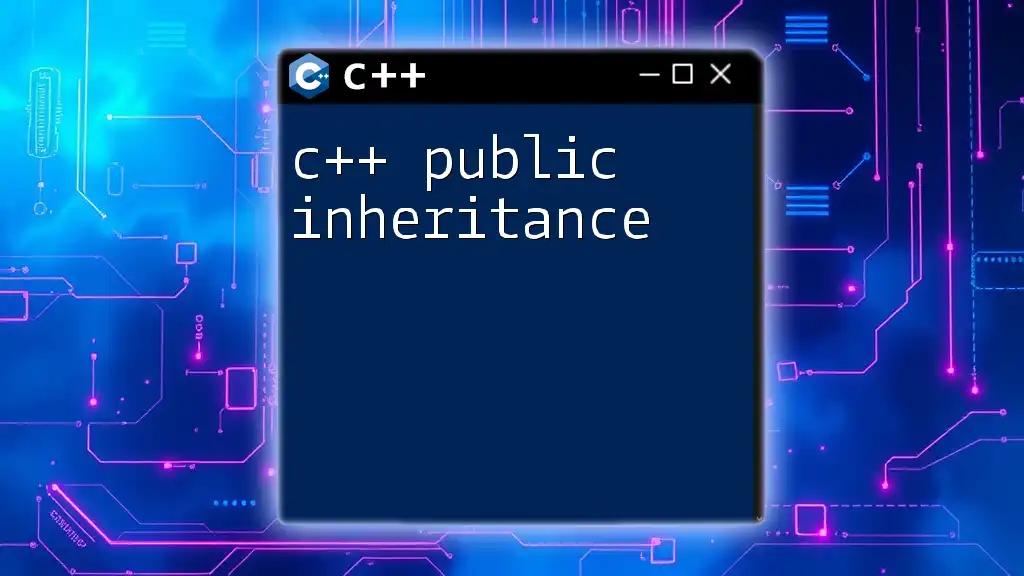
Understanding C++ Structures
Defining a Struct in C++
In C++, a struct is defined using the `struct` keyword. Structs can contain both data members and member functions, similar to classes.
Here’s a basic example of defining a struct:
struct Person {
std::string name;
int age;
};
In this example, the `Person` struct consists of two data members: `name` and `age`.
Key Characteristics of Structs
-
Default Public Access: Members of a struct are public by default, making them accessible outside of the struct. This characteristic emphasizes the use of structs for data-centric designs.
-
Use Cases for Structs Over Classes: Structs are often preferred when creating simple data structures with no or few member functions, such as creating records for database entities.

Basics of Inheritance in C++
What is Inheritance?
Inheritance is a cornerstone of object-oriented programming in C++. It allows one class or struct (the derived class or struct) to inherit properties and behaviors from another (the base class or struct). In C++, inheritance can be public, protected, or private, impacting how members of the base class are accessed from the derived class.
How Inheritance Works in C++
When a struct is derived from another struct, it can access the public and protected members of the base struct, gaining all its attributes and methods. Struct inheritance also affects the memory layout; data from base structs is typically stored at the beginning of the derived struct.

C++ Struct Inheritance
Declaring Derived Structs
To declare a derived struct in C++, you simply use the colon `:` followed by the access specifier (usually `public`) and the base struct's name.
Here's an example of a derived struct:
struct Employee : public Person {
double salary;
};
In this case, `Employee` inherits the members of the `Person` struct while also introducing a new member, `salary`.
Accessing Members of Base and Derived Structs
Members from the base struct can be accessed directly in the derived struct. Here’s how you can access these members:
Employee emp;
emp.name = "John Doe";
emp.age = 30;
emp.salary = 50000.0;
In this snippet, the derived struct `Employee` utilizes the members `name` and `age` inherited from the `Person` struct, demonstrating how inherited members function seamlessly.
Base Class Constructor Calls
When a derived struct is instantiated, the constructor of the base struct can be called to initialize inherited attributes. For instance:
struct Person {
std::string name;
int age;
Person(std::string n, int a) : name(n), age(a) {}
};
struct Employee : public Person {
double salary;
Employee(std::string n, int a, double s) : Person(n, a), salary(s) {}
};
In this example, the `Employee` constructor calls the `Person` constructor to initialize the base members `name` and `age`.

Overriding Members in Derived Structs
Understanding Member Overriding
When a derived struct has a member function with the same name as one in the base struct, this member function can be considered an "override." It allows developers to provide a specialized implementation in the derived struct.
Here’s an example:
struct Vehicle {
virtual void start() { std::cout << "Vehicle starting\n"; }
};
struct Car : public Vehicle {
void start() override { std::cout << "Car starting\n"; }
};
In this example, the `Car` struct overrides the `start` method provided by the `Vehicle` base struct, allowing for customized behavior.

Polymorphism with Structs
What is Polymorphism?
Polymorphism refers to the ability of different structs (or classes) to be treated as instances of the same base struct. This enhances flexibility in programming, allowing functions to operate on objects of varying types through a common interface.
Using Polymorphism in Structs
To demonstrate polymorphism, consider a function that takes a pointer to the base `Vehicle` struct:
void vehicleStart(Vehicle* v) {
v->start();
}
Vehicle* myCar = new Car();
vehicleStart(myCar); // Outputs: Car starting
Here, when `vehicleStart` is called with a `Car` instance as a `Vehicle` pointer, the overridden `start` method of the `Car` class executes, showcasing polymorphic behavior.

Common Pitfalls in C++ Struct Inheritance
Diamond Problem
The "Diamond Problem" occurs in multiple inheritance scenarios where a class inherits from two classes that have a common parent, resulting in ambiguity regarding which parent's methods to invoke. To resolve this issue in C++, virtual inheritance can be used.
Visibility and Access Issues
Structs have public members by default, which can lead to unintended access. Be mindful of access specifiers when designing your structs to avoid exposing sensitive data or methods inadvertently.

Best Practices for Struct Inheritance
When to Use Structs Over Classes
Structs are best utilized for simple data structures without complex behavior. If encapsulation and data hiding are required, classes may be a better choice.
Design Considerations
While inheritance can improve code reusability, it should be used judiciously. Favoring composition over inheritance can provide more flexible and stable code designs by allowing for more modular systems.

Tips for Mastering Struct Inheritance in C++
Recommended Resources for Further Learning
Consider exploring books like “The C++ Programming Language” by Bjarne Stroustrup or online courses on platforms like Coursera and Udemy to deepen your understanding of C++ structures and inheritance.
Practice Problems and Exercises
Engage with practice problems on coding platforms, such as LeetCode or HackerRank, to reinforce your understanding of struct inheritance in C++. Working through problems will enhance both your theoretical and practical coding skills.

Conclusion
Understanding C++ struct inheritance is vital for effective programming in C++. By harnessing the power of struct inheritance, programmers can achieve improved code organization and functionality. By exploring the concepts outlined in this guide, you can enhance your coding capabilities and develop more sophisticated C++ applications.