In C++, the primary difference between a struct and a class is that members of a struct are public by default, while members of a class are private by default.
Here’s an example demonstrating this distinction:
struct MyStruct {
int x; // public by default
};
class MyClass {
int y; // private by default
public:
void setY(int value) { y = value; } // public method to modify private member
};
Understanding the Basics: Struct and Class
In C++, both structs and classes are used to define custom data types that can encapsulate data and functions. Despite their similarities, they come with distinct features that can dictate their usage in programming.
A struct is primarily a collection of variables (also known as data members) under one name. The typical use of a struct is to group related variables together.
On the other hand, a class is a more advanced data type that carries not only data members but also functions (methods) that operate on that data. Classes support concepts like encapsulation, inheritance, and polymorphism, making them suitable for larger, more complex applications.
The key fundamental difference between structs and classes is their default access levels. This point will be addressed in-depth in the following sections.
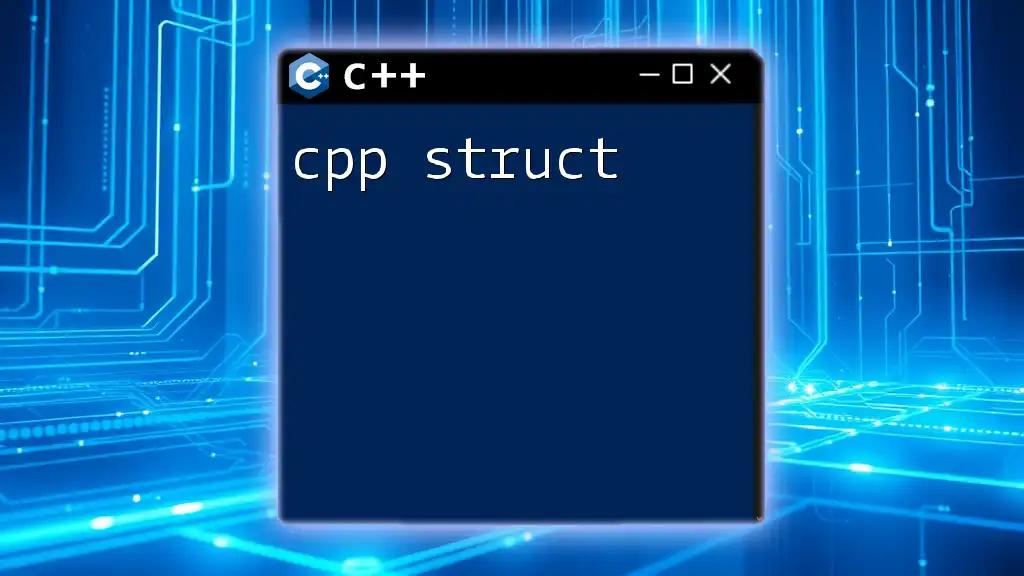
C++ Struct vs Class: Access Specifiers
In C++, access specifiers determine the visibility of class members to other parts of the program.
Public, Private, and Protected
-
Within a struct, members are public by default. This means all data members and member functions can be accessed from outside the struct.
struct MyStruct { int x; // public by default }; MyStruct s; s.x = 5; // This is valid
-
In contrast, members of a class are private by default, which restricts access to them unless explicitly stated otherwise.
class MyClass { int x; // private by default }; MyClass c; // c.x = 5; // This would result in a compilation error
Example demonstrating access specifiers
Understanding access levels is crucial for managing data exposure within your program. Here’s a small illustration:
struct PublicStruct {
int a; // public
};
class PrivateClass {
int b; // private
public:
void setB(int value) {
b = value; // This is how we can set b
}
};
PublicStruct ps;
ps.a = 10; // Allowed
PrivateClass pc;
// pc.b = 20; // Not allowed
pc.setB(20); // Allowed

Memory Management in Structs and Classes
Structs and classes have similar memory layouts, fundamentally differing in terms of default accessibility.
Structs generally have a simpler memory structure because they usually deal with basic data types. Classes, being more complex, may introduce additional overhead, particularly when they contain multiple methods or use inheritance.
Example of memory usage comparison
Here’s a brief comparison of memory consumption in structs and classes:
struct SimpleStruct {
int a;
double b;
};
class SimpleClass {
int a;
double b;
};
int main() {
SimpleStruct ss; // Allocates memory for two members
SimpleClass sc; // Allocates memory for two members but may include overhead for methods
}
Although they may occupy the same amount of memory visually, the class can carry additional weights due to the potential for functions, virtual inheritance, etc. When designing performance-sensitive applications, this can have implications.

Inheritance: Class vs Struct
Understanding Inheritance
Inheritance is a key feature of object-oriented programming that allows a class to inherit properties and behaviors from another class.
How inheritance works with Classes
In C++, a class can derive from another class using the colon `:` operator, and the access specifier determines which members are accessible in the derived class. Classes, by default, derive privately unless specified otherwise:
class BaseClass {
public:
void show() {
// display something
}
};
class DerivedClass : public BaseClass {
};
How inheritance functions with Structs
Structs can also participate in inheritance in a similar manner, but since their members are public by default, it can simplify the syntax when dealing with inherited data members:
struct BaseStruct {
void show() {
// display something
}
};
struct DerivedStruct : BaseStruct {
};
Understanding how inheritance alters the behavior of both structs and classes is fundamental to making informed decisions in C++.

Use Cases: When to Use Structs vs Classes
Choosing between structs and classes often depends on the requirements of the application.
Guidance on Choosing
-
Performance considerations: If you need a lightweight data structure and are only grouping variables together, a struct is often preferred for its simplicity.
-
Situational usage examples:
- Structs are ideal for:
- Simple data groupings like points, coordinates, and configurations.
- Classes are ideal for:
- More complex entities that require encapsulation, polymorphism, and inheritance.
- Structs are ideal for:

Class vs Structure in C++: Member Functions
Defining Member Functions in Structs and Classes
Member functions or methods can be defined in both structs and classes. However, the way they are utilized can differ slightly.
Here are examples comparing member function declarations in both:
struct MyStruct {
void show() {
// Function implementation
std::cout << "I'm a struct member function!" << std::endl;
}
};
class MyClass {
public:
void show() {
// Function implementation
std::cout << "I'm a class member function!" << std::endl;
}
};
Both structs and classes can encapsulate functions, but remember to specify `public` access for class members if you want them accessible outside of that class.

The Role of Constructors and Destructors
Constructors in Structs
Structs can have constructors, but they are generally less complex. A default constructor is created automatically unless otherwise specified.
Constructors in Classes
In contrast, classes can have user-defined constructors that can take parameters, enabling more versatile object creation:
struct MyStruct {
int value;
MyStruct(int val) : value(val) {}
};
class MyClass {
public:
int value;
MyClass(int val) : value(val) {}
};
Destructors in Structs and Classes
Destructors are special member functions that are called when an object goes out of scope. Both structs and classes can have destructors, but typically, structs do not need them unless they manage resources.
struct MyStruct {};
class MyClass {
public:
~MyClass() {
// Cleanup code here
}
};
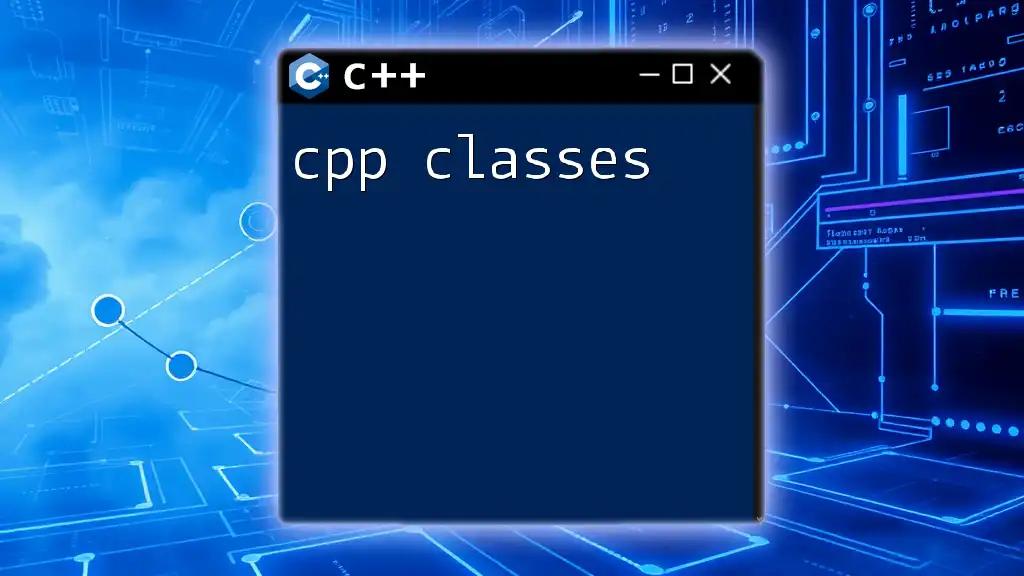
Default Copy Behavior
When it comes to copying, both structs and classes exhibit implicit copy behavior unless explicitly stated otherwise.
Example showcasing copy behavior differences
struct MyStruct {
int a;
};
class MyClass {
int a;
public:
MyClass(int val) : a(val) {}
};
MyStruct s1;
s1.a = 10;
MyStruct s2 = s1; // Copying a struct is straightforward
MyClass c1(20);
MyClass c2 = c1; // This would invoke the copy constructor, if defined
This difference can affect how data is managed and leads developers to define user-specific copy behavior in classes, enhancing control over memory and resources.
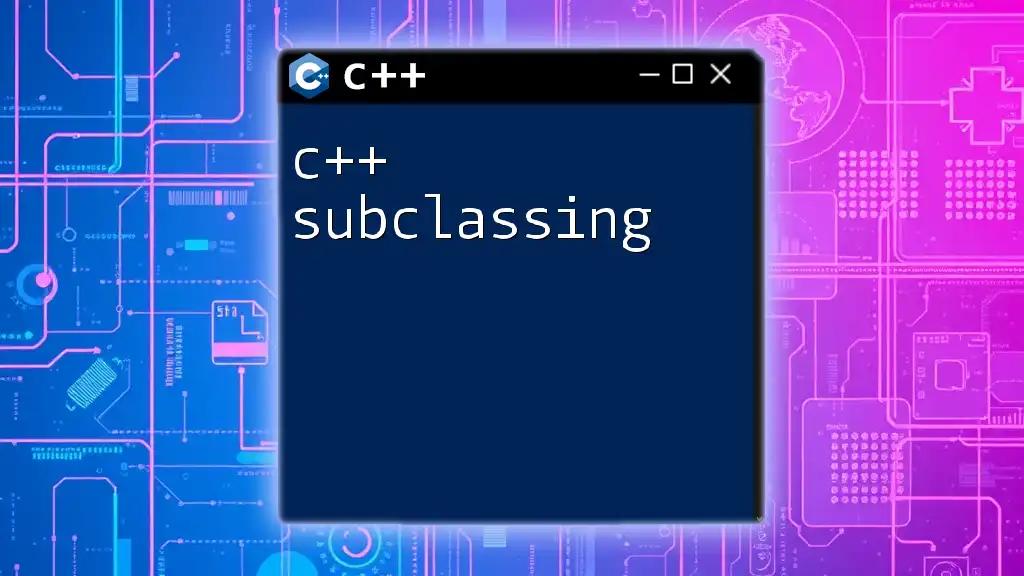
Templates and Polymorphism with Structs and Classes
Use of Templates
Templates can be used with both structs and classes, allowing for the creation of generic types.
template <typename T>
struct GenericStruct {
T data;
};
template <typename T>
class GenericClass {
T data;
};
Polymorphism in Classes
Classes support polymorphism through virtual functions, whereas structs do not typically engage in this practice.
Here’s a simple class demonstrating polymorphic behavior:
class Base {
public:
virtual void show() {
std::cout << "Base Class" << std::endl;
}
};
class Derived : public Base {
public:
void show() override {
std::cout << "Derived Class" << std::endl;
}
};
Understanding these features can significantly enhance the flexibility and robustness of your C++ programs.
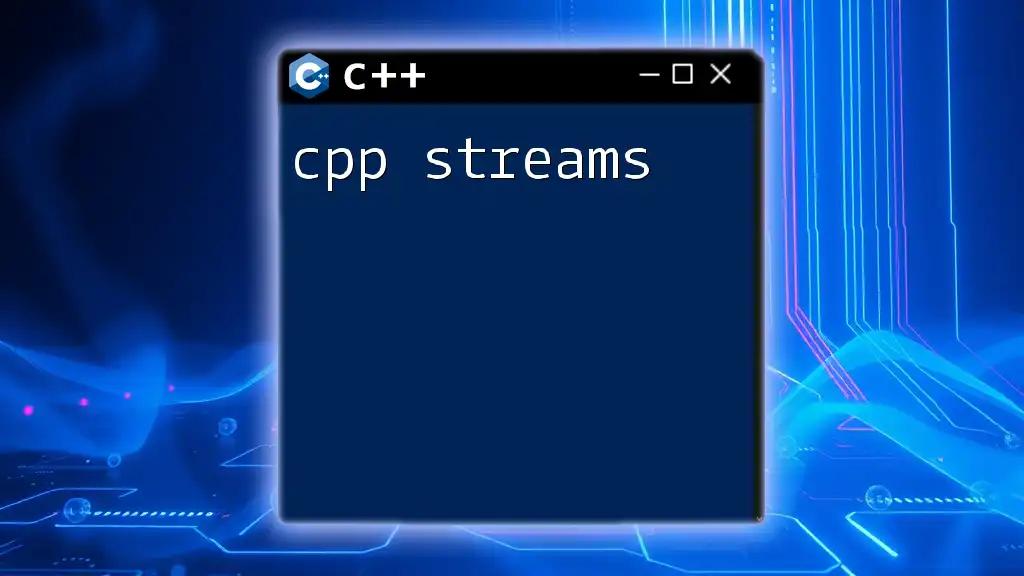
Summary: Key Takeaways
- C++ Struct vs Class: Structs are simple, while classes allow for more complex relationships and behaviors.
- Access specifiers: Default levels vary; know when members are exposed or hidden.
- Memory management: Both occupy similar spaces, but classes may incur additional overhead.
- Inheritance & Polymorphism: Classes support deeper hierarchies and behaviors.
- Choice of data structure: Assess your project requirements to choose wisely between structs and classes.

Conclusion
Mastering the differences between structs and classes in C++ is essential for effective programming. Understanding when and how to use each construct will enhance your ability to design clear, efficient, and maintainable code.
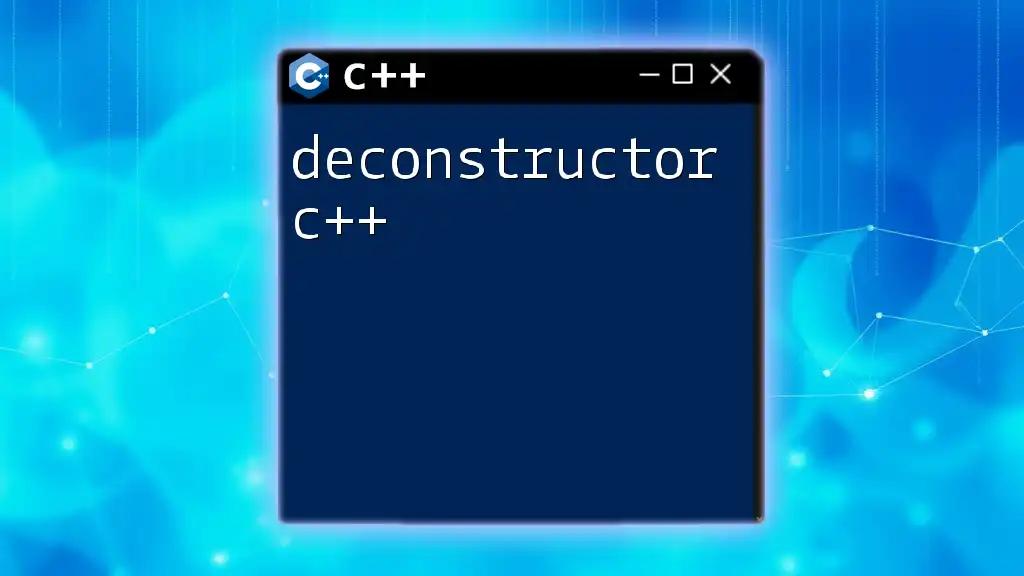
Additional Resources
For further information, consider exploring online tutorials, C++ documentation, and books focusing on object-oriented programming principles.
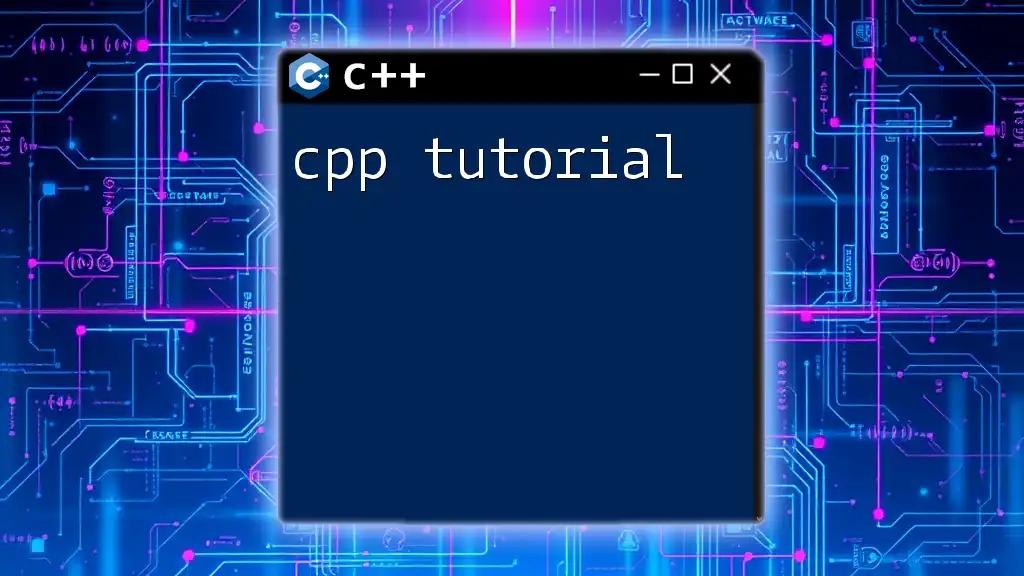
Frequently Asked Questions
-
Can structs have member functions? Yes, structs can have member functions just like classes.
-
When should I use a class instead of a struct? Use a class when you need encapsulation, inheritance, or polymorphism in your design. Use structs for lightweight data grouping.
By understanding and applying these concepts correctly, you'll find that both structs and classes serve vital roles in C++ programming.