In C++, `struct` is a way to define a custom data type that can contain multiple related variables, while `typedef struct` was commonly used in C to create an alias for a struct type, but in C++ it's often unnecessary because structs by default can be used like any other type.
Here's a simple code snippet illustrating both concepts:
// C++ struct definition
struct Person {
std::string name;
int age;
};
// Typedefing a struct in C (not necessary in C++)
typedef struct {
std::string name;
int age;
} PersonType;
Understanding C++ Structures
What is a Struct?
In C++, a `struct` is a user-defined data type that allows you to combine data items of different kinds. It is a means to group related variables together into a single entity, which can be more manageable and comprehensible in larger programs.
Structs are particularly useful when you want to model a real-world entity. For example, if you want to represent a person with specific attributes, a `struct` provides an ideal solution.
struct Person {
std::string name;
int age;
};
Syntax and Basic Usage
The syntax for declaring a `struct` is simple and clear. You define the structure's name and then enclose its data members within curly braces. You can then create instances of the struct and initialize them accordingly.
Person person1;
person1.name = "John";
person1.age = 30;
This basic approach allows you to gather all related information under a single identifier (`person1`), making code more intuitive and organized.
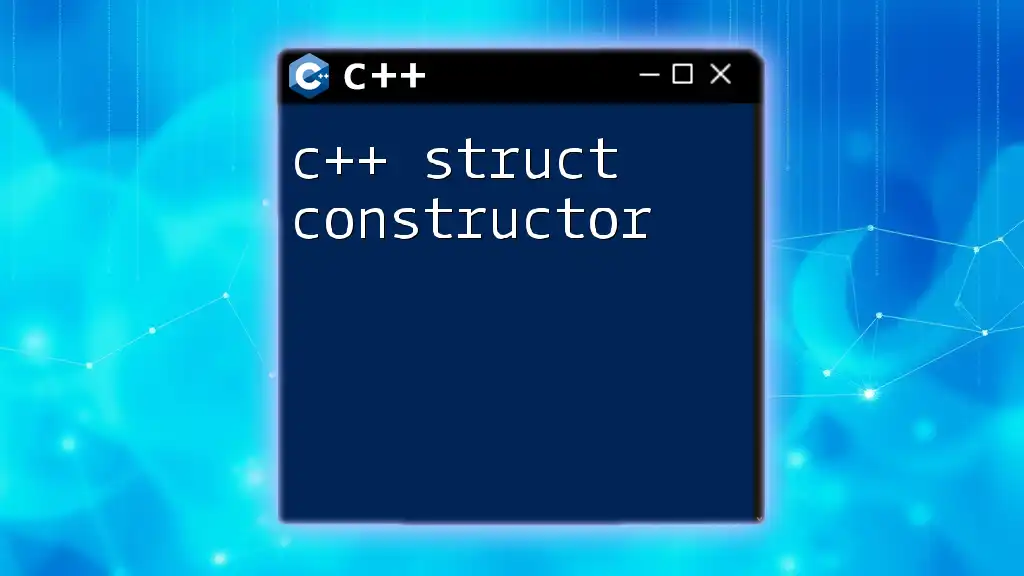
Typedef in C++
What is Typedef?
The `typedef` keyword in C++ is used to create an alias for existing data types. It improves code readability and can make complex data types easier to manage. Although `typedef` is not exclusive to structs, it plays a significant role when structuring data types, particularly when defining data types that are large or intricate.
Declaring a Typedef Struct
When you use `typedef` with `struct`, you simplify how you refer to that structure. Consider the following example:
typedef struct {
std::string name;
int age;
} Person;
In this case, you can directly use `Person` as a new type without having to prepend `struct` each time you declare a variable of that type. This enhances the code's readability as struct usage becomes intuitive.
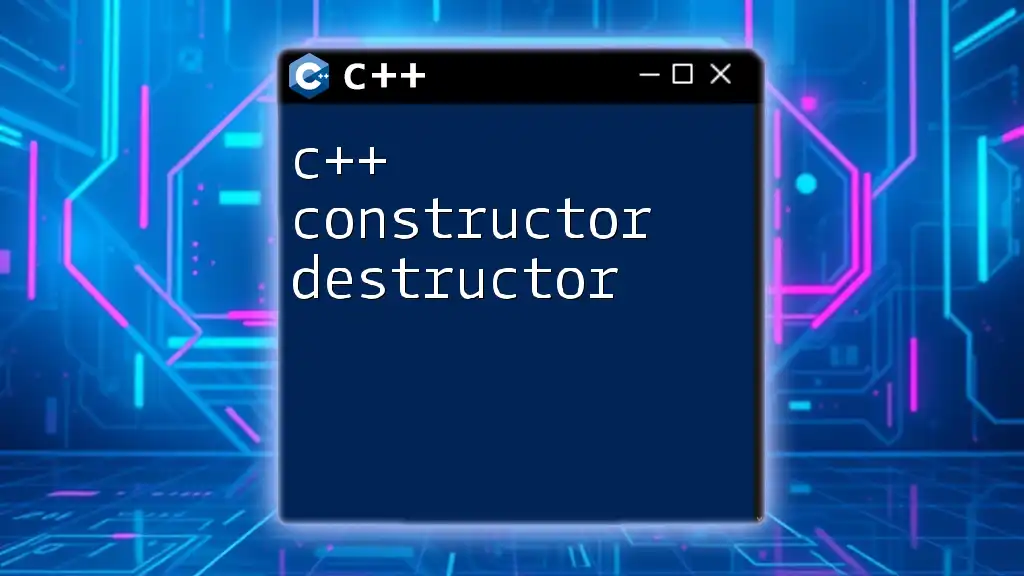
Differences Between Struct and Typedef Struct
Basic Differences
The primary difference between a `struct` and a `typedef struct` lies in how you declare and use them. A plain `struct` requires you to always prepend `struct` when you are declaring new objects, whereas a `typedef struct` allows you to avoid this, making your code cleaner.
Additionally, defining a structure using `typedef` can indirectly contribute to better code maintainability and readability, especially in larger programs.
Memory and Performance Implications
When it comes to memory and performance, both `struct` and `typedef struct` have no significant differences. They occupy the same memory and perform equivalently because the typedef is merely an alias. However, the ease of declaration with `typedef` may lead to less verbose code.
Best Practices
Choosing between `struct` and `typedef struct` often depends on personal or team coding styles. Here are some guidelines:
- For simpler structures that are used briefly, you might opt for `struct` as it is straightforward and recognizable.
- Use `typedef struct` when your structures become more complex or when you find yourself repeatedly referencing the same structure; it can streamline your code and avoid redundancy.
It’s essential to strike a balance for code clarity and maintainability. Remembering to use descriptive names for your structs and typedefs will aid other developers (or yourself in the future) in understanding the code more quickly.
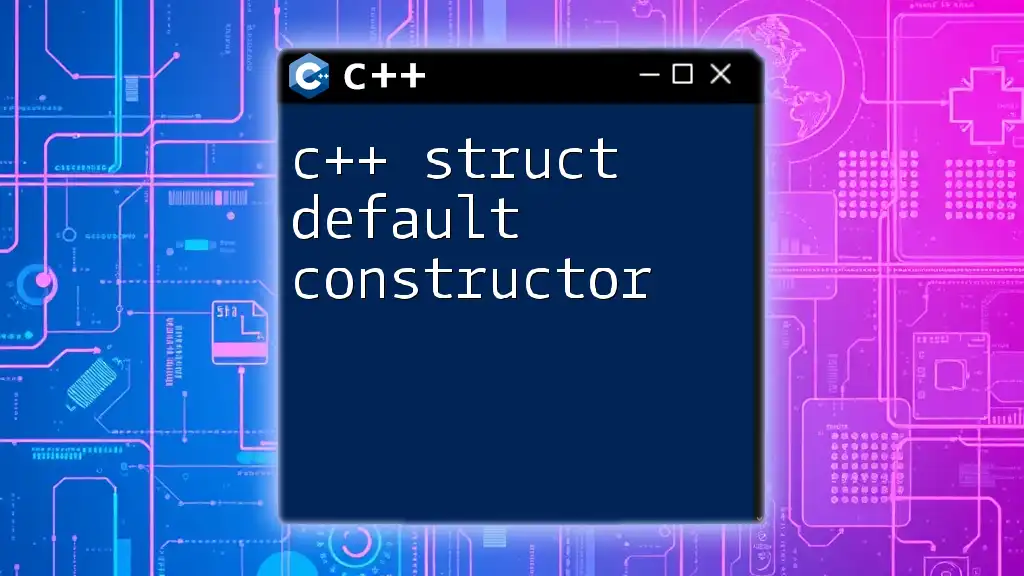
When to Use Each
When to Use Struct
Using a plain `struct` can be beneficial when the data is simple and the struct is used in a limited scope. For example, if you have a structure that represents basic information about a person:
struct Person {
std::string name;
int age;
};
When the program is small, and this `struct` doesn't get used in many other places, the straightforward declaration suffices.
When to Use Typedef Struct
On the other hand, if a struct has multiple instances throughout your code, or if it contains nested structures, using `typedef` can greatly enhance comprehension:
typedef struct {
std::string name;
int age;
} Person;
typedef struct {
Person owner;
std::string make;
std::string model;
} Car;
By using `typedef`, references to the `Person` struct become less verbose, thus making the structure of the code clearer.
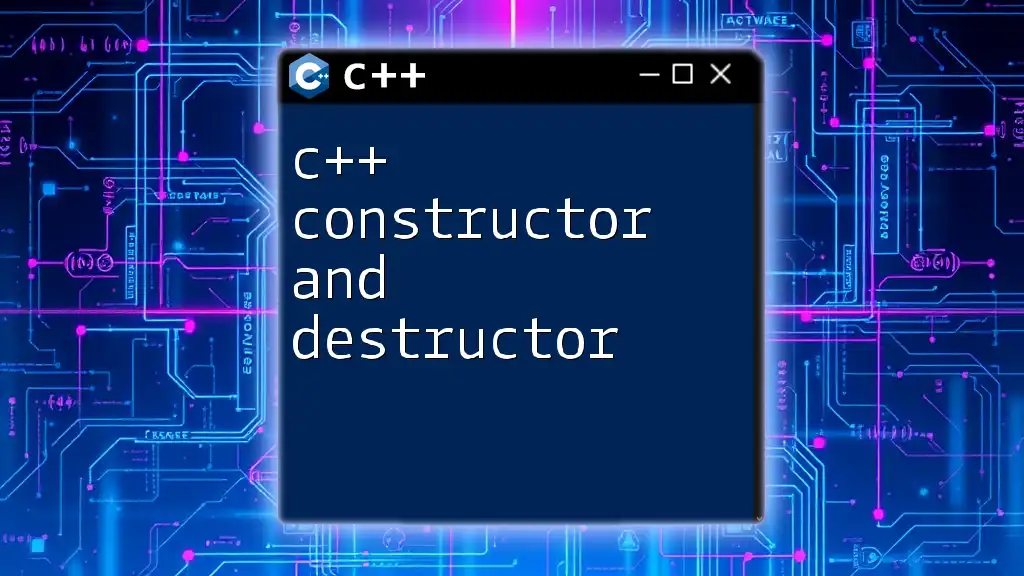
Additional Considerations
C++11 and Beyond
With advancements in C++11 and later versions, new features like `using` can be employed instead of `typedef`. This is a modern syntax that tends to be more readable:
using Person = struct {
std::string name;
int age;
};
This could serve the same purpose as `typedef`, while remaining clear and concise.
Common Mistakes and FAQs
New developers often grapple with a few persistent misconceptions regarding `typedef` and structs:
- Common misunderstanding is whether `typedef` allocates memory. The answer is, no, typedefs themselves don’t consume memory; they merely define how a data type is referenced.
- A frequently asked question is "Is `typedef` necessary in C++?" While it's not strictly necessary, it can significantly improve code readability in complex projects.
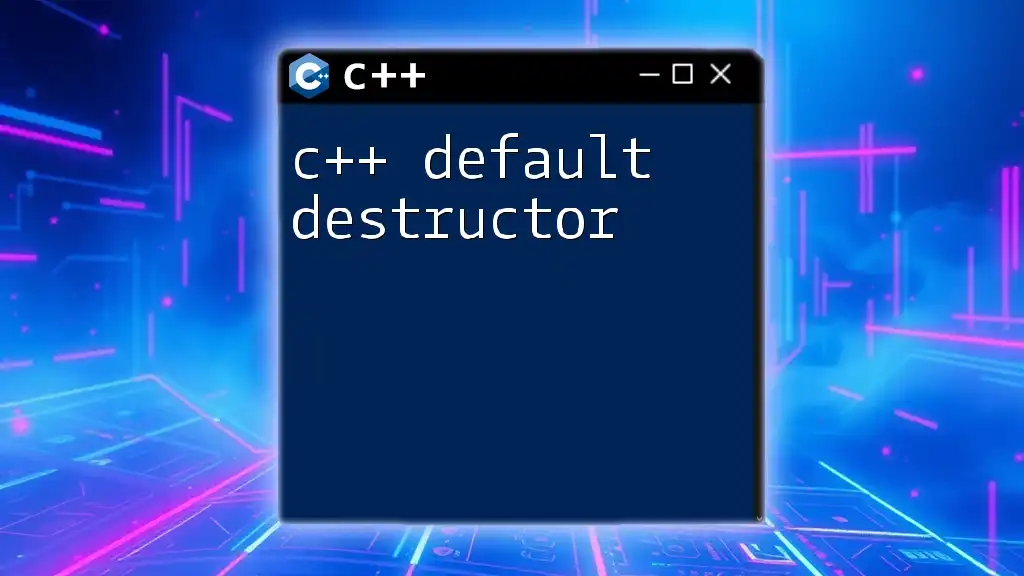
Conclusion
In summary, understanding the differences between c++ struct vs typedef struct is vital as it shapes how we design our programs. Each approach serves unique purposes and has its own set of advantages. By weighing readability and maintainability against simplicity and comprehensibility, you can choose the best approach for your specific case.
Embracing both `struct` and `typedef struct` effectively can enhance your C++ coding skills and liberate your programming style. Now, it's time to dive into coding and put these principles into action!