In C++, a struct can have a default constructor that automatically initializes its members, allowing for clean object creation without the need for explicit initialization.
Here's a simple example:
struct Point {
int x;
int y;
// Default constructor
Point() : x(0), y(0) {} // Initializes x and y to 0
};
// Usage
Point p; // p.x and p.y are both 0
Understanding Structs in C++
What is a Struct?
In C++, a struct is a user-defined data type that allows developers to group variables of different types under a single name. They are particularly useful for organizing related data, such as attributes of an entity.
Structs differ slightly from classes in C++. While both can encapsulate data and functions (methods), the key distinction lies in the default access level: members of a struct are public by default, whereas members of a class are private. This makes structs ideal for simple data structures without the need for complex access control.
Common use cases for structs include representing records or modeled entities like points, rectangles, and other geometric figures, where grouping of data is essential.
Syntax of a Struct
The syntax for declaring a struct in C++ is straightforward. Here’s a simple example:
struct Person {
std::string name;
int age;
};
In this example, we define a struct named `Person` with two member variables: `name` and `age`. Each `Person` struct can hold values for these variables.
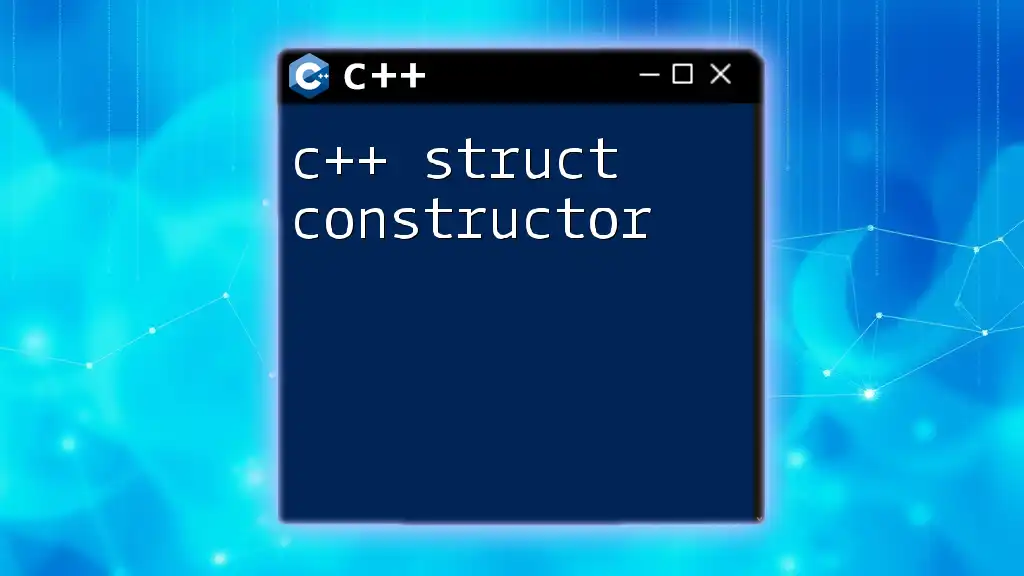
What is a Constructor?
Definition of a Constructor
A constructor is a special member function of a class or struct that initializes objects of that type. When an object is created, the constructor is automatically invoked to set up its initial state. Unlike regular member functions, constructors do not have a return type and have the same name as the struct or class they belong to.
Types of Constructors
In C++, constructors can be classified into several types:
- Default Constructors: No parameters or all parameters have default values.
- Parameterized Constructors: Take parameters to assign initial values.
- Copy Constructors: Used to create a copy of an object from another instance of the same class or struct.
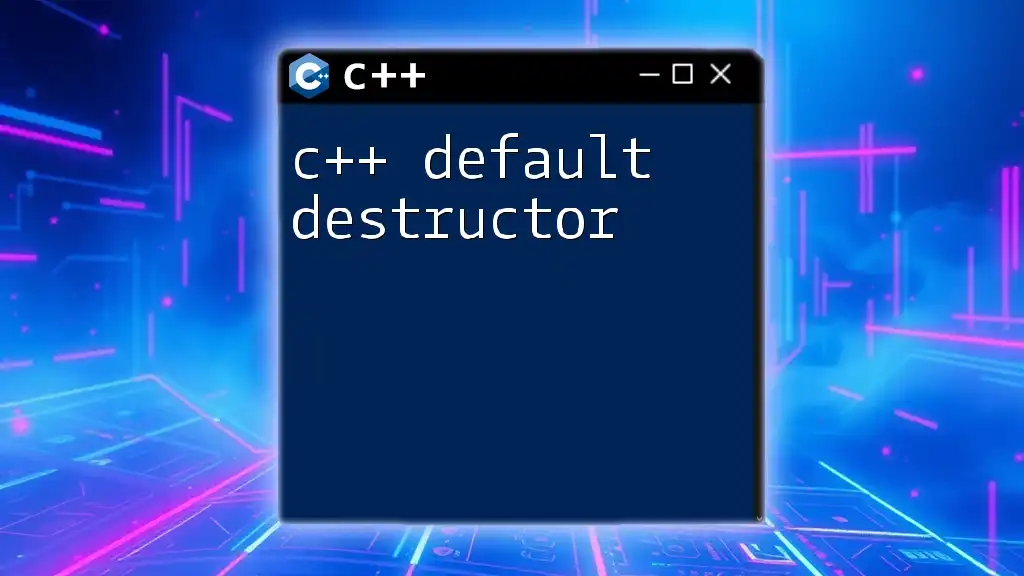
Default Constructor in C++
What is a Default Constructor?
A default constructor is a constructor that either has no parameters or all parameters have default values. Its primary function is to create an object and initialize its members when no specific values are provided during creation.
Role of the Default Constructor
The default constructor plays a crucial role in providing initial values to member variables. If a struct does not explicitly declare any constructor, C++ automatically supplies an implicit default constructor. However, if any constructor is defined, the implicit default constructor is no longer provided unless explicitly defined by the programmer.
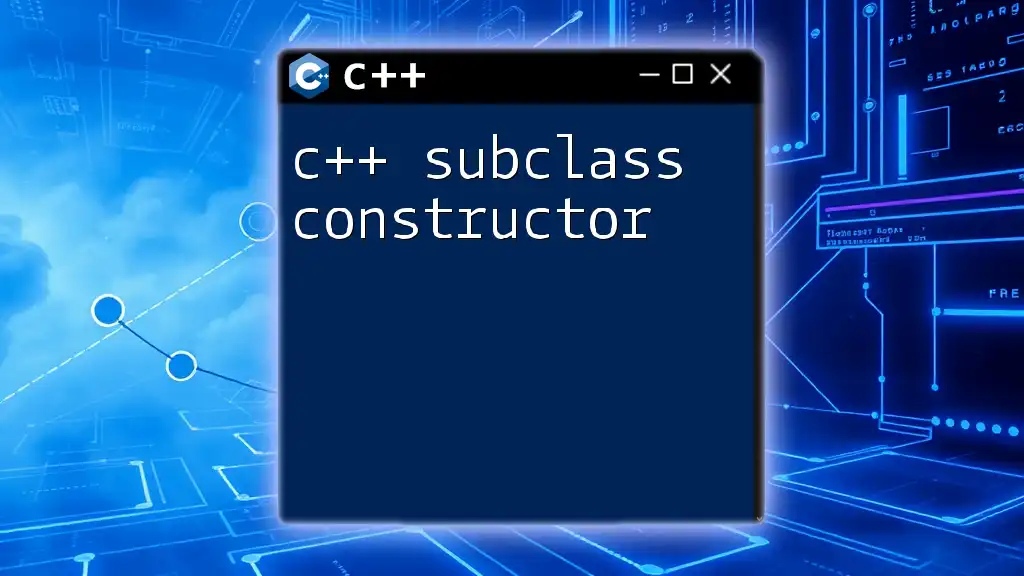
Creating a Default Constructor
Declaring a Default Constructor in a Struct
To define a default constructor in a struct, simply write the constructor as a regular member function inside the struct. Here's how you can create a `Person` struct with a default constructor:
struct Person {
std::string name;
int age;
Person() { // Default constructor
name = "Unknown";
age = 0;
}
};
In this example, the default constructor initializes the `name` to `"Unknown"` and `age` to `0`. This way, every new `Person` object gets a clear starting state.
Demonstrating the Default Constructor
Creating an instance of the struct with the default constructor is straightforward:
int main() {
Person p; // Default values applied
std::cout << "Name: " << p.name << ", Age: " << p.age << std::endl;
return 0;
}
When you run this code, it assigns `p.name` as "Unknown" and `p.age` as `0`, thereby demonstrating how the default constructor initializes member variables.
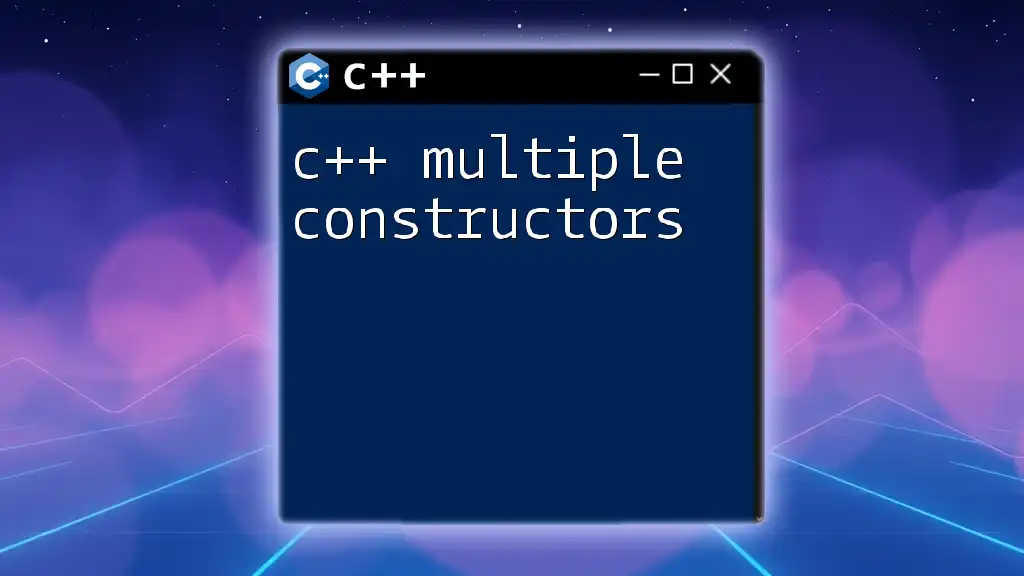
Advantages of Using a Default Constructor
Simplified Object Creation
The primary advantage of using a default constructor is that it significantly reduces boilerplate code. Programmers do not need to manually set each member variable every time they create a new object, making the code more concise and readable.
Automatically Setting Default Values
Default constructors ensure that member variables are initialized with predetermined values, thus avoiding undefined behavior in your program. For instance, if you forgot to initialize `name` and `age`, their values would remain undetermined, leading to bugs or unexpected behavior.
struct Person {
std::string name;
int age; // Potential for uninitialized data without a default constructor
};
In the above scenario, creating an object without a defined constructor could result in unpredictable values, underscoring the value of having a default constructor.
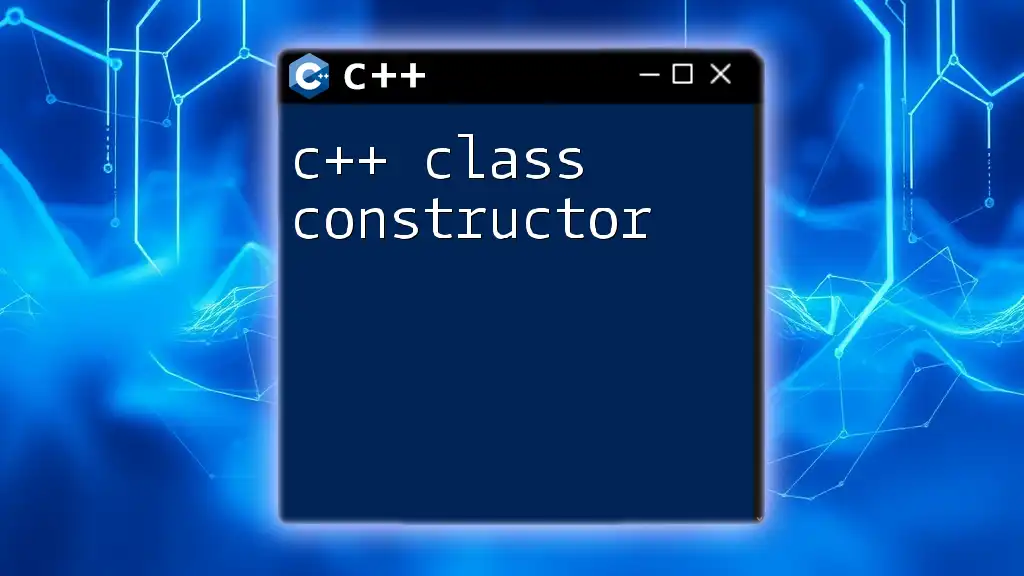
Common Pitfalls & Best Practices
Avoiding the Use of Uninitialized Values
Not providing a default constructor can lead to uninitialized variables, posing significant risks. Always ensure that the default constructor initializes all member variables appropriately.
When to Define a Default Constructor
You should define a default constructor if:
- The struct has member variables that require initialization.
- You want to provide a consistent state when creating instances of the struct.
Conversely, if your struct primarily serves as a data holder without further responsibilities, and all members are guaranteed to be initialized through other means, you may opt to omit the default constructor.
Overloading Default Constructors
It is possible to provide multiple constructors within the same struct, allowing for various ways to create instances. Here's an example that includes both a default and a parameterized constructor:
struct Person {
std::string name;
int age;
Person() : name("Unknown"), age(0) {} // Default constructor
Person(std::string n, int a) : name(n), age(a) {} // Parameterized constructor
};
In this example, the struct can now be initialized either with default values or with specified values—providing flexibility to the user while maintaining clarity in the struct’s behavior.
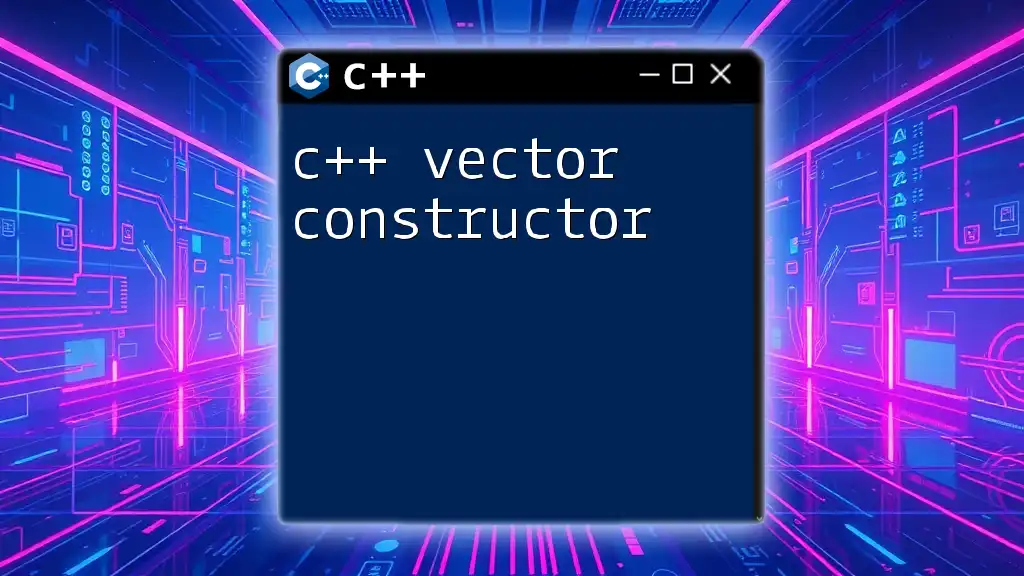
Conclusion
The C++ struct default constructor is a fundamental concept critical to ensuring proper initialization and functionality of structs in your programs. By utilizing default constructors, you can streamline the creation process and avoid various pitfalls associated with uninitialized values. Practicing with examples and experimenting with constructors will enrich your understanding and enhance your C++ skills.
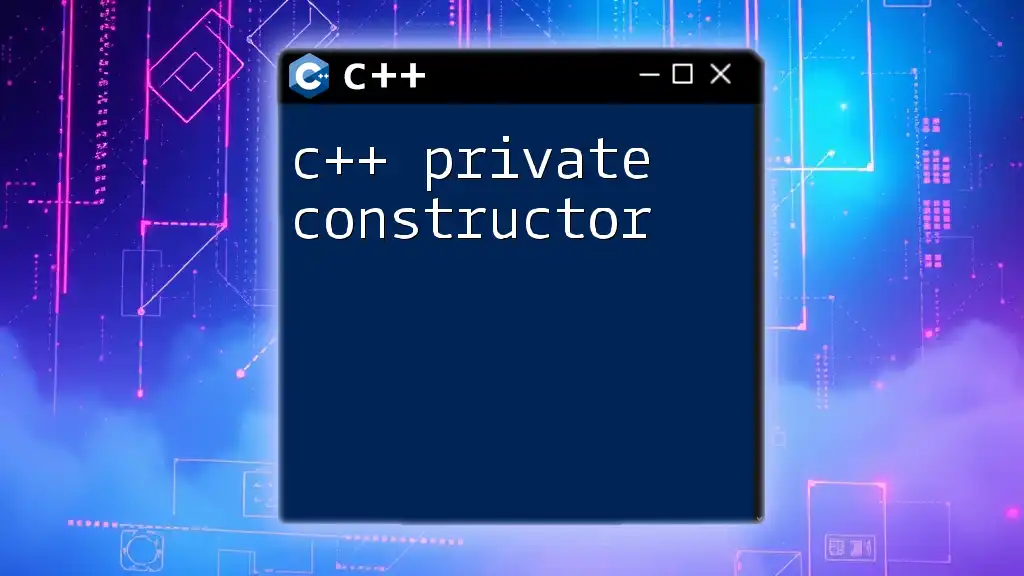
Additional Resources
For further reading, you may want to explore additional C++ programming tutorials that delve into fundamental topics such as classes, inheritance, and object-oriented programming principles.
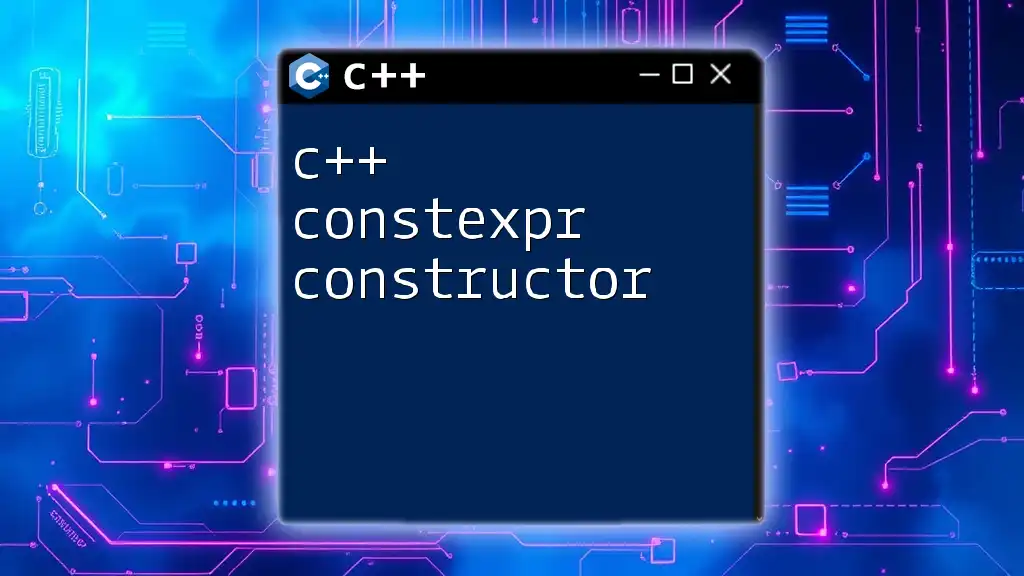
FAQs
-
What happens if I don't define a default constructor?
If you do not define a default constructor, C++ will provide an implicit one unless any constructor is defined. If your struct includes member types that lack default constructors, compilation errors may occur. -
Can structs have multiple constructors?
Yes, structs can have multiple constructors, including default and parameterized constructors, allowing for versatile object initialization. -
How do default constructors affect object copying?
A default constructor is used when creating new instances. Its behavior determines how member variables are initialized during copy operations, sometimes in conjunction with copy constructors (if defined).